Have you ever wondered what the JavaScript double question mark operator does? If you are a web developer, you might have encountered this syntax in some code snippets or tutorials. But what does it actually mean and how can it help you write better code?
What does double question mark mean? The JavaScript double question mark operator, also known as the nullish coalescing operator, is a logical operator that returns the right-hand side operand if the left-hand side operand is null or undefined, and otherwise returns the left-hand side operand. This can be very useful for providing default values for variables or parameters that might be missing or falsy.
In this comprehensive blog, we’ll dive deep into:
- The double question mark operator in JavaScript
- Nullish coalescing operator syntax
- Explore its practical usage with code examples
- Falsy values in JavaScript
- Key differences compared to the double pipe operator (||)
Let’s dive in.
What is JavaScript double question mark operator?
The double question mark operator (??)
is a relatively new addition to JavaScript. It is known as the nullish coalescing operator
and is used to provide a concise way of assigning default values when dealing with null
or undefined
values.
Syntax of nullish coalescing operator
It takes the form of LHS ?? RHS
, where LHS
is the expression to be evaluated, and RHS is the default value assigned if the LHS
expression is null
or undefined
. The Nullish Coalescing Operator (??)
in JavaScript provides a concise way to assign a default value when a JavaScript variable is null
or undefined
.
The syntax for using the nullish coalescing operator
is as follows:
const result = variable ?? defaultValue;
Here, the variable is the expression to be evaluated, and defaultValue
is the value to be assigned if the variable is null
or undefined
.
Usage of the double question mark operator (??)
Here’s an example demonstrating the basic usage of the double question mark in JavaScript:
const userName = null;
const defaultName = "Hello, Guest!";
const result = userName ?? defaultName;
console.log(result);
// Output: "Hello, Guest!"
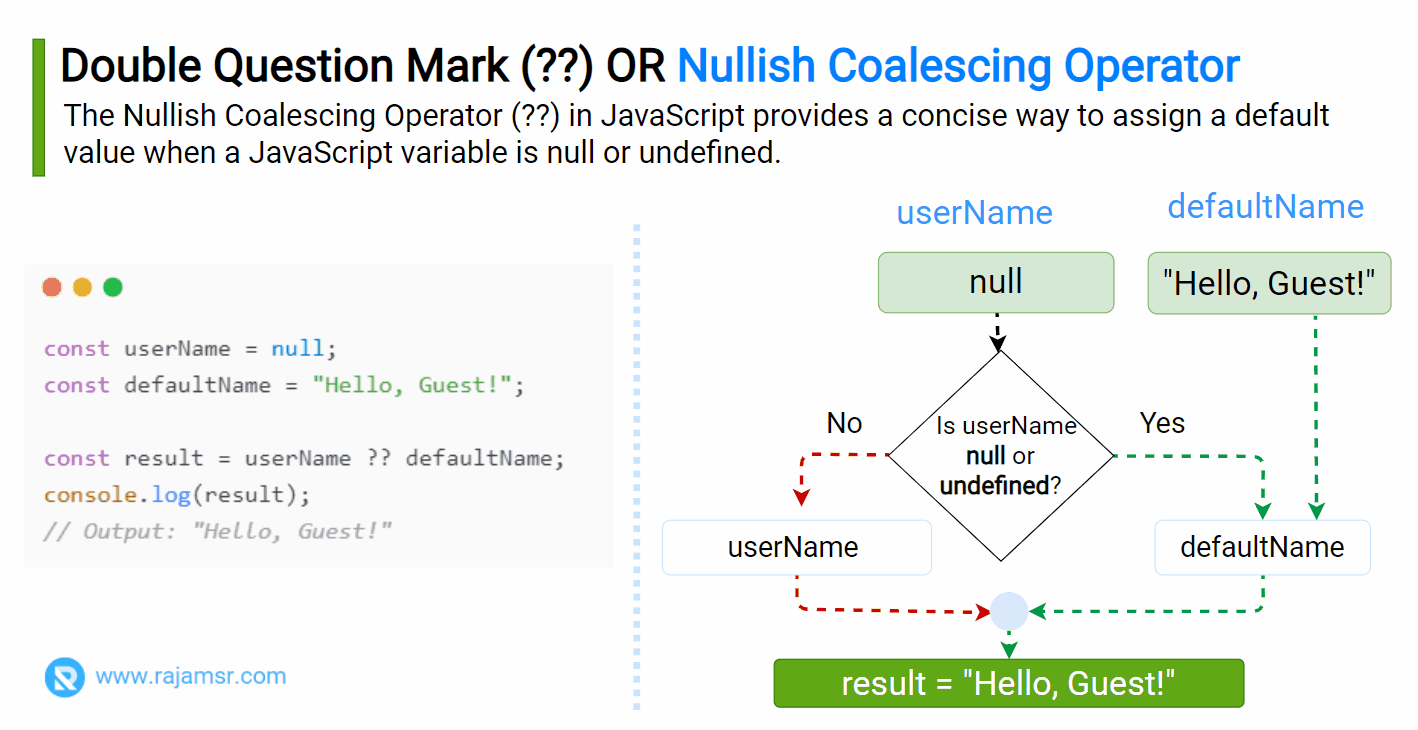
In the above example, the variable name is assigned a null
value. When we use the double question mark operator to assign a default value, it checks if the username
is null or undefined. Since it is null, the default value defaultName
is assigned to the result.
What are the values are considered as falsy in JavaScript?
falsy
:Value | Description |
---|---|
false | The boolean value false. |
0 | The number zero. |
“” | An empty string. |
null | A null value. |
undefined | An undefined value. | NaN | Not-a-Number value. |
When evaluating boolean expressions, these values are treated as false
and will result in a false boolean value. All other values, including non-empty strings
, non-zero numbers
, objects
, and arrays
, are considered truthy
and will result in a true boolean value when evaluated.
Practical examples of the double question mark operator
Let’s see a few real-time examples of how to use double question marks in JavaScript.
1. Assigning Default Values
Here’s a perfect code example demonstrating the use of the double question mark operator:
function greetUser(username) {
const name = username ?? "Guest";
console.log(`Welcome, ${name}!`);
}
greetUser(); // Output: Welcome, Guest!
greetUser("John"); // Output: Welcome, John!
greetUser(null); // Output: Welcome, Guest!
greetUser(undefined); // Output: Welcome, Guest!
In the above example, the function greetUser()
takes a username parameter. By using the double question mark operator, we assign the default value "Guest"
if username
is null or undefined.
2. Nullish Coalescing with Falsy Values
const value = 0;
const result = value ?? 40;
console.log(result); // Output: 0
Here, the variable value has a false value of 0. However, since the double question mark operator checks only for null
or undefined
values, it considers 0 as a valid value and assigns it to the result.
JS Double question mark vs double pipe (||) operators
The double pipe operator (||) is a logical OR operator that performs a boolean comparison between the LHS and RHS expressions. It returns the first truthy value encountered when evaluated from left to right. If all expressions are false, it returns the last expression.
Let’s look at an example using the double pipe operator:
const name = "";
const defaultName = "John Doe";
const result = name || defaultName;
console.log(result);
// Output: "John Doe"
In this example, the variable name is an empty string. When we use the double pipe operator, it evaluates the name as a false value. Since it is false, it proceeds to evaluate the next expression (defaultName
) and assigns it to the result.
Difference between JavaScript double question mark vs double pipe
The double question mark ?? and double pipe || are both used in JavaScript for handling default values, but they have some key differences in their behavior. Here are the few differences between double question mark and double pipe:
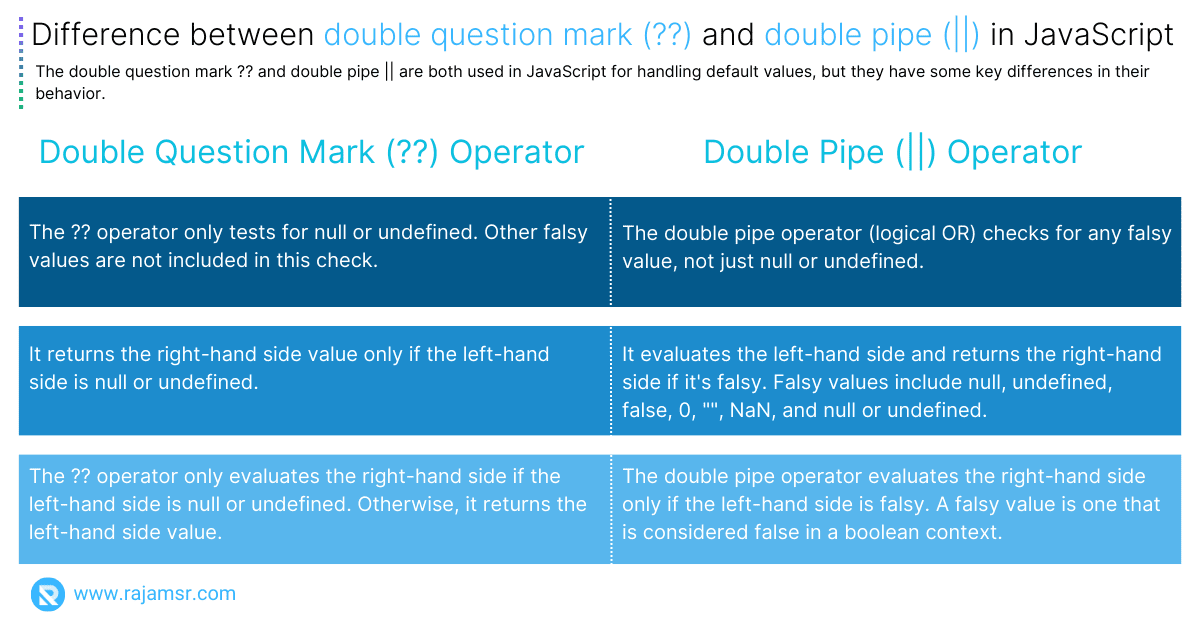
Here are some examples of how JavaScript double pipe and double question mark operators will work with different input values:
const value1 = 0;
const value2 = "";
const value3 = false;
const value4 = null;
const value5 = undefined;
console.log(value1 ?? "Default"); // Output: 0
console.log(value1 || "Default"); // Output: "Default"
console.log(value2 ?? "Default"); // Output: ""
console.log(value2 || "Default"); // Output: "Default"
console.log(value3 ?? "Default"); // Output: false
console.log(value3 || "Default"); // Output: "Default"
console.log(value4 ?? "Default"); // Output: "Default"
console.log(value4 || "Default"); // Output: "Default"
console.log(value5 ?? "Default"); // Output: "Default"
console.log(value5 || "Default"); // Output: "Default"
Choose the operator that best suits your specific use case to ensure accurate and expected results in your JavaScript code.
Double question mark equals in JavaScript (??==)
The ??=
is similar to the Nullish Coalescing Operator
, which was introduced as a logical null assignment implementation in other languages.
The logical nullish assignment operator (x ??= y)
only assigns if x is null (null or undefined). It simply looks for null
and undefined
values, nothing else.
let userName = null;
let defaultUserName = "John!";
console.log(userName ??= defaultUserName)
// Output: "John!"
Common errors and pitfalls
Handling double question mark unexpected token error
When using the double question mark operator, ensure that your JavaScript environment supports ECMAScript 2020 (or a newer version) to avoid unexpected token errors. Older browsers or environments may not recognize this operator. To handle this situation and ensure compatibility with older browsers, you can use alternative approaches.
Here’s one common approach using the logical OR operator (||) in combination with the ternary operator (? :)
var result = (someValue !== null || someValue !== undefined)
? someValue
: defaultValue;
In this example, someValue
is the value you want to check for nullish
values and is the fallback value you want to use if someValue
is null or undefined.
The logical OR operator || returns the first truthy value it encounters or the last value if all operands are false. By checking someValue !== null || someValue !== undefined
, we ensure that the value is neither null nor undefined before considering it true. If someValue
is null or undefined, the ternary operator will assign defaultValue
to the result variable.
Double question mark operator and empty strings
Keep in mind that the double question mark operator does not treat empty strings ("")
as null or undefined. If you need to check for JavaScript empty strings, consider using alternative approaches like the logical OR operator (||) or explicitly checking the JavaScript string length.
const userName = "";
const defaultName = "Hello, Guest!";
const result = userName ?? defaultName;
console.log(result);
// Output: ""
Polyfill method for nullish coalescing operator
If you want to create a polyfill for this operator, you can do so by defining a function that mimics its behavior using conditional checks.
Here’s a simple polyfill
for the nullish
coalescing operator:
if (!('??' in window)) {
window['??'] = function (left, right) {
return left !== null && left !== undefined ? left : right;
};
}
const someValue = null;
const defaultValue = "Default Value";
const result = window['??'](someValue, defaultValue);
console.log(result);
// Output: "Default Value"
In this polyfill
, we first check if the ??
(two question marks) operator is already defined in the global window object to avoid overwriting it if it already exists. Then, we define a function that takes two arguments, left and right. If left is not null or undefined, it returns left; otherwise, it returns right, which serves as the default value.
Conclusion
The JavaScript nullish coalescing operator (??)
is a handy feature that lets you assign a default value to a variable if the original value is null or undefined. This can help you avoid errors and simplify your code.
In this blog post, we have seen how to use the nullish coalescing operator in different scenarios, such as checking for user input, setting default parameters, and accessing nested properties. We have also learned how the nullish coalescing operator
differs from the logical OR operator (||)
and when to use each one.
The nullish coalescing operator (??)
is a modern addition to JavaScript that can make your code more readable and robust. If you haven’t tried it yet, give it a try and see how it can improve your coding experience.