If you are a web developer, you have probably encountered the need to JavaScript merge objects
at some point. Whether you want to combine data from different sources, extend the functionality of existing objects, or create new objects based on others, merging objects is a common and useful task.
But sometimes, you have to deal with multiple objects that contain different pieces of information, and you need to merge objects into one. How do you do that without losing or overwriting any data? Don’t worry; I have the answer for you.
In this article, we will explore:
- How to merge JavaScript objects in a simple way
- Merging one object into another
- JavaScript merges objects with and without overwriting
- Deep merge objects and merge with conditions
- Merging multiple arrays of objects
- Merging objects with different keys
- Merging JSON objects
- Merging objects without undefined values
- Merging objects without duplicates
- Object merging in ES6
- Grouping objects by key and value
By the end of this article, you’ll be able to master the skill of merging JavaScript objects and improve your coding efficiency. So, let’s get started.
How to merge objects in JavaScript?
The object is one of the non-primitive data types in JavaScript. Object merging involves combining the properties and values of two or more objects into a single object.
Here’s a straightforward method to achieve this:
const object1 = { name: 'Alice' };
const object2 = { age: 30 };
Object.assign(object1, object2);
console.log(object1);
// { name: 'Alice', age: 30 }
The Object.assign()
method is used to merge the properties of object2
into object1
.
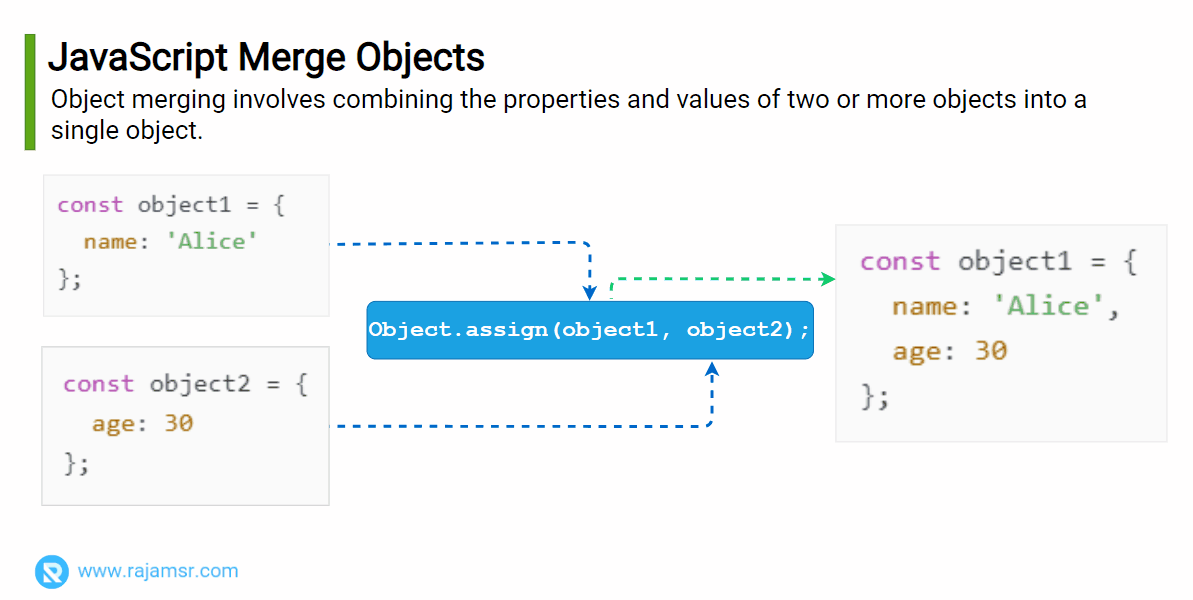
Merging one object into another
Sometimes, you might want to merge the properties of one object into another. Let’s start with a simple example of combining objects using the spread operator (...)
:
const object1 = { name: 'Alice', age: 30 };
const object2 = { profession: 'Engineer', age: 28 };
const mergedObject = { ...object1, ...object2 };
console.log(mergedObject);
// { name: 'Alice', age: 28, profession: 'Engineer' }
This is an example of using the spread operator (...)
to merge objects. The mergedObject
contains all the properties of object1
and object2
. If a property exists in both objects, the value from object2
overwrites the value from object1
. For instance, the age property in mergedObject
has the value from object2
, not object1
.
Merging objects with same keys overwriting existing value
When merging objects with the same keys, the default behavior is to overwrite existing key values. Let’s explore a scenario where we want to merge objects with the same keys and overwrite existing values:
const object1 = { name: 'Alice', age: 30 };
const object2 = { age: 28, profession: 'Engineer' };
const mergedObject = { ...object1, ...object2 };
console.log(mergedObject);
// { name: 'Alice', age: 28, profession: 'Engineer' }
Using the spread operator
to merge two objects, the last object key value overwrites the key value from the previous object if the key already exists. This is why object2
age
property value 28
overwrites the age property value 30
from object1
in this case.
JavaScript merge objects without overwriting
To prevent overwriting values during merging, you can manually check for existing keys before merging:
const object1 = { name: 'Alice', age: 30 };
const object2 = { age: 28, profession: 'Engineer' };
const mergedObject = { ...object1 };
for (const key in object2) {
if (!mergedObject.hasOwnProperty(key)) {
mergedObject[key] = object2[key];
}
}
console.log(mergedObject);
// Output: { name: 'Alice', age: 30, profession: 'Engineer' }
In this approach, the properties age
fromobject2
are only added if they don’t already exist in mergedObject
. The JavaScript hasOwnProperty() method returns true for the object that has its property with the specific name; otherwise, it returns false.
JavaScript Merging objects with spread operator
The spread operator is powerful for merging objects. Let’s see how to use it to merge objects using the spread operator (...)
:
const object1 = { name: 'Alice', hobbies: ['Reading', 'Cooking'] };
const object2 = { age: 28, hobbies: ['Painting', 'Traveling'] };
const mergedObject = {
...object1,
...object2,
hobbies: [...object1.hobbies, ...object2.hobbies],
};
console.log(mergedObject);
// Output: { name: 'Alice', age: 28,
hobbies: ['Reading', 'Cooking', 'Painting', 'Traveling'] }
In this example, properties from both objects are merged, and the hobbies arrays are concatenated to prevent overwriting.
JavaScript merge objects deep
In some cases, you might need to merge objects with nested properties. Shallow merging using the spread operator won’t suffice here, as it only merges top-level properties. For deep object merging, you can utilize a recursive approach:
function deepMerge(target, source) {
for (const key in source) {
if (source[key] instanceof Object) {
if (!target[key]) target[key] = {};
deepMerge(target[key], source[key]);
} else {
target[key] = source[key];
}
}
}
const object1 = { person: { name: 'Alice' } };
const object2 = { person: { age: 28 } };
const mergedObject = { ...object1 };
deepMerge(mergedObject, object2);
console.log(mergedObject); // Output: { person: { name: 'Alice', age: 28 } }
In this example, the deepMerge()
function recursively traverses the properties of object2
and merges them into object1
.
Recursively merge objects with conditions
Building upon the previous concept, let’s explore a scenario where you want to merge objects recursively, but only if a specific condition is met:
function recursiveMerge(target, source, condition) {
for (const key in source) {
if (source[key] instanceof Object) {
if (!target[key]) target[key] = {};
recursiveMerge(target[key], source[key], condition);
} else {
if (condition(key, source[key])) {
target[key] = source[key];
}
}
}
}
const object1 = { person: { name: 'Alice', age: 30 } };
const object2 = { person: { age: 28, profession: 'Engineer' } };
const mergedObject = { ...object1 };
recursiveMerge(mergedObject, object2, (key, value) => key !== 'age');
console.log(mergedObject);
// Output { person: { name: 'Alice', age: 30, profession: 'Engineer' } }
In this case, the recursiveMerge()
function applies the condition to merge properties only if the Object key is not "age"
.
Merging objects with same values
const object1 = { name: 'Alice', age: 30 }
const object2 = { name: 'John', age: 30 }
You want to merge these two objects based on the common value of the age
property. Here’s how you can do it:
function mergeObjectsByCommonValue(obj1, obj2, commonKey) {
if (obj1[commonKey] === obj2[commonKey]) {
const mergedObject = { ...obj1, ...obj2 };
return mergedObject;
} else {
return null; // Objects have different common value, cannot merge
}
}
const commonKey = "age";
const mergedObject = mergeObjectsByCommonValue(object1, object2, commonKey);
if (mergedObject) {
console.log(mergedObject);
} else {
console.log("Objects have different common value, cannot merge.");
}
// Output: {name: "John", age: 30}
In this example, the mergeObjectsByCommonValue()
function takes three arguments: obj1
, obj2
, and commonKey
. It checks if the values of the commonKey
property in both objects are the same. If they are the same, it creates a merged object by using the spread ({ ... })
syntax to copy properties from both objects.
If the common values are different, the function returns null
.
Merging multiple arrays of objects
There might be instances where you need to merge multiple arrays of objects into a single cohesive structure. Here’s a method to achieve that:
function mergeArraysOfObjects(...arrays) {
const mergedArray = [].concat(...arrays);
return mergedArray.reduce((acc, obj) => {
const existingObj = acc.find(item => item.id === obj.id);
if (!existingObj) {
acc.push(obj);
}
return acc;
}, []);
}
const array1 = [{ id: 1, name: 'Alice' }];
const array2 = [{ id: 2, name: 'Bob' }];
const array3 = [{ id: 1, age: 30 }];
const mergedArray = mergeArraysOfObjects(array1, array2, array3);
console.log(mergedArray);
// [{ id: 1, name: 'Alice', age: 30 }, { id: 2, name: 'Bob' }]
In this example, the mergeArraysOfObjects()
function combines multiple arrays while preventing duplicate objects based on a common identifier (in this case, the id
property).
The JavaScript array method find()
array method is used to find the object with the specific Id
.
Merging an array of objects in JavaScript
When dealing with an array of objects, you might want to merge them into a single object. Here’s how you can achieve that:
function mergeArrayOfObjects(array) {
return array.reduce((acc, obj) => ({ ...acc, ...obj }), {});
}
const arrayOfObjects = [
{ name: 'Alice', age: 30 },
{ profession: 'Engineer' },
{ hobbies: ['Reading', 'Cooking'] }
];
const mergedObject = mergeArrayOfObjects(arrayOfObjects);
console.log(mergedObject);
// Output:
{
name: 'Alice', age: 30, profession: 'Engineer,
hobbies: ['Reading', 'Cooking']
}
mergeArrayOfObjects()
function iterates through the array and merges each object into a single object.Merging objects with different keys
Handling objects with different keys can be challenging, but it’s possible to merge them while retaining all properties:
function mergeObjectsWithDifferentKeys(...objects) {
const mergedObj = {};
objects.forEach(obj => {
for (const key in obj) {
if (!mergedObj[key]) {
mergedObj[key] = [];
}
mergedObj[key].push(obj[key]);
}
});
return mergedObj;
}
const obj1 = { name: 'Alice', age: 30 };
const obj2 = { profession: 'Engineer', hobbies: ['Reading', 'Cooking'] };
const mergedObj = mergeObjectsWithDifferentKeys(obj1, obj2);
console.log(mergedObj);
// Output
{
name: ['Alice'],
age: [30],
profession: ['Engineer'],
hobbies: [['Reading', 'Cooking']]
}
mergeObjectsWithDifferentKeys()
function merges objects while creating arrays for properties with different keys.Merging JSON objects
JSON
objects are commonly used in web development for data exchange. Merging JSON
objects involves combining their properties while being mindful of their structure:
const json1 = { "name": "Alice", "age": 30 };
const json2 = { "age": 28, "profession": "Engineer" };
const mergedJson = { ...json1, ...json2 };
console.log(mergedJson);
// Output: { "name": "Alice", "age": 28, "profession": "Engineer" }
In this case, the spread operator efficiently merges properties from both JSON
objects.
Merging objects without undefined values
To avoid merging properties with undefined
values, you can apply the filter()
method before merging:
const object1 = { name: 'Alice', age: undefined };
const object2 = { age: 28, profession: 'Engineer' };
const filteredobject1 = Object.fromEntries(Object.entries(object1)
.filter(([_, v]) => v !== undefined));
const filteredobject2 = Object.fromEntries(Object.entries(object2)
.filter(([_, v]) => v !== undefined));
const mergedObject = { ...filteredobject1, ...filteredobject2 };
console.log(mergedObject);
// Output: {name: "Alice", age: 28, profession: 'Engineer' }
In this example, the Object.entries() and Object.fromEntries()
methods are used to filter out properties with undefined
values before merging.
Merging objects without duplicates
Preventing duplicate properties during merging is crucial for maintaining data integrity. Here’s a way to merge objects while avoiding duplicates:
function mergeObjectsWithoutDuplicates(...objects) {
const mergedObject = {};
objects.forEach(obj => {
for (const key in obj) {
if (!mergedObject.hasOwnProperty(key)) {
mergedObject[key] = obj[key];
}
}
});
return mergedObject;
}
const object1 = { name: 'Alice', age: 30 };
const object2 = { age: 28, profession: 'Engineer' };
const mergedObject = mergeObjectsWithoutDuplicates(object1, object2);
console.log(mergedObject);
// { name: 'Alice', age: 30, profession: 'Engineer' }
mergeObjectsWithoutDuplicates()
function ensures that duplicate properties are not added to the merged object.ES6 merge objects methods
ES6 introduced several features that streamline object merging. Let’s explore some of these enhancements:
1. Object.assign
The Object.assign
method enables concise object merging with multiple sources:
const object1 = { name: 'Alice' };
const object2 = { age: 30 };
const mergedObject = Object.assign({}, object1, object2);
console.log(mergedObject);
// Output: { name: 'Alice', age: 30 }
2. Spread Operator (...)
ES6’s spread operator can be used for object merging as well.
const object1 = { name: 'Alice' };
const additionalProps = { age: 30, profession: 'Engineer' };
const mergedObject = { ...object1, ...additionalProps };
console.log(mergedObject);
// Output: { name: 'Alice', age: 30, profession: 'Engineer' }
Joining object key-value pairs
Combining key-value
pairs from multiple objects is a useful technique. Here’s how you can achieve it:
const object1 = { name: 'Alice', age: 30 };
const object2 = { profession: 'Engineer', hobbies: ['Reading', 'Cooking'] };
const mergedKeyValues = Object.entries(object1).concat(Object.entries(object2));
console.log(Object.fromEntries(mergedKeyValues));
// Output:
{ name: 'Alice',
age: 30,
profession: 'Engineer',
hobbies: ['Reading', 'Cooking']
}
In this example, Object.entries()
and Object.fromEntries()
are used to join the key-value pairs from both objects.
Grouping objects by property value
Grouping objects based on a common property value can be helpful for data organization. Here’s an approach to achieving this:
function groupObjectsByPropertyValue(objects, property) {
return objects.reduce((groups, obj) => {
const value = obj[property];
if (!groups[value]) groups[value] = [];
groups[value].push(obj);
return groups;
}, {});
}
const objects = [
{ category: 'Fruit', name: 'Apple' },
{ category: 'Fruit', name: 'Orange' },
{ category: 'Vegetable', name: 'Carrot' }
];
const groupedByCategory = groupObjectsByPropertyValue(objects, 'category');
console.log(groupedByCategory);
// Output:
{
Fruit: [{ category: 'Fruit', name: 'Apple' }, { category: 'Fruit', name: 'Orange' }],
Vegetable: [{ category: 'Vegetable', name: 'Carrot' }]
}
groupObjectsByPropertyValue()
function groups objects from an array based on a specified property value.Grouping objects by key
Grouping objects by their keys is another technique for efficient data organization. Here’s how it can be done:
function groupObjectsByKey(objects) {
return objects.reduce((groups, obj) => {
for (const key in obj) {
if (!groups[key]) groups[key] = [];
groups[key].push(obj[key]);
}
return groups;
}, {});
}
const objects = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 28 }
];
const groupedByKey = groupObjectsByKey(objects);
console.log(groupedByKey);
// { name: ['Alice', 'Bob'], age: [30, 28] }
In this example, the groupObjectsByKey()
function groups values by their respective keys from the objects.
Conclusion
In this comprehensive guide, we’ve explored into different types of JavaScript object merging. From the basics of merging objects to advanced techniques like deep merging, recursive merging, and grouping objects, you’ve learned a wide array of strategies to efficiently handle and manipulate objects in your JavaScript applications.
You have learned how to merge objects with precision, avoid overwriting, and group data efficiently. These skills will help you tackle different scenarios that require data manipulation and organization.
Over to you: which object merging method are you going to use?