Are you looking for a solution to check whether the JavaScript object instance is of a specific class or constructor? A JavaScript instanceof
operator is the solution.
The instanceof
operator checks if an object is an instance of a particular class or constructor function. It returns true if the object is an instance of the specified constructor and false otherwise.
In this blog post, we will explore the following:
- What is an instanceof operator?
- What is the use of it?
- The different uses of the instanceof operator include string, array, date, object, error, and function.
- Why instanceof can be problematic and how to avoid common pitfalls
- Troubleshooting instanceof errors
Let’s dive in.
What is the instanceof operator?
The instanceof
operator returns a boolean
value. Indicating whether the left-hand side object is an instance of the right-hand side constructor.
The syntax for using instanceof
is as follows:
object instanceof constructor
Parameter | Description |
---|---|
object | The object to be tested. |
constructor | The constructor is the constructor function to which the thing is compared. |
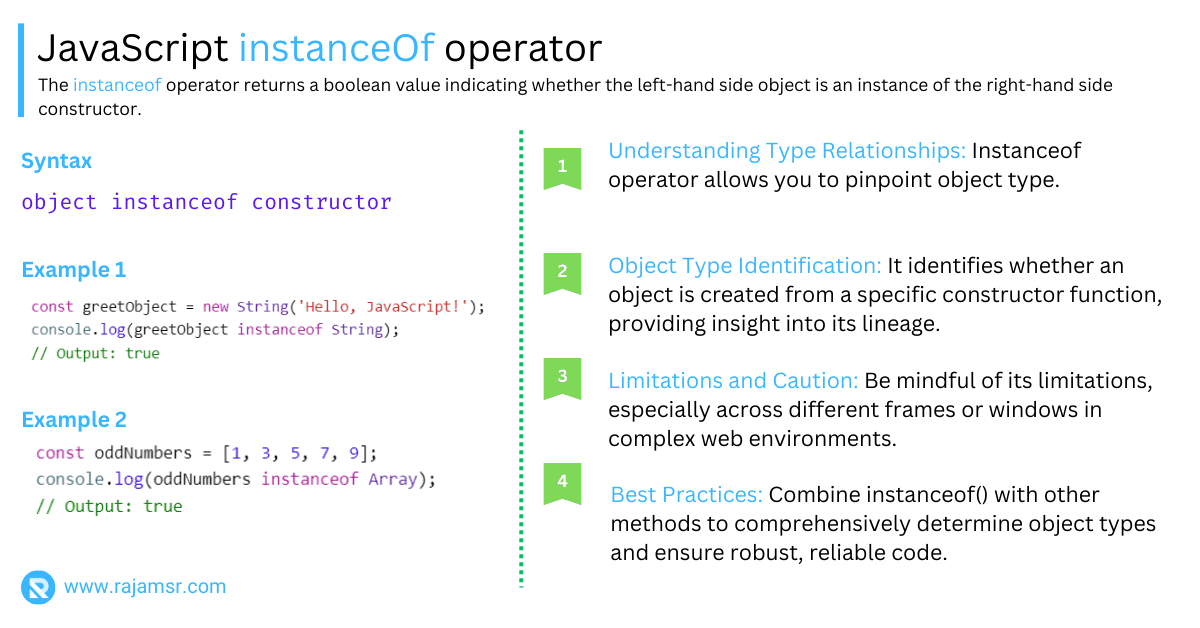
The instanceof
operator returns a boolean
value. Indicating whether the left-hand side object is an instance of the right-hand side constructor.
JavaScript instanceof String
JavaScript String is a primitive data type, but it can also be an object created with the String()
constructor. Let’s take a look at an example of how to use the instanceof
operator with a string:
const greetString = 'Hello, JavaScript!';
const greetObject = new String('Hello, JavaScript!');
console.log(greetString instanceof String);
// Output: false
console.log(greetObject instanceof String);
// Output: true
In this example, we create the string 'Hello, JavaScript!'
. Create a string object named greetObject
using the String()
constructor. When we use the instanceof
operator to determine whether both of them are instances of the String()
constructor.
The output shows that greet is not an instance of the String()
constructor, while greetObject
is.
JavaScript instanceof Array
An array is one of the non-primitive data types in JavaScript. Using the instanceof operator, as shown in the following example, you can make sure whether an object is an instance of the Array()
constructor.
const oddNumbers = [1, 3, 5, 7, 9];
console.log(oddNumbers instanceof Array);
// Output: true
// Alternative Approach
console.log(Array.isArray(oddNumbers));
In this example, we create an array with the values 1, 3, 5, 7, and 7
and assign it to the variable oddNumbers
. And then we used the instanceof
operator to check whether it was an instance of the Array()
constructor. The output shows that the array is indeed an instance of the Array()
constructor.
Alternatively, you can use the Array.isArray()
method to check if the instance is an array type.
JavaScript instanceof Date
A JavaScript Date is an object that is used to represent a date and time. Using this instanceof
, you can make sure the object is an instance of the Date()
constructor.
Here is an example:
const today = new Date();
console.log(today instanceof Date);
// Output: true
instanceof
operator to check whether it was an instance of the Date()
constructor. The output shows that the date object is indeed an instance of the Date()
constructor.JavaScript instanceof Object
In JavaScript, all objects are instances of the Object()
constructor. Like other types, you can use the instanceof
operator. To check whether an object is an instance of the Object()
constructor.
Here is an example:
const person = {};
console.log(person instanceof Object);
// Output: true
In this example, we create an empty person object. Used the instanceof
operator to check whether it is an instance of the Object()
constructor. The output shows that the object is indeed an instance of the Object()
constructor.
JavaScript instanceof error
In JavaScript, we can throw an error when an input validation fails. You can use the instanceof
operator to check whether an object is an instance of an error constructor.
An error object could be one of the following types:
- Error()
- SyntaxError()
- TypeError()
Here is an example of using TypeError
:
try {
const number = 'Hello';
if (isNaN(number)) {
throw new TypeError('Not a number');
}
} catch (err) {
console.log(err instanceof TypeError);
// Output: true
}
In this example, we create a variable number with the value of the string 'Hello'
. We then use the isNaN()
function to check whether it is not a number. If the input number is not valid, then throw a TypeError()
. We catch the error and use the instanceof
operator to check whether it is an instance of the TypeError()
constructor.
The output shows that the error is indeed an instance of the TypeError()
constructor.
Is using instanceof bad idea?
Although the instanceof
operator can be useful in many cases, it can also give unexpected results in some scenarios. One reason is that it only works with objects and not with primitive types.
Refer to the following example:
const number = 1;
console.log(number instanceof Number);
// Output: false
In this example, we create a variable "number"
with the value of 1. We then use the instanceof operator to check whether it is an instance of the Number()
constructor, which returns false. This is because a number is a primitive value and not an object. Another reason why instanceof
can be problematic is that it can be affected by prototype inheritance.
Here is an example:
function Book() { }
function Page() { }
Page.prototype = new Book();
const page = new Page();
console.log(page instanceof Book);
// Output: true
In this example, we create two constructor functions, Book()
and Page()
. We then set the Page()
prototype to an instance of the Book()
constructor, effectively creating a prototype chain between the two.
We create an object page using the Page()
constructor and use the instanceof
operator to check whether it is an instance of the Book()
constructor. The output shows that the page is indeed an instance of the Book()
constructor.
However, this example highlights a potential issue with the instanceof
operator. If we were to change the Page()
prototype later in the code, the instanceof
check might return a different result, even though the object itself has not changed. This can make code that relies heavily on instanceof
difficult to maintain and debug.
JavaScript instanceof vs typeof
The JavaScript typeof operator is used to check the type of an object
in JavaScript, while the instanceof
operator checks whether an object is an instance of a particular constructor function. Let’s look at an example to see the difference:
const index = 10;
console.log(typeof index);
// Output: number
console.log(index instanceof Number);
// Output: false
In this example, we created an integer variable index with a value of 10. We then use the typeof
operator to check the type of the variable, which is the number.
When we use the instanceof
operator to check whether the variable is an instance of the Number()
constructor, which returns false, This is because the index is a primitive value and not an object.
JavaScript instanceof vs Prototype
The instanceof
operator checks whether an object is an instance of a particular constructor function. The prototype property of a constructor function is used to add properties and methods to objects created from that constructor function.
Here is an example:
function Person() {}
Person.prototype.run = function() {
console.log('Running...!');
}
const john = new Person();
console.log(john instanceof Person);
// true
console.log(john.run());
// "Running...!"
In this example, we create a constructor function Person()
and add a method run()
to its prototype. We then create an object named john
using the constructor function and use the instanceof
operator to check whether it is an instance of the Person()
constructor. The output shows that john
is indeed an instance of the Person()
constructor. We also call the run()
method on the john
object, which returns "Running...!"
.
JavaScript instanceof not working
The instanceof
operator may not work as expected in a few scenarios. This is especially true when dealing with objects that have been created in different execution contexts, such as in an iframe or a different window.
In such cases, the instanceof
operator may not recognize the object as an instance of the expected constructor function. This can lead to unexpected behavior and bugs in your code.
Troubleshooting TypeError: right-hand side of 'instanceof' is not an object
The "right-hand side of 'instanceof' is not an object"
error occurs when the right-hand operand of the "instanceof"
operator is not a valid object or constructor function.
To understand this error better, let’s consider an example:
const name = "John";
const person = {name: "John"}
console.log(person instanceof name);
// Outut: TypeError: Right-hand side of 'instanceof' is not an object
To fix this error, you should ensure that the right-hand operand of the instanceof
operator is a valid object or constructor function.
If you want to check if a value is an object, you can use the JavaScript typeof operator in combination with the "==='object'"
comparison. Here’s an updated example:
const name = "John";
const person = {name: "John"}
console.log(typeof person === name);
// Outut: false
By using the appropriate comparison and ensuring that the right-hand operand is a valid object or constructor function, you can fix the "right-hand side of 'instanceof' is not an object"
error in JavaScript.
Conclusion
The JavaScript instanceof
operator is useful for checking whether an object is an instance of a particular constructor function. However, it may not work as expected in some scenarios. It will not work as expected when handling primitive values or prototype inheritance.
By using the instanceof
operator carefully and in conjunction with other methods like typeof
, you can write more reliable and maintainable JavaScript code.