If you’re working with JavaScript, you probably know how to use arrays to store and manipulate data. But do you know how to use the JavaScript array push() method to add new elements to the end of an array? That’s where the JavaScript array push()
method comes in handy!
In this blog post, we will explore the following:
- What is an array?
- How to push an element at the end
- Remove an element from the array using the pop() method
- Push element to the front of an array
- Add multiple values
- Push an object to the array
- Push another array
- How to push
key-value
pairs - Compare
push()
andconcat()
methods - Alternative methods to array push() method
Let us explore each topic in detail.
What is JavaScript array?
Arrays are value collections that may contain any type of data. Numbers
, characters
, objects
, functions
, and even other arrays
can be stored in an array. The array is one of the non-primitive data types in JavaScript.
To create an array, you use square brackets [ ]
and separate the values with commas. For example:
let htmlTags = ["div", "head", "meta", "body"];
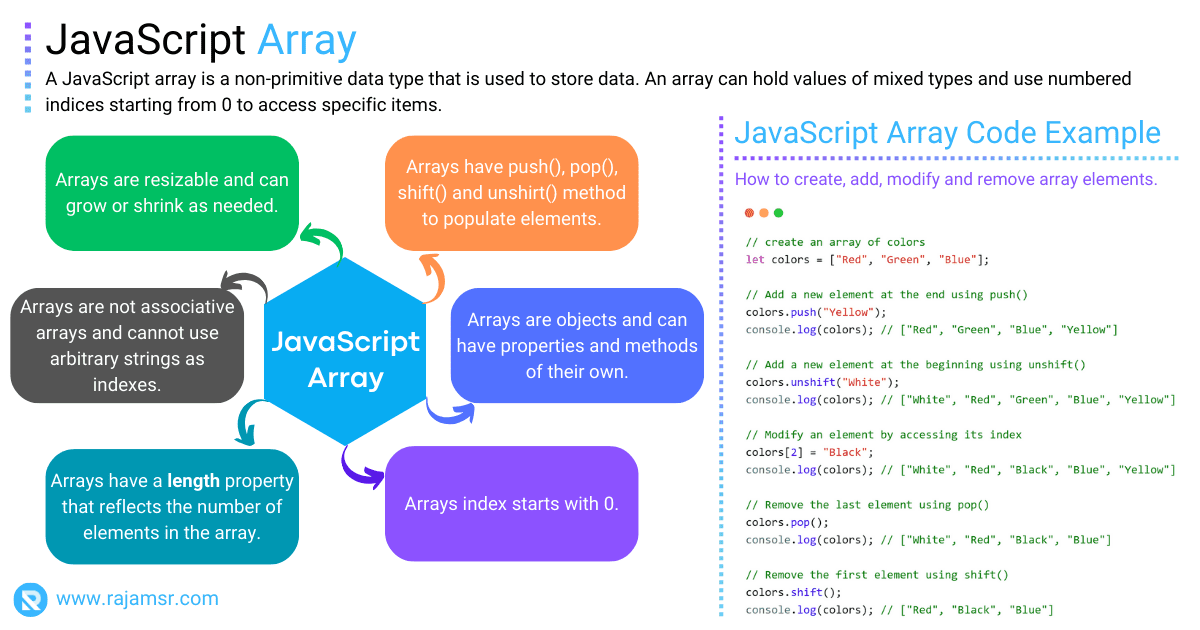
How to use the JavaScript array push() method
push()
method is useful to add one or more elements to the end of an array. It will return the new length of the array. It is one of the JavaScript array methods. Using the JavaScript array push()
method, you can add value to the end of an array.Syntax
The syntax of the push method is as follows:
array.push(element1, element2, ..., elementN)
push()
method takes one or more elements as parameters. It adds them to the end of the array. The order of the elements in the parameters is the same as the order of the elements in the array.Return values
The push() method returns the new length of the array after adding the elements. This can be useful for checking the length of the array or looping through the array.
JavaScript add to array using push() method
let htmlTags = ['div', 'head', 'meta'];
htmlTags.push('body')
console.log(htmlTags)
// Output: ["div", "head", "meta", "body"]
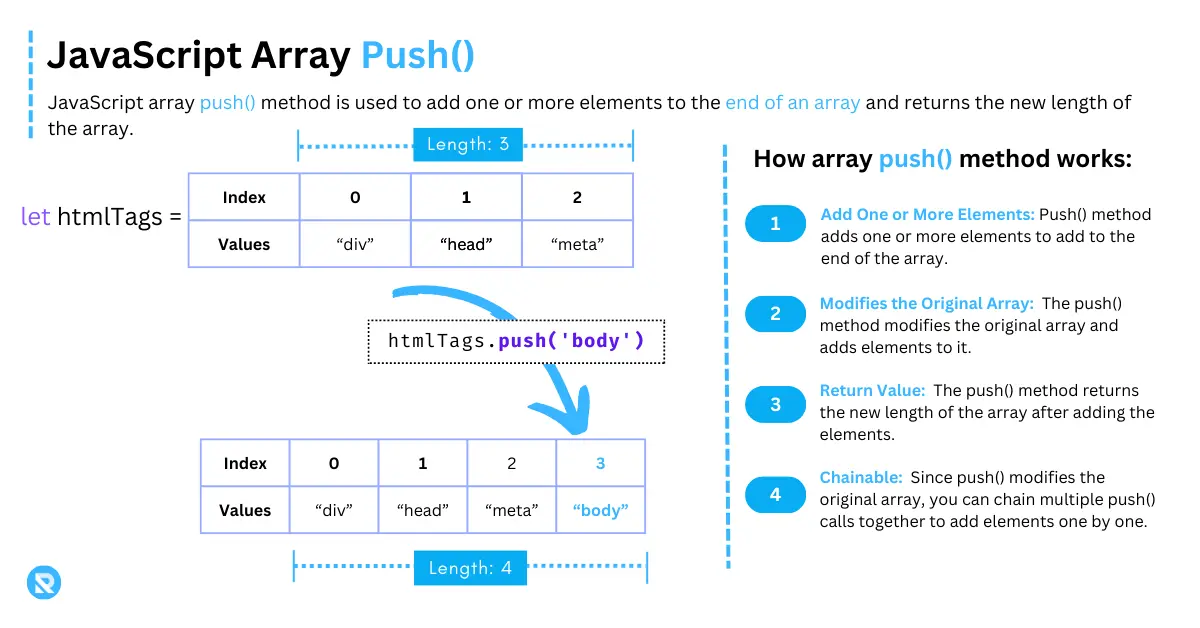
List of HTML
tags created as an array. In this example, we add the string 'body'
tag to the end of the htmlTags
array using the push()
method.
JavaScript array pop element
Consider that you have an array of elements. What if you wanted to remove an element from the end of an array? Using the array pop() method, you can remove elements from the end of an array in JavaScript and get the removed element.
Here’s an example of how to use the pop()
method:
let htmlTags = ['div', 'head', 'meta', 'body'];
var removedElements = htmlTags.pop()
console.log(htmlTags)
// Output: ["div", "head", "meta"]
console.log(removedElements)
// Output: "body"
In this example, the push()
method is called on array htmlTags
. The push()
method removes the last element, "body"
from the array and returns it. The return value is assigned to the variable removedElements
.
Array push element to front of an array
The push()
method always adds elements to the end of an array. So how do you add an element at the beginning of an array? The unshift()
method is the solution.
Using the JavaScript unshift() method is the best way to add an element to the start of an array. Here’s an example of how to use the unshift()
method to add an element to the front of an array:
let options = ['Yes', 'No'];
// Add to front of array
let arrayLength = options.unshift('Select')
console.log(options)
// Output: ["Select", "Yes", "No"]
console.log(arrayLength)
// Output: 3
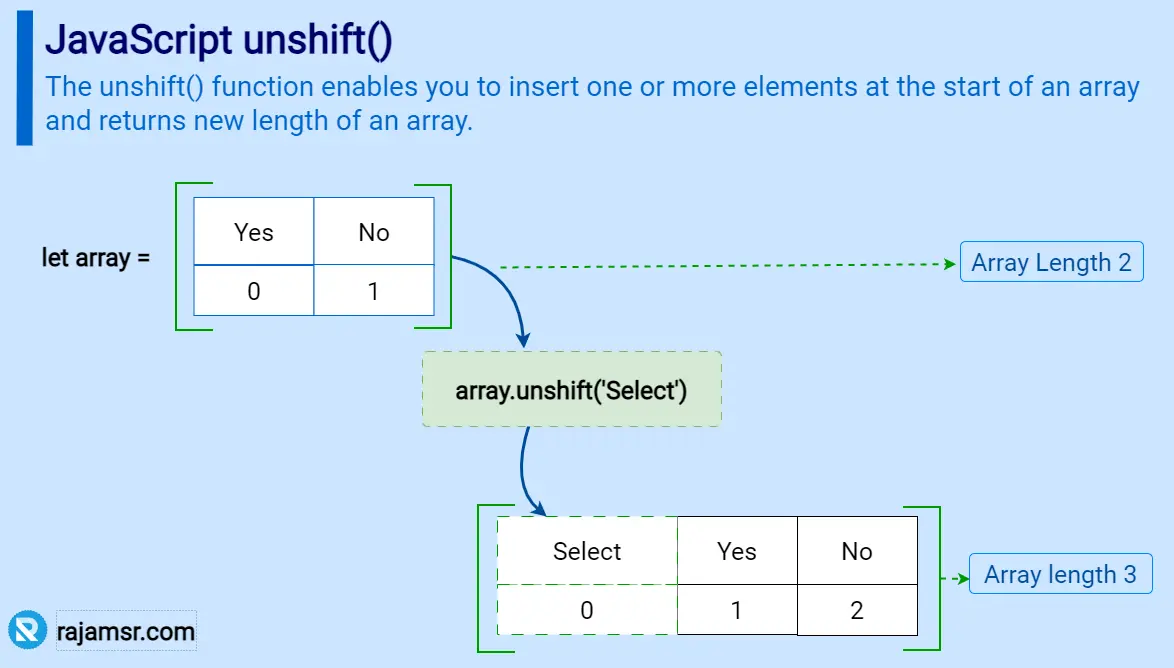
In this example, one new element is inserted into the options array. The unshift()
method returned a new JavaScript array length of 3
, including new elements.
JavaScript push multiple items to array
Using the push()
method, you can add one or more values to the end of an array. To push multiple items to an array using the push()
method, you can pass multiple elements separated by a comma to the method.
Here’s an example of push multiple values to array:
let htmlTags = ['div', 'head', 'meta', 'body'];
let arrayLength = htmlTags.push('html', 'script')
console.log(htmlTags)
// Output: ["div", "head", "meta","body", "html", "script"]
console.log(arrayLength)
// Output: 6
In this example, we first create an array of HTML tags string values. Then, we use the push()
method to push multiple values "html"
and "script"
at the end of the array. The push()
method accepts multiple arguments, so we separate the values with commas. It returns a new array length of 6
, including newly added elements.
Push object to an array
Using an array push()
method, not just numbers and strings you can add objects as well. Here’s an example:
const javaScriptBooks = [
{ bookId: 1, isbn: '9781593275846', title: 'Security Cookbook' },
{ bookId: 2, isbn: '9780596517748', title: 'JavaScript Reference Guide' }
];
let book = { bookId: 3, isbn: '9781449399023', title: 'JavaScript Ultimate' }
javaScriptBooks.push(book)
console.log(javaScriptBooks)
/* Output:[
{ bookId: 1, isbn: '9781593275846', title: 'Security Cookbook' },
{ bookId: 2, isbn: '9780596517748', title: 'JavaScript Reference Guide' },
{ bookId: 3, isbn: '9781449399023', title: 'JavaScript Ultimate' } ]
*/
book
objects stored in the javaScriptBooks
variable. A new book object is created and inserted at the end of an array using the push()
method.Push an array to another array
So far, we have explored how to add strings and objects to an array using the push()
method. What if you wanted to insert another array at the end of an array?
You can use the same array push()
method to add another array to an existing array in JavaScript. Here’s an example:
let htmlTags = ['div', 'head', 'meta'];
let newTags = ['body', 'html', 'script']
htmlTags.push(...newTags)
console.log(htmlTags)
// Output: ["div", "head", "meta","body", "html", "script"]
In this example, we add the elements of newTags
to the end of the htmlTags
array using the push()
method and the spread operator
.
JavaScript add to array key-values
You can use the push()
method to add key-value
pairs to an array using objects. Here’s an example of add to array:
let htmlElements = [];
htmlElements.push({ name: 'button', color: 'blue' });
htmlElements.push({ name: 'input', color: 'black' });
console.log(htmlElements);
// Output: [{name: "button", color: "blue"}, {name: "input", color: "black"}]
In this example, two objects with key-value
pairs are created and added to the htmlElements
array using the push()
method.
Array push unique values
JavaScript does not have any built-in options to create unique array values. However, you write a custom method to make array values unique. The JavaScript includes() array method determines whether an element already exists in the array. If an element is already not in the array, then we can insert it using the push()
method. Here’s an example:
let htmlTags = ['div', 'head', 'meta', 'html'];
const tagToInsert = 'meta';
if (!htmlTags.includes(tagToInsert))
htmlTags.push(tagToInsert)
console.log(htmlTags)
// Output: ["div", "head", "meta", "html"]
In this example, we check if the tagToInsert variable value is already in the htmlTags array using the includes() method. If it isn’t, we add it to the array using the push() method.
Alternatively, you can use the JavaScript indexOf() method to check if an element already exists in an array.
JavaScript has another data type called Sets, which allows you to create unique array values. Both sets and arrays are non-primitive data types in JavaScript.
Comparison of array push() vs concat() methods
The push()
and concat()
methods in JavaScript are used to add elements to an array, but they have some important differences. JavaScript concatenation is the process of combining two or more values.
Here are some examples to compare the two methods:
push() Method | concat() Method |
---|---|
The push() method modifies the original array. | The concat() method concatenates two or more arrays and returns a new array. |
Array push() method can insert one or more elements at the end of an array. | The concat() method can merge two or more arrays or values into a new array. |
JavaScript array push() method returns the new length of the array. | The concat() method returns a new array that contains the merged values. |
let odd = [1, 3, 5];
let even = [2, 4, 6];
const numbers = odd.concat(even);
console.log(numbers)
// Output: [1, 3, 5, 2, 4, 6]
Array push() Method
If you want to add one or more elements to an existing array, you can use the push() method.
Array concat() Method
If you want to merge two or more arrays with the same structure or values into a new array, the concat() method is a better option.
Alternative methods to array push()
the push() method is simple and straightforward to use. However, there are some alternatives and best practices that you should be aware of.
1. Push element using array length property
Assigning a value to the last element of the array using the length property. For example:
let htmlTags = ['div', 'head', 'meta', 'html'];
const tagToInsert = 'meta';
htmlTags[htmlTags.length] = tagToInsert;
console.log(htmlTags);
// Output: ["div", "head", "meta", "html", "meta"]
The array length property can set the last array element. This is quicker and easier than push() method. But it can only add one element.
2. Push multiple elements using spread syntax
Using the spread syntax to create a new array with elements appended to the end. For example
// An array with three elements
let htmlTags = ['div', 'head', 'meta'];
// Create a new array with elements appended to
// the end using the spread syntax
let newTags = [...htmlTags, 'header', 'footer']
// Check the new array contents
console.log(newTags);
// Output: ["div", "head", "meta", "header", "footer"]
The spread syntax is a flexible and elegant way to append elements to an array. However, it does not modify the original array, but creates a new one. This may have an impact on memory and performance.
Conclusion
The JavaScript array push()
method is a powerful option to insert an element at the end of the array. If you want to remove an element from the end of an array, you can use the pop()
method. You can add one or more elements to an array at the end of an array using the push()
method.
Using the unshift()
method, you can add one or more elements to the start of an array, and it will return the new length of the array.
Like numbers and strings, you can add one or more objects to an array at the end using the same push()
method. Using the push()
method, you can add an array of elements to another array.
By default, the array doesn’t have the option to create unique values. You have to take additional measures to make unique array values by using the includes()
method.
By understanding how to use the push()
method effectively, you can easily manipulate and work with arrays in JavaScript!