If you are a web developer, you probably use JavaScript array methods a lot. But do you know how they work and what they can do for you?
In this blog post, we will explore some of the most useful and powerful array methods in JavaScript, such as map, filter, reduce, and more.
Let’s dive in.
What is JavaScript array?
A JavaScript array is a data structure used to store multiple values in a single variable. It is a way to organize and manipulate collections of data. An array can hold elements of any data type, such as numbers, strings, objects, or even other arrays. The array is one of the non-primitive data types in JavaScript.
For example, the following example shows that multiple HTML tags were stored in a single JavaScript variable called htmlTags
.
var htmlTags = ['html', 'head', 'body', 'title', 'nav'];
Arrays in JavaScript offer various methods and properties for manipulation and iteration, allowing you to perform operations like adding, removing, or sorting elements.
Let’s now see array methods in detail.
JavaScript array methods
The JavaScript arrays have a lot of methods and properties, but I only covered 15 of them in this blog post, in no particular order.
The following array-method lists are covered:
- forEach()
- map()
- filter()
- reduce()
- find()
- findIndex()
- some()
- every()
- flat()
- flatMap()
- slice()
- splice()
- unshift()
- shift()
- includes()
Let’s see how to use these JavaScript array methods to manipulate arrays.
1. forEach()
forEach()
array method allows you to loop over an array and perform a function on each element. For example, let’s say we have an array of numbers and we want to print each number to the browser console:const numbers = [1, 2, 3, 4, 5];
numbers.forEach((number) => console.log(number));
// Output:
1
2
3
4
5
This code will print the array numbers 1 through 5 to the browser console.
2. map()
The map()
method allows you to create a new array by performing a function on each element of the original array.
For example, let’s say we have an array of numbers and we want to find a squared number for each number in the array.
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map((number) => number * number);
console.log(squaredNumbers);
// Output: [1, 4, 9, 16, 25]
3. filter()
The filter()
method allows you to create a new array by filtering out elements of the array that don’t meet a certain condition.
For example, let’s say we have an array of numbers and we only want to filter the even numbers. The filter()
array method returns a new array containing only even numbers.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(number => number % 2 == 0);
console.log(evenNumbers);
// Output: [2,4]
In the above code example, the JavaScript modulus operator (%) is used to filter even numbers.
4. reduce()
The reduce()
array method allows you to reduce an array to a single value by performing a function on each element of the array.
For example, let’s say we have an array of numbers and we want to calculate the sum of all the numbers without using any loops.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue);
console.log(sum);
// Output: 15
5. find()
The find() method allows you to find the first element in an array that meets a certain condition.
For example, let’s say we have an array of numbers and we want to find the first number which is less than 4:
const numbers = [1, 2, 3, 4, 5];
const number = numbers.find(number => number < 4);
console.log(number);
// Output: 1
The main distinction between the filter()
and find()
methods is that the filter()
function returns all elements that match the condition.
However, the find()
method will only return the first element that matches the condition, even if multiple elements are matching.
6. findIndex()
The findIndex()
method allows you to find the index of the first element in an array that meets a certain condition.
For example, let’s say we have an array of numbers between 1 and 5, and we want to find the index of the number 5.
const numbers = [1, 2, 3, 4, 5];
const index = numbers.findIndex((number) => number === 5);
console.log(index);
// Output: 5
7. some()
some()
method is a more concise and readable way of determining if an element exists in an array or not.Let’s say we have a number array. To check whether the number 5 exists in the array using the some()
method,const numbers = [1, 2, 3, 4, 5];
const index = numbers.some(number => number === 5);
console.log(index);
// Output: true
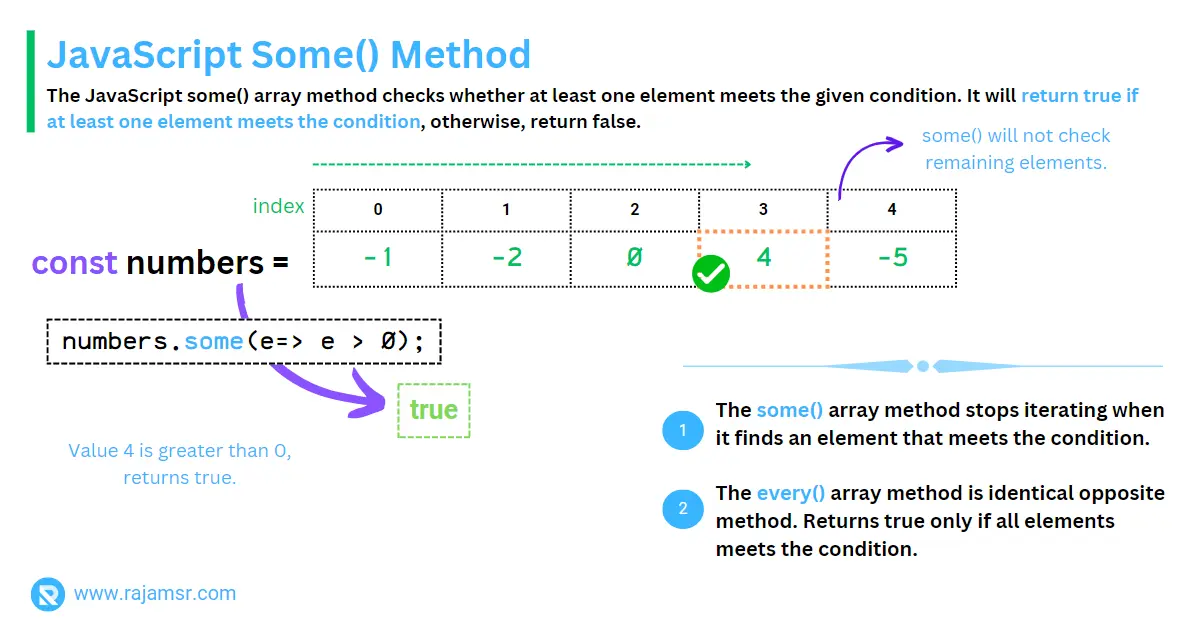
8. every()
The JavaScript every() array method allows you to check if every element in an array meets a certain condition and returns true; otherwise, it returns false.
For example, let’s say we have numbers 2, 4, 6, 8, and 10
in an array and we want to check if all are even numbers.
const numbers = [2, 4, 6, 8, 10];
const allEvenNumbers = numbers.every((number) => number % 2 === 0);
console.log(allEvenNumbers);
// Output: true
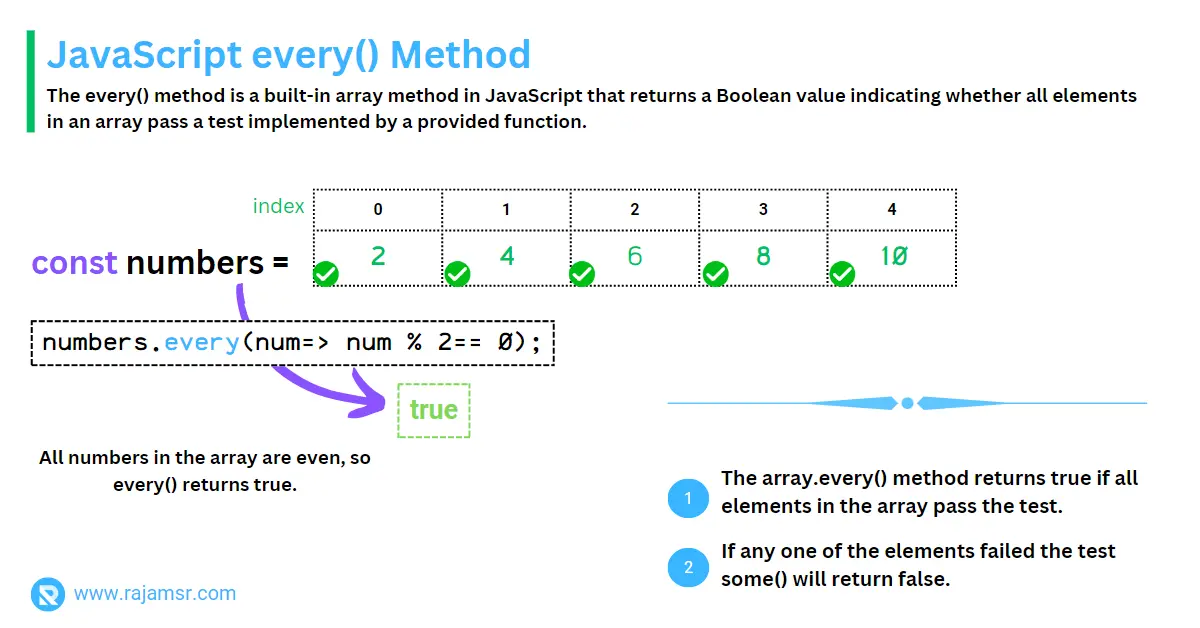
The main distinction between the some()
and every()
method is that some()
returns true if at least one element matches the condition, whereas every()
returns true only if all elements satisfy the condition.
9. flat()
The flat()
method allows you to flatten a nested array into a single-level array. For example, let’s say we have an array of arrays:
const nestedArray = [[1],
[2, 3, 4],
[5, 6,7,8]];
We can use the flat()
method to flatten it into a single-level array.
const flatArray = nestedArray.flat();
console.log(flatArray);
// Output: [1, 2, 3, 4, 5, 6, 7, 8]
10. flatMap()
flatMap()
method allows you to map an array and then flatten the result into a single-level array. For example, let’s say we have an array of words and we want to create a new array with the length of each word:const months = ['January', 'February', 'March', "April", "May"];
const wordLengths = months.flatMap(word => word.length);
console.log(wordLengths);
// Output: [7, 8, 5, 5, 3]
11. slice()
The JavaScript slice() method allows you to create a new array from a portion of an existing array. For example, let’s say we have an array of numbers and we want to create a new array with the first three numbers:
const numbers = [1, 2, 3, 4, 5];
const slicedNumbers = numbers.slice(0, 3);
console.log(slicedNumbers);
// Output: [1, 2, 3]
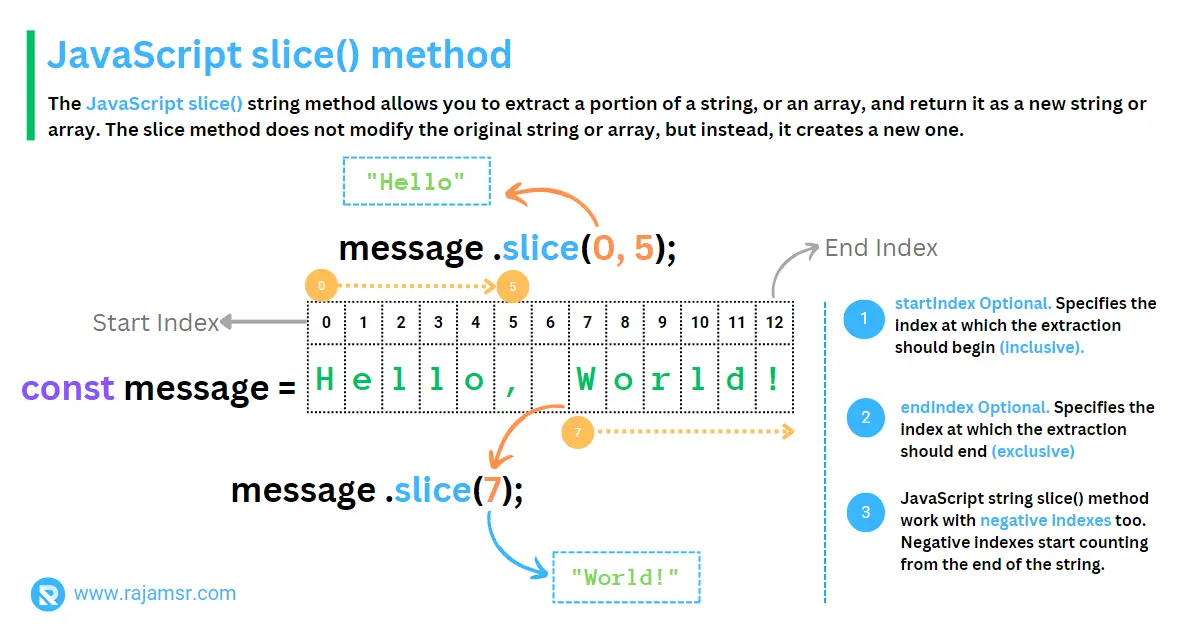
12. splice()
The splice()
method allows you to add or remove elements from an array. For example, let’s say we have an array of numbers and we want to remove one element from index 4
. The splice()
method will update the original array.
const numbers = [1, 2, 3, 4, 5];
numbers.splice(4, 1);
console.log(numbers);
// Output: [1, 2, 3, 4]
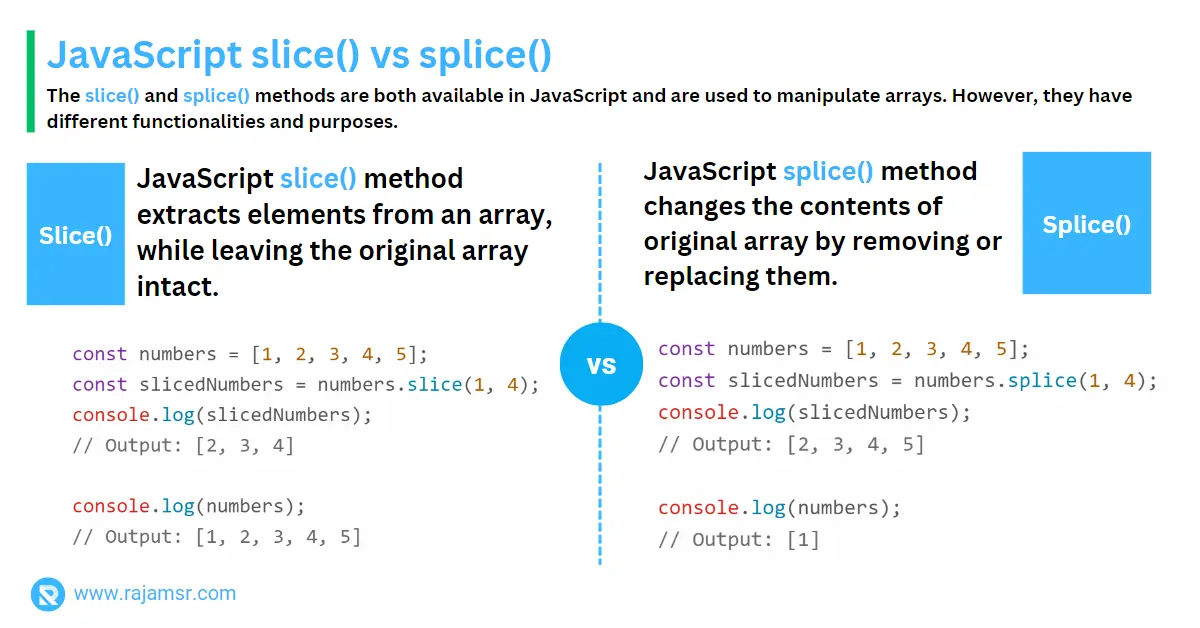
13. unshift()
The JavaScript unshift() function enables you to insert elements at the start of an array. It accepts any number of elements, and each element is added to the array as a new element.
Let’s see an example of how to manipulate arrays with the unshift()
method.
let options = ['Yes', 'No'];
options.unshift('Select');
console.log(options);
// Output: ["Select", "Yes", "No"]
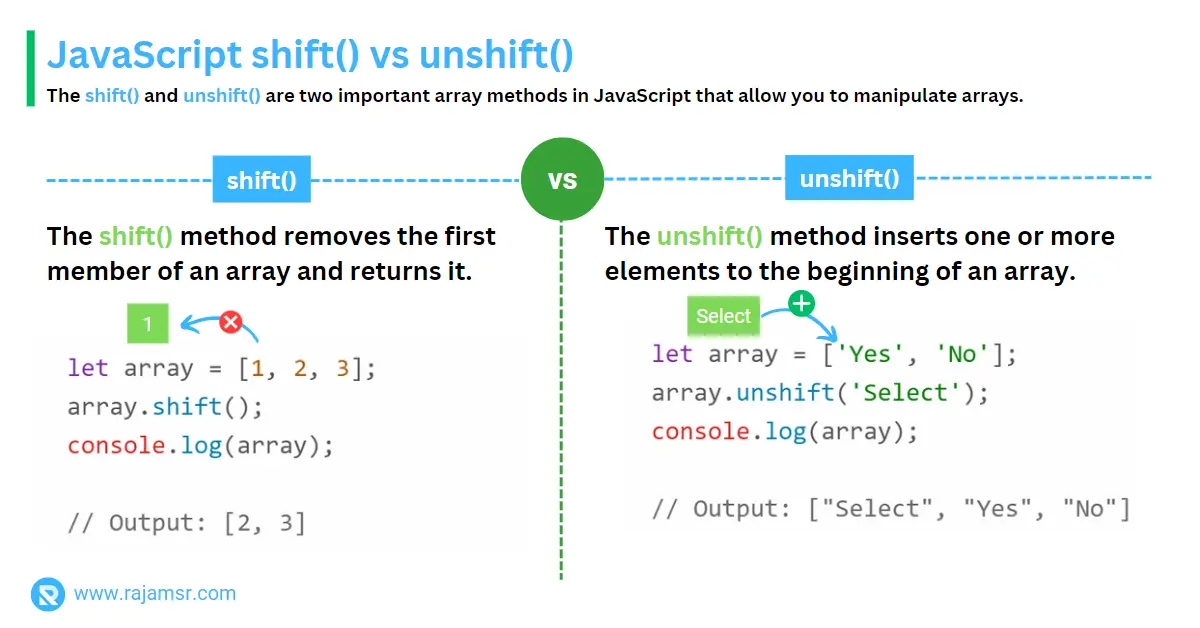
14. shift()
The JavaScript shift() is one of the built-in array methods. Which is used to remove the first element of an array. It will modify the original array, reduce the size, and return the removed elements. If the array is empty, the shift()
method will return "undefined”
and the array is not modified. Example of the shift()
method:
const evenNumbers = [2, 4, 6, 8, 10];
const shiftedNumber = evenNumbers.shift();
console.log(evenNumbers);
// Output: [4, 6, 8, 10]
console.log(shiftedNumber);
// Output: 2
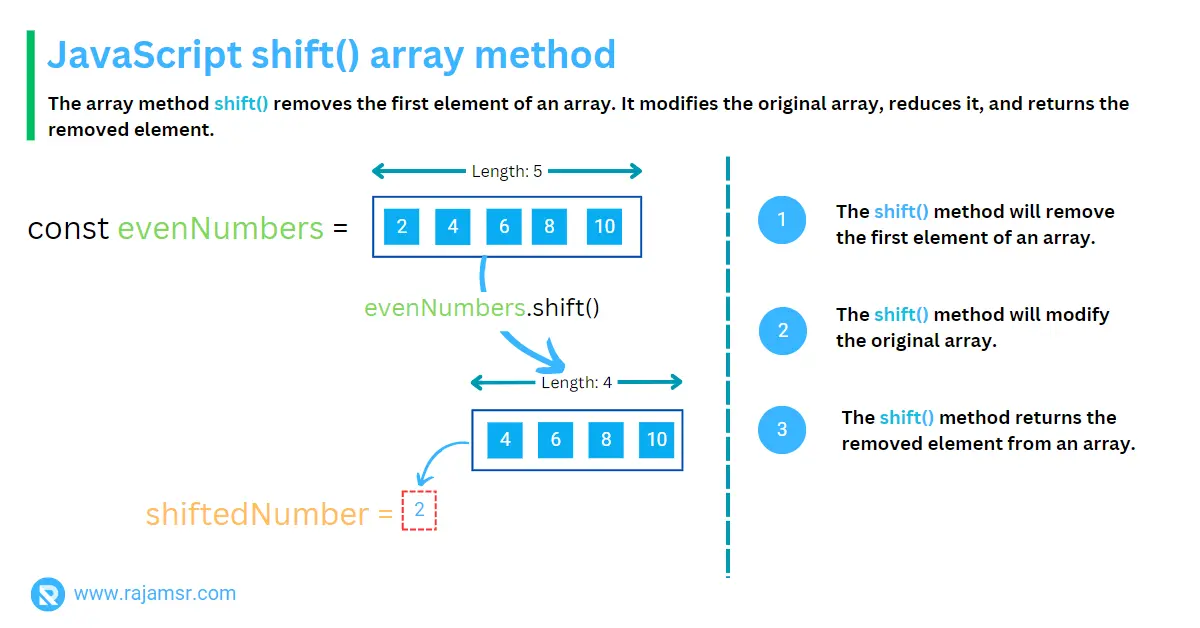
15. includes()
The JavaScript includes() function is a method that is used to check whether a given value or element exists within an array or a string. The includes()
method returns a boolean
value of true if the specified value is found in the array or JavaScript string, and false otherwise.
Example of the includes()
method:
const months = ['Jan', 'Feb', 'Mar', 'Apr', 'May'];
console.log(months.includes('Apr'));
// Output: true
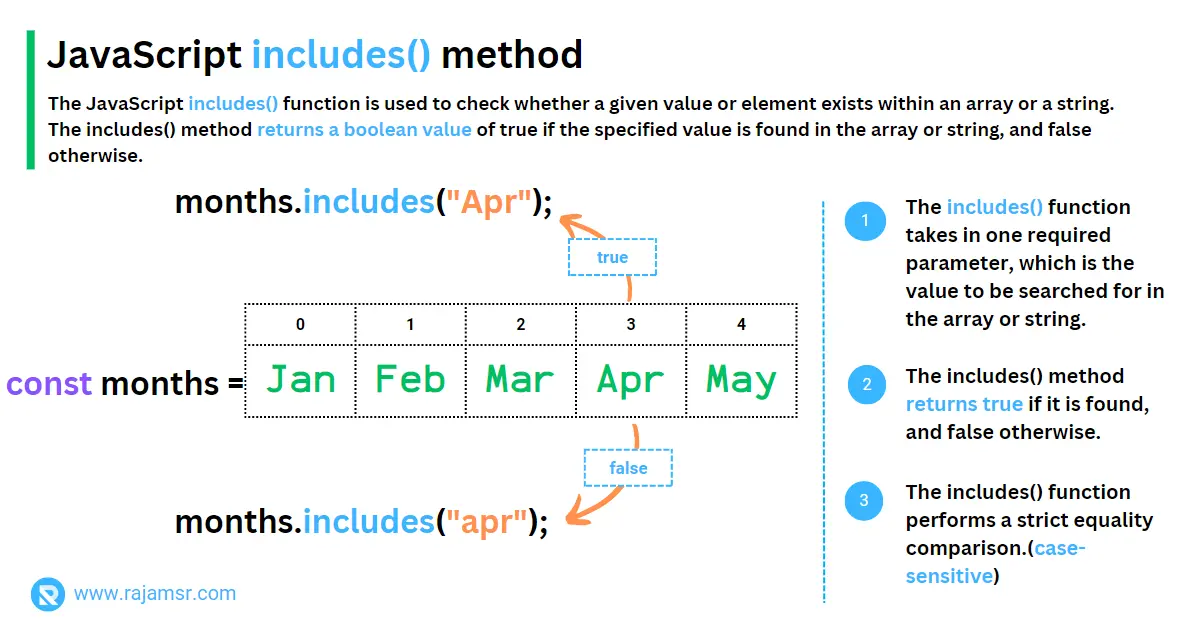
JavaScript array length property
Using the JavaScript array length property (not function), we can set or get the number of elements in the array. You can access the length
property of an array using dot (.) notation, like this:
const numbers = [1, 2, 3, 4, 5];
console.log(numbers.length);
// Output: 5
In the above code example, the length
property returns length of the array numbers.
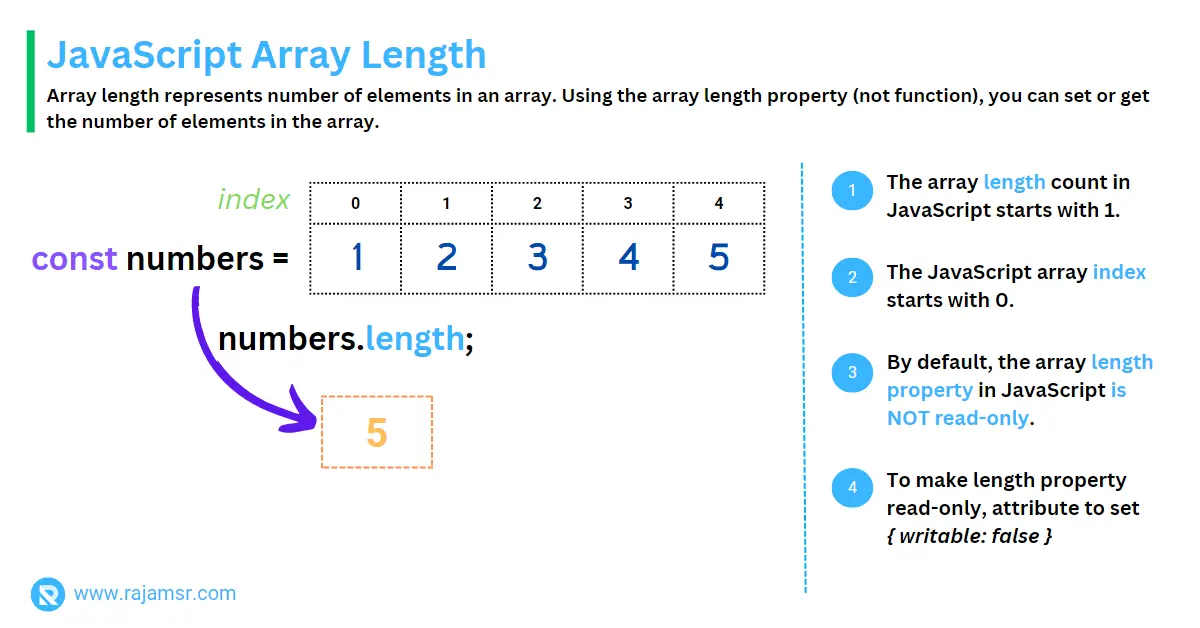
Tips for using JavaScript array methods
Using arrow functions with array methods
Arrow functions are a concise way to define functions in JavaScript, and they’re commonly used with array methods. For example, instead of writing:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
We can use an arrow function:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(number => { console.log(number) });
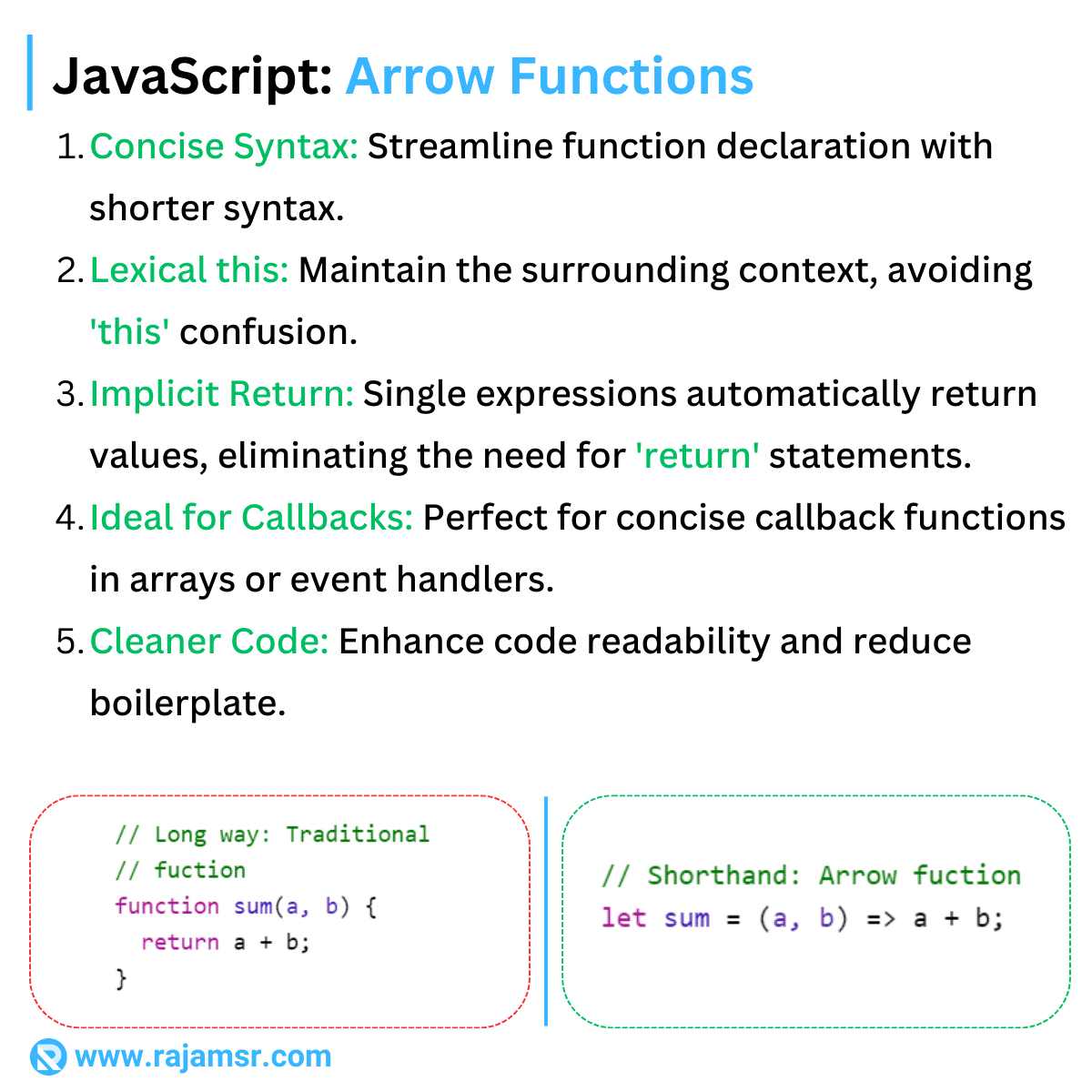
Chaining array methods
One of the benefits of array methods is that you can chain them together to create more complex operations on arrays. For example, let’s say we have an array of numbers and we want to find the sum of the even numbers:
const numbers = [1, 2, 3, 4, 5];
const sumOfEvens = numbers.filter((number) => number % 2 === 0)
.reduce((accumulator, currentValue) => accumulator + currentValue);
console.log(sumOfEvens);
// Output: 6
Using the spread operator to manipulate arrays
A spread operator is a powerful tool for manipulating arrays in JavaScript. It allows you to easily combine, clone, or destructure arrays
. For example, let’s say we have two arrays of numbers and we want to combine them into a single array:
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const combinedArray = [...array1, ...array2];
console.log(combinedArray);
// Output: [1, 2, 3, 4, 5, 6]
Handling empty arrays
When working with arrays, it’s important to handle cases where the array is empty. For example, let’s say we have an array of numbers and we want to find the largest number:
const numbers = [];
const maxNumber = numbers.reduce((accumulator, currentValue) => Math.max(accumulator, currentValue), -Infinity);
console.log(maxNumber);
// Output: -Infinity
If we run this code with an empty array, it will log infinity. To handle this case, we can provide an initial value to the reduce()
method:
const numbers = [];
const maxNumber = numbers.reduce((accumulator, currentValue) => Math.max(accumulator, currentValue), null);
console.log(maxNumber);
// Output: null
Conclusion
In conclusion, JavaScript array methods are powerful tools that can help you manipulate, transform, and organize your data.
Whether you need to sort, filter, map, reduce, or find elements in an array, there is a method that can do the job for you.
In this blog post, I have covered some of the most common and useful array methods in JavaScript, such as push
, pop
, shift
, unshift
, splice
, slice
, concat
, join
, sort
, indexOf
, lastIndexOf
, includes
, every
, some
, find
, findIndex
, filter
, map
, forEach
and reduce
.
I hope you have learned something new and are ready to apply these methods to your own projects.