Are you tired of struggling with sorting dates in your JavaScript projects? Look no further! Sorting dates in JavaScript can be a daunting task, especially when considering the complexities of different date formats, time zones, and sorting criteria.
I have curated this guide to provide you with practical techniques, powerful strategies, and code examples that will empower you to master JavaScript date sorting. Whether you’re sorting dates in ascending or descending order, handling date ranges, or sorting arrays of objects, we have you covered.
In this blog, we will explore:
- Sort an array of dates in ascending or descending order.
- Sort by date and time
- Sort an array of objects by date
- How to use Moment.js library to sort
- Sort by date string
- Sort by date and filter
- Sort by date and other property
- Sort by ISO date
- Sort by date week and more
Get ready to sort by date like a true JavaScript pro!
JavaScript sort by date ascending
To sort an array of dates in ascending order, we can use the basic sort()
function provided by JavaScript. The sort()
function sorts elements of an array in place and uses a comparison function to determine the order.
To sort the elements in ascending order, the sort()
method first converts them to strings. Then, it compares the strings based on their UTF-16
code units values, which are numerical representations of each character.
const dates = ["2023-07-09", "2022-10-15", "2024-02-03", "2023-01-01"];
dates.sort((a, b) => new Date(a) - new Date(b));
console.log(dates);
// Output: ["2022-10-15", "2023-01-01", "2023-07-09", "2024-02-03"]
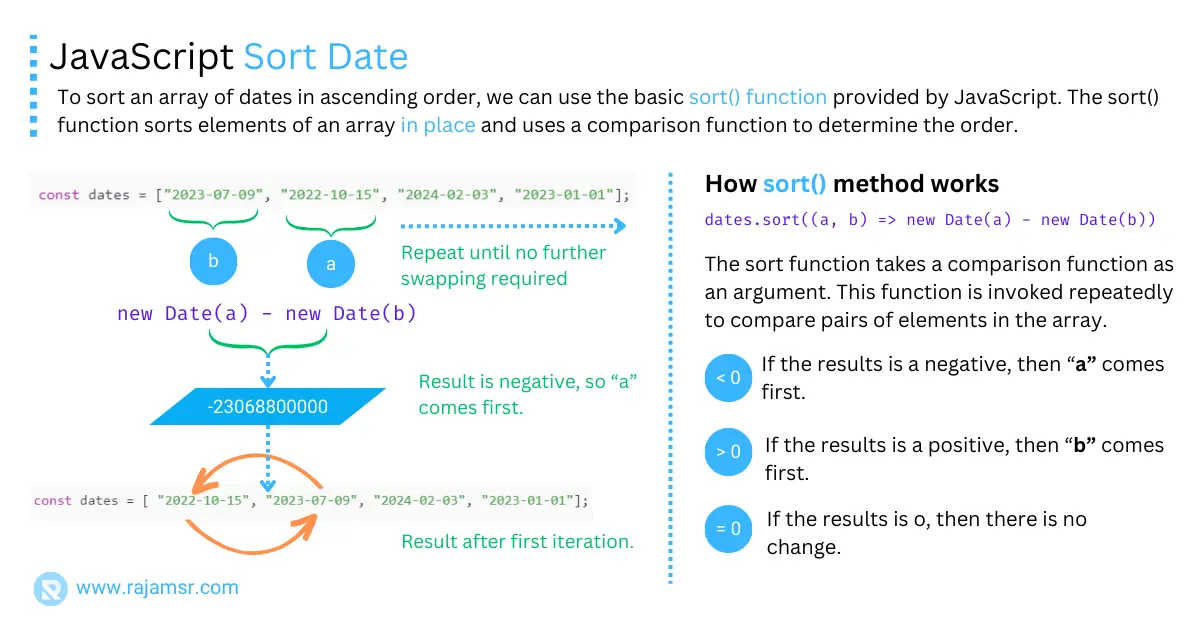
JavaScript sort by date descending
To sort the JavaScript Dates in descending order, we can use the reverse() method after sorting the array in ascending order.
const dates = ["2023-07-09", "2022-10-15", "2024-02-03", "2023-01-01"];
dates.sort((a, b) => new Date(a) - new Date(b)).reverse();
console.log(dates);
// Output: ["2024-02-03", "2023-07-09", "2023-01-01", "2022-10-15"]
"a"
and "b"
in the function that compares them.const dates = ["2023-07-09", "2022-10-15", "2024-02-03", "2023-01-01"];
dates.sort((a, b) => new Date(b) - new Date(a));
console.log(dates);
// Output: ["2024-02-03", "2023-07-09", "2023-01-01", "2022-10-15"]
Sorting by date and time in JavaScript
Sometimes, sorting by date alone is not sufficient, and we need to consider the time component as well. Here’s how you can sort by both date and time in JavaScript:
const datetimeValues = [
"2023-01-01T10:00:00",
"2023-01-01T08:30:00",
"2023-01-02T12:15:00",
"2023-01-01T09:45:00"
];
datetimeValues.sort((a, b) => new Date(a) - new Date(b));
console.log(datetimeValues);
/* Output:
[
"2023-01-01T08:30:00",
"2023-01-01T09:45:00",
"2023-01-01T10:00:00",
"2023-01-02T12:15:00"
]
*/
Sorting by date range with null ends
In some scenarios, date ranges may have null values at either end. Sorting such ranges requires special consideration. Let’s see how we can handle this:
const dateRanges = [
{ start: "2022-05-01", end: "2022-06-01" },
{ start: null, end: "2022-07-01" },
{ start: "2022-03-01", end: "2022-04-01" },
{ start: "2022-01-01", end: null }
];
dateRanges.sort((a, b) => {
const startA = a.start ? new Date(a.start) : new Date();
const startB = b.start ? new Date(b.start) : new Date();
const endA = a.end ? new Date(a.end) : new Date();
const endB = b.end ? new Date(b.end) : new Date();
return startA - startB || endA - endB;
});
console.log(dateRanges);
/* Output:
[
{ start: null, end: "2022-07-01" },
{ start: "2022-01-01", end: null },
{ start: "2022-03-01", end: "2022-04-01" },
{ start: "2022-05-01", end: "2022-06-01" }
]
*/
Sorting an array of objects by date in JavaScript
Sorting an array of objects based on a specific date property requires a custom comparison function. Here’s an example of how to achieve this:
const books = [
{ name: "The Great Gatsby", datePublished: "2021-10-02" },
{ name: "To Kill a Mockingbird", datePublished: "2023-10-05" },
{ name: "Pride and Prejudice", datePublished: "2002-05-12" }
];
books.sort((a, b) => new Date(a.datePublished) - new Date(b.datePublished));
console.log(books);
/* Output:
[
{ name: "Pride and Prejudice", datePublished: "2002-05-12" },
{ name: "The Great Gatsby", datePublished: "2021-10-02" },
{ name: "To Kill a Mockingbird", datePublished: "2023-10-05" }
]
*/
You can use the JavaScript reverse() method on the book’s object, it will sort the book’s array in published date descending order.
Sorting by date using the Moment.js library
Moment.js is a popular library for working with dates and times in JavaScript. Let’s see how we can sort dates using Moment.js:
// Assuming Moment.js is installed and imported
const dates = [
moment("2022-02-01", "YYYY-MM-DD"),
moment("2022-01-01", "YYYY-MM-DD"),
moment("2022-03-01", "YYYY-MM-DD")
];
dates.sort((a, b) => a - b);
console.log(dates);
/* Output:
[
moment("2022-01-01", "YYYY-MM-DD"),
moment("2022-02-01", "YYYY-MM-DD"),
moment("2022-03-01", "YYYY-MM-DD")
]
*/
Sorting by date string
When working with date strings, it’s important to convert them into a sortable format. Here’s an example of sorting by date string in JavaScript:
const dateStrings = ["2022-05-01", "2022-03-01", "2022-07-01"];
dateStrings.sort((a, b) => new Date(a) - new Date(b));
console.log(dateStrings);
// Output: ["2022-03-01", "2022-05-01", "2022-07-01"]
Sorting by date and filter
Combining sorting and filtering functionalities allows us to manipulate data based on specific criteria. Let’s explore how to sort and filter dates in JavaScript:
const dates = ["2022-05-01", "2022-03-01", "2022-07-01", "2022-04-01"];
const filteredAndSortedDates = dates
.filter(date => new Date(date) > new Date("2022-04-01"))
.sort((a, b) => new Date(a) - new Date(b));
console.log(filteredAndSortedDates);
// Output: ["2022-05-01", "2022-07-01"]
Sorting by date and then other value
Sometimes, we may need to sort the data based on multiple criteria. Here’s an example of sorting by published date
and then by another value price
in JavaScript:
const books = [
{ name: "The Great Gatsby", datePublished: "2021-10-02", price: 10 },
{ name: "To Kill a Mockingbird", datePublished: "2023-10-05", price: 15 },
{ name: "Pride and Prejudice", datePublished: "2002-05-12", price: 20 }
];
books.sort((a, b) => {
const dateComparison = new Date(a.datePublished) - new Date(b.datePublished);
return (dateComparison !== 0) ? dateComparison : (a.price - b.price)
});
console.log(books);
/* Output:
[
{ name: "Pride and Prejudice", datePublished: "2002-05-12", price: 20 }
{ name: "The Great Gatsby", datePublished: "2021-10-02", price: 10 },
{ name: "To Kill a Mockingbird", datePublished: "2023-10-05", price: 15 },
]
*/
Sorting by date week
Sorting dates within a specific week requires determining the week numbers
. Let’s see how to sort dates by week in JavaScript:
// Assuming Moment.js is installed and imported
const dates = ["2023-05-01", "2023-03-01", "2023-07-01", "2023-04-01"];
dates.sort((a, b) => {
const weekA = moment(a, "YYYY-MM-DD").week();
const weekB = moment(b, "YYYY-MM-DD").week();
return weekA - weekB || new Date(a) - new Date(b);
});
console.log(dates);
/* Output:
[
"2023-03-01",
"2023-04-01",
"2023-05-01",
"2023-07-01"
]
*/
Sorting by ISO date
ISO 8601 date format is widely used for its universal compatibility. Here’s an example of sorting by ISO dates in JavaScript:
const isoDates = ["2023-05-01T10:00:00Z", "2023-03-01T08:30:00Z", "2023-07-01T12:15:00Z"];
isoDates.sort((a, b) => new Date(a) - new Date(b));
console.log(isoDates);
/* Output:
[
"2023-03-01T08:30:00Z",
"2023-05-01T10:00:00Z",
"2023-07-01T12:15:00Z"
]
*/
Troubleshooting: Sorting by date not working
- Ensure the date format is consistent across the dataset.
- Check for any invalid or malformed date strings.
- Verify that the date objects are being created correctly.
- Debug the custom comparison function to identify any logical errors.
- Consider using a library like
Moment.js
for complex date manipulation.
Conclusion
Sorting data by date is an essential skill for JavaScript developers, and mastering the various techniques covered in this guide will greatly enhance your ability to manipulate and organize date-related information.
By understanding the concepts behind sorting by date, utilizing libraries like Moment.js, and troubleshooting common issues, you can confidently tackle any date sorting challenge in your JavaScript projects!