Are you looking for a solution for how to check if a JavaScript string is empty, null, or undefined? As a JavaScript developer, you’ll often encounter situations where you need to check if a string is empty, null, undefined, or even contain only whitespace.
In this article, I will show you:
- What is JavaScript empty string?
- Why empty strings matter?
- Five different methods to effectively check empty string
- Three different ways to handle string empty
- Handling null, and undefined strings
- Testing for empty, null, or space strings
- Best practices for dealing with empty strings
So, let’s dive in!
What is JavaScript empty string?
An empty string is simply a string without any characters. It is denoted by two quotation marks with nothing in between, like this “”. It’s important to distinguish an empty string
from null
or undefined
values, as they have different meanings in JavaScript.
Why empty strings matter?
An empty string is different from other falsy values in JavaScript, such as null, undefined, 0, false, or NaN. An empty string is still a string, and it has a length property of zero.
Empty strings can occur in various scenarios, such as:
- User Input: A user may enter nothing or press the spacebar in a form field, resulting in an empty string.
- Data Manipulation: You may split, slice, or splice a string and end up with an empty string as a result.
- API Response: You may receive an empty string from an external source, such as a web service or a database.
If you don’t check and handle empty strings, you may encounter some problems, such as:
- Validation Errors: You may want to validate the user input and make sure it is not empty before submitting it to the server.
- Type Errors: You may want to perform some operations on the string, such as concatenation, conversion, or calculation, and get an unexpected result or an error.
- Logic Errors: You may want to use the string as a condition or a parameter in a function, and get a wrong output or a side effect.
Therefore, it is important to check and handle empty strings in JavaScript to avoid these issues and ensure the quality and functionality of your code.
How to check if a JavaScript string is empty?
There are a lot of ways to check if a string is empty in JavaScript. However, in this post, we will see the top three approaches.
1. Check string empty using length property in JavaScript
One of the simplest ways to check if a string is empty is by utilizing the JavaScript string length property. This property returns the number of characters in a string. If the length is 0
, it means the string is empty. If the length is greater than 0
, then the string is not empty.
Here’s an example of how to check a JavaScript empty string:
// Using the string length property to check empty string
let string1 = ""; // Empty string
let string2 = " "; // String with one space
let string3 = "JavaScript"; // Non-empty string
let string4 = "0"; // String with zero
let string5 = "false"; // String with false
console.log(string1.length === 0); // Output: true
console.log(string2.length === 0); // Output: false
console.log(string3.length === 0); // Output: false
console.log(string4.length === 0); // Output: false
console.log(string5.length === 0); // Output: false
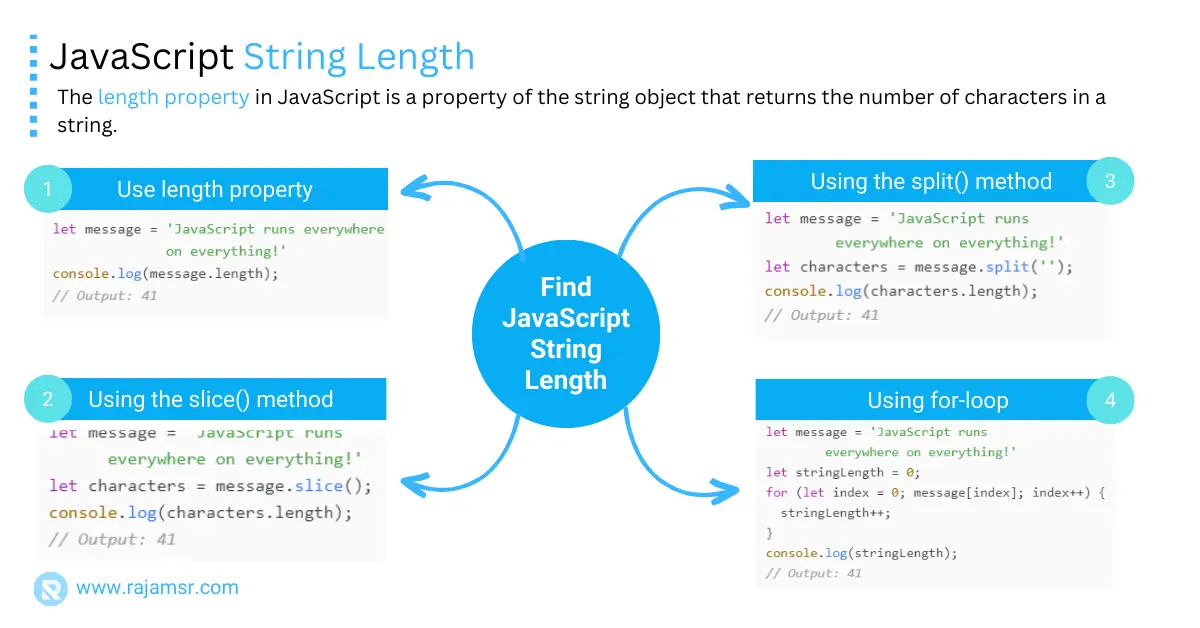
2. Utilizing the trim() method to check empty string
The trim()
method removes whitespace from both ends of a string. By applying JavaScript trim() to a string, we can check if it becomes empty after removing whitespace from the string. Take a look at the following code snippet:
// Check empty string using trim() method
const string1 = ''; // Empty String
const string2 = ' '; // String with space
const string3 = '\t\n'; // Whitespace characters
const string4 = ' JavaScript '; // String with leading and trailing space
const string5 = 'true'; // Boolean string
console.log(string1.trim() === '') // Output: true
console.log(string2.trim() === '') // Output: true
console.log(string3.trim() === '') // Output: true
console.log(string4.trim() === '') // Output: false
console.log(string5.trim() === '') // Output: false
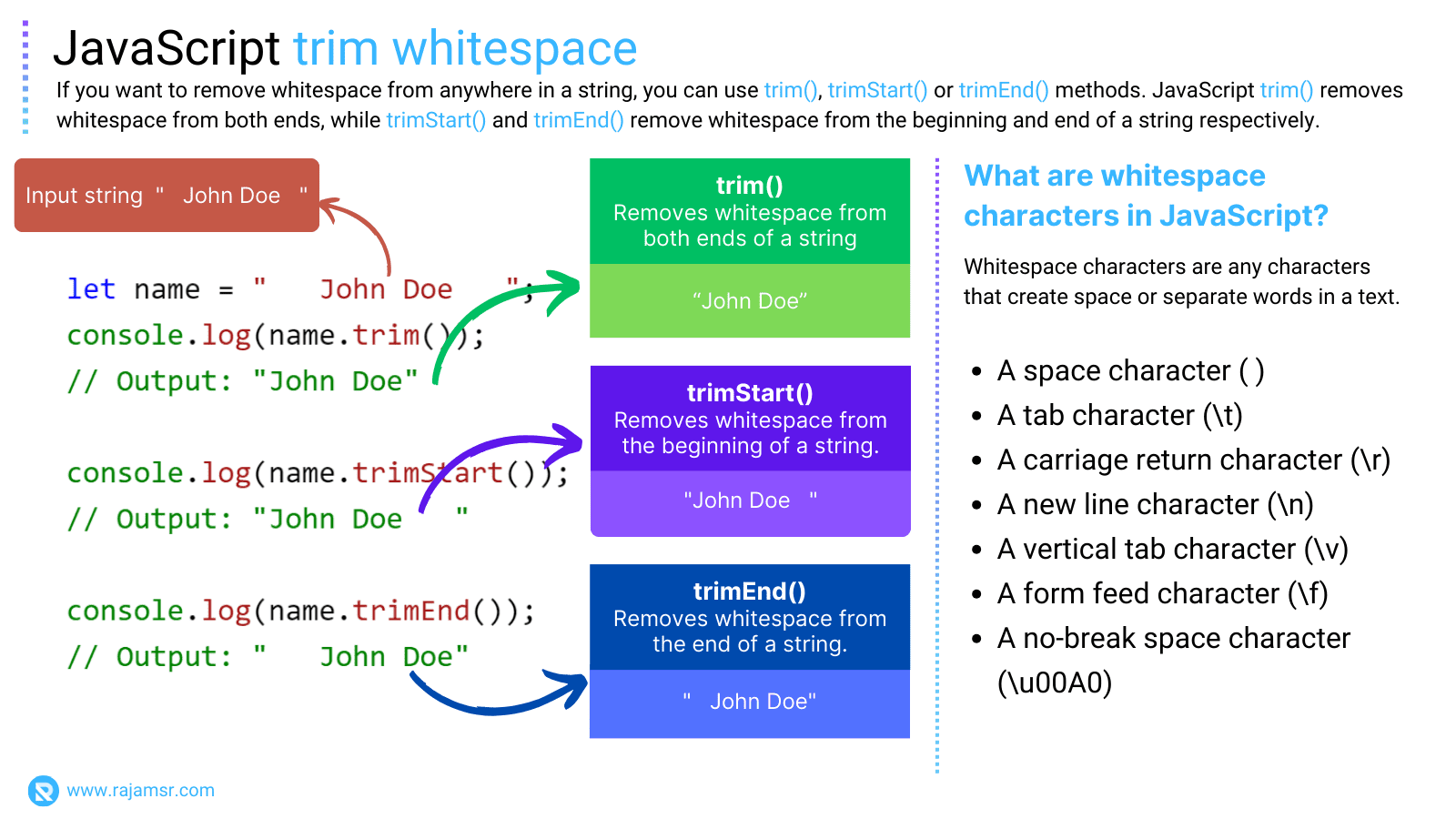
3. Using the Boolean constructor
You can convert the string to a boolean value, and then check if it is false. This is a concise and elegant way to check for empty strings, but it may not be intuitive, and it may also return false for other falsy values, such as 0 or false.
// Using the Boolean constructor to check empty string
let string1 = ""; // Empty string
let string2 = " "; // String with one space
let string3 = "JavaScript"; // Non-empty string
let string4 = "0"; // String with zero
let string5 = "false"; // String with false
console.log(Boolean(string1) === false); // Output: true
console.log(Boolean(string2) === false); // Output: false
console.log(Boolean(string3) === false); // Output: false
console.log(Boolean(string4) === false); // Output: false
console.log(Boolean(string5) === false); // Output: false
4. Using the ! operator to check empty string
You can use the logical NOT operator to negate the string, and then check if it is true. This is a shorthand and expressive way to check for empty strings, but it may also be confusing, and it may also return true for other falsy values, such as 0 or false.
// Examples: Using the ! operator to check empty string
let string1 = ""; // Empty string
let string2 = " "; // String with one space
let string3 = "Hello"; // Non-empty string
let string4 = "0"; // String with zero
let string5 = "false"; // String with false
console.log(!string1 === true); // Output: true
console.log(!string2 === true); // Output: false
console.log(!string3 === true); // Output: false
console.log(!string4 === true); // Output: false
console.log(!string5 === true); // Output: false
5. Applying regular expressions to check for empty string
Regular expressions are powerful methods for pattern matching. We can use regular expressions
to check if a string is empty by searching for any non-space characters. Here’s an example:
// Check empty string using regular expression
const string1 = ''; // Empty String
const string2 = ' '; // String with space
const string3 = '\t\n'; // Whitespce characters
const string4 = ' JavaScript '; // String with leading and trailing space
const string5 = 'true'; // Boolean string
console.log(/^\s*$/.test(string1)) // Output: true
console.log(/^\s*$/.test(string2)) // Output: true
console.log(/^\s*$/.test(string3)) // Output: true
console.log(/^\s*$/.test(string4)) // Output: false
console.log(/^\s*$/.test(string5)) // Output: false
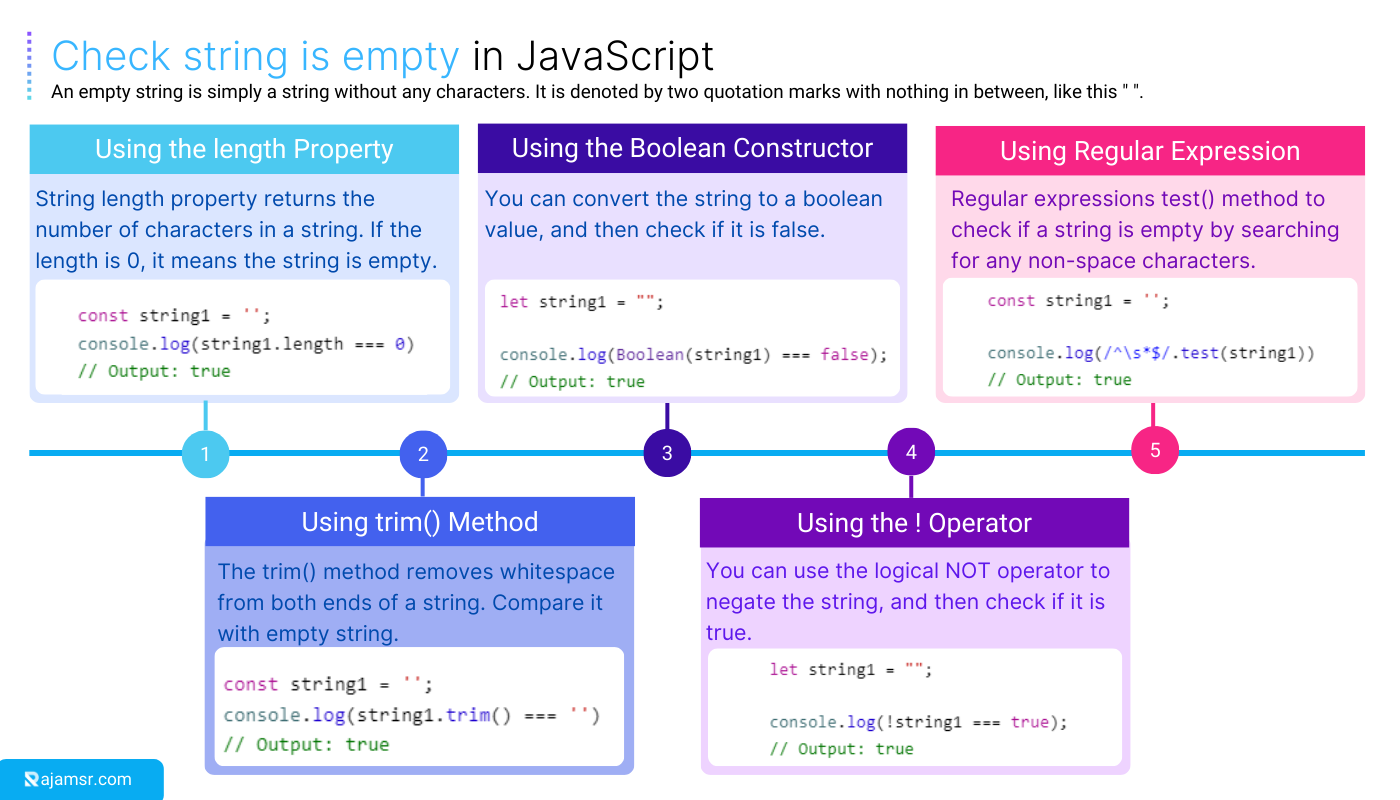
How to handle empty strings?
Once you have checked for empty strings, you may want to handle them in different ways, depending on your needs and preferences. Here are three most common ways to handle empty strings in JavaScript:
1. Using a default value
You can assign a default value to the string if it is empty, like this: str = str || “default”. This is a convenient and concise way to handle empty strings, but it may also assign the default value to other falsy values, such as 0 or false.
// Using a default value
let defaultUser = "Guest";
let uesrname = "John";
// Assign defaultUser to string variable only if left string is empty
let string1 = "" || defaultUser;
let string2 = uesrname || defaultUser;
console.log(string1); // "Guest"
console.log(string2); // "John"
2. Using a ternary operator
You can use a conditional operator to check if the string is empty, and then return a different value. This is a more explicit and flexible way to handle empty strings, but it may be less readable and more verbose.
// Using ternary operator
let defaultUser = "";
let uesrname = "Guest";
console.log(defaultUser ? defaultUser : uesrname); // Output: "Guest"
defaultUser ="John"
console.log(defaultUser ? defaultUser : uesrname); // Output: "John"
3. Using an if statement
You can use a conditional statement to check if the string is empty, and then execute some code. This is a more clear and structured way to handle empty strings, but it may be more complex and require more lines of code.
// Using if statement
let defaultUser = "Guest";
let uesrname = "";
if (uesrname.length === 0) {
uesrname = defaultUser;
}
console.log(uesrname); // Output: "Guest"
Comparison of string empty and handing method Pros and Cons
To help you choose the best method for checking and handling empty strings in JavaScript, here is a comparison table that shows the pros and cons of each method:
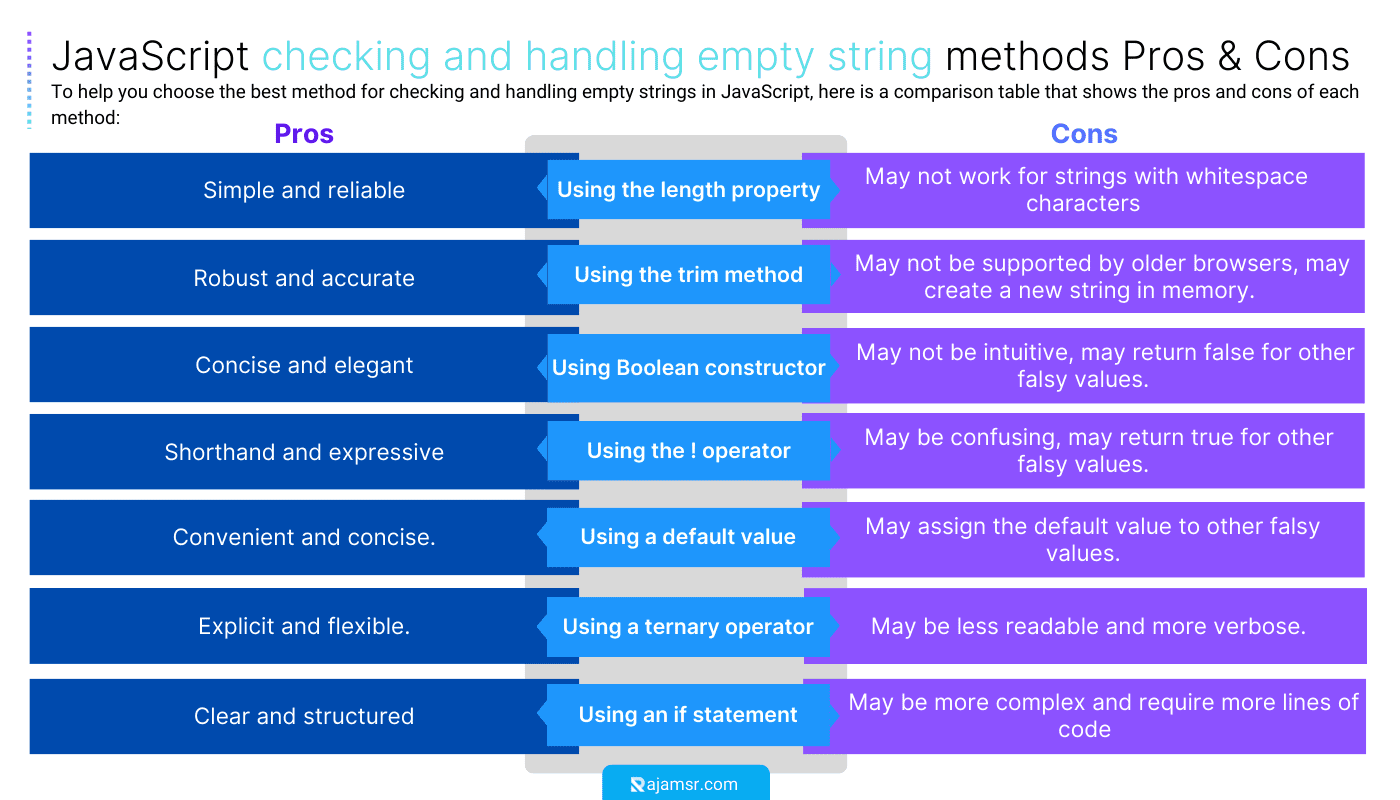
Handling null, or undefined strings
Now we know how to check if a string is empty. Let’s explore how to handle strings that are null
or undefined
as well. It’s crucial to differentiate between these values to ensure appropriate handling. Consider the following guidelines:
How to check if a JavaScript string is null
A JavaScript null
represents the absence of any value. It’s essential to check for null
explicitly using the strict JavaScript string equality operator (===
) like this:
const message = null;
if (message === null) {
console.log("The message is null.");
}
// Output: "The message is null."
Undefined Strings
Undefined signifies the absence of a defined value. To check for undefined
strings, you can use the JavaScript typeof operator like this:
let message;
if (typeof message === "undefined") {
console.log("The message is undefined.");
}
// Output: "The message is undefined."
Checking JavaScript string null or whitespace
In some cases, you might also need to consider strings that contain only whitespace characters. To test if a JavaScript string is null
, empty
, or whitespace
, you can use the following code:
let message = ' ';
if (message && message.trim() !== '') {
console.log("The string is NOT empty.");
} else {
console.log("The string is empty, null, or contains only whitespace.");
}
In the above code, the JavaScript trim() method will remove all whitespace characters from the beginning or end of the string.
Removing empty strings from array in JavaScript
Empty strings in arrays can affect the performance and clarity of your code. You can use these methods to remove them and improve your code quality.
function removeEmptyTitles(titles) {
return titles.filter(b => b !== "");
}
const bookTitles = [
'Eloquent JavaScript',
'',
'',
'Effective Javascript'
];
console.log(removeEmptyTitles(bookTitles))
// Output: ['Eloquent JavaScript', 'Effective Javascript']
Best practices for dealing with empty strings
When handling strings, it’s essential to practice defensive programming and account for edge cases. Here are a few best practices to consider:
- Always check for null and undefined values explicitly.
- Document your code to clarify how empty strings are handled.
- Regularly test your code with various input scenarios to ensure robustness.
Frequently asked questions
How to append to an empty string in JavaScript?
To append to an empty string, you can simply use the +=
operator. Here is an example:
let emptyString = ''; emptyString += 'Appended text'; console.log(emptyString); // Output: 'Appended text'
How to remove empty spaces in a string in JavaScript?
whitespace
) from a string using the trim()
method.const textWithSpace = ' Remove spaces '; const trimmedString = textWithSpace.trim(); console.log(trimmedString); // Output: 'Remove spaces'
How to replace an empty string with '0' in JavaScript?
You can replace an empty string with '0'
using the replace()
method. Here’s an example
let message = ''; message = message.replace('', '0'); console.log(message); // Output: '0'
Conclusion
Handling empty strings in JavaScript is a fundamental aspect of writing clean and reliable code.
By using the methods we’ve discussed, such as checkinglength
, applying trim()
, or utilizing regular expressions
, you can effectively detect and handle empty
, null
, undefined
, and whitespace strings
.
Remember to follow best practices and consider edge cases to enhance the quality of your code.