If you are looking for a solution to get object properties and values using a simple method then you are landed in the correct place. Using the JavaScript object keys static method you can access all object keys. The object keys are nothing but object property names.
In this article, we’ll discuss the following items:
- What are object keys?
- Object keys on integers, special characters, and underscore
- Iterating over object keys
- Sort object keys
- What are object values
- Iterate through object value
Let’s see each topic in detail.
What are JavaScript object keys?
JavaScript Object is nothing but key-value pairs. The object is one of the non-primitive data types in JavaScript. JavaScript Object.keys()
static method returns objects’ property names as an array of strings. An object can have any number of keys or properties. Each key can have a value, it could be anything.
Let’s see an example of how to get the keys of an object in JavaScript:
const person = {
name: 'John',
age: 30,
city: 'New York'
};
const objectKeys = Object.keys(person);
console.log(objectKeys);
// Output: ["name", "age", "city"]
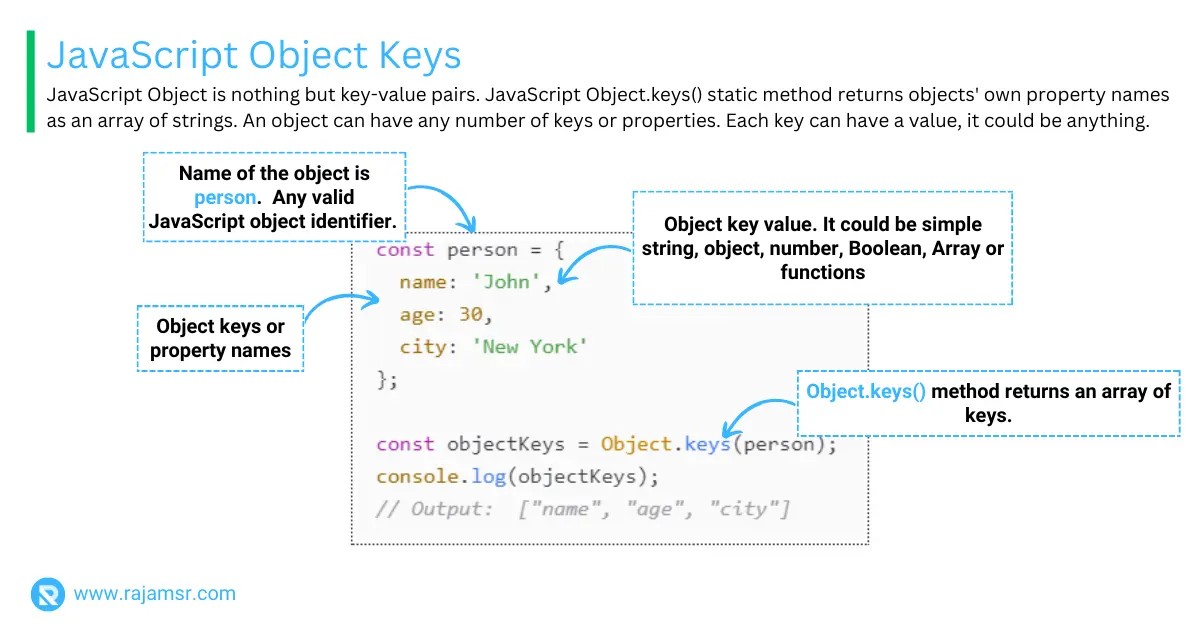
In the above code, Object.keys()
returns an array of keys. You can use the length property on the keys array to find the JavaScript object length or the number of properties in an object.
Object integer keys
The keys can also be integers
. If an object’s keys are integers, they will be sorted in ascending order when accessed.
const person =
{
1: 'John',
3: 'Doe',
2: 'Smith'
};
const objectKeys = Object.keys(person);
console.log(objectKeys);
// Output: ['1', '2', '3']
Keys with special character
The keys can also contain special characters
, such as spaces
or hyphens
. However, it’s not recommended to use special characters in keys, as it can lead to confusion and errors.
For example, the following example shows that keys contain empty spaces.
const person =
{
'first name': 'John',
'last name': 'Doe'
};
const objectKeys = Object.keys(person);
console.log(objectKeys);
// Output: ['first name', 'last name']
Keys beginning with underscore
The keys can also begin with an underscore. However, convention dictates that keys beginning with an underscore
are private and should not be accessed outside of the object.
const person = {
_firstName: 'John',
_lastName: 'Doe'
};
const objectKeys = Object.keys(person);
console.log(objectKeys);
// Output: ['_firstName', '_lastName']
You cannot use other special characters such as hyphens (-), dot (.), &, (, ),
and any JavaScript keywords
in object key names. You can use any valid JavaScript identifier as the property name.
JavaScript object keys loop
There are several ways in JavaScript to loop through keys. The most popular ones are the forEach()
loop method.
The forEach()
method can be used to loop through object keys and access their corresponding values.
const person =
{
name: 'John',
age: 30,
city: 'New York'
};
Object.keys(person).forEach(key => {
console.log(key + ': ' + person[key]);
});
// Output:
// name: John
// age: 30
// city: New York
Sort object keys
You can use the sort()
method to sort object keys in ascending or descending order. By default, it will sort keys in ascending order.
const person =
{
name: 'John',
age: 30,
city: 'New York'
};
console.log(Object.keys(person).sort())
// Output: ["age", "city", "name"]
How to use Object.keys () with arrays and strings
Object.keys()
returns an array of the property names of an object. But did you know that you can also use it with arrays and strings? Let’s see how.Object keys on arrays
When you use Object.keys()
with an array, it returns an array of the indices of the array elements. For example:
let colors = ["Red", "Green", "Blue"];
console.log(Object.keys(colors));
// Output: ["0", "1", "2"]
This can be useful when you want to iterate over the array elements using a for loop. For example:
let colors = ["Red", "Green", "Blue"];
let keys = Object.keys(colors);
for (let i = 0; i < keys.length; i++) {
let key = keys[i];
console.log(key + ": " + colors[key]);
}
/* Output:
0: Red
1: Green
2: Blue
*/
Object keys on strings
When you use Object.keys()
with a JavaScript string, it returns an array of the indices of the string characters. For example:
let greet = "Hello";
console.log(Object.keys(greet));
// Output: ["0", "1", "2", "3", "4"]
This can be useful when you want to manipulate the string characters like JavaScript string reverse, capitalizing the first letter of a word. For example:
// Reverse the string
let originalString = "Hello";
let reversed = Object.keys(originalString)
.reverse()
.map((key) => originalString[key])
.join("");
console.log(reversed);
// Output: "olleH"
// Capitalize the first letter
let greet = "javascript";
let capitalized = Object.keys(greet)
.map((key, index) => (index === 0 ? greet[key].toUpperCase() : greet[key]))
.join("");
console.log(capitalized);
// Output: "Javascript"
Object.keys () vs other methods
Object.keys()
is not the only method that can return an array of property names in JavaScript. There are also other methods, such as Object.getOwnPropertyNames()
and JavaScript Object.entries(), that can do similar things. However, there are some differences between them that you should be aware of.
Here is a comparison table that summarizes the main differences:
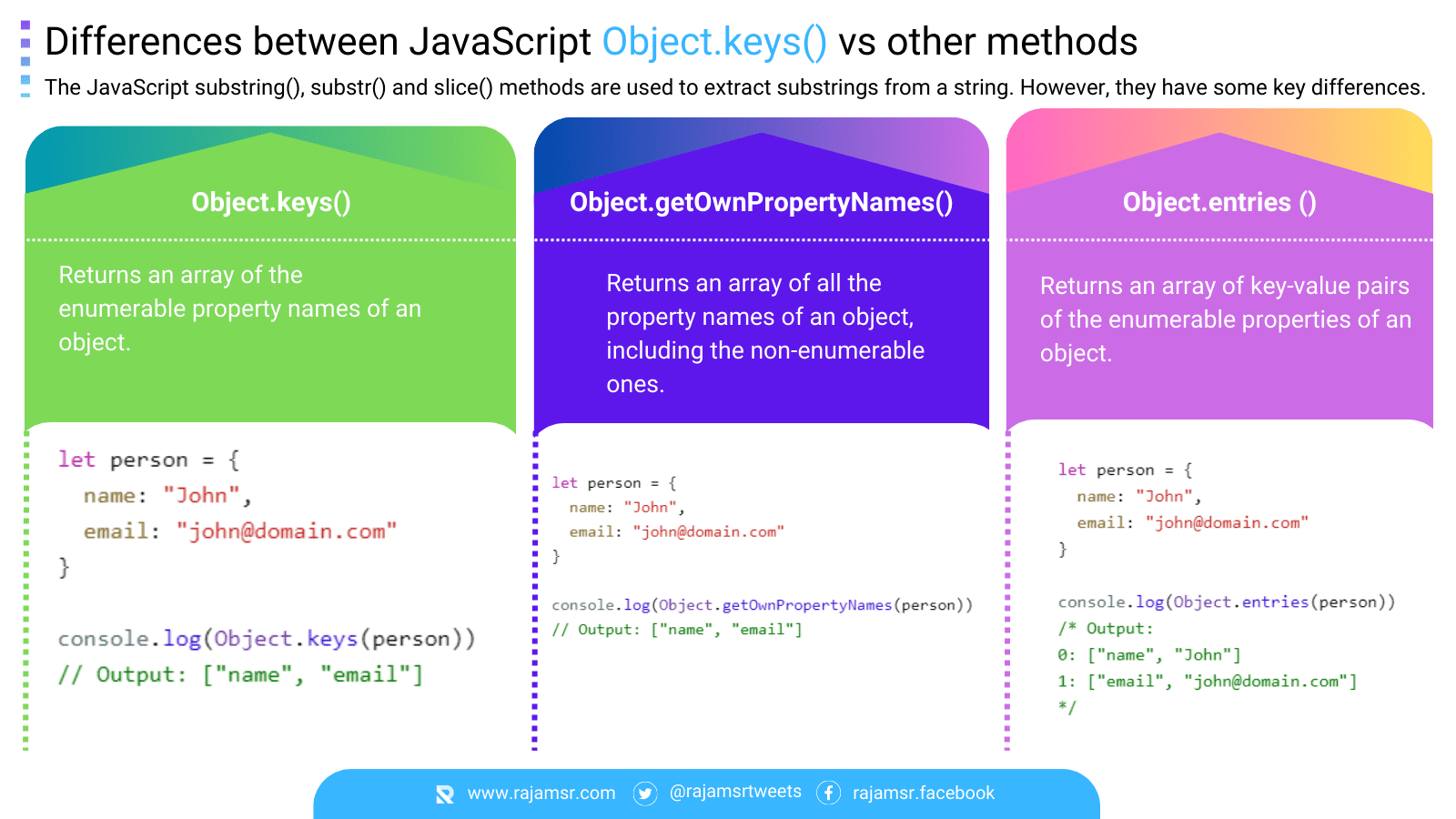
JavaScript object values using Object.values()
JavaScript object values are the corresponding values of keys. You can access them using the Object.values()
static method as shown in the following example:
const person =
{
name: 'John',
age: 30,
city: 'New York'
};
const objectValues = Object.values(person);
console.log(objectValues);
// Output: ['John', 30, 'New York']
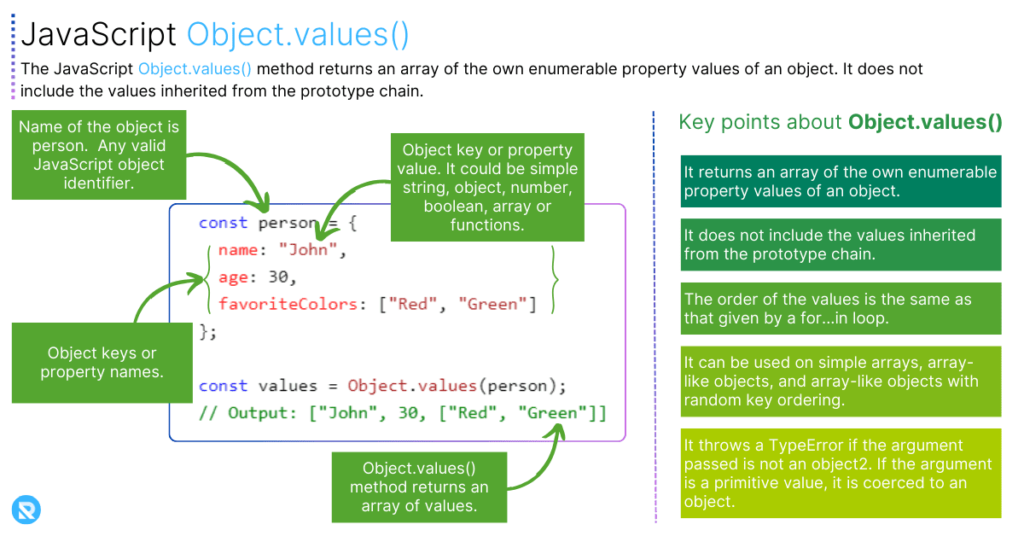
Alternatively, you can use Object.entries()
method to read object properties as key-value
pairs as an array.
Iterate through JavaScript object values
You can loop over object values using the forEach()
method.
const myObject = { name: 'John', age: 30, city: 'New York' };
Object.values(myObject).forEach(value => {
console.log(value);
});
// Output:
// John
// 30
// New York
ECMAScript standards and browser compatibility
Object.keys () was introduced in ECMAScript 5, which was released in 2009. Since then, it has been widely supported by most modern browsers, such as Chrome, Firefox, Safari, and Edge.
However, some older browsers, such as Internet Explorer 8 or below, do not support Object.keys(). If you want to use Object.keys () in these browsers. You will need to use a polyfills. The polyfill is a block of code that mimics the functionality of a newer feature in an older environment.
Conclusion
In conclusion, JavaScript object keys and iteration methods are important to understand when working with objects in JavaScript.
You have learned how to access object keys, iterate over them using various methods, and access JavaScript object values.
By mastering these concepts, you can write more efficient and error-free code.