Do you want to learn how to get the JavaScript string length of any text in your code? If you are working with strings in JavaScript, you might need to know how many characters are in a string, or how long it is. This can be useful for many tasks, such as validating user input, truncating long strings, looping through the characters of a string, and so on.
However, getting the JavaScript string length is not always straightforward, especially if your string contains emojis
, mathematical symbols
, or other Unicode
characters. These characters can cause unexpected results when using the length property of strings, and you might need to use different methods to count them correctly.
In this blog post, I will show you:
- How to access the length property of a string
- Four different approaches to getting the string length
- JavaScript string length in bytes
- Handle undefined string length
- String length comparison
- Validation of user input
- Performance benchmark
- How to solve the Unicode text length problem
By the end of this post, you will be able to work with strings in JavaScript more confidently and efficiently.
How to access the JavaScript string length property?
length
of a string in JavaScript is to use the length
property (not method) of the string object. The length
property returns the number of code units in the string, which is usually the same as the number of characters.For example, let’s say you have a JavaScript string variable called userName
, and you want to get its length. You can do this by using the dot notation
, like this:let userName = 'John';
console.log(userName.length); // Output: 4
You can also use the bracket notation
, like this:
let userName = 'John';
console.log(userName['length']); // Output: 4
Both dot notation and bracket notation will give us the same result, but dot notation is more common and preferred.
You can also use the .length
property directly on a string literal, without assigning it to a variable, like this:
let userName = 'John'.length; // Output: 4
length
property is useful for many tasks, such as validating user input, truncating strings, looping through the characters of a string, and so on.There are different ways to find the length
of a string. In this post, you will see four different approaches
: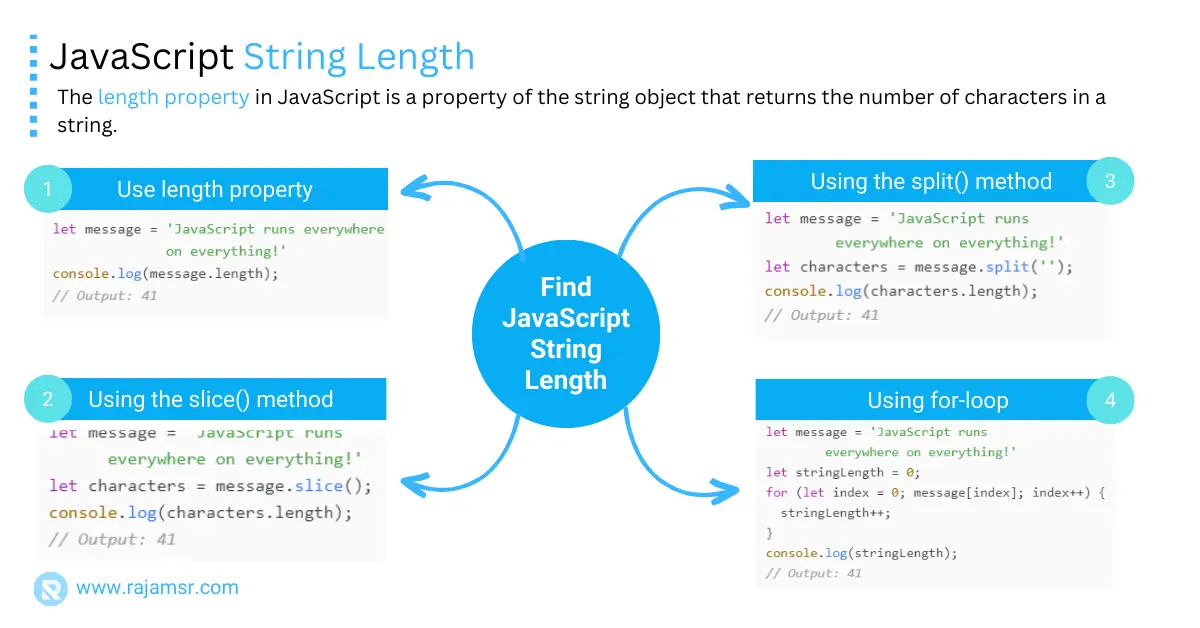
1. How to use the length property in JavaScript
Using the length
property in JavaScript is straightforward. To access the length
property, add .length
to the end of a string. The result will be an integer that represents the number of characters in the string.
Here’s an example of how to get the length
of a string in JavaScript:
let message = 'JavaScript runs everywhere on everything!'
console.log(message.length);
// Output: 41
length
property with different types of strings:let string = "Hello World";
let stringLength = string.length;
console.log(stringLength);
// Output: 11
let emptyString = "";
let emptyStringLength = emptyString.length;
console.log(emptyStringLength);
// Output: 0
let stringWithSpaces = " ";
let stringWithSpacesLength = stringWithSpaces.length;
console.log(stringWithSpacesLength);
// Output: 3
Though we have other options to get the string size, using the string.length
property is one of the best approaches to calculating the length of a string. Based on text lowercase or uppercase length will not change.
When getting the length of a string, if you want to ignore the white from the start and end, you have to use JavaScript string trim methods.
Using the following three methods, you can find string length without using the length
property.
2. Using the split() method to find the length of a string in JavaScript
The split()
method in JavaScript can also be used to find the length of a string. You can split a string into an array of substrings with the split()
method and get the length of the array.
Here’s an example of how to get the length of a string:
let message = 'JavaScript runs everywhere on everything!'
let characters = message.split('');
console.log(characters.length);
// Output: 41
3. Using slice() method to find string length in JavaScript
The JavaScript slice() method can also be used to find the length of a string. You can slice a string into an array of characters with the slice()
method and get the length of the array.
Here’s an example of how to find the length of a string in JavaScript:
let message = 'JavaScript runs everywhere on everything!'
let characters = message.slice();
console.log(characters.length);
// Output: 41
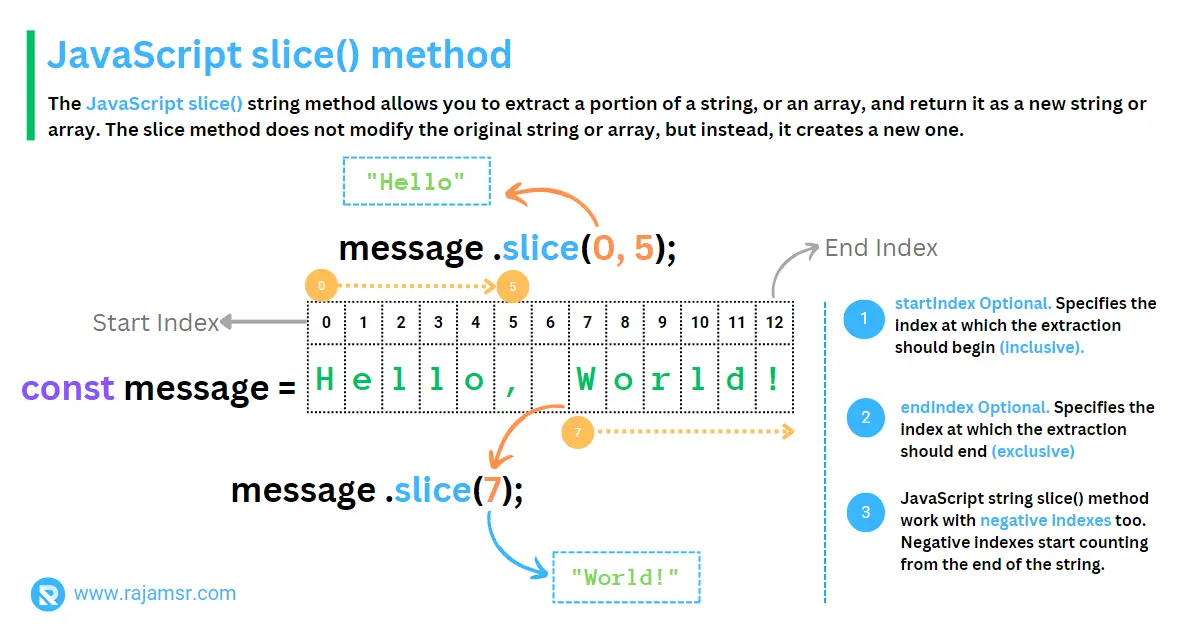
4. Find length of string in JavaScript using for...loop
A for...loop
can also be used to find the length of a string in JavaScript. The for...loop
iterates through each character in the string, and the length of the string is equal to the number of iterations.
Here’s an example of getting the length of the string using for...loop
:
let message = 'JavaScript runs everywhere on everything!'
let stringLength = 0;
for (let index = 0; message[index]; index++) {
stringLength++;
}
console.log(stringLength);
// Output: 41
JavaScript string length in bytes
To work with JavaScript strings in bytes, you need to know how many bytes each string uses. This is important for internationalization and character encoding purposes.
function getByteLength(inputString) {
// Assuming UTF-8 encoding
return new TextEncoder().encode(inputString).length;
}
const byteLength = getByteLength("String in Bytes");
console.log(byteLength);
// Output: 15
This code example above shows how to calculate the byte length of a string, which tells us how much memory the string occupies. This is useful for different encoding situations.
String length undefined
In JavaScript, string length may be undefined
in scenarios where the string itself is null
or undefined
.
const undefinedString = null; // or undefined
const length = undefinedString
? undefinedString.length
console.log(length);
// Output: 0
Calling the length
property on the null
or undefined
string will throw a “Cannot read properties of null” exception. This code example ensures a default length of 0
is assigned to prevent potential issues when working with undefined string lengths.
How to deal with strings that contain emojis, mathematical symbols, or other Unicode characters?
In JavaScript, the length
property of a string does not count the number of Unicode characters but instead counts the number of 16-bit code units
(UTF-16 code units
). This can sometimes lead to unexpected results when working with Unicode characters, especially those outside the Basic Multilingual Plane (BMP)
, which includes characters from U+0000
to U+FFFF
.
Characters outside the BMP</code are represented using surrogate pairs, which consist of two 16-bit code units.
Here’s an example to illustrate how the length
property behaves with Unicode characters:
let message = '' console.log(message.length); // Output: 2
In the example above, the string message contains two code units. So, the length
property returns 2. This can cause problems if you want to manipulate the string, such as slicing, reversing a string, or comparing it. For example, if you try to slice the emoji
string, you might end up with an invalid character, like this:
let emoji = '😊'; let slicedEmoji = emoji.slice(0, 1); console.log(slicedEmoji) // Output: '�'
Array.from()
method, the spread operator
, or the for...of
loop or regular expression. These methods will treat each character as a single element, regardless of how many code units
it is composed of.1. Using Array.from() method
For example, you can use the Array.from()
method to convert the string into an array of characters, and then use the length
property of the array, like this:
let emoji = '😊'; let emojiArray = Array.from(emoji); // emojiArray is ['😊'] console.log(emojiArray.length); // Output: 1
2. Using spread operator method(...)
You can also use the spread operator (...)
to create an array of characters from the string, and then use the length
property of the array, like this:
let emoji = '😊'; let emojiArray = [...emoji]; // emojiArray is ['😊'] console.log(emojiArray.length); // Output: 1
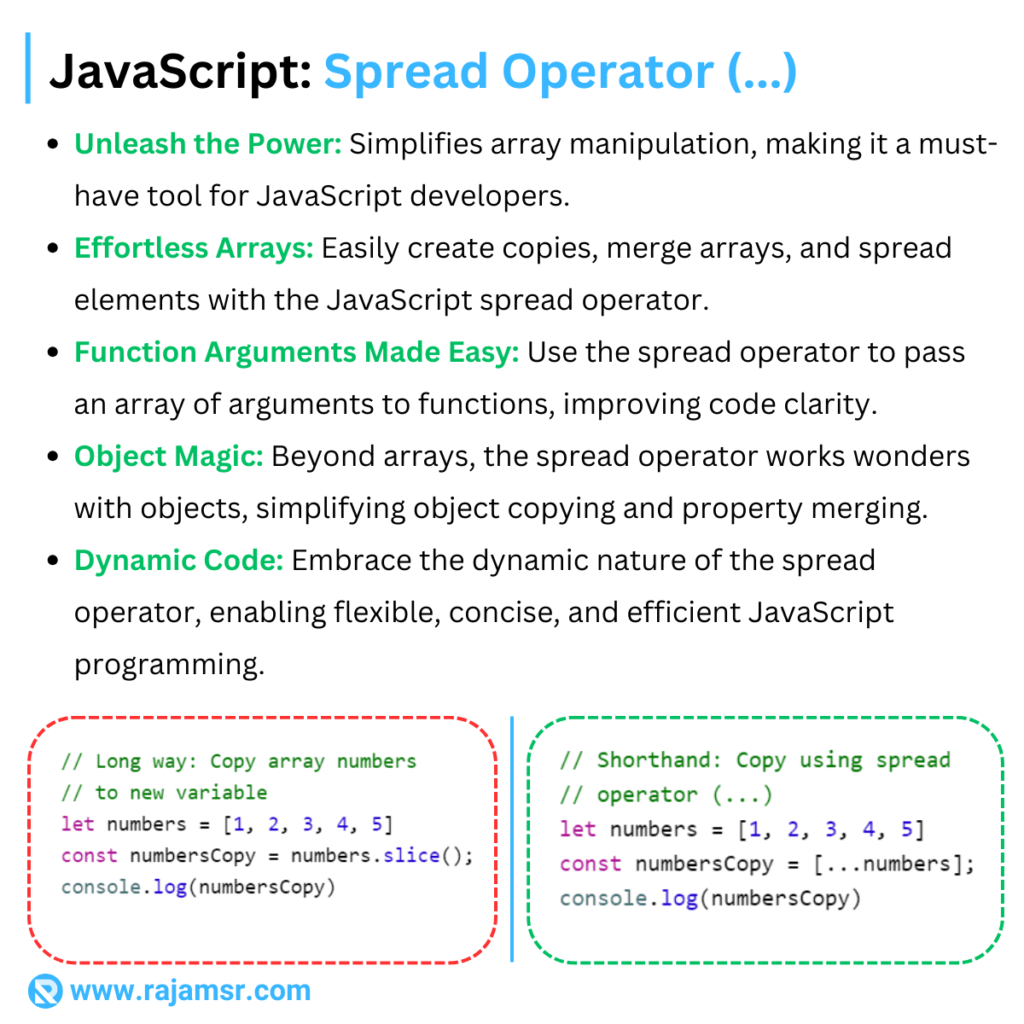
3. Using for...of loop
Alternatively, you can use the for...of
loop to iterate over the characters of the string, and use a counter variable to keep track of the number of characters, like this:
let emoji = '😊'; let emojiLength = 0; for (let char of emoji) { emojiLength++; } console.log(emojiLength) // Output: 1
4. Using regular expression to match Unicode characters
regular expressions
to find out the length.function countUnicodeCharacters(str) { const regex = /[\uD800-\uDBFF][\uDC00-\uDFFF]|[\S\s]/g; const matches = str.match(regex); return matches ? matches.length : 0; } const emoji = ""; console.log(countUnicodeCharacters(emoji)); // Outputs: 1
The countUnicodeCharacters()
function uses a regex pattern
to match Unicode
surrogate pairs and individual characters in a given string. This code example shows that counting the number of Unicode
characters in the string “” produces an output of
1
, reflecting the correct count considering the presence of a single emoji.
These methods will work for any string that contains emojis
, mathematical symbols
like π
, √a
or other Unicode
characters, and they will give us the correct number of characters in the string.
String length comparison
Using JavaScript string length comparison, efficient sorting and ordering can be achieved by leveraging the length property. By using the JavaScript sort() method with a custom comparison function allows for precise organization based on string lengths.
const stringsToCompare = ["Yellow", "Light Gray", "Blue"];
stringsToCompare.sort((a, b) => a.length - b.length);
console.log("Sorted strings by length:", stringsToCompare);
// Output: Sorted strings by length: ["Blue", "Yellow", "Light Gray"]
This code demonstrates how to compare and sort an array of strings based on their lengths.
Validation of user input
You can often use the length
property in web development to validate user input. For example, a name
field may require a certain number of characters (min 5, maximum 20
), and you can use the length
property to ensure that the user has entered the correct amount of data.
Here’s an example of validating user input for min and maximum length:
function validateStringLength(inputString, minLength, maxLength) {
const length = inputString.length;
if (length < minLength || length > maxLength) {
return false;
}
return true;
}
const isValid = validateStringLength("John Doe", 5, 20);
console.log(isValid);
// Output: true
This code validates if a string’s length falls within a specified range.
JavaScript length of string performance benchmark
The results show that the built-in property length
performs the best among the four methods, with 151,587
operations per second. The split()
and splice()
methods are slightly slower, with 147,823
and 139,925
operations per second, respectively.
The for loop is the worst performer, with only 132,852
operations per second.
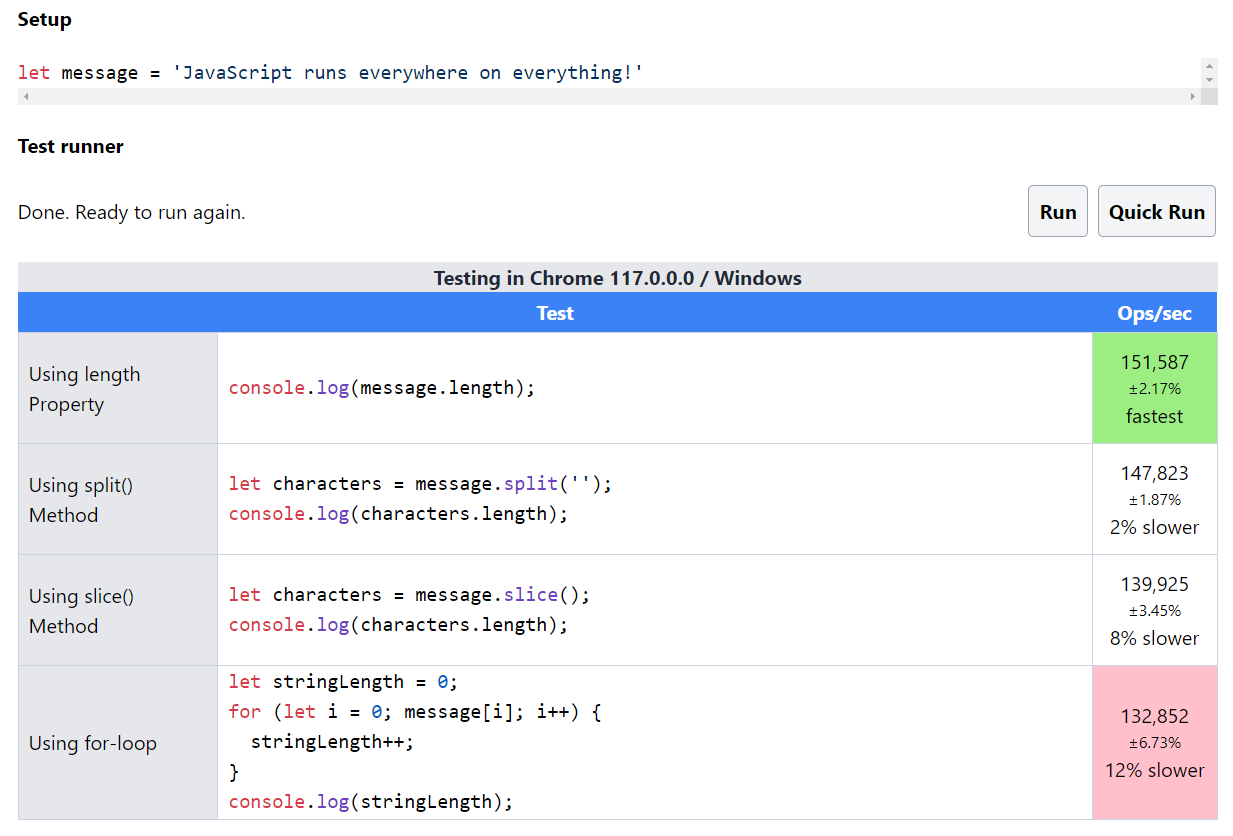
Conclusion
In this post, you have learned how to find the JavaScript string length
using different methods. You have learned how to deal with some common issues and situations that may affect the string length calculation.
You have also learned how to calculate the string length in bytes, how to handle undefined strings, and how to work with strings that have emojis
, symbols
, or other Unicode
characters.
Additionally, you have seen how to use the string length property to validate user input and to sort an array of strings.
I hope this post has been useful and informative for you, and that you have gained some new and valuable knowledge.