If you are a web developer, you must know how to work with JavaScript dates. The date helps you manage events, tasks, and actions on the web. But dates and time zones can be hard to handle, especially if you want to use a standard format like ISO 8601. How can you turn a JavaScript date into an ISO string?
You can use the toISOString() method, which is part of the Date object in JavaScript. It gives you a string that shows the date in the ISO 8601 format, like this: YYYY-MM-DDTHH:mm:ss.sssZ. The T separates the date and time, and the Z means that the time is in UTC (Coordinated Universal Time).
But what if you want to change your ISO string, for example, by removing the seconds or changing the time zone? Then you need to do more work, which I will show you in this guide.
In this article, I will show you:
- How to convert date to ISO format string
- How to handle time zones
- Working with the current date
- JavaScript date to ISO string without time
- Convert ISO date string to local time
- How to format ISO date strings
- Convert ISO date string to a specific format
- How to handle time zone offset
- Parsing ISO date string to date object
Let’s explore.
Converting JavaScript date to ISO string
When working with JavaScript dates, you often need to represent them in a standardized format like ISO 8601. The ISO string format ensures consistency and compatibility across different systems. JavaScript provides a built-in method called toISOString()
to achieve this conversion effortlessly.
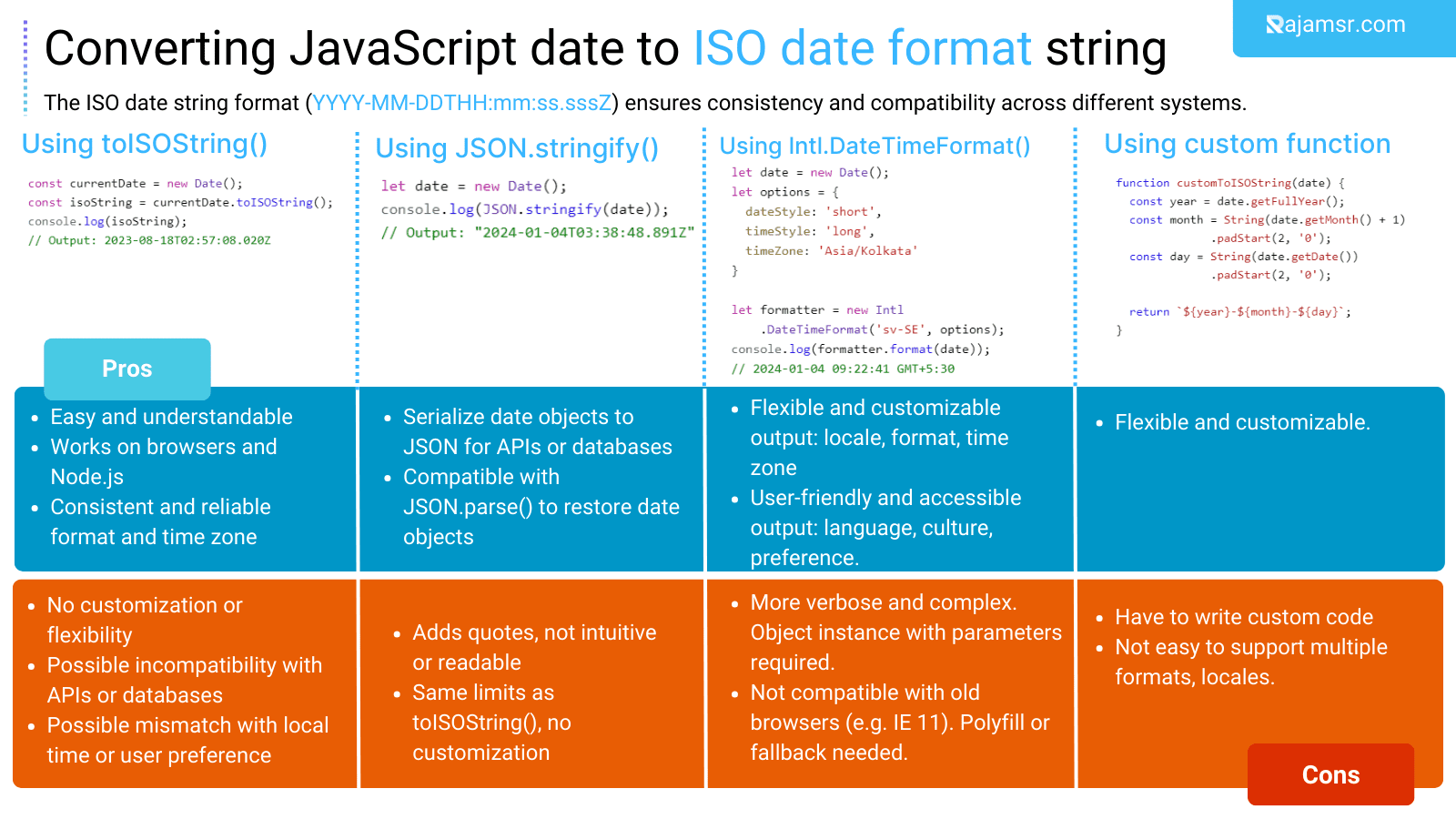
Method 1. Using new date().toISOstring() method
The toISOString()
method is available on JavaScript Date objects and returns a string representation of the date in the ISO 8601 format.
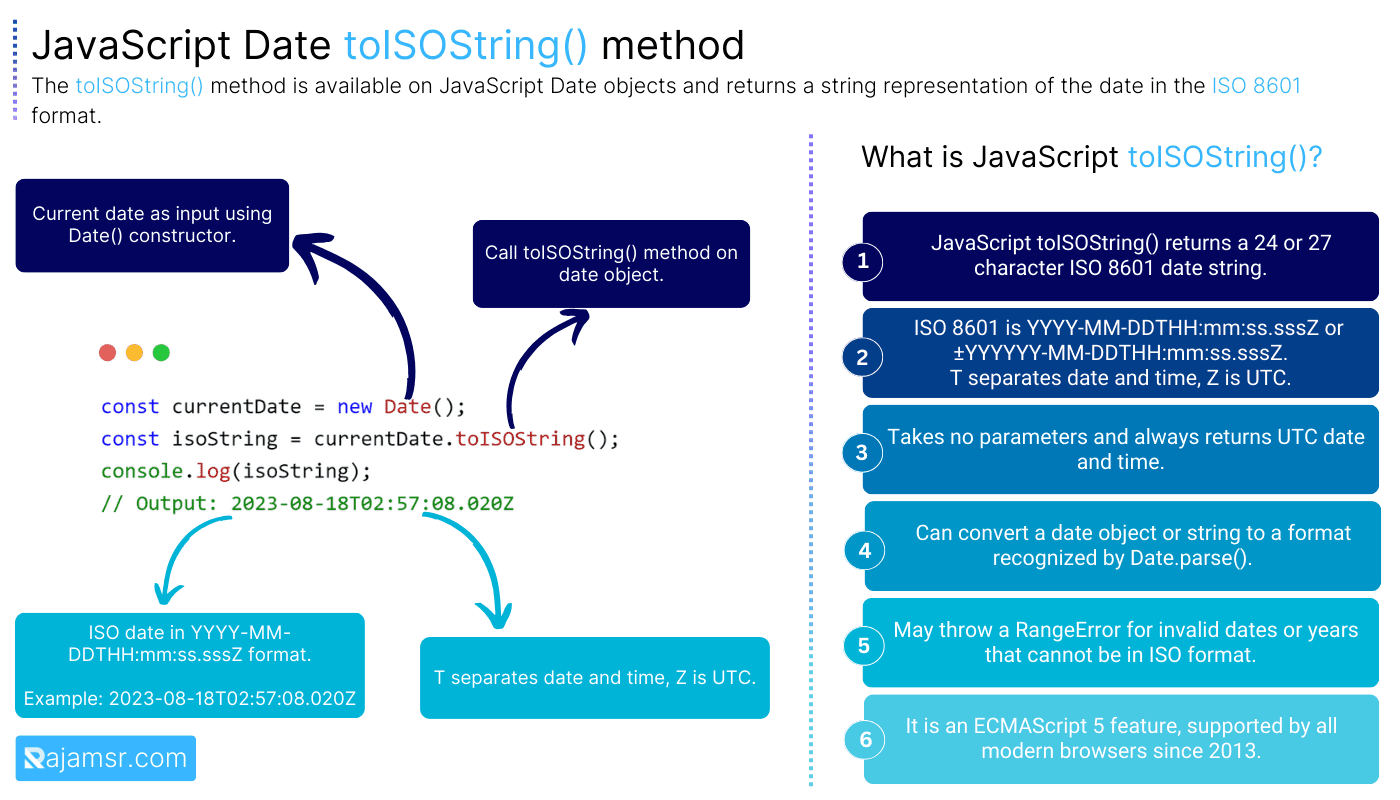
Here’s an example of how to convert a new date to ISO format in JavaScript:
const isoString = new Date().toISOString();
console.log(isoString);
// Output: 2023-08-18T02:57:08.020Z
In this code, the new date object is created using the current date and time. The toISOString()
method is then called on this object, producing an ISO string in the following format: YYYY-MM-DDTHH:mm:ss.sssZ
.
Method 2: Using JSON.stringify() date to iso string
JSON.stringify()
method is another way to convert a date object to an ISO string. It returns a string in the same format as the toISOString()
method, but with double quotes around it. For example:let date = new Date();
console.log(JSON.stringify(date));
// Output: "2024-01-04T03:38:48.891Z"
Method 3: The Intl.DateTimeFormat() method convert date to ISO string with timezone
The Intl.DateTimeFormat()
object is a more advanced and powerful way to convert a date object to an ISO string. It returns a string in a format that is customized according to the given locale
, options
, and time zone
. For example, JavaScript date to ISO string with timezone:
let date = new Date();
let options = {
dateStyle: 'short',
timeStyle: 'long',
timeZone: 'Asia/Kolkata'
}
let formatter = new Intl.DateTimeFormat('sv-SE', options);
console.log(formatter.format(date));
// 2024-01-04 09:22:41 GMT+5:30
This method does not return the exact ISO format string for the time component. Moreover, the date format may vary depending on the locale.
A custom function can be a solution if the methods we discussed earlier are not suitable for your situation. Here is an example of how to write one.
4. Custom ISO string conversion
While toISOString()
provides a standard ISO string format, sometimes you might need a different format. In such cases, you can manually construct an ISO-like string representation:
function customToISOString(date) {
const year = date.getFullYear();
const month = String(date.getMonth() + 1).padStart(2, '0');
const day = String(date.getDate()).padStart(2, '0');
return `${year}-${month}-${day}`;
}
const currentDate = new Date();
const customIsoString = customToISOString(currentDate);
console.log(customIsoString);
// Output: 2023-08-18
customToISOString()
function extracts the year
, month
, and day
components of the date to create a custom ISO-like string in the format YYYY-MM-DD
.Handling time zones
Converting JavaScript ISO string to date
Dealing with time zones is crucial when working with dates, as different users and systems might be in different time zones. Converting an ISO date string to the local time of a user or system requires proper handling of time zones.
In this code, the ISO date string is first converted to a Date object. Then, the toLocaleString()
method is used to convert the date to a local date and time representation based on the user’s or system’s time zone.
const isoDateString = "2023-08-17T12:00:00.000Z";
const date = new Date(isoDateString);
const localDateString = date.toLocaleString();
console.log(localDateString);
// Output: 8/17/2023, 5:30:00 PM
Adding Time zone information to ISO strings
To include time zone information in an ISO string, you can use the JavaScript toISOString()
method. Here’s how you can create an ISO string with a time zone:
const currentDate = new Date();
const isoWithTimezone = currentDate.toISOString();
console.log(isoWithTimezone);
// Output: 2023-08-18T02:59:34.205Z
The resulting ISO string will include the time zone information: YYYY-MM-DDTHH:mm:ss.sssZ
.
Converting current date to ISO string in JavaScript
Converting the current date to an ISO string is a common task when you need to timestamp events or data.
const currentDate = new Date();
const isoString = currentDate.toISOString();
console.log(isoString);
// Output: 2023-08-18T03:00:23.917Z
The code snippet creates a Date
object representing the current date and time and then converts it to an ISO string using the toISOString()
method.
The resulting ISO string will include both date and time components.
Excluding time zone from ISO strings
1. Creating ISO String without Time zone
In some cases, you might want an ISO string without time zone information. Here’s how to achieve that:
const currentDate = new Date();
const isoWithoutTimezone = currentDate.toISOString().slice(0, 19);
console.log(isoWithoutTimezone);
// Output: 2023-08-18T03:02:53
The toISOString()
method provides the complete ISO string, but using JavaScript slice() extracts only the date and time part without the time zone information.
2. Date to ISO string without time using JavaScript toISOString() method
To create an ISO date string without the time component, you can use split() method. Here is an example of date to ISO string without time:
const currentDate = new Date();
const isoDateOnly = currentDate.toISOString().split('T')[0];
console.log(isoDateOnly);
// Output: 2023-08-18
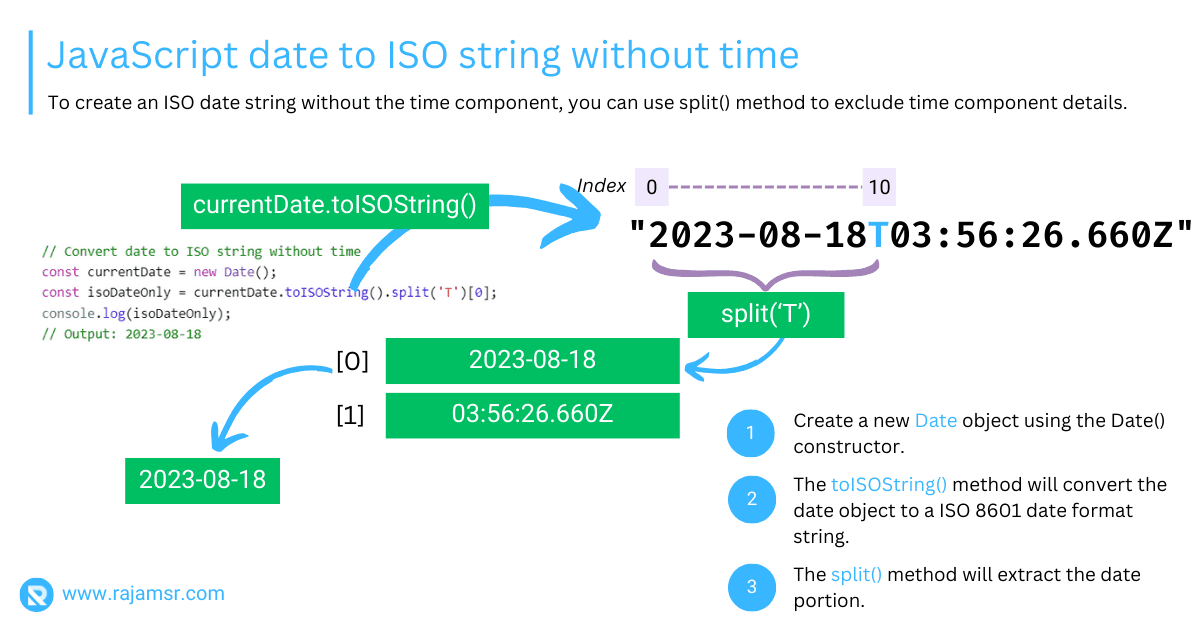
By splitting the ISO string at the ‘T’ character and taking the first part, you get an ISO date without the time.
This code will give you an ISO date string in the format YYYY-MM-DD.
Converting an ISO string to JavaScript date
Converting an ISO date string to the local time of a specific time zone can be achieved using toLocaleString()
. Here is an example of how to convert an ISO string to a date in JavaScript:
const isoDateString = "2023-08-18T12:00:00.000Z";
const date = new Date(isoDateString);
const options = { timeZone: 'America/New_York' };
const localDateString = date.toLocaleString('en-US', options);
console.log(localDateString);
// Output: 8/18/2023, 8:00:00 AM
In this example, the options object specifies the target time zone. The toLocaleString()
method is used with the time zone option to convert the ISO date string to the local time of the specified time zone.
Formatting ISO date strings
JavaScript’s Date object doesn’t provide built-in formatting options, but you can create a function to format ISO date strings as desired:
function formatISODate(isoDateString, format) {
const date = new Date(isoDateString);
const options = {
year: 'numeric',
month: 'short',
day: 'numeric'
};
return new Intl.DateTimeFormat('en-US', options).format(date);
}
const isoDateString = "2023-08-18T12:00:00.000Z";
const formattedDate = formatISODate(isoDateString);
console.log(formattedDate);
// Output: Aug 18, 2023
In this code, the formatISODate()
function takes an ISO date string and a format string as parameters. It then converts the ISO date string to a Date object and uses the Intl.DateTimeFormat
object to format the date according to the provided format.
ISO string without seconds and milliseconds
If you need an ISO string without seconds and milliseconds, you can modify the toISOString()
result:
const currentDate = new Date();
const isoStringWithoutSeconds = currentDate.toISOString().slice(0, 16) + 'Z';
console.log(isoStringWithoutSeconds);
// Output: 2023-08-18T03:08Z
By using the slice (0, 16)
method, you remove the seconds and milliseconds, and then you add ‘Z’ to indicate the UTC
time zone.
Convert ISO date string to local date
To convert an ISO date string to a local Date
object while accounting for time zones:
function convertISOToLocalDate(isoDateString) {
const date = new Date(isoDateString);
const utcMilliseconds = date.getTime();
const offset = date.getTimezoneOffset() * 60 * 1000;
return new Date(utcMilliseconds + offset);
}
const isoDateString = "2023-08-18T12:00:00.000Z";
const localDate = convertISOToLocalDate(isoDateString);
console.log(localDate);
// Output: Fri Aug 18 2023 12:00:00 GMT+0530 (India Standard Time)
This function calculates the UTC milliseconds from the ISO date string, adds the time zone offset in milliseconds, and returns a local Date object.
Converting ISO date string to specific format
If you need to convert an ISO date string to a specific format, you can achieve this using string manipulation and the Date object:
function convertISOToSpecificFormat(isoDateString, format) {
const date = new Date(isoDateString);
const day = String(date.getDate()).padStart(2, '0');
const month = String(date.getMonth() + 1).padStart(2, '0');
const year = date.getFullYear();
return format
.replace('dd', day)
.replace('mm', month)
.replace('yyyy', year);
}
const isoDateString = "2023-08-18T12:00:00.000Z";
const formattedDate = convertISOToSpecificFormat(isoDateString, 'dd/mm/yyyy');
console.log(formattedDate);
// Output: 18/08/2023
In this example, the convertISOToSpecificFormat()
function takes an ISO date string and a format string as parameters. The format string is replaced with the corresponding day, month, and year values from the Date object.
Handling time zone offset
To convert an ISO date string to a local Date object while considering the time zone offset:
function convertISOToOffsetLocalDate(isoDateString, offsetMinutes) {
const date = new Date(isoDateString);
const utcMilliseconds = date.getTime();
const offsetMilliseconds = offsetMinutes * 60 * 1000;
return new Date(utcMilliseconds + offsetMilliseconds);
}
const isoDateString = "2023-08-18T12:00:00.000Z";
const localDate = convertISOToOffsetLocalDate(isoDateString, -240);
console.log(localDate);
// Output: Fri Aug 18 2023 13:30:00 GMT+0530 (India Standard Time)
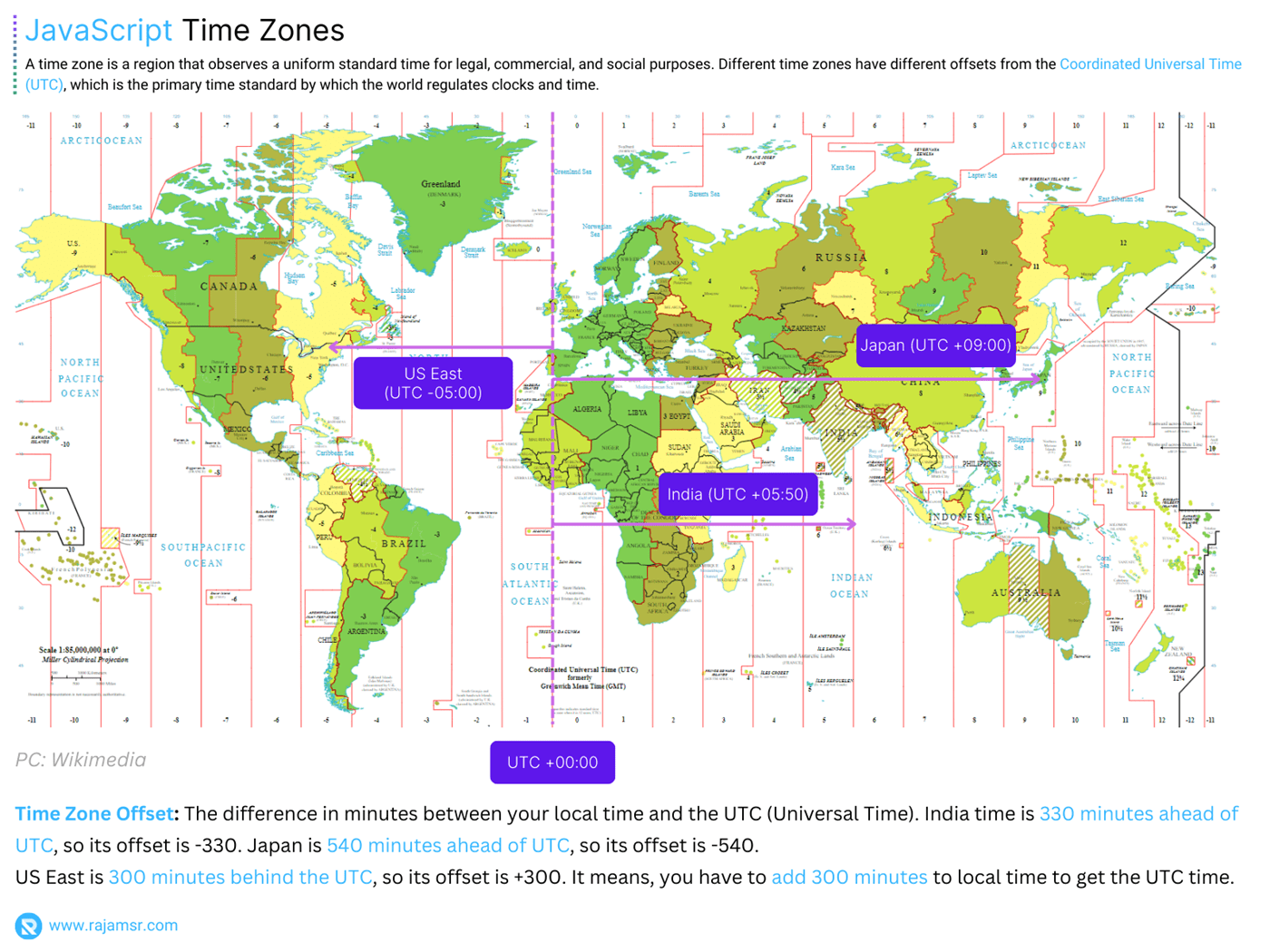
In this code, the convertISOToOffsetLocalDate()
function accepts an ISO date string and an offset in minutes. It then calculates the local date by adding the offset to the UTC milliseconds.
ISO date string with time zone offset
To include the time zone offset in the ISO date string:
function getISOWithTimezoneOffset(date) {
const offsetMinutes = date.getTimezoneOffset();
const offsetHours = -Math.floor(offsetMinutes / 60);
const offsetMinutesRemainder = Math.abs(offsetMinutes) % 60;
const timezoneOffset =
`${offsetHours.toString().padStart(2, '0')}:${offsetMinutesRemainder.toString().padStart(2, '0')}`;
return `${date.toISOString().slice(0, 19)}${timezoneOffset}`;
}
const currentDate = new Date();
const isoWithOffset = getISOWithTimezoneOffset(currentDate);
console.log(isoWithOffset);
// Output: 2023-08-18T03:15:2506:30
In this code, the getISOWithTimezoneOffset()
function calculates the time zone offset in hours and minutes and appends it to the ISO string.
Converting ISO date string to local time zone
To convert an ISO date string to the local time zone of the user’s device:
function convertISOToLocalTimezone(isoDateString) {
const date = new Date(isoDateString);
const localDate = new Date(date.getTime() + (date.getTimezoneOffset() * 60 * 1000));
return localDate;
}
const isoDateString = "2023-08-18T12:00:00.000Z";
const localDate = convertISOToLocalTimezone(isoDateString);
console.log(localDate);
// Output: Fri Aug 18 2023 12:00:00 GMT+0530 (India Standard Time)
In this example, the convertISOToLocalTimezone()
function calculates the local time by adding the time zone offset to the UTC milliseconds.
Working with moment.js library
Moment.js
is a popular JavaScript library that simplifies date and time manipulation. If you prefer using libraries for such tasks, Moment.js provides an easy way to work with ISO date strings:
const isoDateString = "2023-08-17T12:00:00.000Z";
const formattedDate = moment(isoDateString).format('MMMM Do YYYY, h:mm:ss a');
console.log(formattedDate);
In this example, the Moment.js library is used to parse the ISO date string and then format it using the desired pattern.
Handling ISO date string with local time zone
To convert an ISO date string to a local date and time with the user’s local time zone:
const isoDateString = "2023-08-18T12:00:00.000Z";
const localDate = new Date(isoDateString);
console.log(localDate.toLocaleString());
// Output: 8/18/2023, 5:30:00 PM
In this code, the toLocaleString()
method is used directly on the JavaScript Date constructor object, which takes care of converting the ISO date string to the local date and time representation using the user’s local time zone.
Convert ISO date string to specific format and time zone
To convert an ISO date string to a specific format and also adjust it to a specific time zone:
function formatDateTime(date, format, timeZone) {
const options = {
year: 'numeric',
month: '2-digit',
day: '2-digit',
hour: '2-digit',
minute: '2-digit',
second: '2-digit',
timeZone: timeZone,
timeZoneName: 'short'
};
const formatter = new Intl.DateTimeFormat('en-US', options);
const parts = formatter.formatToParts(date);
const formattedDate = format.replace(/(yyyy|MM|dd|HH|mm|ss|zzz)/g, match => {
switch (match) {
case 'yyyy': return parts.find(part => part.type === 'year').value;
case 'MM': return parts.find(part => part.type === 'month').value;
case 'dd': return parts.find(part => part.type === 'day').value;
case 'HH': return parts.find(part => part.type === 'hour').value;
case 'mm': return parts.find(part => part.type === 'minute').value;
case 'ss': return parts.find(part => part.type === 'second').value;
case 'zzz': return parts.find(part => part.type === 'timeZoneName').value;
default: return match;
}
});
return formattedDate;
}
formatDateTime()
method:// Example usage:
const isoDateString = '2022-01-04T12:30:00Z';
const targetTimeZone = 'America/New_York';
// Your desired format string
const targetFormat = 'yyyy-MM-dd HH:mm:ss zzz';
const date = new Date(isoDateString);
const result = formatDateTime(date, targetFormat, targetTimeZone);
console.log(result);
// Output: 2022-01-04 07:30:00 EST
In this example, the formatDateTime()
function takes an ISO date string, a target format string, and a target timezone. It converts and formats the date according to the provided format and timezone.
Parsing ISO date string to date object
To parse an ISO date string and convert it to a JavaScript Date object:
const isoDateString = "2023-08-18T12:00:00.000Z";
const dateObject = new Date(isoDateString);
console.log(dateObject);
// Output: Fri Aug 18 2023 17:30:00 GMT+0530 (India Standard Time)
In this code, simply creating a new Date object with the ISO date string as an argument automatically converts it into a JavaScript Date object.
Frequently asked questions
To convert a date string to ISO format in JavaScript, you can parse the date string and then use the toISOString()
method:
const dateString = "2023-10-28"; const date = new Date(dateString); const isoString = date.toISOString(); console.log(isoString); // Output: "2023-10-28T00:00:00.000Z"
You can convert a timestamp to an ISO date in JavaScript. To do that, create a Date object with the timestamp and then use the toISOString()
method:
const timestamp = 1602722400000; const date = new Date(timestamp); const isoString = date.toISOString(); console.log(isoString); // Output: "2020-10-15T00:40:00.000Z"
To convert an ISO date string to a JavaScript Date object with local time, you can parse the ISO string and then use the Date constructor:
const isoString = "2023-10-28T12:00:00.000Z"; const date = new Date(isoString); const localDate = new Date(date.getTime() + date.getTimezoneOffset() * 60000); console.log(localDate); // Output: Sat Oct 28 2023 12:00:00 GMT+0530 (India Standard Time)
Conclusion
In this article, you learned how to use the JavaScript Date object to create and manipulate ISO strings. ISO strings are a standardized way of representing dates and times in a human-readable format. Each method has its own advantages and disadvantages, and you should choose the one that suits your needs and goals. Here are some tips to help you decide:
- If you need a simple and consistent way to convert a date object to an ISO string, use the
toISOString()
method. - If you need to serialize a date object to JSON format, use the
JSON.stringify()
method. - If you need a flexible and user-friendly way to convert a date object to an ISO string, use the
Intl.DateTimeFormat()
object. - In case, nothing suites your need you can write custom function.
By using ISO strings, you can ensure that your dates and times are consistent and accurate across different platforms and applications.