JavaScript math functions can help you perform complex calculations and solve mathematical problems with ease. Whether you’re building a simple calculator app or working on a complex data analysis project, these math functions can make your life a whole lot easier.
In this blog, we’ll dive into the methods of math functions:
- Basic math functions
- Trigonometric functions
- Logarithmic Functions
- Advanced math functions
- Most commonly used functions and showing you how to put them to work in your projects.
Let’s look into each math function in JavaScript.
Basic JavaScript math functions
JavaScript provides several basic math functions that allow you to perform simple calculations on numbers. Math
is not a constructor. You can call any property or method without creating an instance of a Math
object.
In basic math functions, we will look into the following functions:
- Math.abs()
- Math.ceil()
- Math.floor()
- Math.round()
- Math.random()
JavaScript math functions Math.abs()
The Math.abs()
function returns JavaScript absolute value (without sign) for a number. Let’s ses an example of how to get JavaScript math absolute value for -1000:
let number = -1000;
let absoluteNumber = Math.abs(number);
console.log(absoluteNumber);
// Output: 1000
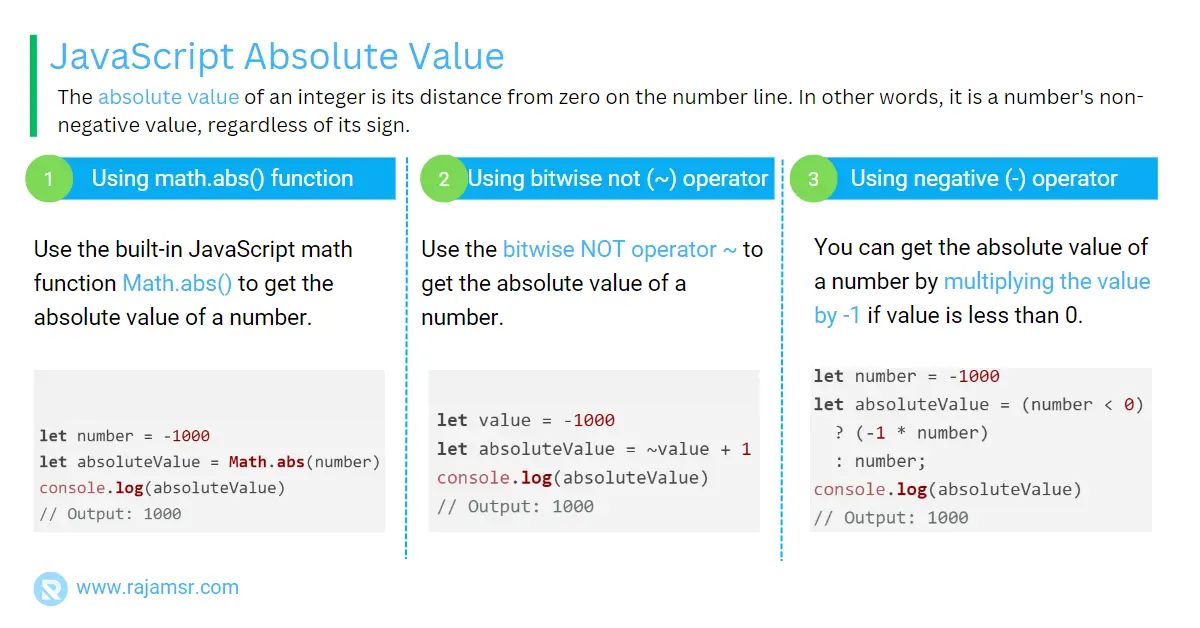
Math.ceil()
Math.ceil()
rounds a number to the closest integer. Let’s see an example of a JavaScript math ceiling function to round up a number 3.9
:let number = 3.9;
let ceilNumber = Math.ceil(number);
console.log(ceilNumber);
// Output: 4
Math.floor()
JavaScript Math.floor() function rounds down a number to the nearest integer. Let’s see an example for JavaScript math floor function:
let number = 3.9;
let floorNumber = Math.floor(number);
console.log(floorNumber);
// Output: 3
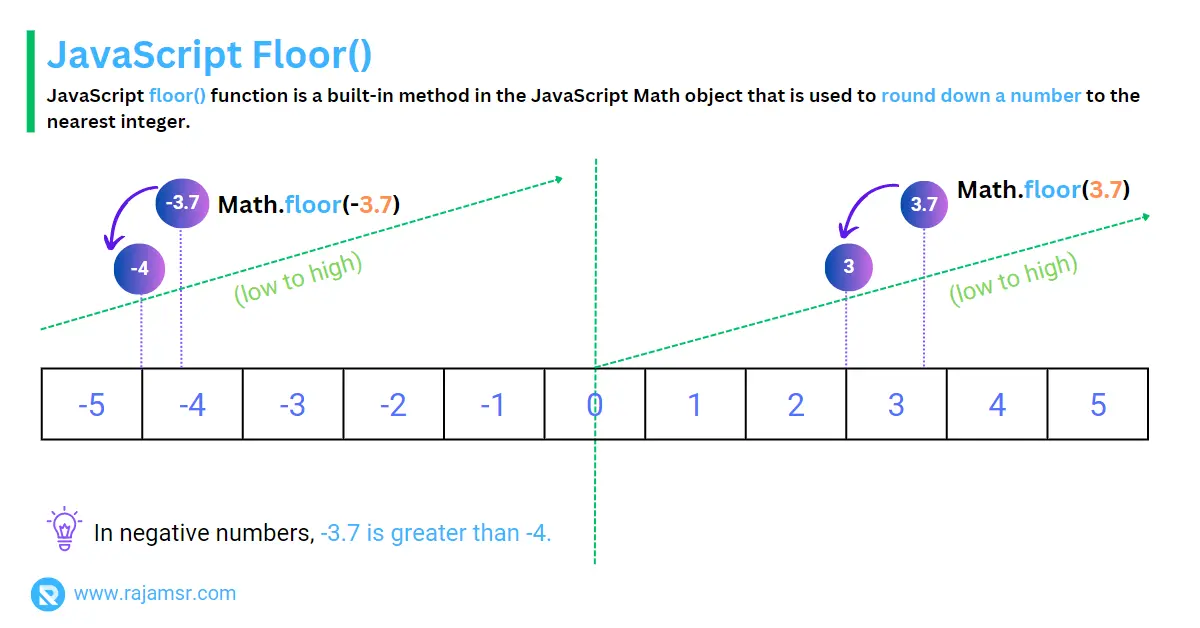
Math.round()
Round a number to the closest integer using the Math.round()
function. For example:
let number = 4.5;
let roundNumber = Math.round(number);
console.log(roundNumber);
// Output: 5
Round a number to 2 decimal places is a common requirement when dealing with decimal or currency values. You have to use the JavaScript toFixed() function along with other number conversion-related functions.
Math.random()
To generate a random decimal between 0 and 1, you can use the Math.random()
function. Let’s see how to use the JavaScript math random function:
let randNumber = Math.random();
console.log(randNumber);
// Output: 0.6807606392621475 (random number between 0 and 1)
This code generates a random floating-point number in the range of 0 to 1. The result will vary each time you run the code.
Trigonometric JavaScript math functions
Let’s take a closer look at some of the commonly used trigonometric functions available in JavaScript’s Math
object.
- Math.sign()
- Math.cos()
- Math.tan()
- Math.atan()
- Math.atan2()
These JavaScript math functions are used to calculate angles and distances between points.
Math.sign()
The Math.sin()
function returns the sine of a given angle in radians. For example:
let angle = 45;
let sinValue = Math.sin(angle);
console.log(sinValue);
// Output: 0.7071067811865476
Math.sin()
function and prints the result (approximately 0.7071067811865476
) to the console.Math.cos()
Math.cos()
trigonometric function returns the cosine value of an angle in radians. For example:
let angle = 45;
let cosValue = Math.cos(angle);
console.log(cosValue);
// Output: 0.7071067811865475
45
degrees and prints the result, which is approximately 0.7071067811865475
.Math.tan()
Math.tan()
function returns the tangent of an angle in radians. For example:
let angle = 45;
let tanValue = Math.tan(angle);
console.log(tanValue);
// Output: 1.0
45-degree angle
using the Math.tan()
function and prints the result, which is 1.0
.Math.atan()
This Math.atan()
function returns the arctangent of a number. This is the inverse of the tangent
function. It’s commonly used to calculate the angle of a right triangle. For example:
let number = 2;
let arctangentValue = Math.atan(number);
console.log(arctangentValue);
// Output: 1.1071487177940904
arctangent (inverse tangent)
of the number 2 using the Math.atan()
function and prints the result, which is approximately 1.1071487177940904
.Math.atan2()
Math.atan2()
returns the arctangent of the quotient of two specified numbers. Using this Math.atan2()
function, you can calculate the angle between two points on a coordinate plane. For example:
let y = 1;
let x = 1;
let atan2Value = Math.atan2(y, x);
console.log(atan2Value);
// Output: 0.7853981633974483
The code calculates the arctangent of the ratio of y
to x
using the Math.atan2()
function, where y
is 1
and x
is 1
and prints the result, which is approximately 0.7853981633974483
.
Logarithmic JavaScript math functions
JavaScript Math
Object provides four built-in functions for calculating logarithmic and exponential values:
- Math.log()
- Math.log10()
- Math.log2()
- Math.exp()
Exponential and logarithmic calculations could be simplified using the above-listed logarithmic functions.
Math.log()
Math.log()
function returns the natural logarithm (base e) of a number. Let’s see an example of how to use JavaScript math log function:
let number = 15;
let logValue = Math.log(number);
console.log(logValue);
// Output: 2.70805020110221
natural logarithm
of the number 15
using the Math.log()
function and prints the result, which is approximately 2.70805020110221
.Math.log10()
The Math.log10()
function is used to calculate the logarithm to the base 10 of a number. It takes one argument, which is the number whose logarithm needs to be calculated. For example:
let number = 1000;
let log10Value = Math.log10(number);
console.log(log10Value);
// Output: 3
The code calculates the base-10 logarithm
of the number 1000
using the Math.log10()
function and prints the result, which is 3
.
Math.log2()
The Math.log2()
function is used to calculate the logarithm to the base 2
of a number. It takes one argument, which is the number whose logarithm needs to be calculated. For example:
let number = 8;
let log2Value = Math.log2(number);
console.log(log2Value);
// Output: 3
The code calculates the base-2 logarithm
of the number 8
using the Math.log2()
function and prints the result, which is 3
.
Math.exp()
Math.exp()
function returns the exponential value of a number
. For example, the following code returns the exponential value of 3:
let number = 3;
let exponentValue = Math.exp(number);
console.log(exponentValue);
// Output: 20.085536923187668
power of 3
using the Math.exp()
function and prints the result, which is approximately 20.085536923187668
.Advanced JavaScript math functions
JavaScript’s Math object includes several advanced math functions that can perform more complex calculations. These functions can be used in various scenarios and can significantly simplify the code needed to perform such calculations.
JavaScript also provides advanced math functions such as:
- Math.pow()
- Math.sqrt()
- Math.cbrt()
- Math.max()
- Math.min()
Using these advanced functions, you can do more complex calculations with ease.
Math.pow()
Math.pow()
function returns the value of a base raised to the power of an exponent (23
= 2 x 2 x 2
). For example, how to use Math.pow() JavaScript math function:
let base = 2;
let exponent = 3;
let powValue = Math.pow(base, exponent);
console.log(powValue);
// Output: 8
base 2
to the power of exponent 3
using the Math.pow()
function and prints the result, which is 8
.Math.sqrt()
Using the Math.sqrt()
method, you can calculate the square root of a number. It takes one argument, which is the number whose square root needs to be calculated.
For example, Math.sqrt(81)
will return the square root 9
. This is the square root of 81
.
let number = 81; // 9 x 9
let squareRootValue = Math.sqrt(number);
console.log(squareRootValue);
// Output: 9
Math.cbrt()
Math.cbrt()
function returns the cube root of a number. For example:
let number = 8; // 2 x 2 x 2
let cubeRootValue = Math.cbrt(number);
console.log(cubeRootValue);
// Output: 2
The code calculates the cube root
of the number 8
using the Math.cbrt()
function and prints the result, which is 2
.
Math.max()
The Math.max()
function finds the maximum value in an array of numbers. An array is one of the non-primitive data types in JavaScript. It accepts two or more inputs and returns the highest value. For example:
let numbers = [12, 2, 23, 8];
let maximumValue = Math.max(...numbers);
console.log(maximumValue);
// Output: 23
The code finds and prints the maximum value from the array [12, 2, 23, 8]
using the ES6 spread operator (…) with the Math.max()
function, resulting in the output 23
.
Math.min()
The Math.min()
function is used to find the minimum value among a set of numbers. It takes two or more arguments, and it returns the lowest value. For example:
let numbers = [15, 12, 4, 24];
let minimumValue = Math.min(...numbers);
console.log(minimumValue);
// Output: 4
The code determines and prints the minimum value from the array [15, 12, 4, 24]
using the spread operator (...)
with the Math.min()
function, resulting in the output 4
.
Examples of using JavaScript math functions
Let’s take a look at some examples of how these math functions can be used in real-world scenarios.
Finding the absolute value of a number
Assume we have a number with a negative value. We can use Math.abs()
function to find its absolute value.
let number = -10;
let absoluteNumber = Math.abs(number);
console.log(absoluteNumber);
// Output: 10
Rounding a number to a certain decimal place
Assume we have a JavaScript variable with a decimal number. You can use the Math.round()
function to round it to a certain decimal place.
let number = 124.567;
let roundedNumber = Math.round(number * 100) / 100;
console.log(roundedNumber);
// Output: 124.57
Generating a random number within a range
Assume you want to generate a random number between a range of numbers like 11 and 20. You can use the Math.random()
function to achieve this, as shown below:
let randNumber = Math.floor(Math.random() * 10) + 11;
console.log(randNumber);
// Output: 17 (a random number between 11 and 20)
Calculating the distance between two points
Assume we have two variables with the coordinates of two locations on a plane. The Math.sqrt()
function can be used to determine the distance between two points.
let x1 = 1;
let y1 = 2;
let x2 = 4;
let y2 = 6;
let point1 = Math.pow(x2 - x1, 2);
let point2 = Math.pow(y2 - y1, 2)
let distanceBetweenPoints = Math.sqrt(point1 + point2);
console.log(distanceBetweenPoints);
// Output: 5
Conclusion
In conclusion, JavaScript math functions that come in handy when it comes to performing both simple and complex calculations. Whether you’re trying to figure out the absolute value of a number or calculating the distance between two points on a plane, these functions have got you covered.
With the assistance of JavaScript math functions
, you can do complex calculations in just a few lines of code. Otherwise, you’d have to write hundreds of lines of code to accomplish the same thing.
Whether you’re a seasoned developer or just starting, having a good understanding of JavaScript math functions
is vital to building robust and effective applications that can take your project to the next level.