Have you ever wondered how to convert a string to a number in JavaScript? If you have, you might have come across the JavaScript parseInt() function, which is one of the most common ways to do it. But do you know how it works and what are the pitfalls to avoid?
In this blog, I will show you:
- What is the
parseInt()
function? - How to use the
parseInt()
function? - What is the radix parameter?
- Using parseInt() with different number systems
- Common pitfalls and errors of the parseInt function
- Other similar functions to the parseInt() function
- Code examples of the parseInt() function
So, let’s dive in!
What is the JavaScript parseInt() function?
The parseInt()
function is a built-in JavaScript function that converts a JavaScript string into an integer, or a whole number. It is useful when you need to perform arithmetic operations or comparisons on numeric values that are stored or received as strings.
For example, if you have a string "40"
and you want to add 10
to it, you cannot simply do "40" + 10
, because that will result in the string "4010"
. Instead, you need to use the parseInt()
function to convert the string "40"
into the number 40
, and then you can do 40 + 10
, which will give you the correct answer of 50
.
let number = "40";
console.log(number + 10); // Output: "4010"
How to use the parseInt() JavaScript function?
The parseInt function has the following syntax:
parseInt(string, radix)
The function takes two parameters: string
and radix
.
Parameter | Description |
---|---|
string | The string parameter is the string that you want to convert into an integer. |
radix | The radix parameter is an optional value that specifies the base or the numerical system of the string. The default value of the radix parameter depends on the format of the string. |
The function returns the integer value that corresponds to the string, or NaN (Not a Number)
if the string cannot be converted into a valid number.
Here are some examples of using the parseInt()
function:
let numberString = "123";
let radix = 10;
let number = parseInt(numberString, radix);
console.log(number); // Output: 123
console.log(parseInt("40")); // Output: 40
console.log(parseInt("3.14")); // Output: 3 (Decimal part is ignored)
console.log(parseInt("hello")); // Output: NaN (String is not a valid number)
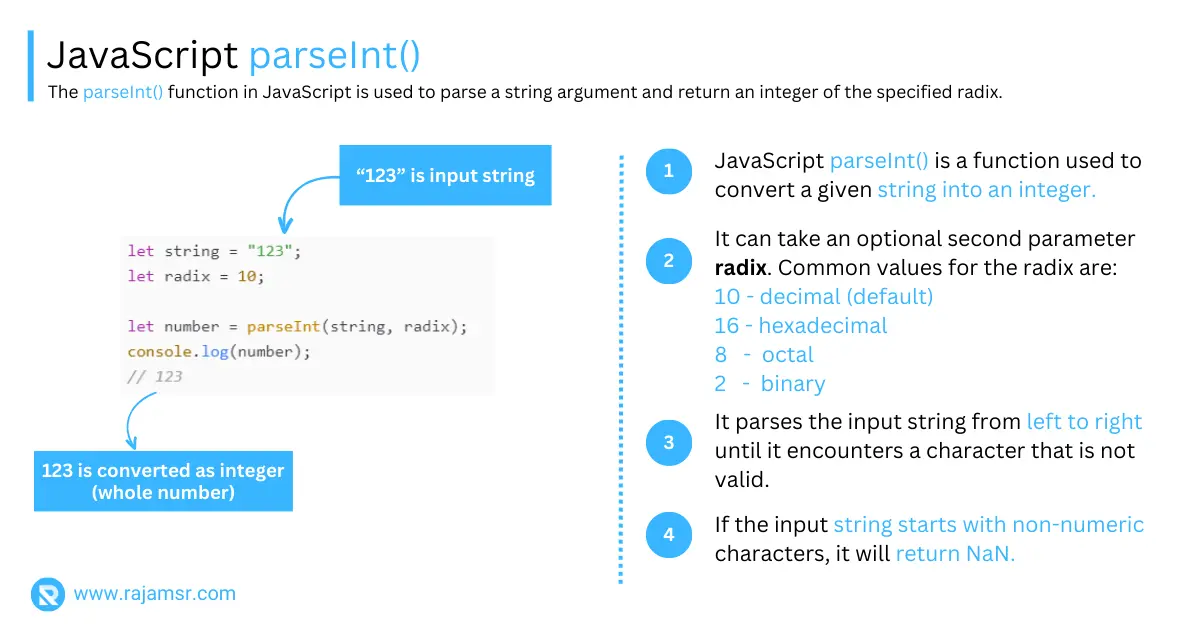
In this example, the string "123"
is converted to the number 123
using a radix of 10, which represents the decimal system. The result of the parseInt()
method is then printed to the browser console.
What is the radix parameter?
The radix
parameter is a value that indicates the base or the numerical system of the string that you want to convert into an integer.
Radix | Description |
---|---|
2 | Binary. |
8 | Octal. If the string starts with “0”, the radix is assumed to be 8 (octal) in some older browsers, but 10 (decimal) in modern browsers. |
10 | Decimal. If the string does not start with any of these prefixes, the radix is assumed to be 10 (decimal). |
16 | Hexadecimal. If the string starts with “0x” or “0X”, the radix is assumed to be 16 (hexadecimal). |
The radix
parameter is optional. However, it is recommended to always specify it. Because the default value of the radix parameter depends on the format of the string.
Therefore, to avoid confusion and unexpected results, it is better to always provide the radix parameter when using the parseInt()
function.
How to use JavaScript parseInt() with different number systems
parseInt()
function better, here are some code examples that you can try out.This example shows how to use the parseInt()
function with different radix values.// Convert binary strings to integers
console.log(parseInt("1010", 2)); // Output: 10
console.log(parseInt("1111", 2)); // Output: 15
console.log(parseInt("100000", 2)); // Output: 32
// Convert octal strings to integers
console.log(parseInt("12", 8)); // Output: 10
console.log(parseInt("77", 8)); // Output: 63
console.log(parseInt("1000", 8)); // Output: 512
// Convert hexadecimal strings to integers
console.log(parseInt("A", 16)); // Output: 10
console.log(parseInt("FF", 16)); // Output: 255
console.log(parseInt("1000", 16)); // Output: 4096
Common pitfalls and best practices when using parseInt()
The parseInt()
function is a powerful and handy method, but it also has some limitations and quirks that you need to be aware of.
Here are some of the common pitfalls and best practices that you should follow when using the parseInt()
function:
1. The parseInt()
function only converts the first valid numeric part of the string and ignores the rest.
For example, parseInt("40px")
returns 40
, and parseInt("5.14.15")
returns 5
. This may lead to unexpected results or data loss if the string contains more than one number or other characters.
console.log(parseInt("40px")); // Output: 40
console.log(parseInt("5.14.15")); // Output: 5
2. The parseInt()
function does not check for overflow or underflow. This means that if the string represents a number that is too large
or too small
to fit in the JavaScript number type, the function will return an incorrect value.
For example, parseInt("9007199254740993")
returns 9007199254740992
, and parseInt("-9007199254740993")
returns -9007199254740992
.
In JavaScript, 9007199254740991
and -9007199254740991
are the largest and smallest integers that can be represented in JavaScript, and any number beyond them will lose precision.
console.log(parseInt("9007199254740993")); // Output: 9007199254740992
console.log(parseInt("-9007199254740993")); // Output: -9007199254740992
3. The parseInt()
function does not handle leading zeros correctly. This is because leading zeros are often used to indicate octal (base 8)
numbers, but not all browsers follow this convention.
For example, parseInt("010")
may return 10
in some browsers, but 8
in others. To avoid this ambiguity, you should either remove the leading zeros from the string or specify the radix
parameter as 10
.
console.log(parseInt("010", 10)); // Output: 10
parseInt()
function may return NaN
if the string is not a valid number, or if the radix
parameter is invalid.For example, parseInt("hello")
returns NaN
, and parseInt("42", 37)
also returns NaN
. To handle these cases, you should always check the return value of the parseInt()
function using IsNaN()
before using it. Otherwise, use a default value if the function returns NaN
.console.log(parseInt("Hello")); // Output: NaN
console.log(parseInt("40", 50)); // Output: NaN (invlid radix)
Use cases of parseInt() JavaScript method
The parseInt()
method has several use cases; in this post, I covered four different use cases:
1. Convert JavaScript string to integer using JavaScript parseInt() method
Converting a string to a number is one of the traditional use cases. The primary purpose of the parseInt()
method is to convert a string to an integer, as shown in the following example:
let numberString = '123';
let number = parseInt(numberString);
console.log(number);
// Output: 123
In this example, the string "123"
is converted to the number 123
using the parseInt()
method. The result of the method is then displayed in the browser console.
2. Converting radix-based numbers to decimal
You can use the parseInt()
method to convert radix-based numbers to decimals, as in the following example:
let string = '1011';
let radix = 2;
let number = parseInt(string, radix);
console.log(number);
// Output: 11
In this example, the string "1011"
is converted to the number 11 using a radix of 2, which represents the binary system. The result of the parseInt()
method is then logged into the console.
Use the JavaScript parseFloat() method to convert strings to floating-point numbers.
3. ParseInt() removing leading zeros from a string
If you are getting input from external sources, numbers may be prefixed with a leading zero. Using the parseInt()
method, you can remove the leading zeros from a string by converting it to an integer.
let stringWithZeros = '00050';
let number = parseInt(stringWithZeros);
console.log(number);
// Output: 50
In this example, the string "00050"
is converted to the number 50
using the parseInt()
method.
4. Using parseInt() in JavaScript with other conversion functions
The parseInt()
method can be used in conjunction with other conversion functions in JavaScript. You can use Number
and parseFloat()
to achieve a more specific result.
let string = "12.34";
let number = Number(string);
let integer = parseInt(number);
console.log(integer);
// Output: 12
In this example, the string "12.34"
is first converted to the decimal number 12.34
using the Number
function. The result of the Number
function is then passed to the parseInt()
method. It then rounds it down to the nearest integer 12. The result of the parseInt()
method is then logged into the console.
Alternatives to the parseInt() function
In JavaScript, the parseInt()
function is commonly used to convert a string into an integer. However, there are alternative methods available for parsing integers or converting strings to numbers.
Here are some of the common alternatives to parseInt()
function:
1. Number
The Number()
function is another way to convert a string into a number, but it is more strict and less forgiving than the parseInt()
function. The Number()
function converts the entire string into a number. Returns NaN
if the string is not a valid number.
For example, Number("40")
returns 40
, but Number("40px")
returns NaN
. The Number()
function also does not have a radix parameter. It always assumes that the string is in decimal format.
console.log(Number("40")); // Output: 40
console.log(Number("40px")); // Output: NaN
2. parseFloat()
The JavaScript parseFloat() function is similar to the parseInt() function. But, it converts a string into a floating-point number or a number with a decimal part.
console.log(parseFloat("12.34")); // Output: 12.34
console.log(parseFloat("40")); // Output: 40
In this example, parseFloat("12.34")
returns 12.34
, and parseFloat("40")
returns 40
. The parseFloat()
function does not have a radix parameter. It always assumes that the string is in decimal format.
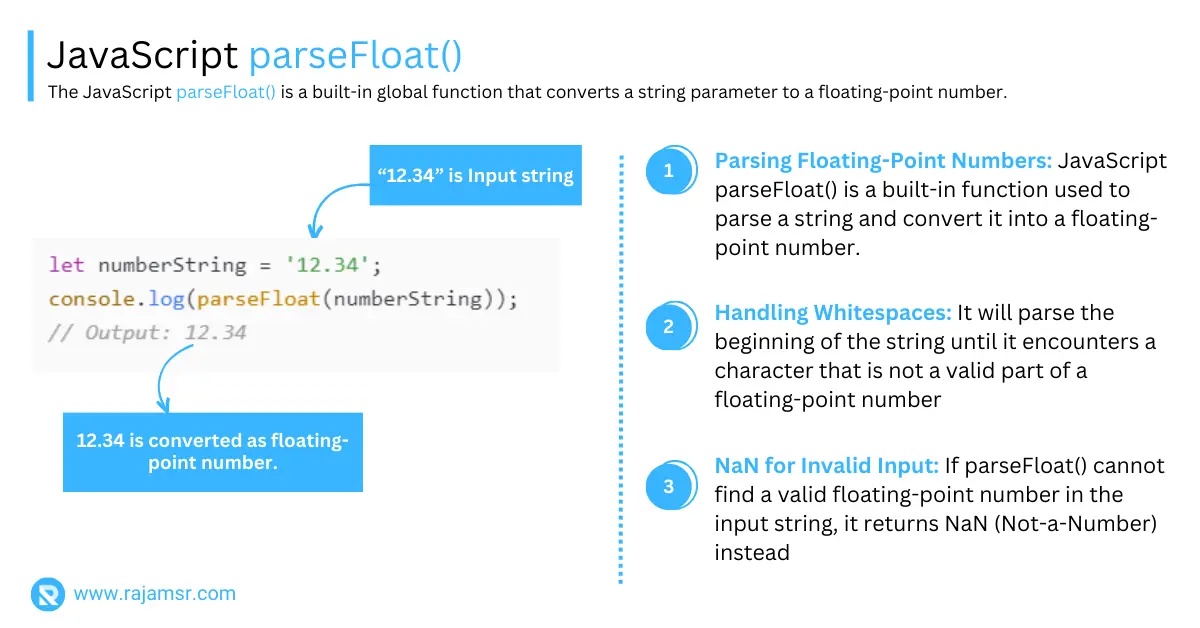
3. Math.floor()
The Math.floor()
is one of the JavaScript math functions that is not a conversion function that rounds a number down to the nearest integer. For example, Math.floor(3.7)
returns 3
, and Math.floor(-3.7)
returns -4
.
console.log(Math.floor("3.7")); // Output: 3
console.log(Math.floor("-3.7")); // Output: -4
console.log(Math.floor(parseFloat("3.7"))); // Output: 3
console.log(Math.floor(Number("3.7"))); // Output: 3
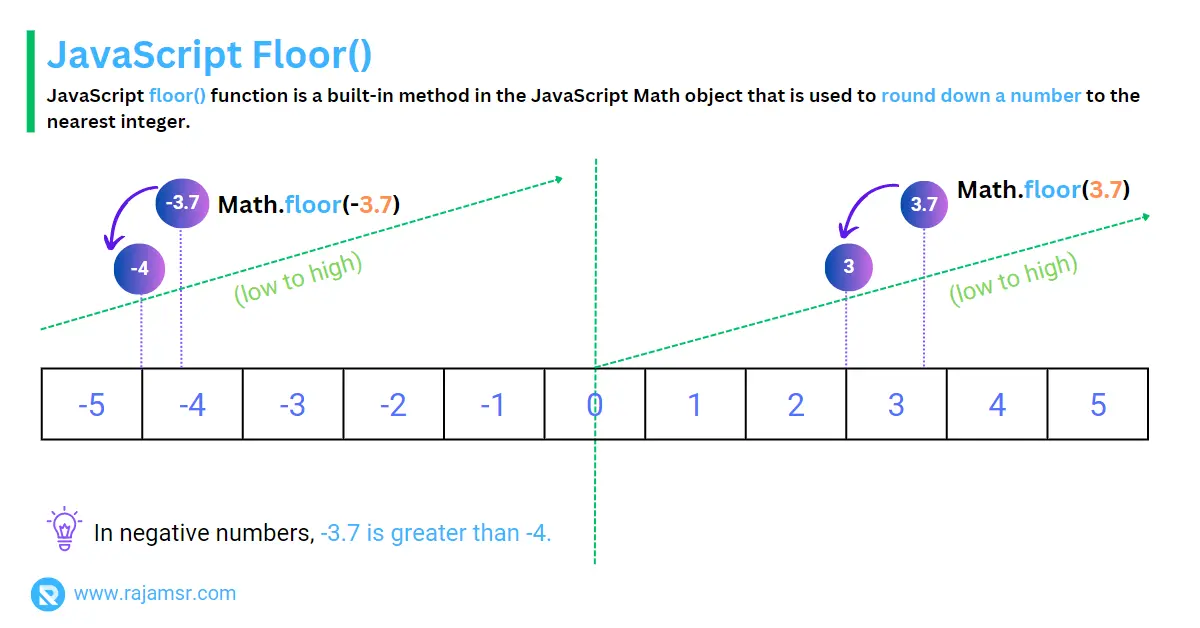
Math.floor()
function can be combined with the parseFloat()
or Number()
function to convert a string into an integer. For example, Math.floor(parseFloat("3.7"))
returns 3
, and Math.floor(Number("3.7"))
also returns 3
.Conclusion
In this blog post, you learned about the parseInt() function in JavaScript, and how to use it to convert strings into integers. You also learned about the radix parameter, the common pitfalls and errors of the function, and the other similar functions that you can use.
The parseInt() function is a useful tool for working with numeric values in JavaScript, but it also has some limitations and quirks that you need to be aware of. By following the tips and best practices that we discussed, you can avoid the common mistakes and make the most of the function.
So, if you’re looking to convert strings to integers in JavaScript, make sure to utilize the parseInt()
method and keep these tips and best practices in mind. With the right approach, you’ll be able to use this method effectively and avoid potential errors and unexpected results.