- What is the typeof operator?
- What is the use of it?
- How it works
- How you can use it to determine the data type of a value or expression for different types
- Summary of return types of typeof operator
typeof
operator in JavaScript!Syntax and usage of the typeof operator
The typeof operator is a unary operator that takes any value as an operand and returns a string indicating its data type. The syntax of the typeof operator is:
typeof operand
or
typeof(operand)
The operand can be a literal value, a variable, an expression, or a function. For example:
console.log(typeof 42) // "number"
console.log(typeof "Hello") // "string"
console.log(typeof true) // "boolean"
console.log(typeof x) // "undefined" if x is not declared or assigned
console.log(typeof (2 + 3)) // "number"
console.log(typeof Math.sqrt) // "function"
What is JavaScript typeof operator?
JavaScript typeof
is a built-in operator in JavaScript. You can use the typeof
operator to determine the data type of a given value or expression. It is an essential operator for debugging, testing, and validating code, as it allows you to identify the data type of a value and handle it accordingly.
The typeof is an operator, not a method or function
.
Possible Return Values of the typeof Operator
The typeof operator can return one of the following seven strings as the data type of the operand:
number
: For any numeric value, including integers, decimals, NaN (not a number), and Infinitystring
: For any text value, enclosed in single or double quotesboolean
: For either true or false valuesundefined
: For values that have not been declared or assignedobject
: For any complex data type, such as arrays, objects, null, dates, etc.function
: For any function or methodsymbol
: For any value created with the JavaScript Symbol() constructor

This example uses the typeof
operator to check that the "greet"
variable has a string data type. The output of the typeof
operator returns the data type of a variable greet
.
What is the use of typeof?
You can use the typeof
operator when you need to handle different types of data differently in your code. For example, you are reading user input and want to make sure it’s a valid number. You could use the typeof
operator to check if the input is a number as shown in the following example:
let userInput = '10';
if (typeof userInput === 'number') {
console.log(userInput * 2);
} else {
console.log(`${userInput} is not a number.`);
}
// Output: "10 is not a number."
In this example, if the userInput
variable is a number, the console will log the result of the userInput
value multiplied by 2. If it’s not a number, the console will log "... is not a number."
.
Overall, typeof
is useful when working with different JavaScript data types. Understanding how typeof
of operators work can help you write more efficient and bug-free code.
JavaScript typeof array - How to check data type?
typeof
operator is the difference between primitive and non-primitive data types in JavaScript.Primitive data types are the basic and immutable data types that can hold only one value at a time. They are stored by value, which means that each variable has its copy of the value. Primitive data types
in JavaScript are:- number
- string
- boolean
- undefined
- symbol
- arrays
- objects
- functions
- maps
- sets
- WeakMaps and WeakSets
typeof
operator can help you distinguish between primitive and non-primitive data types.JavaScript typeof array - How to check data type?
We can store multiple values in a single variable by using arrays. The array
is one of the non-primitive data types in JavaScript. JavaScript treats arrays as objects. To determine if the type is an array, you can use the typeof operator.
Arrays are objects, so the typeof
operator returns “object”
for them.
let colors = ["Red", "Green", "Blue"];
console.log(typeof colors);
// Output: "object"
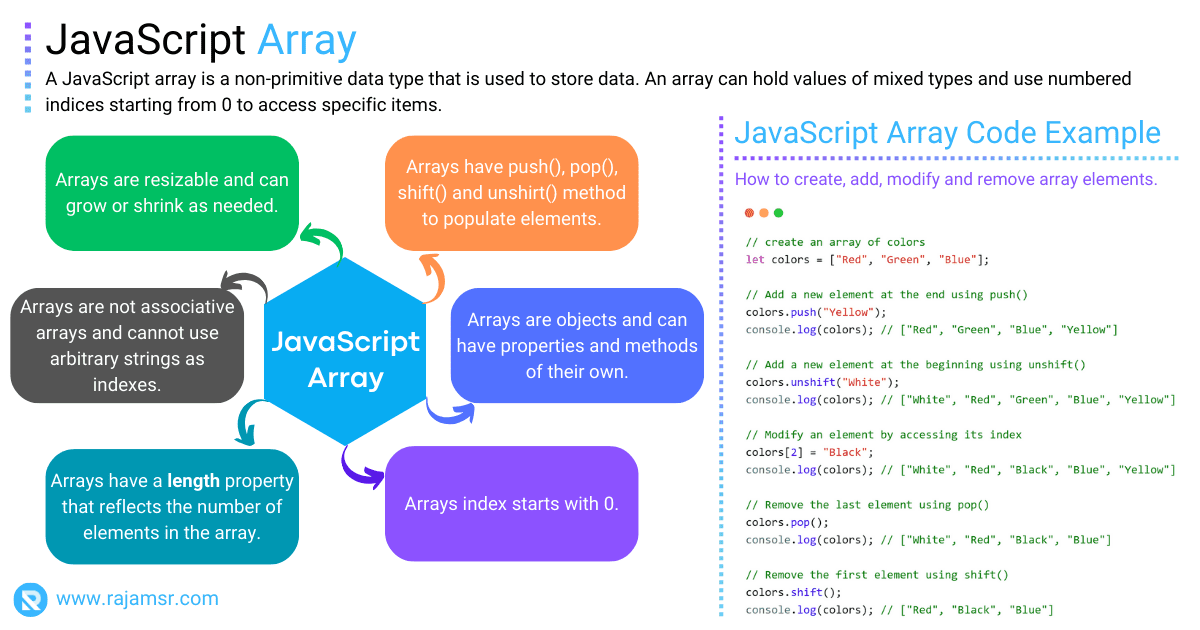
typeof string
A sequence of characters is what JavaScript strings represent. Primitive data types are what strings are in JavaScript. You can use the typeof operator to check the type is a string as shown in the following example.
Strings are primitive data types, so the typeof operator returns “string” for them.
let message = "JavaScript runs everywhere!";
console.log(typeof message);
// Output: "string"
typeof undefined
Undefined
is a special value in JavaScript that represents the absence of a value. If a JavaScript variable is declared but not assigned a value, it is undefined
. To determine if a value is undefined, you can use the typeof operator.
Undefined values are special values that represent the absence of a value, so the typeof
operator returns "undefined"
for them.
let value;
console.log(typeof value);
// outputs "undefined"
JavaScript typeof date is object
JavaScript Date objects are used to work with dates and times. To determine the type of a date object, you can use the typeof
operator.
Date objects are objects that work with dates and times, so the typeof operator returns "object"
for them.
let today = new Date();
console.log(typeof today);
// Output: "object"
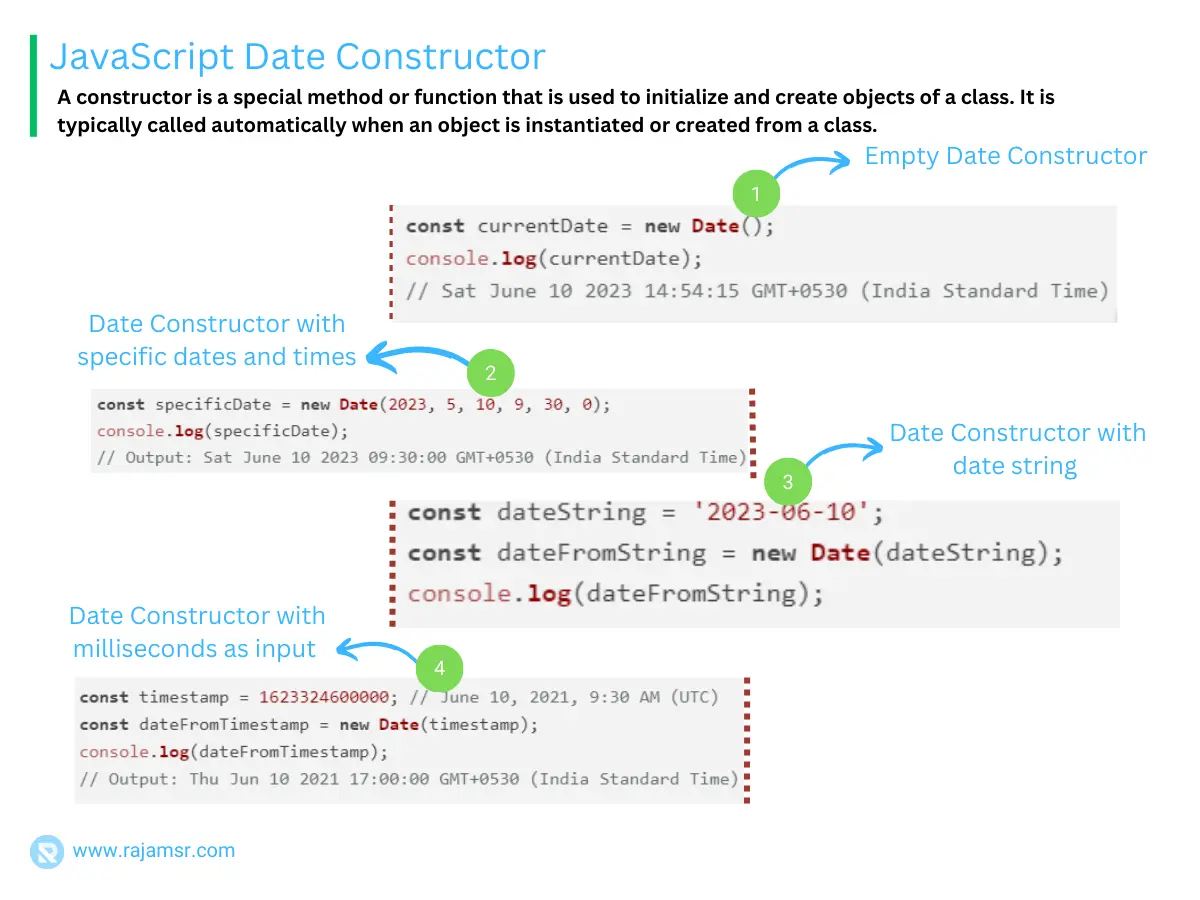
In this example, we create a new Date object and assign it to the today
variable. We then use the typeof
operator to check the data type of the today variable. The output of the typeof operator, in this case, is the string "object"
, which indicates that the value is a Date object.
Using JavaScript instanceof date, you can make sure the object is an instance of the Date() constructor.
JavaScript typeof object is object
Objects are used to store collections of data in JavaScript. Objects are considered non-primitive data types in JavaScript. You can use the typeof operator to check if the type is an Object, as shown in the following example.
Objects are collections of key-value
pairs, so the typeof operator returns "object"
for them. Let’s assume we have a person
object:
let person = {
name: "John Doe",
age: 30
};
The typeof
operator returns as "object"
for person
object.
console.log(typeof person);
// Output: "object"
JavaScript typeof class is function
Classes
are a new feature introduced in ES6. It allows you to create an object with methods
and properties
like in other programming languages. Let’s assume we have a Person
class, as shown in the following example:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
You can use the typeof
operator to check if the type is a class. Classes
are functions that create objects with methods and properties, so the typeof
operator returns "function"
for them. However, for the class instance type, it will return as an object.
let myPerson = new Person("John Doe", 25);
console.log(typeof myPerson);
// Output: "object"
console.log(typeof Person);
// Output: "function"
JavaScript typeof custom object is object
Custom objects are user-defined
objects that can have methods and properties. To check the type of a custom object, you can use the typeof
operator.
function Car(make, model, year) {
this.make = make;
this.model = model;
this.year = year;
}
Custom objects return "object"
for them.
let car = new Car("Honda", "Hundai", 2022);
console.log(typeof car);
// Output: "object"
typeof function
Functions
are used to perform specific tasks in JavaScript. To check the type of a function, you can use the typeof
operator.
Functions
are blocks of code that perform specific tasks, so the typeof
operator returns "function"
for them.
function greet(name) {
console.log(`Hello, ${name}!`);
}
console.log(typeof greet);
// Output: "function"
typeof number
Numbers
are used to represent numerical data in JavaScript. In JavaScript, numbers are considered primitive data types
. To check the type of a number, you can use the typeof
operator.
Numbers are primitive data types
that represent numerical data, so the typeof
operator returns "number"
for them.
let thousand = 1000;
console.log(typeof thousand);
// Output: "number"
typeof boolean
Boolean
is used to represent true
or false
data in JavaScript. In JavaScript, booleans are considered primitive data types
. To check the type of a boolean, you can use the typeof
operator.
Booleans are primitive data types that represent true or false data, so the typeof operator returns "boolean"
for them.
let toggleDisplay = true;
console.log(typeof toggleDisplay);
// Output: "boolean"
typeof symbol
When you apply the typeof
operator to a symbol
, it returns the string "symbol"
. Symbols are a primitive data type
introduced in ECMAScript 2015 (ES6) and represent unique and immutable values.
Here’s an example that demonstrates the usage of typeof
with symbols:
const symbol = Symbol("mySymbol");
console.log(typeof symbol);
// Output: "symbol"
Difference between JavaScript instanceof vs typeof
It’s important to note that the typeof
operator doesn’t differentiate between different types of objects. You can check whether an object is an instance of a particular class using the instanceof
operator.
So, if you want to check if an object is a Date object specifically, you can use the JavaScript instanceof() operator instead.
You can use typeof and instanceof
operators to write a defensive code, as shown in the following example:
let today = new Date();
if (typeof today === 'object' && today instanceof Date) {
console.log(today.toLocaleString())
} else {
console.log(`${today} is not a date type.`);
}
// Output: 4/9/2023, 1:25:23 PM
Common pitfalls and edge cases of using typeof
The typeof
operator is a useful tool, but it is not perfect. There are some pitfalls and edge cases that you should be aware of when using it. Here are some of them: 1. The typeof
operator returns "object"
for null values, even though null
is a primitive data type. This is a bug in the original implementation of JavaScript that has been preserved for compatibility reasons.
To check if a value is null
, you can use the JavaScript strict equality operator (===) instead. For example:
console.log(typeof null) // Output: "object"
console.log(null === null) // Output: true
2. The typeof
operator returns "object"
for arrays, even though arrays are a special kind of object that have a numeric length property and methods for manipulating elements. To check if a value is an array, you can use the Array.isArray()
method instead. For example:
console.log(typeof [1, 2, 3]) // Output: "object"
console.log(Array.isArray([1, 2, 3])) // Output: true
3. The typeof
operator returns "number"
for NaN
values, even though NaN
stands for not a number. This is because NaN
is a special value that represents the result of an invalid arithmetic operation.
To check if a value is NaN
, you can use the Number.isNaN()
method instead. For example:
typeof NaN // Output: "number"
Number.isNaN(NaN) // Output: true
Best Practices and Alternatives to Using typeof
The typeof
operator is a handy way to check the data type of a value, but it is not the only way. Depending on your use case, you may want to use some of the best practices and alternatives to using typeof
.
Here are some of them:
1. Use the constructor
property to get the name of the constructor function that created the value. This can give you more specific information than the typeof
operator, especially for non-primitive data types. For example:
typeof new Date() // Output: "object"
new Date().constructor.name // Output: "Date"
2. Use the instanceof
operator to check if a value is an instance of a certain constructor function or class. This can help you determine the inheritance and prototype chain of the value. For example:
console.log(typeof []) // Output: "object"
console.log([] instanceof Array) // Output: true
console.log([] instanceof Object) // Output: true
Object.prototype.toString
method to get the internal class name of the value. This can help you avoid some of the pitfalls and edge cases of the typeof operator, such as null
and array values. For example:console.log(typeof null) // Output: "object"
console.log(Object.prototype.toString.call(null)) // Output: "[object Null]"
console.log(typeof []) // Output: "object"
console.log(Object.prototype.toString.call([])) // Output: "[object Array]"
Conclusion
In conclusion, we use the JavaScript typeof operator
to find out the data type of a value or expression. It is an essential operator for writing safe and error-free code.
Understanding the typeof
operator and how it works with different data types is crucial for any JavaScript developer.
The typeof
operator cannot differentiate between different kinds of objects. You must use the instanceof
operator to determine whether an object is of a given type.
I hope this blog post has helped you understand what an typeof
operator is and how and where you can use it in real-time application code.