If you are a JavaScript developer, you probably know how useful objects are for storing and manipulating data. Do you know that there is a built-in static method in JavaScript that can help you iterate over the key-value pairs of an object simply and elegantly? It’s called Object.entries()
.
In this blog post, we will explore the following:
- What is the
Object.entries()
method, and what is the benefit? - Iterate through object entries using
forEach()
andMap()
methods - How to use
filter()
method on object entries - Differences between Object
keys()
and entries() methods - Object.entries vs for..in loop
- Troubleshoot
object()
entries, not a function error
Let’s explore the topic in detail with examples.
Object entries in JavaScript
Objects are one of the non-primitive data types in JavaScript. So, what is itObject.entries()
? Object.entries() is a static method that returns an array of a given object’s enumerable string-keyed property [key, value] pairs. It essentially returns an array of arrays. Each array element contains the key and value of an object’s property.
This is very handy when you want to iterate over an object’s properties, filter them, compare them, or transform them into another data structure.
Let’s look at a simple code example to understand how to use the object.entries()
method:
const person = {
name: "John Doe",
age: 30,
email: "john.doe@example.com"
};
console.log(Object.entries(person))
// Output:
// ["name", "John Doe"]
// ["age", 30]
// ["email", "john.doe@example.com"]
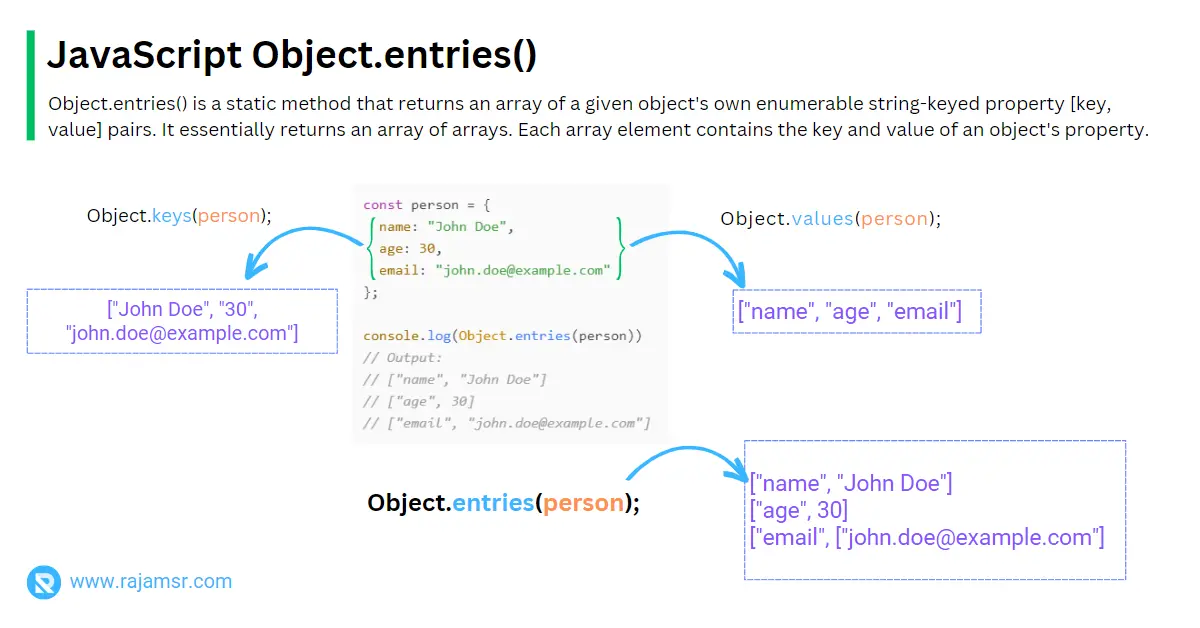
Object.entries()
method returns an array of arrays. Each array element is the key and value of the object’s properties.Iterate through object entries
The forEach()
, and map()
JavaScript array methods can be used to loop over array elements. It is often used with Object.entries()
to perform object manipulation which returns the key-value
pairs of an object.
1. Iterate JavaScript object entries using foreach
The common way to use Object.entries()
is with the forEach()
method. It allows you to loop through each element of the array and perform some action on it.
For example, you can use it to log each key-value pair to the console:
const person = {
name: "John Doe",
age: 30,
email: "john.doe@example.com"
};
Object.entries(person).forEach(([key, value]) => {
console.log(`${key}: ${value}`);
});
// Output:
// name: John Doe
// age: 30
// email: john.doe@example.com
In this example, we used Object.entries()
to get an array of arrays containing the key-value pairs of the person object. We then used the forEach()
method to loop over each key-value pair and log them to the console.
2. Iterate Object entries using map() method
entries()
and map()
together in JavaScript:const person = {
name: "John Doe",
age: 30,
email: "john.doe@example.com"
};
let mapped = Object.entries(person).map(([key, value]) => {
return `${key} = ${value}`;
});
console.log(mapped)
// Output:
// "name = John Doe"
// "age = 30"
// "email = john.doe@example.com"
In this example, we used Object.entries()
to get an array of arrays containing the key-value
pairs of the person object. The map()
array method is used to iterate array elements and return each key-value string.
Object.entries() filter
Another useful way to use Object.entries()
is with the filter()
method. The filter() method is used to create a new array with all elements that pass certain conditions. Let’s see an example of an object entry filter:
const books = [
{
title: 'Eloquent JavaScript',
price: 29.99,
isbn: '978-1593275846'
},
{
title: 'JavaScript: The Good Parts',
price: 15.99,
isbn: '978-0596517748'
},
{
title: 'You Don\'t Know JS',
price: 19.99,
isbn: '978-1491904244'
}
];
const filteredBooks = Object.entries(books)
.filter(([key, value]) => {
return value.price < 20
});
console.log(filteredBooks);
// Output
// [ {title: "JavaScript: The Good Parts" price: 15.99,isbn: '978-0596517748'}]
// [{title: "You Don't Know JS", price: 19.99, isbn: '978-1491904244'}]
In the example above, we first create an array of book
objects with three properties: title
, price
, and ISBN
.
We then use the entries (books
) method to get an array of [key, value]
pairs from books. We are using the JavaScript array method filter()
to filter all books with prices less than 20. In the books array, two objects match the condition, so those two books are returned as a result.
JavaScript Object.entries() vs Object.keys()
Let’s take a look at the differences between the Object entries()
and keys()
methods.
Object Keys | Object Entries |
---|---|
The JavaScript Object.keys() method returns an array of a given object’s own enumerable property names. The key names are in the same order as we get with a normal loop. | The entries() method returns an array of arrays, where each array element is the key and value of the object’s properties. |
If you only need the object keys or properties, you should use the | If you need both the key and value, you should use the Object entries() method. |
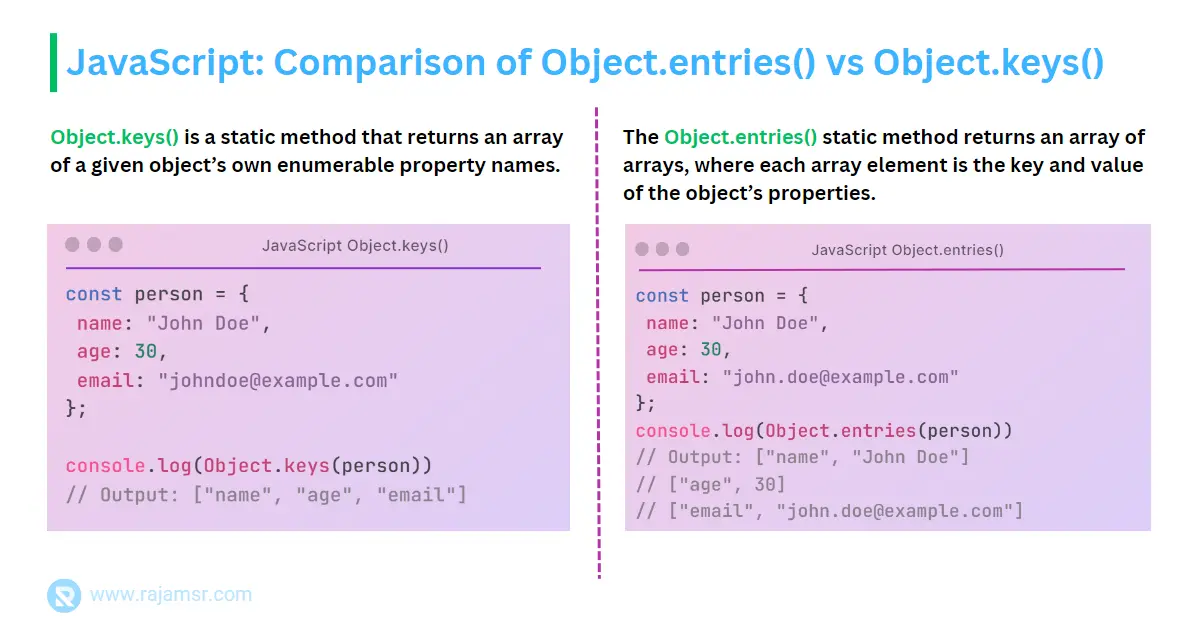
When to use the Object entries()
and when to use the keys()
method depends on what you need to do with the object.
JavaScript object.entries() vs for in loop
In JavaScript, Object.entries()
and for...in
are both used to iterate over the properties of an object. However, they have some key differences in terms of functionality and behavior. Let’s look at each of them:
Similarities between object.entries vs for in
- Both
Object.entries()
andfor...in
loop iterate over the enumerable properties of an object. - They both allow you to access the keys and values of an object.
Differences between object.entries vs for in
Object.entries()
returns an array of [key, value] pairs, whereasfor...in
loop directly gives you the key.Object.entries()
returns only own enumerable properties, whilefor...in
loop iterates over all enumerable properties, including inherited ones.Object.entries()
returns an array, which you can use array methods on, while the for…in loop doesn’t provide an array structure.
Let’s see how to use for..in
loop to iterate over an object.
const person = {
name: "John Doe",
age: 30,
email: "john.doe@example.com"
};
for (let key in person) {
console.log(`${key} = ${person[key]}`);
}
// Output:
// name = John Doe
// age = 30
// email = john.doe@example.com
Troubleshooting object.entries is not a function error
Sometimes you might get an error saying that "object.entries is not a function"
. This usually happens when you run your code in an Internet Explorer browser. According to the MDN site, this function is supported on all modern browsers.
You can add polyfills
to the Object.entries()
method to ensure your code works on all browsers.
if (!Object.entries) {
Object.entries = function (obj) {
var ownProperties = Object.keys(obj),
index = ownProperties.length,
result = new Array(index);
while (index--) {
result[index] = [ownProperties[index], obj[ownProperties[index]]];
}
return result;
};
}
If you use the Object entries()
method on non-object types such as numbers
, and booleans
, it will return an empty array. It will not throw any exceptions at modern browsers.
console.log(Object.entries(100));
// Output: []
console.log(Object.entries(true));
// Output: []
In the above object.entries()
example, object entries return an empty array for the number 100 and a boolean
value of true.
Conclusion
In conclusion, you can use the JavaScript Object.entries()
method to convert an object into an array of key-value pairs. This method can be useful when used along with other JavaScript methods for iterating over the properties or transforming them into a new data structure.
It can be used with forEach()
and filter()
to perform operations on the key-value pairs of an object. Additionally, we learned about the difference between object entries()
and keys()
methods and when to use each one.
Finally, we covered some troubleshooting tips for the "Object entries() is not a function"
error message.
Over to you: for which scenario are you going to use the Object entries()
method?