Have you ever wondered how to split a JavaScript array into chunks? If you are working with large arrays, you might want to divide them into smaller pieces for easier processing. But how can you do that without losing any data or messing up the order of the elements?
When dealing with extensive data sets in JavaScript programming, effectively managing arrays becomes a crucial challenge. This is where the technique of an array being split into chunks comes to the rescue.
In this blog post, I will show you:
- The basics of splitting the array into chunks
- How to use the slice() method to create subarrays of a given size
- Split an array into a specific size
- Group array items into pairs
- Chunk array elements based on values
- Chunk array elements based on certain conditions
- How to use ES6 methods for array chunking
Let’s explore this in detail.
JavaScript split array into chunks
The process of splitting an array into chunks involves dividing a larger array into smaller, more manageable segments. By using this technique, developers can enhance data processing, memory efficiency, and code readability. It enables tasks like pagination and data visualization to be performed more effectively.
For instance, when an array is divided into chunks, such as splitting an array into chunks of a specific size, it becomes easier to process and manipulate data while maintaining optimal performance and organization.
Let’s explore splitting an array into chunks with a few code examples.
1. JavaScript split array into chunks of n
One fundamental requirement is to divide an array into equal chunks. JavaScript provides various techniques to achieve this, such as using the JavaScript slice() method in conjunction with loops to iterate through the array and create equal-sized chunks
.
Here is an example of a split array into chunks of size n:
// Splitting an array into equal chunks
function chunkArray(arr, chunkSize) {
const chunkedArray = [];
for (let i = 0; i < arr.length; i += chunkSize) {
chunkedArray.push(arr.slice(i, i + chunkSize));
}
return chunkedArray;
}
const originalArray = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const chunkSize = 3;
const chunks = chunkArray(originalArray, chunkSize);
console.log(chunks);
// Output: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
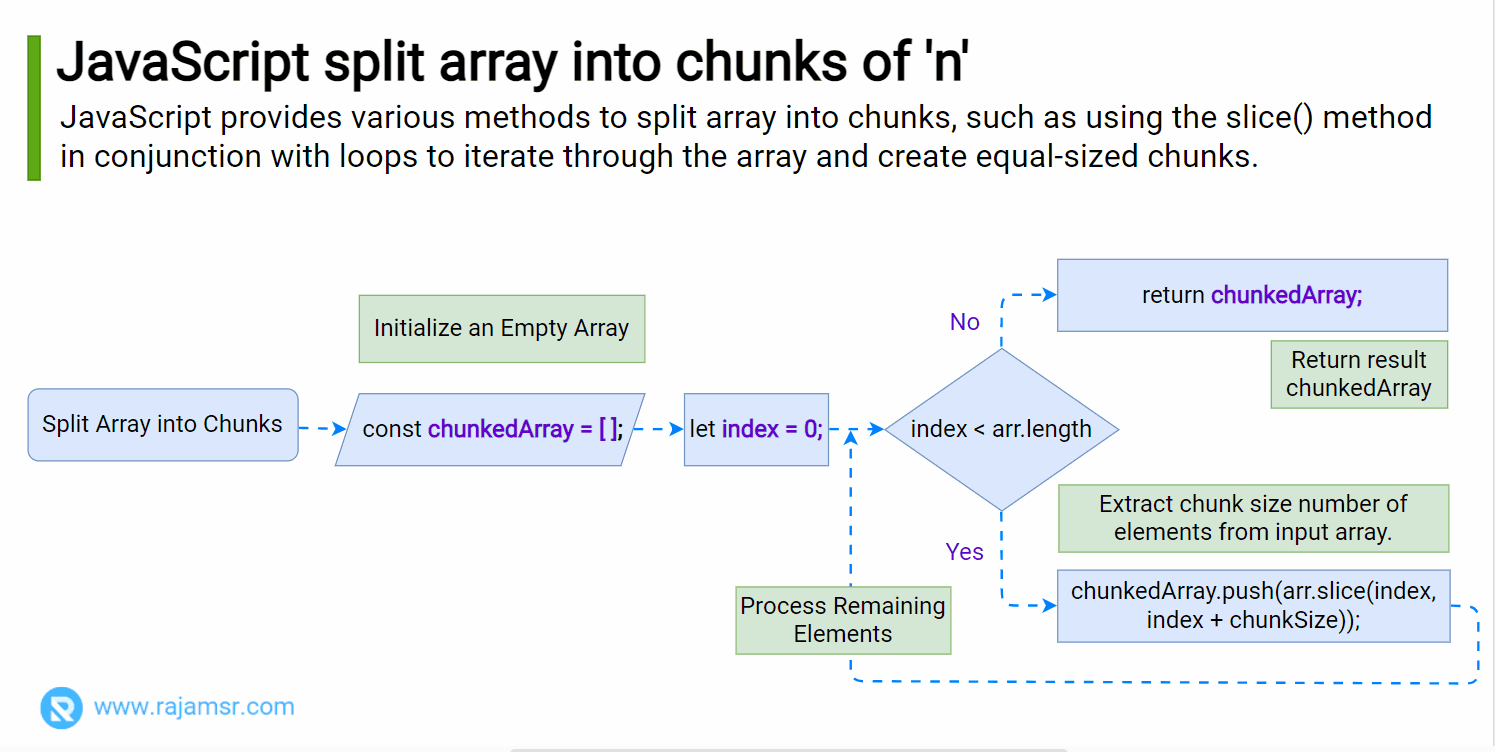
slice()
, it creates equal-sized chunks.For instance, if the original array is [1, 2, 3, 4, 5, 6, 7, 8, 9]
and chunkSize
is 3, the output will be [[1, 2, 3]
, [4, 5, 6]
, [7, 8, 9]]
.2. Split an array into chunks with a specified size
In scenarios where you need custom-sized chunks instead, you can modify the chunkArray()
function to create chunks of a specific size ‘n’.
This approach is particularly useful when you want to split an array into chunks of size n.
// Creating custom-sized chunks
function chunkArray(arr, chunkSize) {
const chunkedArray = [];
for (let index = 0; index < arr.length; index += chunkSize) {
chunkedArray.push(arr.slice(index, index + chunkSize));
}
return chunkedArray;
}
const originalArray = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const chunkSize = 4;
const chunks = chunkArray(originalArray, chunkSize);
console.log(chunks);
// Output: [[1, 2, 3, 4], [5, 6, 7, 8], [9]]
This JavaScript function, named chunkArray()
, takes an array and a chunk size as input. It divides the input array into smaller arrays of the specified chunk size and returns them as a new array called chunkedArray
.
This example splits the originalArray
into chunks of size 4 and outputs the result [[1, 2, 3, 4], [5, 6, 7, 8], [9]] to the console.
3. JavaScript group array into chunks
An interesting use case is when you need to pair consecutive array items. JavaScript offers a concise solution using the same chunkArray
function.
Let’s see an example of splitting an array into chunks of two:
// Pairing consecutive array items
function chunkArray(arr, chunkSize) {
const chunkedArray = [];
for (let index = 0; index < arr.length; index += chunkSize) {
chunkedArray.push(arr.slice(index, index + chunkSize));
}
return chunkedArray;
}
const originalArray = ['a', 'A', 'b', 'B', 'c', 'C'];
const chunkSize = 2;
const pairs = chunkArray(originalArray, chunkSize);
console.log(pairs);
// Output: [['a', 'A'], ['b', 'B'], ['c', 'C']]
chunkArray
, pairs consecutive items from the input array by dividing them into chunks of the specified chunkSize
.It iterates through the array, using the slice()
method to extract chunks. When applied to [‘a’, ‘A’, ‘b’, ‘B’, ‘c’, ‘C’] with chunkSize
2, it outputs [[‘a’, ‘A’], [‘b’, ‘B’], [‘c’, ‘C’]].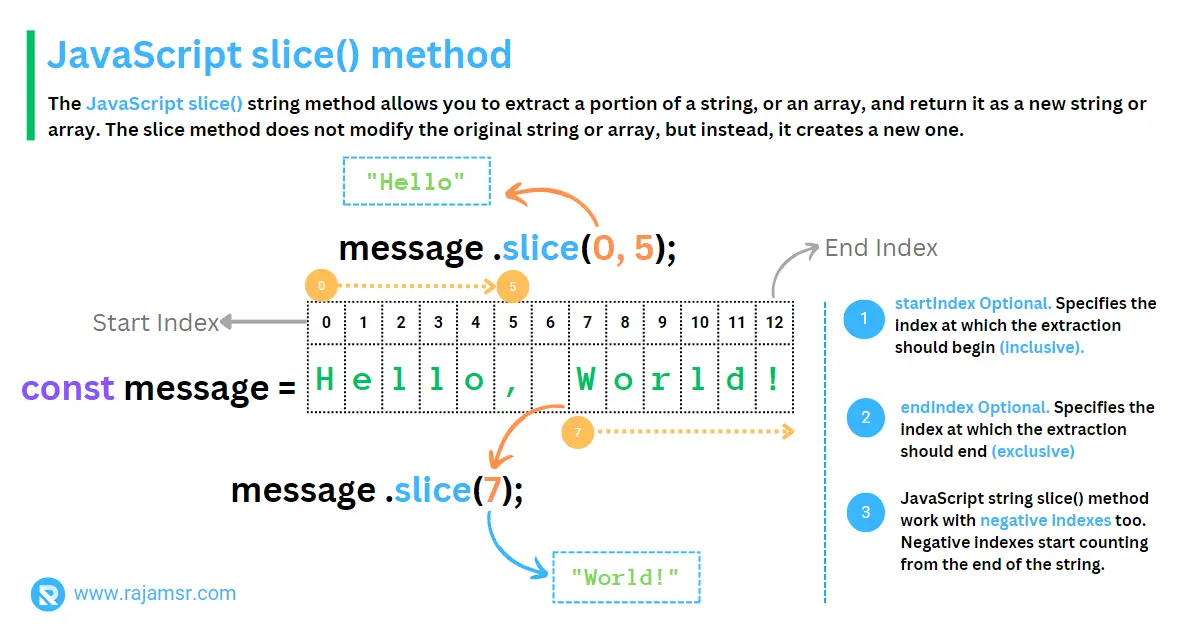
4. Split array into chunks based on value
For situations where you need to split array into chunks based on value, the filter()
function becomes instrumental.
// Splitting array based on specific values
function chunkArrayByValue(arr, value) {
return arr.reduce((acc, current) => {
if (current === value) {
acc.push([]);
}
acc[acc.length - 1].push(current);
return acc;
}, [[]]);
}
const originalArray = [1, 2, 2, 3, 2, 4, 5, 2, 6];
const valueToChunkBy = 2;
const valueChunks = chunkArrayByValue(originalArray, valueToChunkBy);
console.log(valueChunks);
// Output: [[1], [2, 2], [3], [2], [4, 5], [2], [6]]
This JavaScript function chunkArrayByValue()
takes an array and a specific value as inputs. It uses the array reduce() method to split the array into sub-arrays based on occurrences of the specified value. Each sub-array contains consecutive elements, and a new sub-array is started whenever the value appears.
The example demonstrates using this function to split the originalArray
based on the value 2, resulting in the displayed output [[1], [2, 2], [3], [2], [4, 5], [2], [6]].
5. Split array into chunks with ES6 method
The introduction of ES6 revolutionized JavaScript and brought forth features that simplify array operations, including array chunking.
// Advanced array chunking with ES6
function chunkArrayES6(arr, chunkSize) {
return Array.from({ length: Math.ceil(arr.length / chunkSize) }, (_, index) =>
arr.slice(index * chunkSize, index * chunkSize + chunkSize)
);
}
const originalArray = [10, 20, 30, 40, 50, 60];
const chunkSize = 2;
const es6Chunks = chunkArrayES6(originalArray, chunkSize);
console.log(es6Chunks);
// Output: [[10, 20], [30, 40], [50, 60]]
This JavaScript function chunkArrayES6()
uses ES6 features to split an array into chunks of a specified size. It utilizes the Array.from()
method with the slice() function to create an array of sub-arrays.
The example demonstrates splitting originalArray
into chunks of size 2, resulting in es6Chunks
output [[10, 20], [30, 40], [50, 60]].
6. Splitting array into chunks based on condition
In scenarios that demand splitting arrays based on a condition, leveraging the reduce()
and JavaScript array push() functions is a powerful approach.
// Example: splitting array based on a condition
function chunkArrayByCondition(arr, condition) {
return arr.reduce((acc, current) => {
if (condition(current)) {
acc.push([]);
}
acc[acc.length - 1].push(current);
return acc;
}, [[]]);
}
const originalArray = [15, 25, 5, 30, 10, 40];
const threshold = 20;
const conditionChunks = chunkArrayByCondition(originalArray, value => value > threshold);
console.log(conditionChunks);
// Output: [[15, 25], [5], [30], [10, 40]]
Inside the reduce()
loop, the condition (current) is evaluated for each element current in the array. If the condition is met (in this case, if the element is greater than the threshold), a new empty array is pushed into the accumulator variable acc
. This creates a new chunk to hold the subsequent elements that satisfy the condition.
After the condition check, the current element is added to the last chunk created in the accumulator variable acc
. This builds the chunks based on the condition.
7. Practical use case
Array chunking finds applications in various real-world scenarios. For instance, when dealing with large datasets, chunking allows you to process data in smaller portions, preventing memory and performance issues. In UI development, array chunking helps with pagination, loading data in manageable chunks for smoother user experiences.
Dealing with large datasets
Imagine you’re working on a web application that needs to process a large dataset retrieved from a database or an API. If you try to process the entire dataset at once, you might encounter memory and performance issues. By using array chunking, you can break down the dataset into smaller portions, allowing you to process each chunk individually without overwhelming system resources.
Let’s see how this works with a JavaScript code example:
function processChunkedData(data, chunkSize) {
for (let i = 0; i < data.length; i += chunkSize) {
const chunk = data.slice(i, i + chunkSize);
// Process the current chunk, e.g., perform calculations, transformations, etc.
console.log(`Processing chunk ${i / chunkSize + 1}:`, chunk);
}
}
// Generating an array of numbers from 0 to 999
const largeDataset = [...Array(1000).keys()];
const chunkSize = 50;
processChunkedData(largeDataset, chunkSize);
processChunkedData()
function takes a large dataset and a chunkSize
as parameters. It iterates through the dataset in chunks of the specified size, allowing you to perform necessary operations on each smaller portion.Best practices for efficient array chunking
- Choose the Right Chunk Size: Select a suitable chunk size for a balance between memory usage and performance optimization.
- Handle Errors: Address edge cases, including empty arrays, while implementing your array chunking logic.
- Modular Code: Encapsulate array chunking logic in dedicated functions for better code organization and reusability.
- Optimize Performance: Prioritize memory efficiency, especially for large arrays, and employ techniques that minimize unnecessary overhead.
Conclusion
Master the art of splitting an array into chunks in JavaScript, unlocking a potent skill to amplify data processing capabilities.
Whether splitting arrays evenly, forming custom groups, pairing elements, or condition-based chunking, these techniques empower you to conquer diverse scenarios adeptly.
Optimize code for performance, tailor methods to your project’s needs, and witness applications gain responsiveness and efficiency.
Over to you: Which method are you going to use to split an array into chunks?