toLocaleDateString()
method. The toLocaleDateString()
method is a handy method that can convert a JavaScript date object to a string that represents the date in a format that is appropriate for the user’s locale. It’s like having your personal date translator!In this blog, we’ll take a closer look:- What is the
toLocaleDateString()
method? - What is the use of
toLocaleDateString()
? - How it works
- 5 Real-time examples using the
toLocaleDateString()
method
JavaScript Date localization using toLocaleDateString()
The toLocaleDateString()
is a method in the JavaScript Date object. It converts the date to local string format that’s appropriate for the user’s locale. It’s like having your own personal date translator!
The method returns a localized string representation of the date object. So, you can use it to display your user’s dates in their preferred format.
Assume that your user lives in the United States
and visits your website. You have to display the date in the MM/DD/YYYY
format. If another user browses your site from India
, the same date should appear in DD/MM/YYYY
format.
How JavaScript toLocaleDateString() format works?
The toLocaleDateString()
method works by using the user’s locale settings to determine the appropriate format for the date. The method takes into account the user’s time zone and other local settings to determine the correct format for the date.
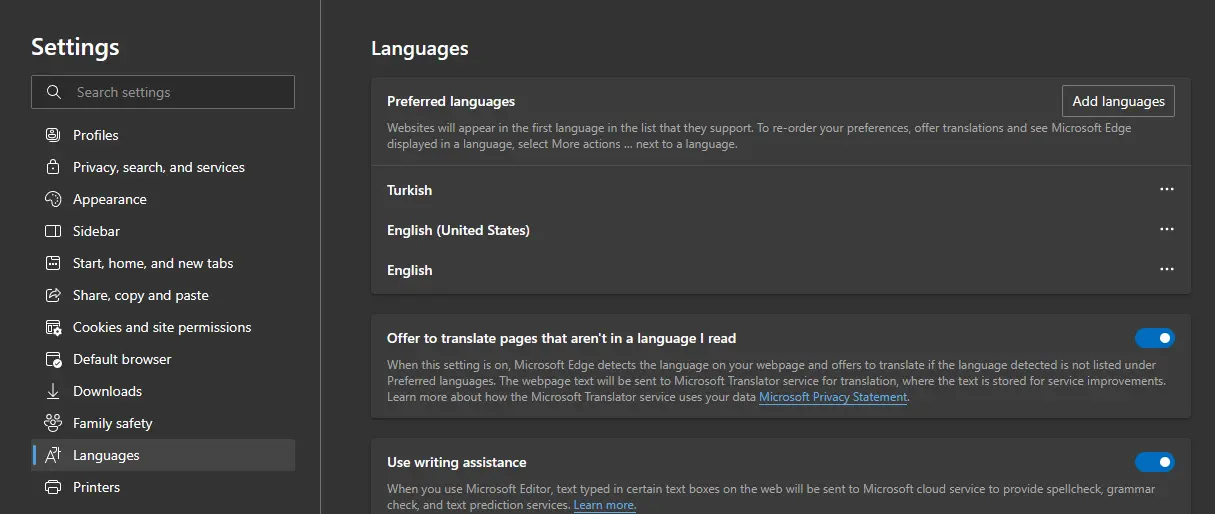
How to use date toLocaleDateString() method
JavaScript date localization using toLocaleDateString() method
To use the method, create a new JavaScript Date object and call the toLocaleDateString()
method on that object. It’s that easy!
Here’s an example of how to use toLocaleDateString():
var date = new Date();
var dateString = date.toLocaleDateString();
console.log(dateString);
// Output: "1/1/2024"
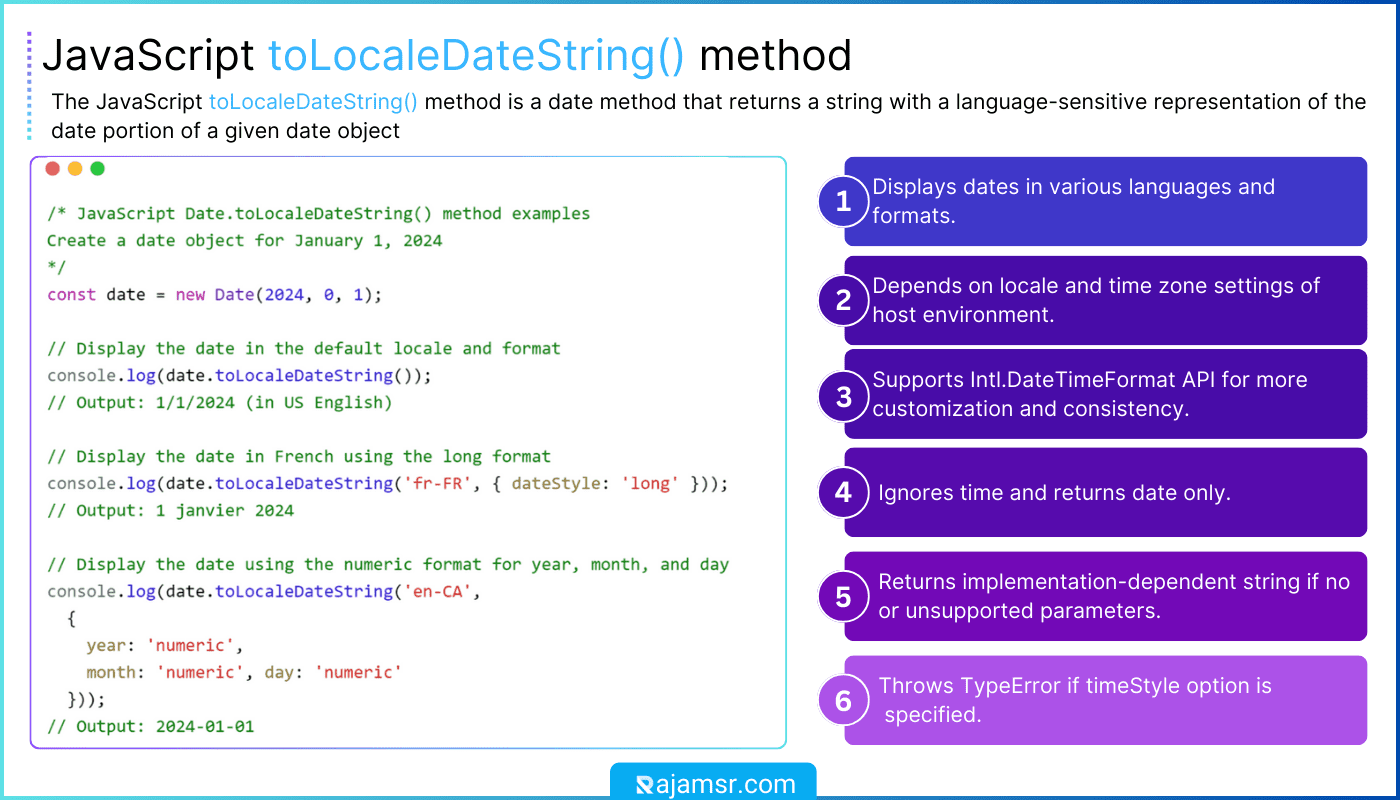
The above code creates a new Date
object and assigns it to the date variable. Then call the toLocaleDateString()
method on the date object and assign the result to a variable called dateString
. It’s that simple!
Using toLocaleDateString() Options Parameter
The toLocaleDateString()
method accepts an optional options parameter. To specify the locale
, the date format
, and the time zone
, you can use the options
parameter. This parameter is optional, but it can help you customize the output of the date and time methods.
Here’s an example of how to use the date toLocaleDateString()
options parameter:
var date = new Date();
var options = {
dateStyle: "short"
};
var dateString = date.toLocaleDateString("en-US", options);
console.log(dateString);
// Output: 3/26/23
The above code creates a new Date
object and assigns it to the date variable. Then the toLocaleDateString()
method is called on the date object, and the result is assigned to a variable called dateString
.
Setting the locale
To format the date, you use the locale property of the options object. This property specifies the locale
to which you want to apply. This means you can format dates in a way that makes sense for your users! To specify the locale, use a string that follows the format language region.
For example, en-US
for English (United States)
or fr-CA for French (Canada)
. You can easily avoid magic strings by using JavaScript Enum in your code.
Specifying the date format
To specify the format of the date, use the dateStyle
property of the options object. There are several possible values for this property, including:
- full: The system will display the
full date
, including theweekday
,month
,day
, andyear
. - long: The system will display a long format of the date, including the
weekday
,month
,day
, andyear
. - medium: The system will display a medium format of the
date
, including themonth
,day
, andyear
. - short: The system will display a short format of the date, including the
month
andday
.
Setting the time zone
To format the date, we use the options
object and timeZone
property to specify the time zone. This means you can format dates in a way that makes sense for your users in different time zones! The format Asia/Shanghai
specifies the time zone as a string.
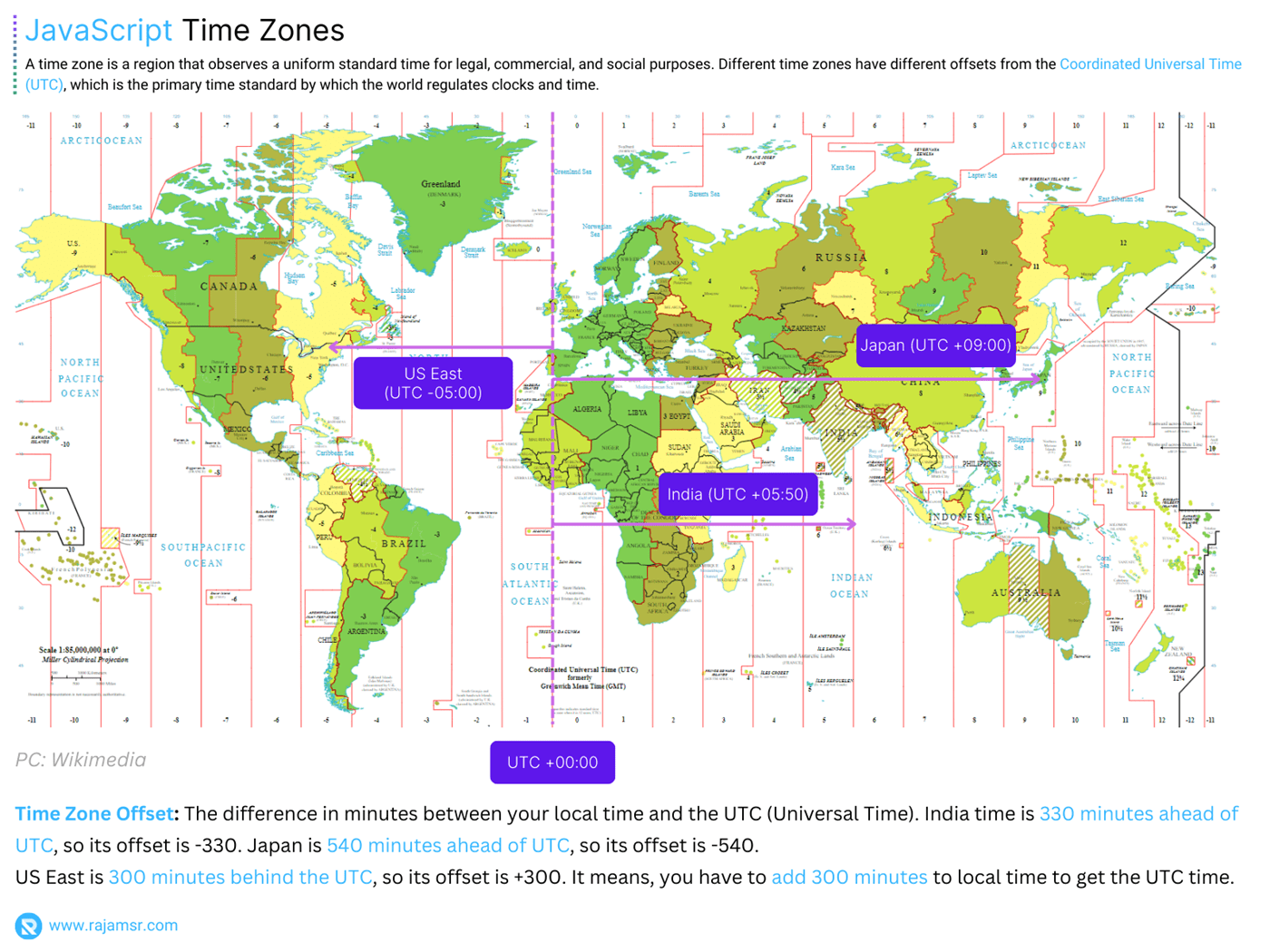
The date toLocaleDateString() examples
toLocaleDateString()
method.1. Basic JavaScript date format toLocaleDateString()
var date = new Date();
var dateString = date.toLocaleDateString();
console.log(dateString);
// Output: 3/26/2023
This example creates a new Date
object and assigns it to a variable called date
. Call the toLocaleDateString()
method on the date object and store the result in a variable called dateString
. Finally, we display the value of dateString
on the console.
2. Using the Options Parameter to Set the Locale
var date = new Date();
var options = {
locale: "en-US",
dateStyle: "short"
};
var dateString = date.toLocaleDateString("en-US", options);
console.log(dateString);
// Output: 3/26/23
In this example, we created a new Date object and assigned it to the date
variable. We called the toLocaleDateString()
method on the date object and stored the result in a variable called dateString
. Finally, we log the value of dateString
to the console.
3. Using the Options Parameter to Specify the Date Format
var date = new Date();
var options = {
dateStyle: "full"
};
var dateString = date.toLocaleDateString("en-US", options);
console.log(dateString);
// Output: Sunday, March 26, 2023
// Display the same date in Tamil (India)
dateString = date.toLocaleDateString("ta-IN", options);
console.log(dateString);
// ஞாயிறு, 26 மார்ச், 2023
In this example, we create an options object with one property: dateStyle
. To specify the full date format, we set the dateStyle
property to "full"
. Then we call the toLocaleDateString()
method on the date object and pass in the options object as the second parameter. The code stores the result in the variable dateString
and then logs it to the console.
4. Using the toLocaleDateString() Options Parameter to Set the Time Zone
var date = new Date();
var options = {
timeZone: "Asia/Shanghai"
};
var dateString = date.toLocaleDateString("zh-hk", options);
console.log(dateString);
// Output: 26/3/2023
In this example, we create an options object with one property: timeZone
. To specify the time zone Shanghai, China
, we set the timeZone
property to "Asia/Shanghai"
Then we call the toLocaleDateString()
method on the date object and pass in the options
object as the second parameter. The code stores the result in the variable dateString
and then logs it to the console.
5. Using the Options Parameter to Set the Locale, Date Format, and Time Zone
var date = new Date();
var options = {
timeZone: "Asia/Shanghai"
};
var dateString = date.toLocaleDateString("zh-hk", options);
console.log(dateString);
// Output: 26/3/2023
This example creates an options object with three properties: locale
, dateStyle
, and timeZone
. It uses these properties to format a date object according to the specified parameters.
- To specify the
United States English
locale, we set the locale property to“en-US”
. - To specify the full date format, we set the
dateStyle
property to“full”
. - To specify the time zone for
Shanghai, China
, we set thetimeZone
property to“Asia/Shanghai”
.
Then we call the toLocaleDateString()
method on the date object and pass in the options
object as the second parameter. The code stores the result in the variable dateString
and then logs it to the console.
6. Format dates in YYYY-MM-DD using toLocaleDateString()
The toLocaleDateString()
method can be used to format dates in the format YYYY-MM-DD.
let date = new Date();
let options = { year: 'numeric', month: '2-digit', day: '2-digit' }
let formattedDate = date.toLocaleDateString('en-GB', options)
.split('/')
.reverse()
.join('-');
console.log(formattedDate);
// Output: 2023-03-26
Troubleshooting "toLocaleDateString is not a function" error
In real-time, most of the time you might get input from a user or an external system. It might not be in the data type you expected. If you invoke the toLocaleDateString()
method on a non-date data type in this scenario, the exception "toLocaleDateString is not a function"
will be thrown.
var date = 'Hello, JavaScript';
var dateString = date.toLocaleDateString();
console.log(dateString);
// TypeError: date.toLocaleDateString is not a function
So, how to avoid this type of exception? Before calling the toLocaleDateString()
method, you can check the data type. To check if a variable is a date object in JavaScript, you can use the typeof
and instanceof
methods. Using the JavaScript typeof operator, you can check the type of object. You can check whether an object is an instance of a particular class using the JavaScript instanceof operator.
Here’s an example of how to avoid “.toLocaleDateString is not a function” error:
var date = new Date();
if (typeof date === 'object' && date instanceof Date) {
var dateString = date.toLocaleDateString();
console.log(dateString);
}
else {
console.log(`${date} is not date type.`)
}

Date()
then this method will not throw any exceptions. The message "... is not a date type"
will be printed in the browser console.You can use the third-party library moment.js to manipulate date and time in JavaScript.In JavaScript, you can add days or subtract days from a Date
object using simple arithmetic operations with milliseconds.Conclusion
We discussed the toLocaleDateString()
method in JavaScript and how it can display dates in the localized text.
We’ve seen how to utilize the toLocaleDateString()
method options parameter to set the locale
, date format
, and time zone
. I explained various examples of how to format dates in a localized format using the toLocaleDateString()
method.
Use typeof
and instanceof
type-checking methods to avoid the "toLocaleDateString is not a function"
error when using non-date types.
Whether you’re developing a web application or a standalone script, the toLocaleDateString()
method is a valuable function for formatting dates in a user-friendly manner.