If you are a web developer, you may have encountered the JavaScript floor()
function. This function is used to round down a number to the nearest integer. For example, floor(3.14)
returns 3
, and floor(-1.5)
returns -2
. But why do we need this function? And how can we use it effectively in our code?
In this blog post, we will explore:
- What is floor() function?
- How to round down in JavaScript using floor() on division
- How to use floor() inside a function
- Round down a number to 2 decimal places using floor()
- How to use floor() on floating-point and negative numbers
- Round down a number to the nearest 10
Let’s dive deep.
JavaScript round down using floor() function
The floor()
function is a built-in method in the JavaScript Math object that is used to round down a number to the nearest integer. The floor()
function is often used when you want to perform mathematical operations on numbers and need to round down a result to the nearest integer.
Here’s an example of how to round down in JavaScript using the floor()
function:
const number = 3.7;
const result = Math.floor(number);
console.log(result);
// Output: 3
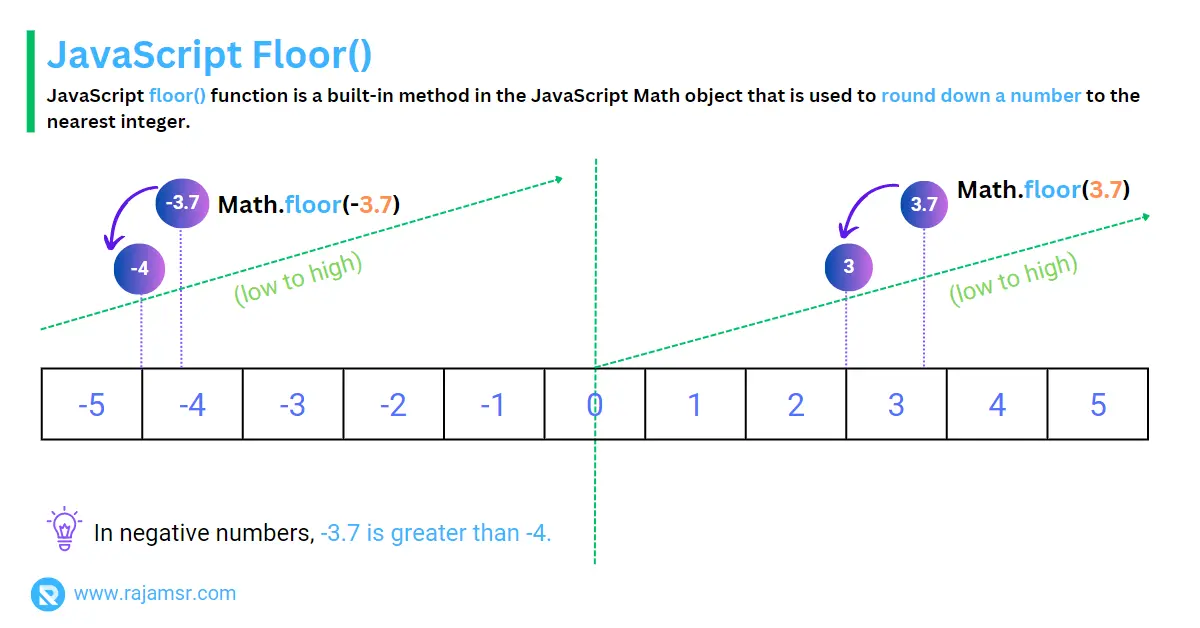
In this example, we have declared a number-type JavaScript variable. I assigned it the value of 3.7
. We then call the floor()
function on the number variable and assign the result to the result variable.
The console.log()
function is used to print the result to the console, which in this case is 3
. Since 3.7
is rounded down to the nearest floor value of 3
.
JavaScript divide round down using floor()
First, let’s talk about how to use the floor()
function on number divisions. The division is dividing two numbers and rounding down the result to the nearest integer. We use the slash operator (/)
in JavaScript to perform division.
Here’s an example of a JavaScript division round-down:
const result = 19 / 3;
console.log(result)
// Output: 6.333333333333333
console.log(Math.floor(result))
// Output: 6
In this example, we divide 19 by 3
and the result is 6.333333333333333
, but the floor()
function rounds the number down to 6.
Using JavaScript floor() in a function
Most of the time, you need to use the floor()
function inside a function, not just simple statements. You can use the Math.floor()
JavaScript function inside a function, as shown in the following example:
const genrateRandomNumber = () => {
const min = 1;
const max = 100;
const randomNumber = Math.floor(Math.random() * (max - min + 1)) + min;
return randomNumber;
}
console.log(genrateRandomNumber())
// Output: 27 // A random integer between 1 and 100
In this example, we created a function called generateRandomNumber()
. Whenever the method generateRandomNumber()
is called, it will return a random number between 1 and 100.
Round down JavaScript number using floor() to 2 decimal places
What if you need to round down a number to two decimal places? You can use the Math.floor()
method in JavaScript combined with the Math.pow()
method to get a round-downed number with two decimal places.
Here is a round-down JavaScript code example to 2 decimal places:
function roundDownToTwoDecimals(number) {
const factor = Math.pow(10, 2);
return Math.floor(number * factor) / factor;
}
console.log(roundDownToTwoDecimals(5.16959));
// Output: 5.16
In this example, we created a function called roundDownToTwoDecimals()
. The roundDownToTwoDecimals()
function takes a number as a parameter. Returns the rounded-down value to two decimal places.
Round down integer and floats using floor()
What if you’re dealing with floating-point numbers instead of integers? Don’t worry; the Math.floor()
method works with floating points too.
Here’s an example:
console.log(Math.floor(3));
// Output: 3
console.log(Math.floor(4.34159));
// Output: 4
In this example, we used the Math.floor()
method to round down integers as well as a floating-point number
. When using the Math.floor()
function on integers, there won’t be any changes in the input number.
Comparison of floor() vs trunc() functions
You may have noticed that there’s also a Math.trunc()
method in JavaScript. So, what’s the difference between Math.floor()
and Math.trunc()
?
Here’s an example to illustrate the difference:
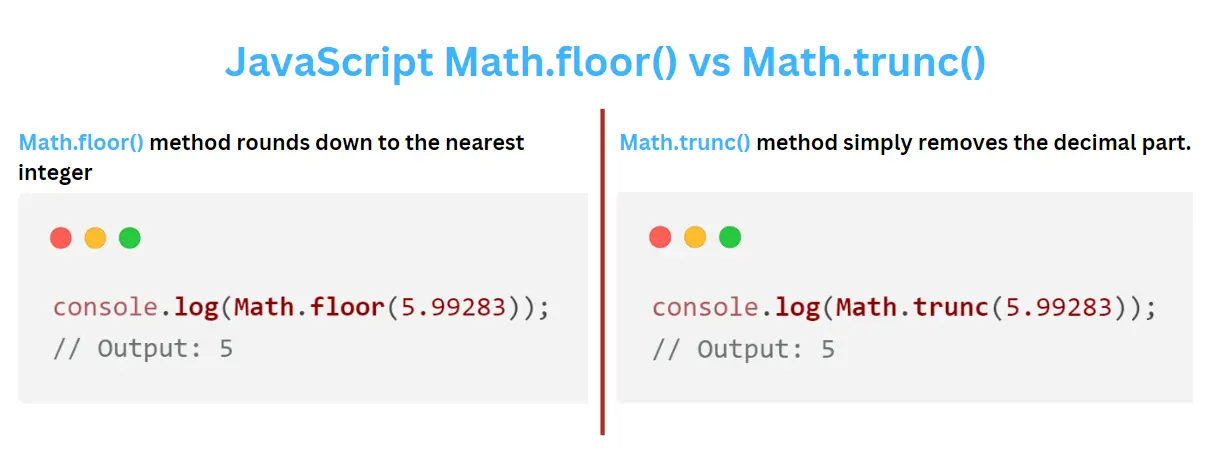
In this example, we used both methods with the same input number 5.99283
. The Math.floor()
method rounds it down to 5, while the Math.trunc()
method simply removes the decimal part and returns 5.
How to use math round down Math.floor() on negative number?
Speaking of negative numbers, what happens when you use the Math.floor()
method on a negative number
? It still rounds down to the nearest integer, but it will be a smaller integer than the original value.
Here’s an example of a round down in JavaScript on a negative number:
const number = -3.7;
const result = Math.floor(number);
console.log(result);
// Output: -4
In this example, we used the Math.floor()
method on a negative floating-point number -3.7
and it rounds it down to -4
.
Round down a number using floor() to nearest 10
Lastly, what if you need to round down a number to the nearest 10
? You can use some math tricks to achieve this. Here’s an example:
function roundDownToNearestTen(number) {
return Math.floor(number / 10) * 10;
}
console.log(roundDownToNearestTen(15));
// Output: 10
In this example, we created a function called roundDownToNearestTen()
that takes a number as a parameter. Returns the rounded-down value to the nearest 10
.
Conclusion
In conclusion, the JavaScript floor()
function is handy when you need to round integers to the next integer or decimal point. The floor function is useful whether you’re working with integers or floats, positive or negative numbers. It will work on all types of numbers.
Math.floor()
On the other hand, it rounds down the number to the nearest integer and Math.trunc()
just truncates the decimal part of the number.
When you use the Math.floor()
function on a negative floating-point number, it rounds down to the nearest integer. The result is a smaller integer than the original value.
Remember the floor function the next time you need to round down a value in JavaScript!