If you are a web developer, you probably have encountered the need to convert a string to a number in JavaScript. This is a common task when you want to perform calculations, comparisons, or validations on user input. But how do you do it correctly? The JavaScript parseFloat() function is the solution.
In this blog post, I will show you:
- What is the parseFloat() function?
- How to use the parseFloat() function
- How does the parseFloat() function work with different input values?
- Pitfalls and edge cases
- Convert parseFloat() to two decimal places
- NaN values and their significance
- Differences between parseFloat() vs parseInt() vs Number() vs Unary Plus Operator
Whether you’re looking to build complex mathematical equations or simply need to convert user input into numbers, this guide will help you unlock the full potential of the parseFloat()
function in your JavaScript code. Let’s see it in detail!
What is parseFloat() JavaScript method?
The JavaScript parseFloat()
is a built-in global function that converts a JavaScript string parameter to a floating-point number
. The parseFloat()
function takes a string as an argument and returns a floating-point number or a number with a decimal point.
Syntax for the parseFloat()
JavaScript function:
parseFloat(string)
string
represents the input string that you want to convert to a floating-point number.How to convert string to float using parseFloat() JavaScript function?
Here’s an example of how to parse a string to float using the parseFloat()
function in JavaScript:
let numberString = '12.34';
console.log(parseFloat(numberString));
// Output: 12.34
In this example, the string "12.34"
is converted to a floating-point number, 12.34
, by the parseFloat()
function.
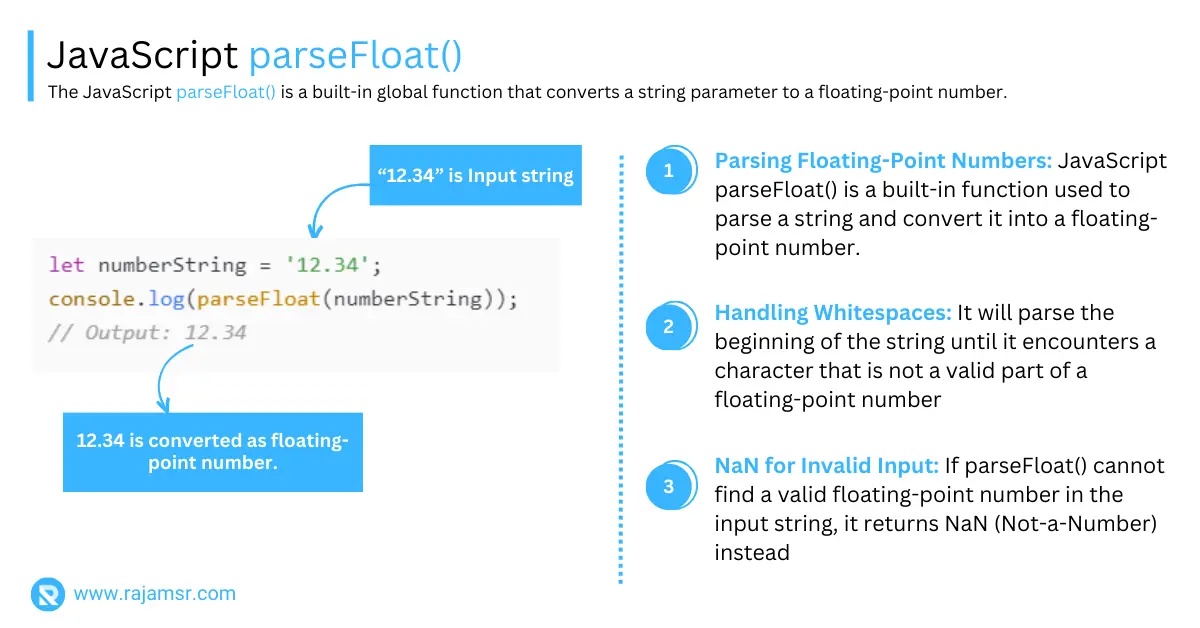
JavaScript string to floating-point numbers conversion using parseFloat()
There are three different ways in JavaScript to check if the string is a number. When a string is passed to parseFloat()
, it will search the string for the first valid number. If a valid number is found, then it converts it to a floating-point integer.
The parseFloat()
function in JavaScript returns NaN
if the string does not contain a valid number. (Not a number
).
JavaScript string to float conversion
Here is an example of string conversions with parseFloat()
in JavaScript:
console.log(parseFloat('12.34'));
// Output: 12.34
Convert string to float with non-numeric characters in the middle
ParseFloat()
only converts the part of the string that appears before the first invalid character. The code converts the numbers before the character "abc"
to floating-point numbers in this example and ignores the rest of the number 726
.
console.log(parseFloat("12.34abc726"));
// Output: 12.34
Convert a string starts with non-numeric characters returns NaN
The parseFloat()
JavaScript function returns NaN
if the input string starts with a non-numeric character like alphabets.
In the following example, the string starts with "abc"
, so the parseFloat()
function returns NaN
.
console.log(parseFloat("abc12.34"));
// Output NaN
Convert string with leading or trailing space to floating-point number
The parseFloat()
function trims the leading and trailing whitespace from the input string and converts the value to a floating-point number.
In the following example, we have empty spaces before and after the number in the string. The parseFloat()
method removed the spaces before converting the value to a floating-point number
.
console.log(parseFloat(" 12.3456 "));
// Output: 12.3456
Convert empty string to float
The parseFloat()
function returns NaN
if the input is an empty JavaScript string. In the following example, the input string is an empty character without a number. So, the parseFloat()
method returns NaN
.
console.log(parseFloat(' '));
// Output: nan
The JavaScript parseFloat() negative number
To convert a string with a negative value to a floating-point number
, use parseFloat()
. The converted result will retain the negative sign.
However, for numbers with a + sign
, the parseFloat()
function will ignore the sign in the converted number.
console.log(parseFloat('-1234.56'));
// Output: -12.34
console.log(parseFloat('+1234.56'));
// Output: 1234.56
Pitfalls and edge cases of JavaScript parseFloat() method
parseFloat()
is a handy function, it is not perfect. There are some situations where it can produce unexpected or incorrect results. Here are some of the most common ones:1. NaN and parseFloat() function
NaN
stands for Not a Number. It is a special value that indicates that the input string is not a valid number. For example, if you try to parse a string that contains letters or symbols, you will get NaN
as a result:
console.log(parseFloat("JavaScript")); // Output: NaN
console.log(parseFloat("$3.14")) // Output: NaN
NaN
is a tricky value. Because, it is not equal to anything, not even itself. This means that you cannot use the normal comparison operators (==, !=, <, >, etc.)
to check if a value is NaN
or not. Instead, you need to use a special function called isNaN()
, which returns true if the argument is NaN
and false otherwise. For example:let result1 = parseFloat("JavaScript"); // Output: NaN
let result2 = parseFloat("$314") // Output: NaN
console.log(isNaN(result1)); // Output: true
console.log(isNaN(result2)); // Output: true
isNaN()
to validate the input string before you use parseFloat()
. Otherwise, you can handle the NaN
value after the use of parseFloat()
function.2. Infinity and parseFloat() function
JavaScript Infinity is another special value. It indicates that the input string is too large or too small to be represented as a finite number. For example, if you try to parse a string that contains a very large or very small number, you will get Infinity
or -Infinity
as a result:
console.log(parseFloat("1e1000")); // Output: Infinity
console.log(parseFloat("-1e1000")); // Output: -Infinity
Infinity
and -Infinity
are valid numbers. They can be used for calculations. However, they can also cause problems, such as overflow or underflow errors, or inaccurate results. For example, if you try to add or subtract anything from Infinity
, you will still get Infinity as a result.let largeNumber = parseFloat("1e1000"); // Infinity
let piValue = parseFloat("3.14"); // 3.14
// Any number with Infinity, returns Infinity not just addition
console.log(largeNumber + piValue); // Output: Infinity
parseFloat()
. One way to do this is to use a function called Number.isFinite()
. The isFinite()
method returns true if the argument is a finite number, and false otherwise. For example:let largeNumber = parseFloat("1e1000"); // Infinity
let piValue = parseFloat("3.14"); // 3.14
Number.isFinite(largeNumber)
? console.log(largeNumber + piValue)
: console.log('largeNumber is Infinite.')
// Output: largeNumber is Infinite.
3. Rounding errors with parseFloat() function
0.1
cannot be represented exactly in binary, and it is rounded to 0.1000000000000000055511151231257827021181583404541015625
. This can cause errors when we perform calculations or comparisons with these numbers. For example:let value1 = parseFloat("0.1");
// value1 is 0.1000000000000000055511151231257827021181583404541015625
let value2 = parseFloat("0.2");
// value2 is 0.200000000000000011102230246251565404236316680908203125
let sum = value1 + value2;
// sum is 0.30000000000000004
console.log(sum === 0.3);
// Output: false
To avoid these errors, you must be careful when using floating-point numbers. One way to address this issue is using the JavaScript toFixed() function.
let value1 = parseFloat("0.1");
let value2 = parseFloat("0.2");
let sum = value1 + value2;
// sum is 0.3000000000000000444089209850062616169452667236328125
let sumString = sum.toFixed(2); // sumString is "0.30" (a string)
console.log(sumString === "0.30"); // true
JavaScript parseFloat() to 2 decimal places
What if you want to limit the result of the parseFloat()
method round to two decimal places? This is a typical requirement when converting a string to a number.
Here’s an example of how to use the toFixed()
method with parameter 2 to convert a string to a floating-point number
with two decimal places:
let string = "12.3456";
let num = parseFloat(string).toFixed(2);
console.log(num);
// Output: 12.35
Using the toFixed()
method you can mention how many digits are required after the decimal place. The toFixed()
method fractionDigits
parameter accepts values from 0 to 100
.
In the above code example, toFixed(2)
is used, so as a result, it returns two digits after the decimal place.
Alternatives to parseFloat() JavaScript function
parseFloat()
is not the only function that can convert a string to a number. There are other functions that can do the same thing, but with some differences. Here are some of the most common ones:1. JavaScript parseInt()
radix (or base)
as arguments. Returns an integer, or a number without a decimal point. The radix specifies the number system that the string uses, such as 2 for binary, 10 for decimal, or 16 for hexadecimal. For example:console.log(parseInt("3.14")); // Output: 3
console.log(parseInt("3.14", 10)); // Output: 3
console.log(parseInt("3.14", 16)); // Output: 3
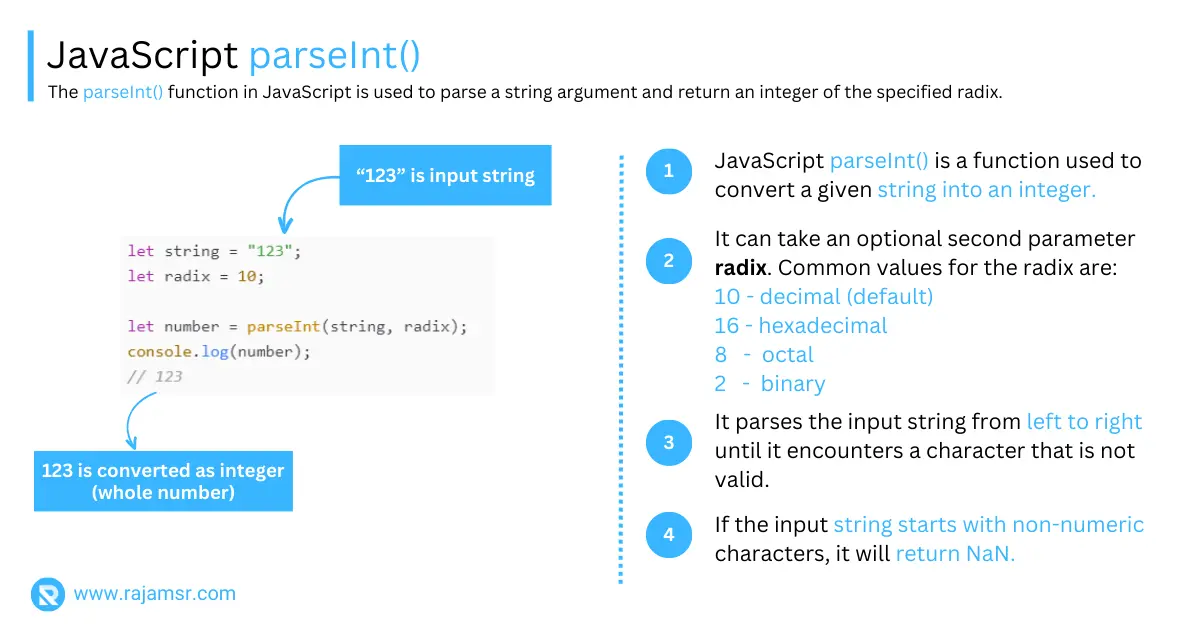
2. JavaScript Number()
Number()
function takes a string as an argument. Returns a number that represents the value of the string. The number can be either an integer or a floating-point number, depending on the format of the string. For example:
console.log(Number("3.14")); // Output: 3.14
console.log(Number("3")); // Output: 3
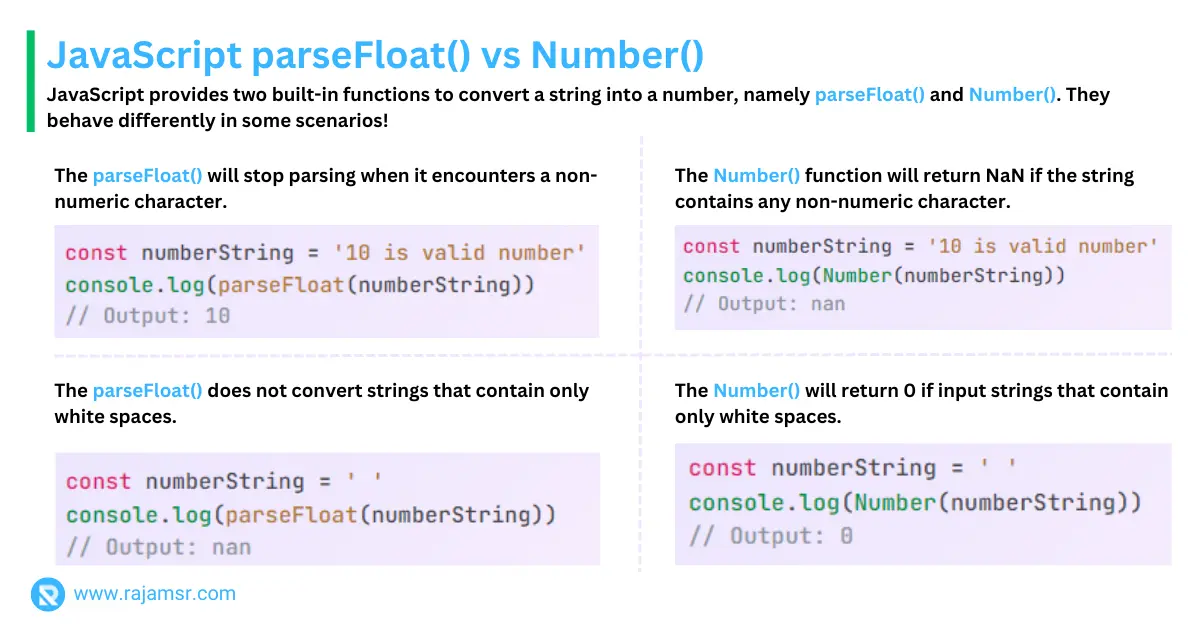
3. Unary plus (+)
Unary plus (+)
is an operator that can be used to convert a string to a number. It works by placing a plus sign (+) before the string. For example:console.log(+"3.14"); // Output: 3.14
console.log(+"3"); // Output: 3
Differences between parseFloat() vs parseInt() vs Number() vs Unary Plus operator
Here is a table that summarizes the similarities and differences between them:
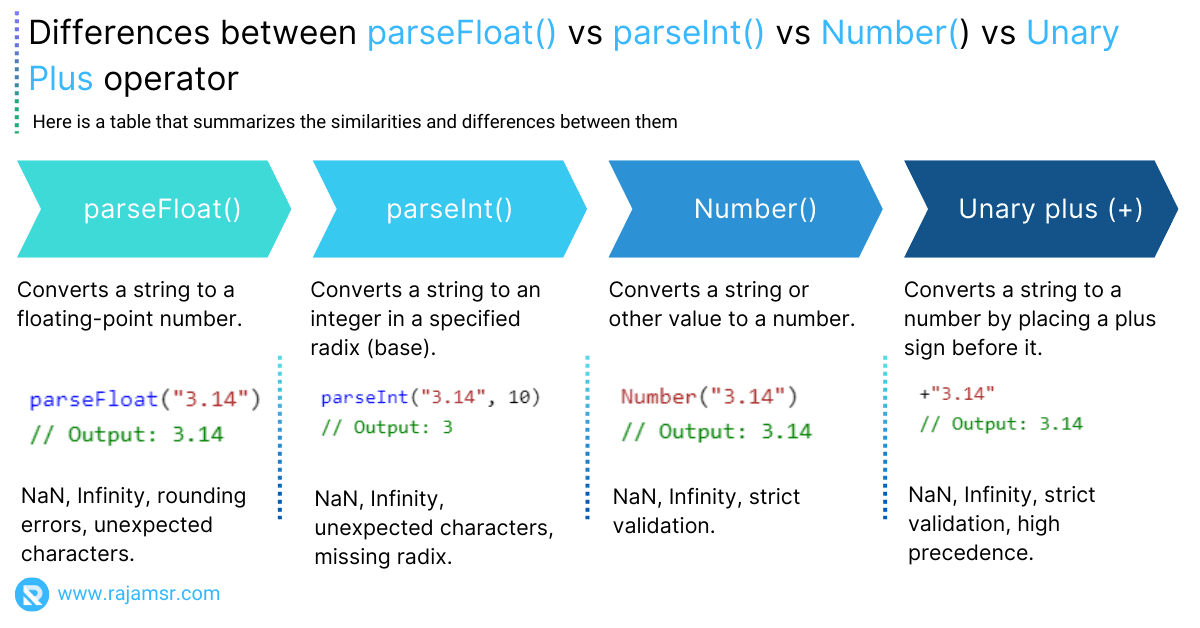
Conclusion
This blog covered the JavaScript parseFloat()
global function, including its syntax and utilization, a string-to-floating-point number conversion, returned values, best practices, and pitfalls.
To specify the number of digits after the decimal places, you can use the toFixed()
method. The isNan()
function is always a good choice to check the result of the parseFloat()
function because parseFloat()
returns NaN
if the conversion fails.
You have learned JavaScript parseFloat() with other similar functions, such as parseInt(), Number(), and unary plus (+), and seen when to use each one.
With examples, we discussed the differences and parallels between the global functions parseFloat()
and Number()
.
I hope that this blog post has helped you understand and use parseFloat() better, and that you have enjoyed reading!