- What is absolute value?
- How to get absolute value using
Maht.abs()
, usingbitwise ~
operator and using negative (-) operator - Five different use cases of
Math.abs()
function.
What is JavaScript absolute value?
Math.abs()
method in JavaScript returns the absolute value for the number. Here is the syntax for the JavaScript math absolute method:Math.abs(number)
Here"number"
is the number you want to find the absolute value of, regardless of its sign. For example:
console.log(Math.abs(-5))
// Output: 5
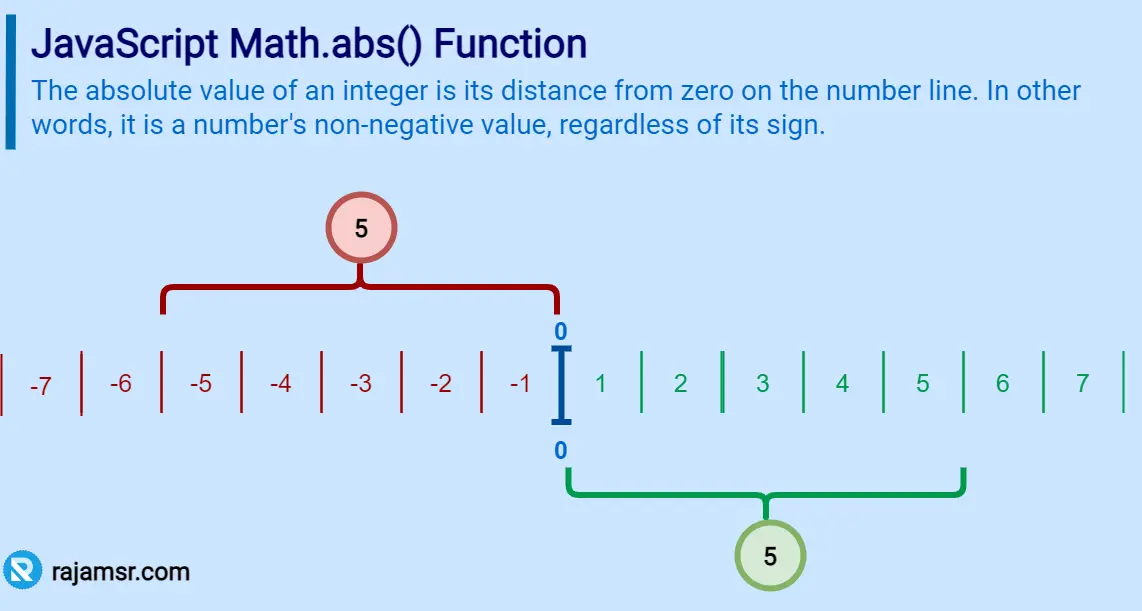
How to get absolute value in JavaScript?
There are multiple ways to get the absolute value of a number. However, in this post, we will look at three different approaches.
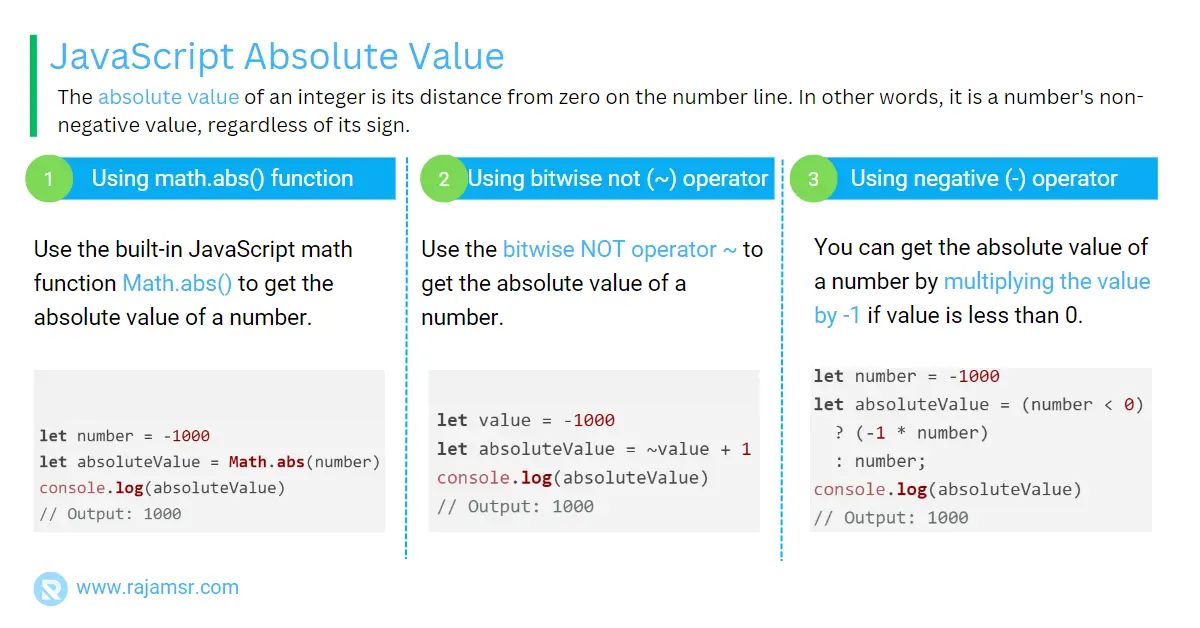
1. Get absolute number using math.abs() function
To get the absolute value, you can use the built-in JavaScript math function Math.abs(). For example, to find the absolute value for the number -1000, you can write the following code:
let number = -1000
let absoluteValue = Math.abs(number)
console.log(absoluteValue)
// Output: 1000
Using Math.abs()
in JavaScript for converting a number to an absolute value is the standard approach. How do I get an absolute value in JavaScript without using the Math.abs()
function?
You can use the following two approaches to get the absolute value in JavaScript without using the Math.abs()
function:
2. Get absolute value in JavaScript using bitwise not (~) operator
You may also use the bitwise NOT operator ~ to get the absolute value of a number. For example, to find the bitwise absolute value of -1000 using the bitwise not operator , use the following code:
let value = -1000
let absoluteValue = ~value + 1
console.log(absoluteValue)
// Output: 1000
3. Using negative (-) operator
let number = -1000
let absoluteValue = (number < 0)
? (-1 * number)
: number;
console.log(absoluteValue)
// Output: 1000
This is one of the ways to get absolute value in JavaScript without using the Math.abs()
function.
Applications of JavaScript absolute values
There are many ways that absolute value can be used in JavaScript, but some common applications include:
1. Absolute difference value between two numbers
In some cases, we must get the absolute difference value of two numbers regardless of their number order. This is a common application scenario.
To get the absolute difference in JavaScript between two numbers, use the code below.
const absoluteNumbersDiff = (n1, n2) => {
return Math.abs(n1 - n2)
}
console.log(absoluteNumbersDiff(10, 40)) // 30
console.log(absoluteNumbersDiff(40, 10)) // 30
2. Calculate absolute value of date difference
We sometimes need the number of days between two days, just like we did for numbers in the previous example. To get the absolute value of a date difference between two dates, you can use the below code:
const daysDiff = (d1, d2) => {
var differenceInMilliseconds = d1.getTime() - d2.getTime();
let millisecondsPerDay = (1000 * 3600 * 24)
return Math.abs(differenceInMilliseconds / millisecondsPerDay)
}
var date1 = new Date("01/01/2023"); // MM/dd/yyyy
var date2 = new Date("01/31/2023"); // MM/dd/yyyy
// We wil get the days difference regardless of date parameter order.
console.log(daysDiff(date1, date2)) // 30
console.log(daysDiff(date2, date1)) // 30
In the above example, we are calculating the day’s difference between two dates "01/01/2023"
and "01/31/2023"
. The custom daysDiff()
function will return the day difference in absolute value, regardless of the JavaScript Date order parameter.
3. Validating user input
By ensuring that a user’s input is a positive number, you can prevent errors and ensure that your program is working correctly.
Let’s assume you are getting age input from a user. Age cannot be a negative number. So you may compare its absolute value to the user’s real input. If both are the same, the age input is a valid number. Otherwise, you can confirm the given age input is a negative number.
let age=-10; // Assume this value is coming from user input
if (Math.abs(age) === age) {
console.log('Input value for agen correct.')
} else {
console.error(`${age} is not a valid value for age.`)
}
// -10 is not a valid value for age.
4. Calculating distance between two points
The distance between two points on a coordinate plane can be calculated using an absolute value, which can be useful in game development and other applications.
Using the following code, you can calculate the distance between two points on a coordinate plane:
function calculateDistance(x1, y1, x2, y2) {
const xDiff = Math.abs(x1 - x2);
const yDiff = Math.abs(y1 - y2);
return Math.sqrt(xDiff ** 2 + yDiff ** 2);
}
let distance = calculateDistance(-12, -10, 12, 10);
console.log(distance)
// Output: 31.240998703626616
5. Find largest absolute number
Absolute value is important for sorting and searching since it can be used to compare values in an array and find the largest or smallest value.
Assuming you have an array of positive and negative values, You can write code like this to find the largest absolute value:
const numbers = [3, -12, 10, -5, 1];
let largest = Math.abs(numbers[0]);
for (let index = 1; index < numbers.length; index++) {
if (Math.abs(numbers[index]) > largest) {
largest = Math.abs(numbers[index]);
}
}
console.log(largest);
// Output: 12
What if you want to find a smaller absolute value? In the above code block, you have to replace greater than(>)
with less than (<)
on line #5, and then it will return the smallest absolute value.
Conclusion
In conclusion, we can get the JavaScript absolute value using the Math.abs()
function. If you don’t want to use Math.abs()
to get an absolute number, you can either use the bitwise NOT operator or multiply the number by minus one.
Using the Math.abs()
function, you can get the absolute value between two numbers or dates, regardless of their value order.
Math.abs()
allows you to validate user input, calculate the distance between two points, or find the largest absolute number in an array of positive and negative numbers.
Understanding how to use absolute value is an essential part of any programmer’s skill set, and it may help you develop more efficient and effective code.
Which method are you going to use to get the absolute value?