Have you ever struggled with formatting dates in JavaScript? If you have, you are not alone. Many developers find it challenging to work with dates, especially when they need to use a specific JavaScript date format YYYY-MM-DD. This format is commonly used for storing and displaying dates in databases, APIs, and web applications.
In this post, I will show you:
- How to format date in yyyy-mm-dd
- JavaScript date format: yyyy-mm-dd hh:mm:ss
- Convert a string to a date in JavaScript
- Convert the date to a locale-specific format
By the end of this post, you will be able to format any date in JavaScript using the YYYY-MM-DD format with confidence and ease.
JavaScript date format yyyy-mm-dd example
JavaScript provides a built-in Date() constructor that simplifies working with date and time. The following code will format a date in yyyy-mm-dd
format:
const date = new Date();
const formattedDate = date.toISOString().slice(0, 10);
console.log(formattedDate);
// Output: 2023-05-07
In the above code, we first create a new JavaScript date object using the Date() constructor. We then use the toISOString() method. The toISOString()
method converts the date object to an ISO 8601 date format string.
Finally, we use the string JavaScript slice() method to extract the date (yyyy-mm-dd
) portion of the string.
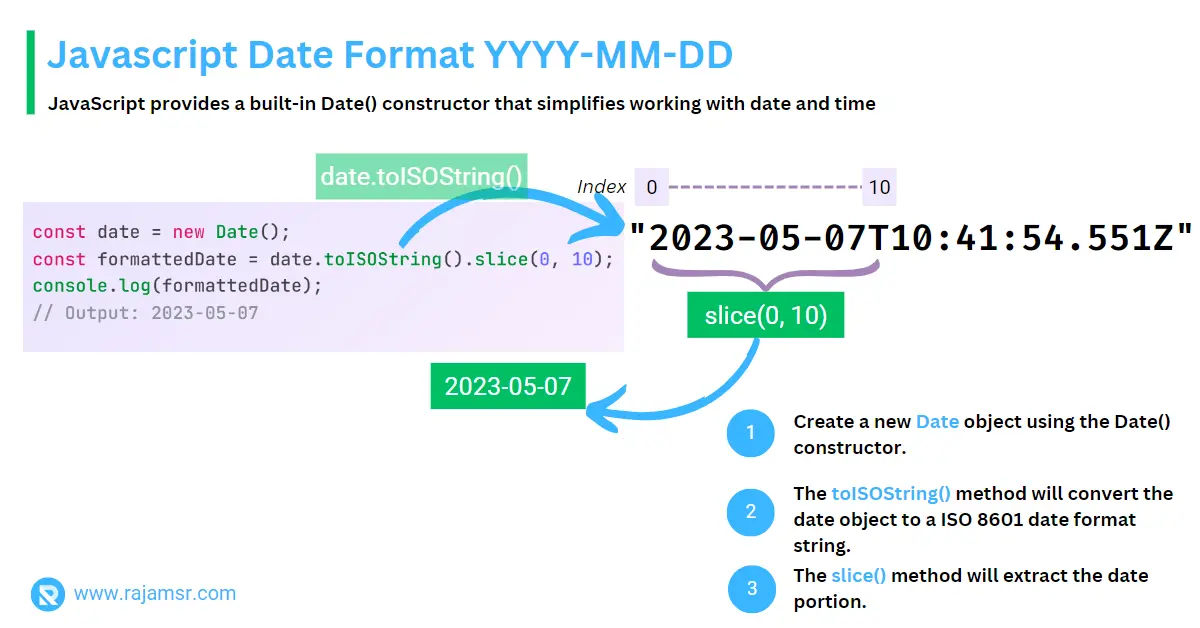
JavaScript format date yyyy-mm-dd hh:mm:ss
What if you wanted to display the time along with the date? To show the time as well as the date, you can apply the JavaScript toISOString() method and the JavaScript slice() method with different index parameters.
The following code demonstrates how to date format yyyy-mm-dd hh:mm:ss
const date = new Date();
const formattedDate = date.toISOString().slice(0, 19).replace('T', ' ');
console.log(formattedDate);
// Output: 2023-05-07 06:22:28
The above code creates a new Date object with the Date() constructor
. Then, it converts the date object to a string in the ISO 8601 format with the toISOString() method.
The slice()
method extracts the date and time portion of the string yyyy-mm-dd hh:mm:ss
and replaces the ‘T’
separator with an empty space.
How to convert a string to a date in JavaScript?
Let’s assume you are getting a date
input as a string and you want to convert it to a date. If you have a valid date string, then you can use the following code to convert the string to a date:
const dateString = '2022-05-07';
const numberOfMilliSeconds = Date.parse(dateString)
console.log(new Date(date))
// Output: Thu Jan 01 1970 05:30:00 GMT+0530 (India Standard Time)
We use the Date.parse()
method in the above code to convert a date. The Date.parse()
method returns the number of milliseconds between a date and midnight Jan 1, 1970
by parsing a string containing the date. We can create a date with the Date() constructor
using those milliseconds.
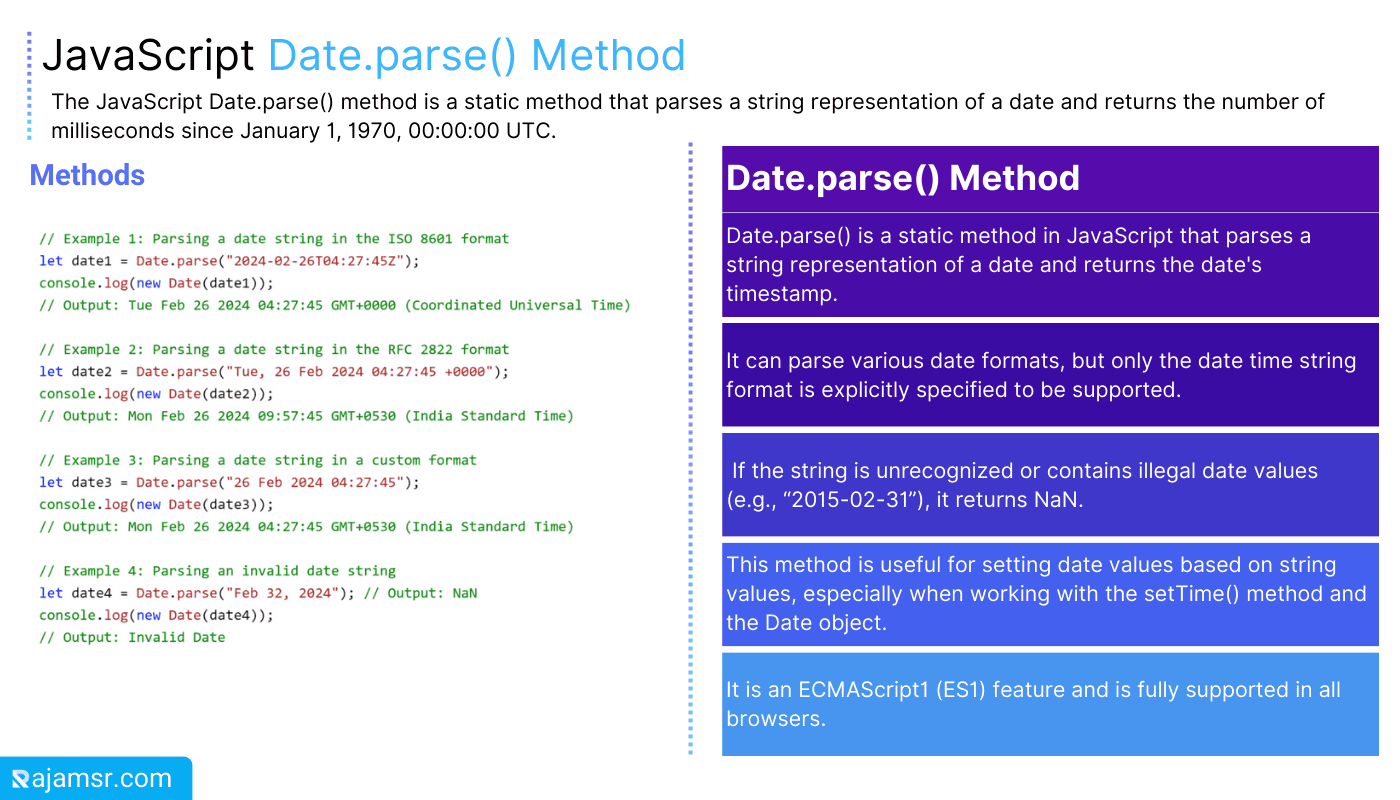
Let’s assume you have a date string in the yyyy-mm-dd
format and you need to convert it to a date object. You can use the following code:
const dateString = '2022-05-07';
const dateParts = dateString.split('-');
const year = parseInt(dateParts[0]);
const month = parseInt(dateParts[1]) - 1;
const day = parseInt(dateParts[2]);
const date = new Date(year, month, day);
console.log(date);
// Output: Sat May 07 2022 00:00:00 GMT+0530 (India Standard Time)
In the above code, we first define a string variable dateString
with the value '2022-05-07'
. Using the string split()
method, split the string into an array of three parts: year
, month
, and day
. Then convert each part into an integer using the JavaScript parseInt() method.
We create a new date object using the Date() constructor
and the year, month, and day variables.
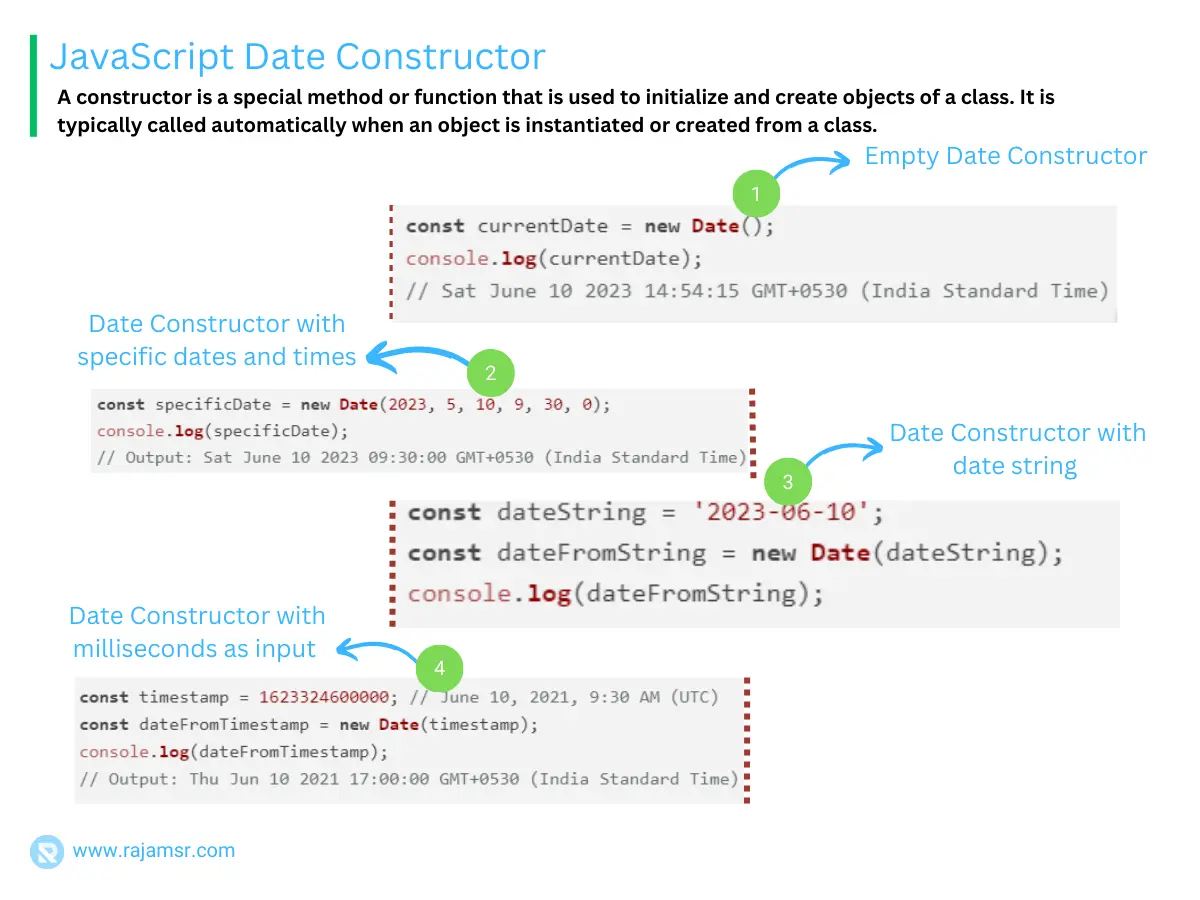
Localize date using toLocaleDateString() format yyyy-mm-dd
If you need to format the date in a specific locale, you can use the toLocaleDateString() method. Here’s an example date format yyyy-mm-dd using toLocaleDateString() with “en-CA” locale:
var date = new Date();
var options = { year: "numeric", month: "2-digit", day: "2-digit" };
var formattedDate = date.toLocaleDateString("en-CA", options);
console.log(formattedDate);
// Output: "2024-01-28"
In the above code, a new Date
object is created using the Date() constructor
. You can use an options object to customize the date, which specifies the date format you want to use. The toLocaleDateString()
method formats the date in the specified locale. Date format is depends on the locale.
JavaScript toLocaleDateString() format yyyy-mm-dd hh:mm:ss
To format a JavaScript date using toLocaleDateString() with the format yyyy-mm-dd hh:mm:ss, you can use the following code. Add the options parameter values for the time component and replace the comma from the result string.
var date = new Date();
let options = { year: 'numeric',
month: '2-digit',
day: '2-digit',
hour: '2-digit',
minute: '2-digit',
second: '2-digit',
hour12: false };
var formattedDate = date.toLocaleDateString("en-CA", options);
console.log(formattedDate.replace(',',''));
// Output: "2024-03-03 17:02:10"
How to format a date in JavaScript in the mm/dd/yyyy format?
The following code will format the current date in the frequently used mm/dd/yyyy
format.
const date = new Date();
const month = date.getMonth() + 1;
const day = date.getDate();
const year = date.getFullYear();
const formattedDate = `${month}/${day}/${year}`;
console.log(formattedDate);
// Output: 5/7/2023
In the above code, a new Date
object is created using the Date() constructor. You can use the getMonth()
, getFullYear()
, and getDate()
methods to extract the month, day, and year from the date object.
Finally, we use string interpolation to format the date in the mm/dd/yyyy
format. By customizing this method, you can format JavaScript dates in any format that you require without using any third-party libraries like moment.js.
Conclusion
This blog post explored the JavaScript date format yyyy-mm-dd
. In addition to this, we explored how to format dates with the time yyyy-mm-dd hh:mm:ss
format and the mm/dd/yyyy
format.
To format dates in a specific locale, you have to use the toLocaleDateString()
method with custom options.
Using date methods like getMonth()
, getDate()
, and getFullYear()
, you can display dates in any format without using third-party libraries.