Have you ever wondered how to JavaScript round to 2 decimal places? If you are working with numbers in JavaScript, you might encounter situations where you need to display or manipulate decimal values with a certain precision. Maybe you need to display a currency value or a percentage in a user-friendly format. Or maybe you want to avoid floating-point errors when performing calculations with decimals.
Whatever the reason, you can use a simple and elegant solution to round any number to two decimal places in JavaScript.
In this post, we will explore:
- Round to 2 decimal places
- Rounding number with trailing zeros
- Rounding number without trailing zeros
- Rounding for currency
- Using BigRational library
- Handling whole numbers and converting the rounded result back to a number data type.
By the end of this post, you will be able to round to two decimal places with ease and confidence.
JavaScript round to 2 decimals
To round a number in JavaScript, you can make use of the JavaScript math function Math.round()
. It rounds the number to the nearest integer. But how can we round to two decimal places? Let’s see an example of round to 2 decimal places:
const number = 3.14159;
const roundedNumber = Math.round(number * 100) / 100; // 314.159/100
console.log(roundedNumber);
// Output: 3.14
In the above code, we multiplied the number by 100
to shift the decimal point two places to the right. Then, we used Math.round()
to round the result. Finally, we divided it by 100 to shift the decimal point back to the original position.
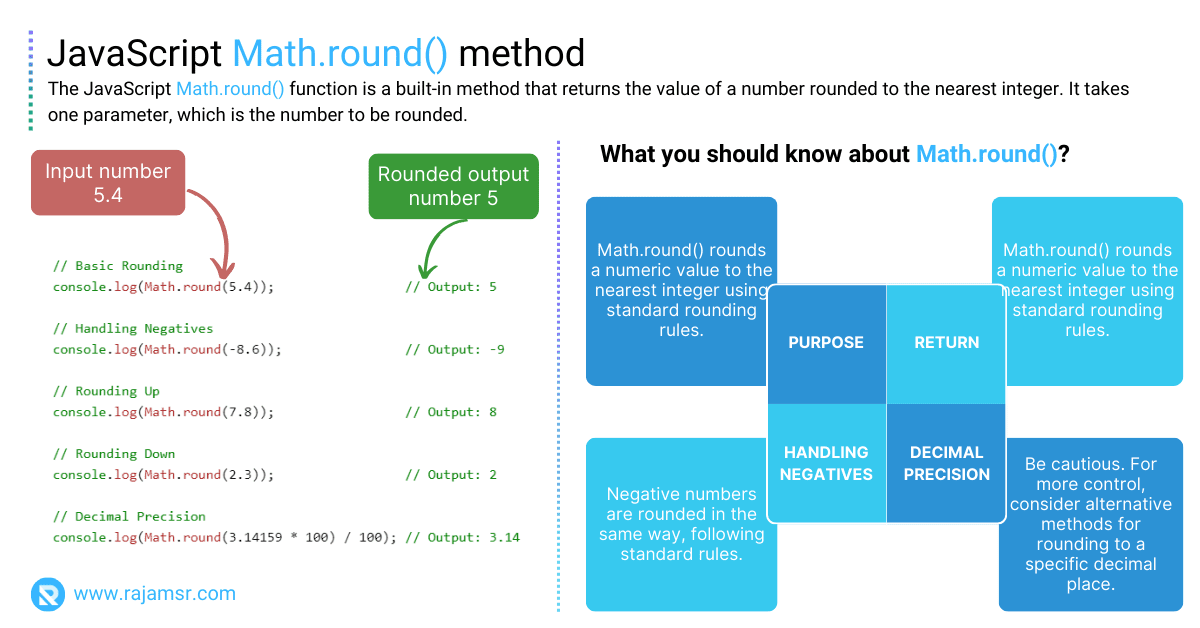
JavaScript round to 2 decimal places with trailing zeros
Sometimes, it’s necessary to include trailing zeros in rounded numbers, especially when dealing with currencies. To achieve this, you can use the JavaScript toFixed() method. Consider the following floating point number example round to 2 decimal places with trailing zeros:
const number = 10.5;
const roundedNumber = number.toFixed(2);
console.log(roundedNumber);
// Output: "10.50"
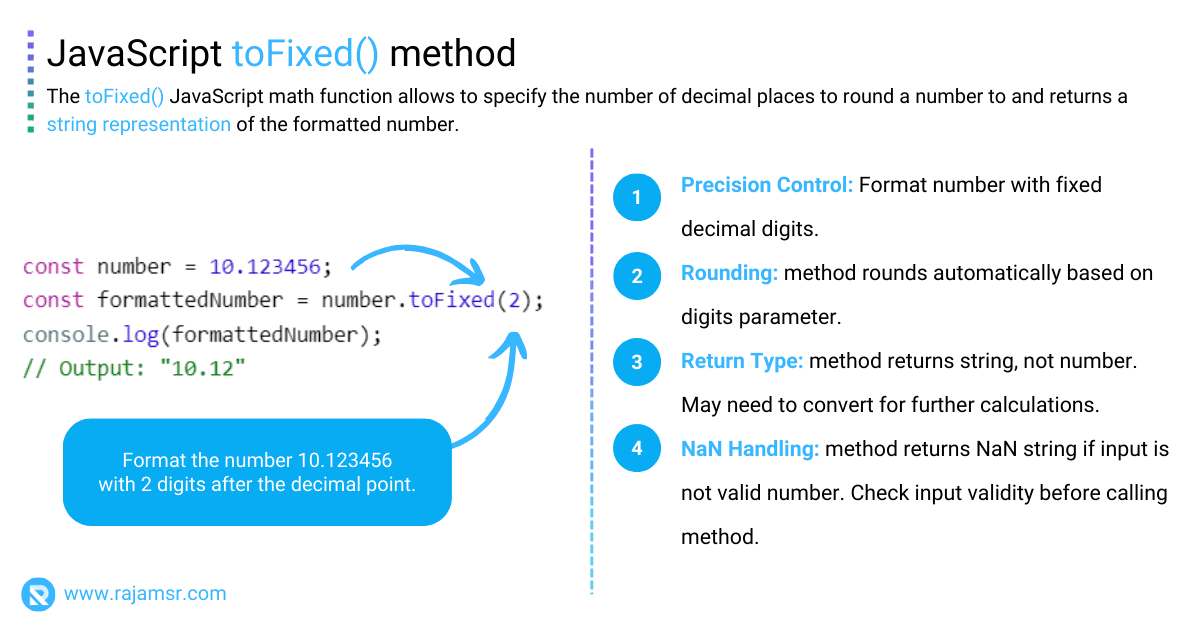
In the above code, we used the toFixed()
method and passed the desired number of decimal places (2 in this case) as an argument. It returns a string representation of the rounded number, including trailing zeros.
If you want to round to 4 decimal places, you can use 4 as the toFixed()
parameter. It will return a string representation of that number.
const number = 10.5343434;
const roundedNumber = number.toFixed(4);
console.log(roundedNumber);
// Output: "10.5343"
JavaScript round to 2 decimal places without trailing zeros
On the other hand, you might encounter situations where trailing zeros are undesired. In such cases, you can use the JavaScript parseFloat() method to convert the rounded string back to a number while removing the trailing zeros.
Let’s take a look at round a number without trailing zeros:
const number = 8.7500;
const roundedNumber = parseFloat(number.toFixed(2));
console.log(roundedNumber);
// Output: 8.75
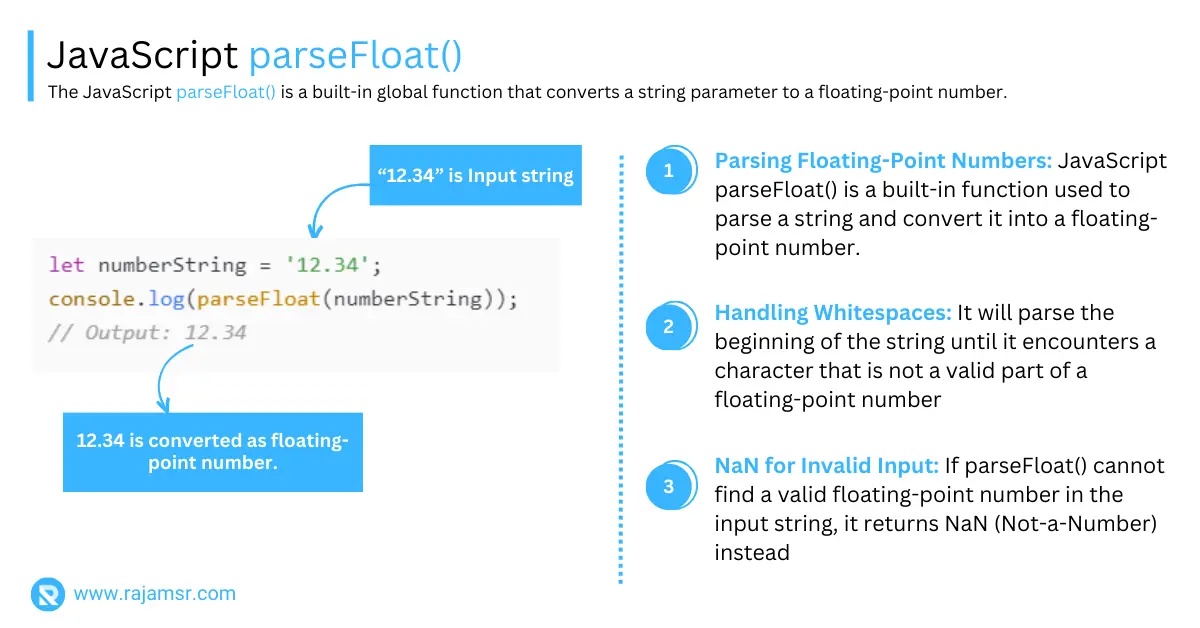
In the above code, we used toFixed()
as before, but then applied parseFloat()
to convert the string representation back to a number. parseFloat()
removes the trailing zeros and returns a numeric value.
JavaScript round to 2 decimal places for currency
Accurate representation of currency is crucial in financial applications. To round numbers specifically for currency, we can combine the toFixed()
and parseFloat()
methods.
Here’s an example:
const number = 99.9999;
const roundedNumber = parseFloat(number.toFixed(2));
console.log(roundedNumber);
// Output: 100
toFixed()
to round the number to two decimal places. Then, we applied parseFloat()
to remove any trailing zeros and convert it back to a number. The result is rounded to the nearest whole number, as per currency rounding conventions.JavaScript round 2 decimals unless it is whole
In certain cases, you might want to skip rounding if the number is a whole number. You can achieve this by checking if the number is whole before applying rounding. Here’s an example of round 2 decimals unless it is whole number:
const number = 4.0;
const roundedNumber = (number % 1 === 0)
? number
: parseFloat(number.toFixed(2));
console.log(roundedNumber);
// Output: 4
In the above code, we used the JavaScript modulus operator (%) to check if the number is a whole number (i.e., the remainder after division by 1 is 0). If it is, we skip rounding and return the original number. Otherwise, we apply rounding using parseFloat()
, as shown before.
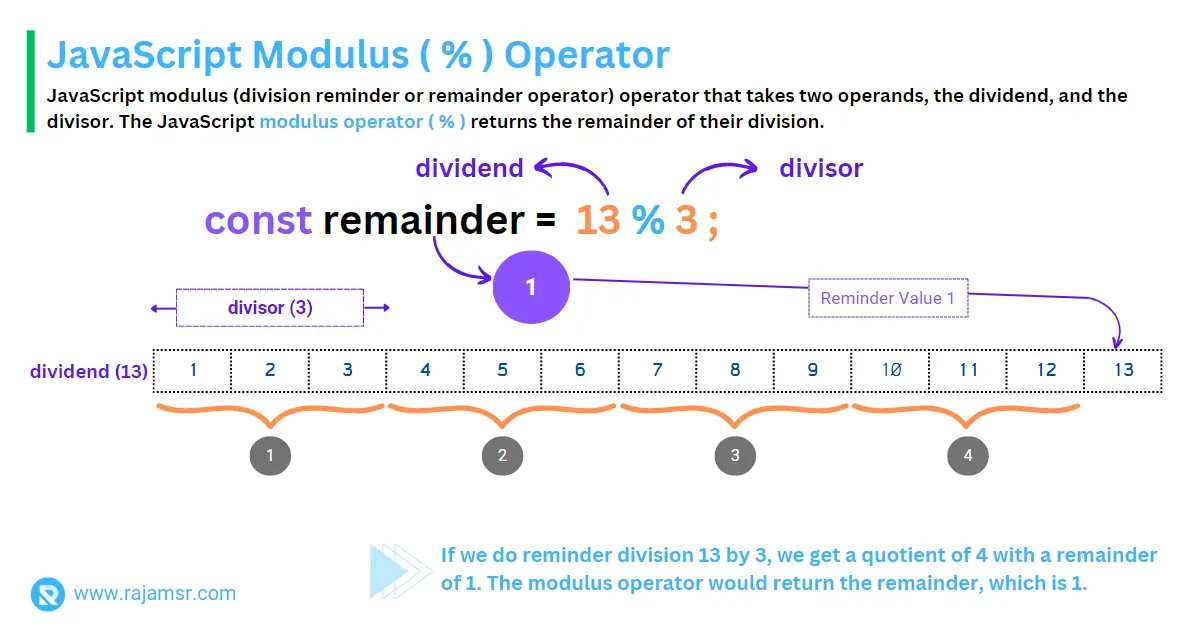
JavaScript math round to 2 decimal places as a number
After rounding a number, it is sometimes necessary to convert the result back to a number data type for further calculations. We can achieve this using parseFloat()
or the Number()
function. Here’s an example:
const roundedNumber = "5.75";
const convertedNumber1 = parseFloat(roundedNumber);
const convertedNumber2 = Number(roundedNumber);
console.log(convertedNumber1);
// Output: 5.75
console.log(convertedNumber2);
// Output: 5.75
In the above code, we used parseFloat()
and Number()
to convert the rounded number, represented as a string, back to a number. Both methods produce the same numeric result.
Rounding numbers using BigRational library
When dealing with highly precise calculations, the built-in JavaScript number type may not suffice. In such cases, we can employ the BigRational library. You’ll need to install and set it up first. Here’s an example of round to two decimals using library:
// Installation: npm install big-rational
const BigRational = require('big-rational');
const number = BigRational(1.23456789);
const roundedNumber = number.toFixed(2);
console.log(roundedNumber.toString());
// Output: "1.23"
In the above code, we imported the BigRational
library and created an BigRational
object with the desired number. Then, we used the toFixed()
method to round it to two decimal places. Finally, we converted the result to a string representation for display.
Frequently asked questions
Yes, you can round a number down to 2 decimal places using Math.floor()
. Here’s an example:
const number = 3.98765; const roundedDownNumber = Math.floor(number * 100) / 100; console.log(roundedDownNumber) // Output: 3.98
You can round a float to 2 decimal places using JavaScript toFixed() or Math.round()
.
const number = 5.456; const roundedNumber = Math.round(number * 100) / 100; console.log(roundedNumber) // Output: 5.46
Math.ceil()
. Here’s an example:const number = 4.023; const roundedUpNumber = Math.ceil(number * 100) / 100; console.log(roundedUpNumber) // Output: 4.03
To truncate a decimal to two places without rounding, you can use Math.trunc()
. Here’s an example:
const number = 5.789; const truncatedNumber = Math.trunc(number * 100) / 100; console.log(truncatedNumber) // Output: 5.78
let percentage = 0.1234; // Method 1 let roundedPercentage = percentage.toFixed(2); console.log(roundedPercentage); // Output: "0.12" // Method 2 console.log(Math.round(percentage * 100) / 100); // Output: 0.12
Conclusion
Rounding numbers to two decimal places in JavaScript is a common task in many applications. We have explored various techniques, including using Math.round()
, toFixed()
, parseFloat()
, the BigRational
library, handling whole numbers, and converting the result back to a number.
With these methods in your toolkit, you’ll be able to handle rounding scenarios effectively and precisely.