If you are looking for a solution to store your key-value pairs, like settings and lookup data, then a JavaScript dictionary is the right choice for you!
The dictionary is a data structure that stores data in key-value pairs. It is used to store and efficiently retrieve data. It allows you to store and retrieve data using key-value pairs. In contrast to arrays, which store data in a linear order, dictionaries in JavaScript allow you to access data using a unique key rather than an index. This makes dictionaries ideal for storing data that must be accessible quickly and readily, such as a user database or a product list.
In this blog post, we will explore the following:
- Create a dictionary in three different ways
- Add key-value pair to the dictionary
- Update value for a key
- Accessing items from the dictionary
- Iterating over a dictionary with three different approaches
- Get all dictionary keys
- Get all values from a dictionary
- Get the length of dictionary keys
- Check whether a key exists in the dictionary
- Delete an item from the dictionary
Let’s dive deep.
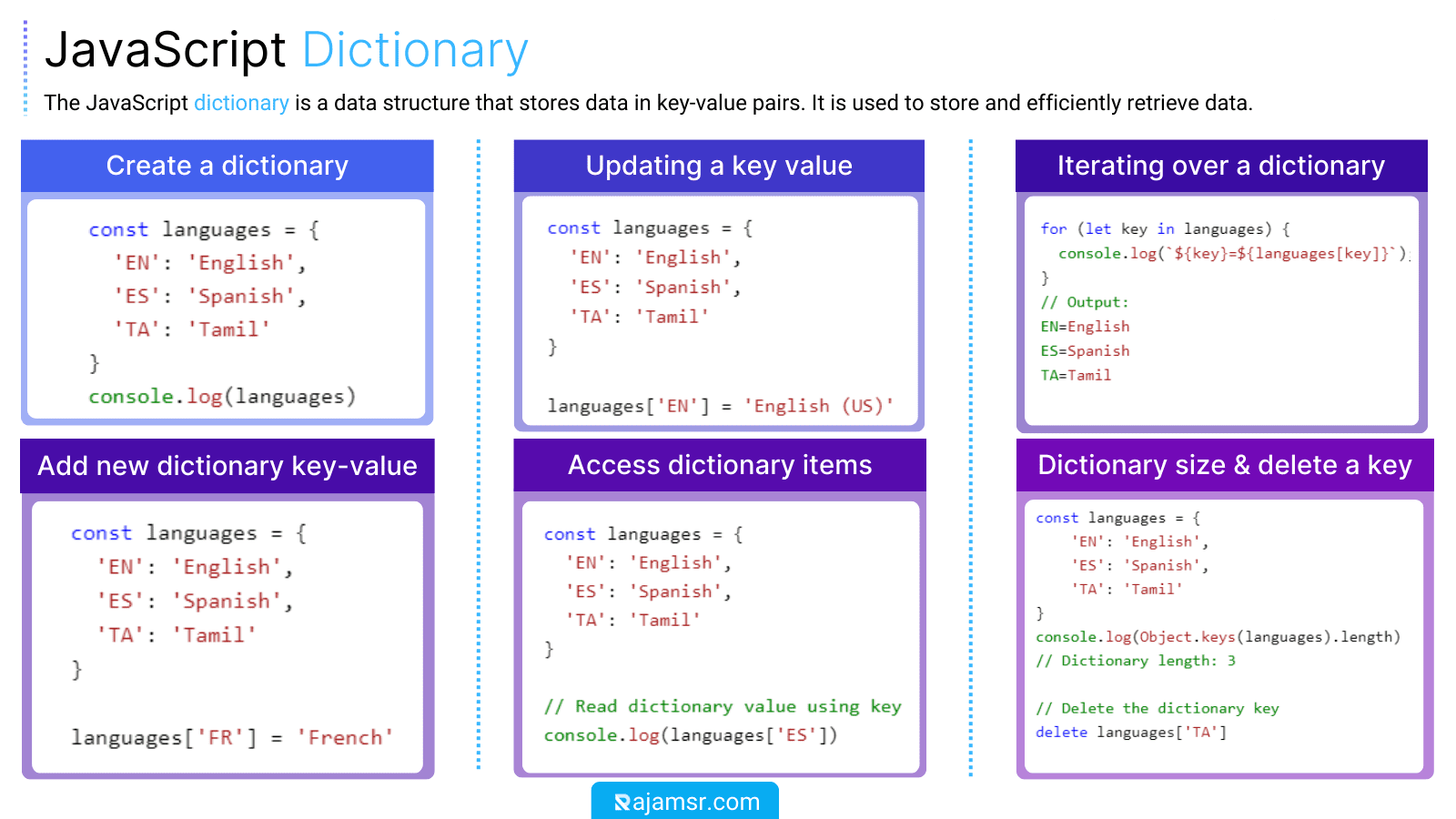
Creating a Dictionary in JavaScript
Unlike other strongly typed programming languages like C# and JavaScript, JavaScript does not have any native data types of dictionary. However, we can create custom dictionary (dict) kinds of JavaScript objects. The dictionary allows you to store data in key-value format.
There are multiple ways to create a dictionary in JavaScript. In this blog post, we will see three different approaches:
1. Using the object literal notation
key-value
pairs to it by using the object literal syntax.Here is a dictionary example:const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
console.log(languages)
// Output: { EN: 'English', ES: 'Spanish', TA: 'Tamil' }
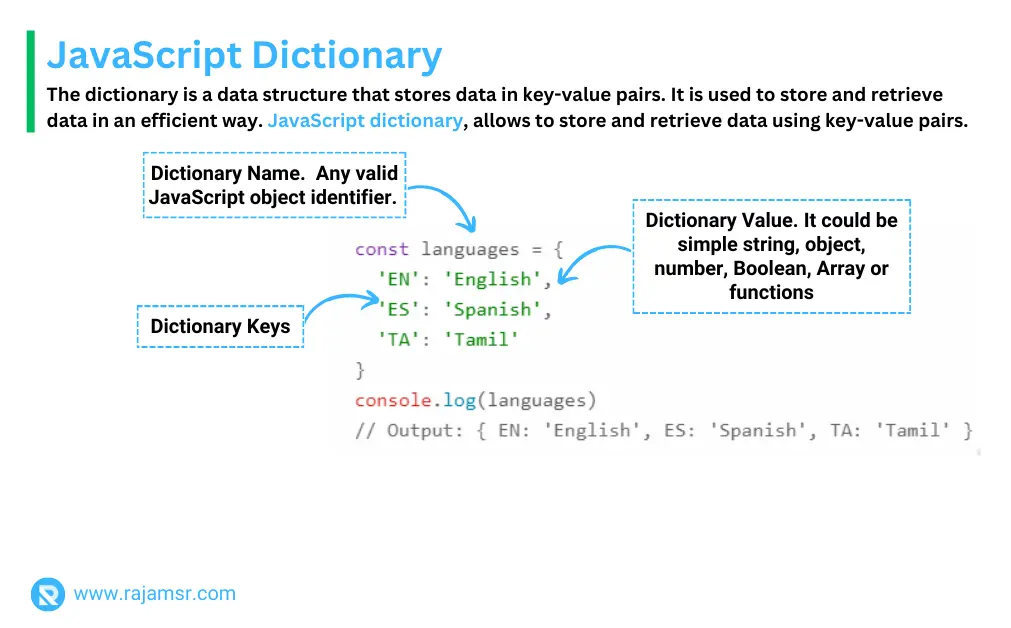
2. Create dictionary in JavaScript using the Map() object
Map()
is one of the non-primitive data types in JavaScript. Using the Map()
object you can create a dictionary that can hold any type of value. You can create an empty map by using the new Map()
. You can add key-value pairs to the map by using the set() method. For example, create a dictionary using the Map()
object:const languages = new Map()
languages.set('EN', 'English');
languages.set('ES', 'Spanish');
languages.set('TA', 'Tamil');
Not just with JavaScript string, you can use any type as the value in the dictionary as shown in the following JavaScript dictionaries example:
// Empty dictionary object
let lookupData = {}
// Dictionary with single key-value pair
let name = { "name": "John Doe" }
// Dictionary with multiple key-value pairs
let person = { "name": "John Doe", "age": 25 }
// Dictionary with numeric keys
let days = { 1: "Monday", 2: "Tuesday", 3: "Wednesday" }
// Dictionary with boolean values
let status = { "isActive": true, "hasAccess": false }
// Nested dictionary object
let people = { "person": { "name": "John Doe", "age": 25 } }
// Dictionary with array as value
let fruits = { "fruits": ["apple", "banana", "orange"] }
// Dictionary with function as value
let functions = { "calculate": function (a, b) { return a + b; } }
// Dictionary with undefined and null values
let edgeCases = { "name": undefined, "age": null }
3. Create a dict in JavaScript using Using The Object.create() method
This method allows you to create a dictionary by inheriting properties from another object.
For example:
let languages = Object.create({});
languages.EN = 'English';
languages.ES = 'Spanish';
languages.TA = 'Tamil';
console.log(languages)
Though we can create JavaScript dictionaries in different ways, I followed the first way to create a dictionary in this article. Let’s explore different JavaScript dictionary methods to manipulate it.
Adding key-value pair to a dictionary
So dictionary is created, let’s see how to add, modify and remove key-value pairs from the dictionary. The key names should be unique in the dictionary. Of course, key names are case-sensitive.
You can add items to a dictionary by using the square bracket notation. You can use the set()
method if you created your dictionary using the Map()
method.
For example, the following example shows how to add a new key-value pair using bracket notation:
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
languages['FR'] = 'French'
console.log(languages)
// Output: { EN: 'English', ES: 'Spanish', TA: 'Tamil', FR: 'French' }
You can use the following dot notations as well to add a key-value pair:
languages.FR = 'French'
Updating value of a dictionary key
You can update the value in a dictionary by using the square bracket or dot notation method. For example:
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
languages['EN'] = 'English (US)'
console.log(languages)
// Output: { EN: 'English (US)', ES: 'Spanish', TA: 'Tamil' }
In this example, the “English” value is updated with the value “English (US)” using the unique key ‘EN’.
Access dictionary items in JavaScript
You can access dictionary-stored key-value pairs by using the square bracket notation, the get()
method (if created using the Map()
method), the for...in
loop, or the for...of
loop.
The following example shows how to get the value of a key in an object:
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
// Read dictionary value using key
console.log(languages['ES'])
// Output: "Spanish"
You can use the following dictionary methods and properties to manipulate dictionary object.
Iterating over a dictionary
Because the dictionary is built with objects, we can quickly iterate through its keys. There are many ways to iterate over the dictionary object. Each method has its own set of benefits and drawbacks.
In this section, we will look at three methods for iterating through a dictionary object.
1. Using the for...in loop
This is one of the most common and easy ways to iterate over a dictionary. It allows you to access the keys of the dictionary, one at a time. Here is an example of how to use a for loop in a dictionary to get the key and value:
for (let key in languages) {
console.log(`${key}=${languages[key]}`);
}
// Output:
EN=English
ES=Spanish
TA=Tamil
2. Using the for...of loop
Object.entries()
is one of the object methods. Using this method along with the for...of
loop allows you to access the values of the dictionary as an array
. For example:
for (let key of Object.entries(languages)) {
console.log(key);
}
// Output:
[ 'EN', 'English' ]
[ 'ES', 'Spanish' ]
[ 'TA', 'Tamil' ]
3. Using the entries() method
Using the JavaScript Object.entries() method you to access the key-value pairs of the dictionary as shown in the following example:
for (let [key, value] of Object.entries(languages)) {
console.log(`${key}=${value}`);
}
// Output:
EN=English
ES=Spanish
TA=Tamil
Get all dictionary keys and print
Keys are the primary means of accessing values in a JavaScript dictionary object. Dictionary keys can be any valid JavaScript type, including strings, numbers, and symbols.
Here’s an example of how to create a dictionary object with string keys:
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
console.log(Object.keys(languages))
// Output: ["EN", "ES", "TA"]
In this example, the keys are ‘EN’, ‘ES’, and ‘TA’. The corresponding values are ‘English’, ‘Spanish’, and ‘Tamil’ for those keys. Using the JavaScript Object.keys() method, you can access all dictionary keys.
If you use a console.table()
instead of console.log()
, it will print the arrays in tabular format.
Get all dictionary values
To retrieve the values of a JavaScript dictionary object, you can use the Object.values() method. This method returns values as an array.
Here’s an example:
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
console.log(Object.values(languages));
// Output: [ 'English', 'Spanish', 'Tamil' ]
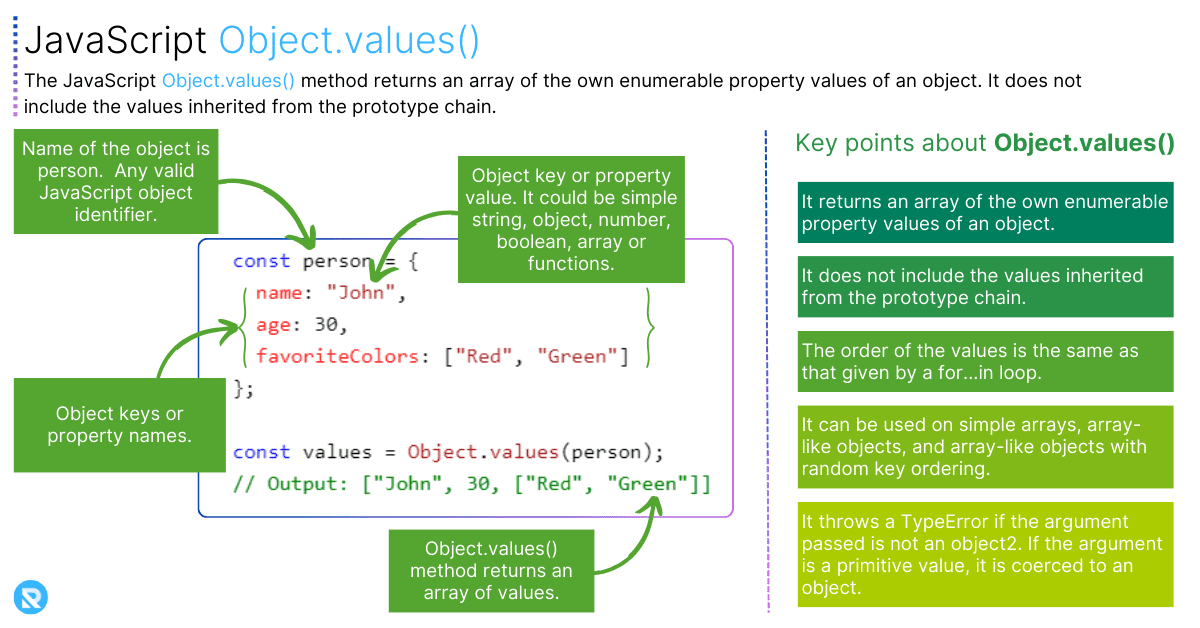
Get value from dictionary
Here is an example of get value for a key from dictionary:
console.log(languages['EN']);
// Output: "English"
JavaScript dictionary size or length of dictionary
The determine the dictionary JavaScript object length, you can use one of the object methods Object.keys()
or Object.values()
.
For example, if you are using JavaScript Object.keys() to determine the length, it will return keys as an array. Using the JavaScript array length property you can get the dictionary length.
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
console.log(Object.keys(languages).length)
// Output: 3
In this example, the Object.keys()
method returns an array of the keys in the dictionary object ('EN'
, 'ES'
, and 'TA'
). The length property of that array is used to determine the length of the dictionary object. In this case, there are three keys, so the length is 3.
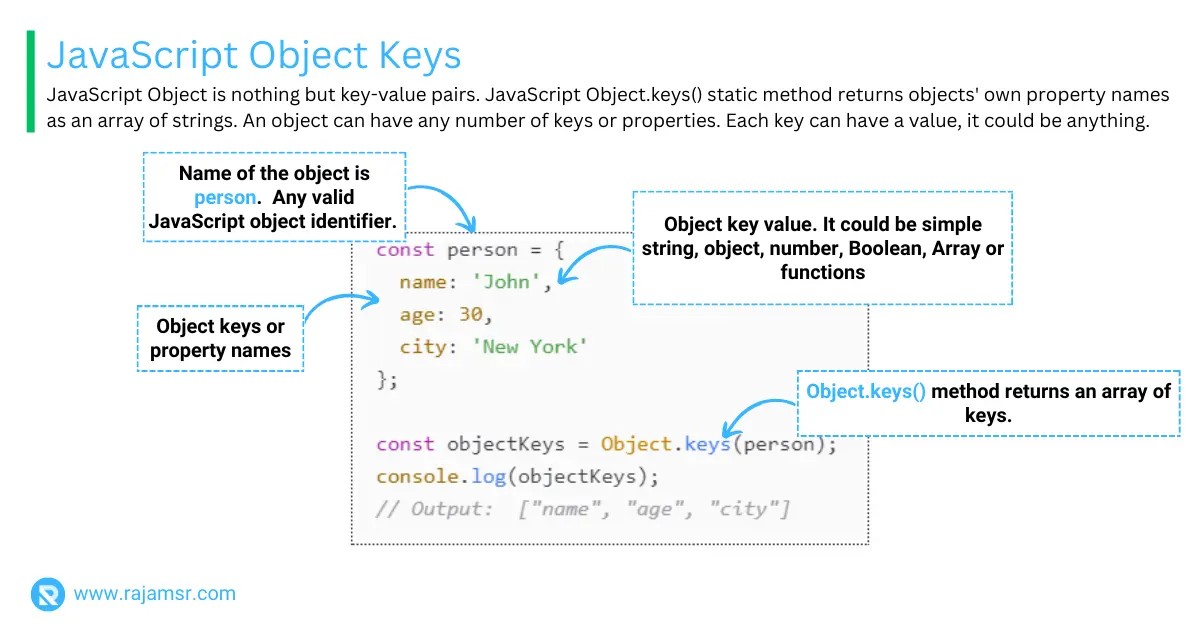
JavaScript dictionary contains key
To check if the dictionary key exists, you can use the includes()
or the hasOwnProperty() methods. Here’s an example of how to check if a key exists in the dictionary:
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
console.log(Object.keys(languages).includes('EN'));
// Output: true
console.log(Object.keys(languages).includes('en'));
// Output: false
In this example, the Object.keys()
method will return dictionary keys as an array. You can use the JavaScript includes() method to check if the 'EN'
keys exist in the dictionary object.
In JavaScript, string values are case-sensitive
. So the dictionary key 'en'
search returns false because the actual key is in capital letters.
There are two ways to search dictionary keys in a case-insensitive manner. Convert both keys and search text to JavaScript lowercase or convert the keys and text to JavaScript uppercase before searching.
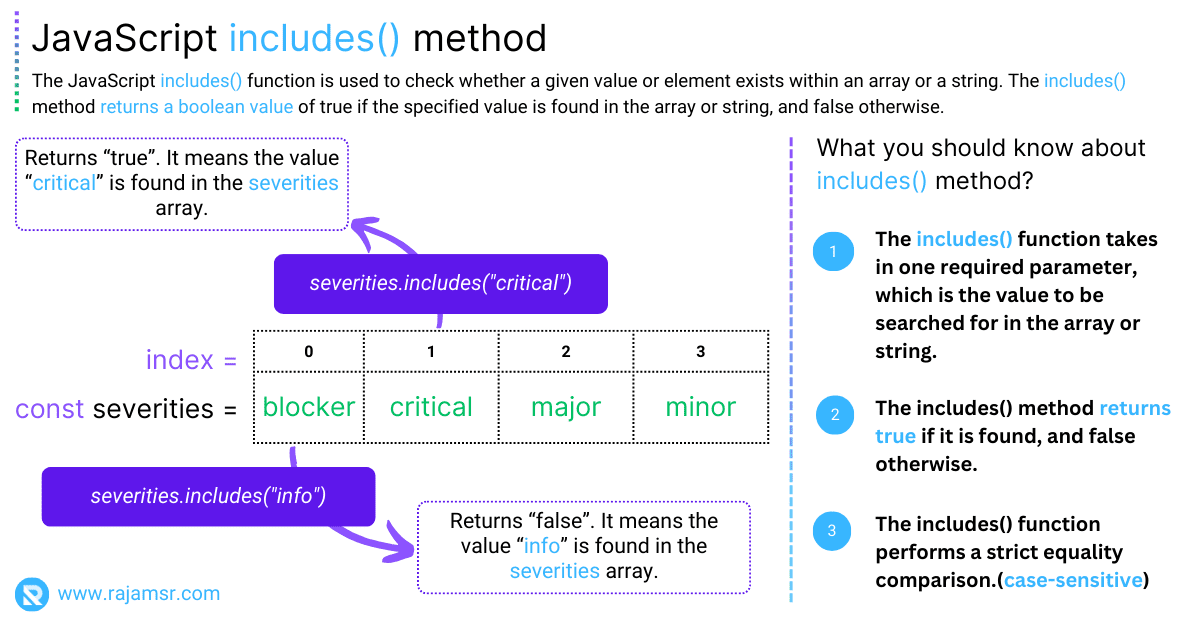
JavaScript dictionary get value by key
To retrieve the value associated with a specific key in a dictionary object, you can use the bracket notation or the get()
method. Here’s an example of dictionary get value by key using the bracket notation:
const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
console.log(languages['EN']);
// Output: "English"
console.log(languages.EN);
// Output: "English"
JavaScript dictionary delete key
delete
operator can be used to remove a key-value pair from a dictionary object. Here’s an example of how to use the delete
operator:const languages = {
'EN': 'English',
'ES': 'Spanish',
'TA': 'Tamil'
}
delete languages['TA']
console.log(Object.values(languages));
// Output: [ 'English', 'Spanish' ]
In this example, the language Tamil
was deleted from the dictionary using the key 'TA'
. If we print the dictionary object "languages"
, it will print the remaining key-value pairs.
Frequently asked questions about dictionary
No. However, we can create custom Dictionary kinds of JavaScript objects.
JavaScript dictionaries (objects) have built-in methods such as Object.keys(), Object.values(), and Object.entries() to work with them.
const severity = {
high: 'High',
medium: 'Medium',
low: 'Low'
};
const keys = Object.keys(severity);
console.log(keys);
// Output: ["high", "medium", "low"]
JSON
string, you can use JSON.stringify()
.const severity = { high: 'High', medium: 'Medium', low: 'Low' }; const jsonString = JSON.stringify(severity); console.log(jsonString) // Output: {"high":"High","medium":"Medium","low":"Low"}
JavaScript dictionaries (objects) don’t have an inherent order, but you can sort their key-value pairs in an array based on the values.
const colors = {
green: 'green',
blue: 'blue',
red: 'red',
};
const sortedArray = Object.entries(colors).sort();
// Converting the sorted array back to a dictionary
const sortedDict = Object.fromEntries(sortedArray);
console.log(sortedDict);
// Output: {blue: "blue", green: "green", red: "red"}
Map()
is a built-in data structure. The main difference is that Map()
allows any data type as keys, whereas object keys are converted to strings.// Dictionary (Object) const colors = { green: 'green', blue: 'blue' }; // Map const colors = new Map(); colors.set('green', 'green'); colors.set('blue', 'blue');
forEach()
, you need to convert the object keys into an array and then use the array forEach()
method.const colors = { green: 'green', blue: 'blue' }; const dictionaryKeys = Object.keys(colors) dictionaryKeys.forEach(key => console.log(`${key}=${colors[key]}`))
In JavaScript, you can store multiple values for a single key by using arrays or objects as values.
const lookup = {
colors: ['green', 'red', 'blue'],
size: ['small', 'medium', 'large']
};
console.log(lookup.colors)
// Output: ["green", "red", "blue"]
reduce()
method.const keysArray = ['red', 'green', 'blue']; const valuesArray = ['#FF0000', '#00FF00', '#0000FF']; const colorMap = keysArray.reduce((acc, key, index) => { acc[key] = valuesArray[index]; return acc; }, {}); console.log(colorMap); // {red: "#FF0000", green: "#00FF00", blue:"#0000FF"}
Conclusion
In conclusion, JavaScript does not have a native dictionary data type. However, we can create JavaScript dictionary objects. The dictionary data structure allows you to store and retrieve data using key-value pairs. They are especially useful when you need rapidly and readily accessible data.
We explored how to create a dictionary and add or modify dictionary values. There are multiple methods for iterating over a dictionary. You can use the for...in
loop, the for...of
loop, and the Object.entries()
methods.
You can check the dictionary’s length by counting its keys. Using the array includes()
method, you can check whether the key already exists in a dictionary. The JavaScript delete
operator can help you remove a key-value pair from a dictionary object.
What are you planning to use the JavaScript dictionary object for?