If you are a web developer, you probably have used JavaScript substring and slice methods to manipulate strings. But do you know the difference between JavaScript substring vs slice methods? When to use each one?
In this blog post, I will explain:
- What are the
substring()
andslice()
methods? - What is substr() method?
- How do they assist you in extracting substrings?
- The distinctions between substring and slice in JavaScript
- Performance comparison of
slice vs. substring
.
Understanding substring() method
To extract a portion of a string, specified by a start and an end index, we use the JavaScript substring() method. Let’s see a few examples of using the substring()
method to extract parts of a string:
let greet = "Hello World";
console.log(greet.substring(0, 5));
// Output: "Hello"
console.log(greet.substring(6));
// Output: "World"
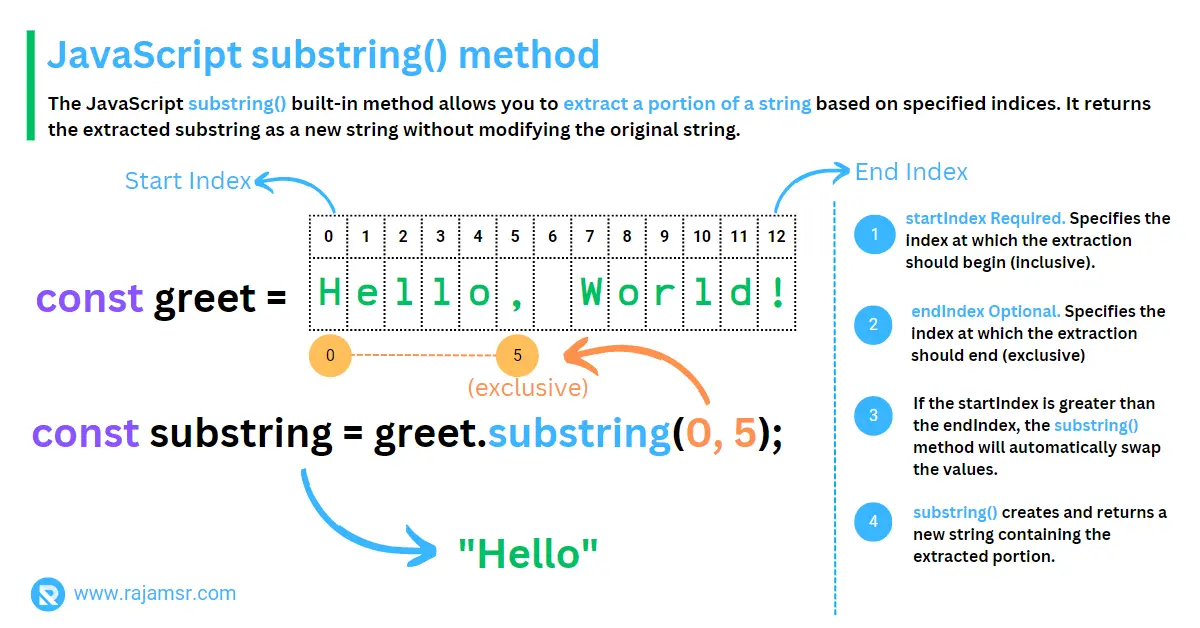
The substring method has some drawbacks, the most significant being its inability to handle negative indexes
. JavaScript treats a negative index as 0 when it is passed as an argument.
Understanding slice() method
To extract a portion of a string, specified by a start and an end index, we use the JavaScript slice() method. Let’s see a few examples of using the slice()
method:
let greet = "Hello World";
// Extract string starting from index 0 to 5
console.log(greet.slice(0, 5));
// Output: "Hello"
// Extract string starting from index 6 to end of string
console.log(greet.slice(6));
// Output: "World"
// Extract 5 characters from end (negative index) of the string
console.log(greet.slice(-5));
// Output: "World"
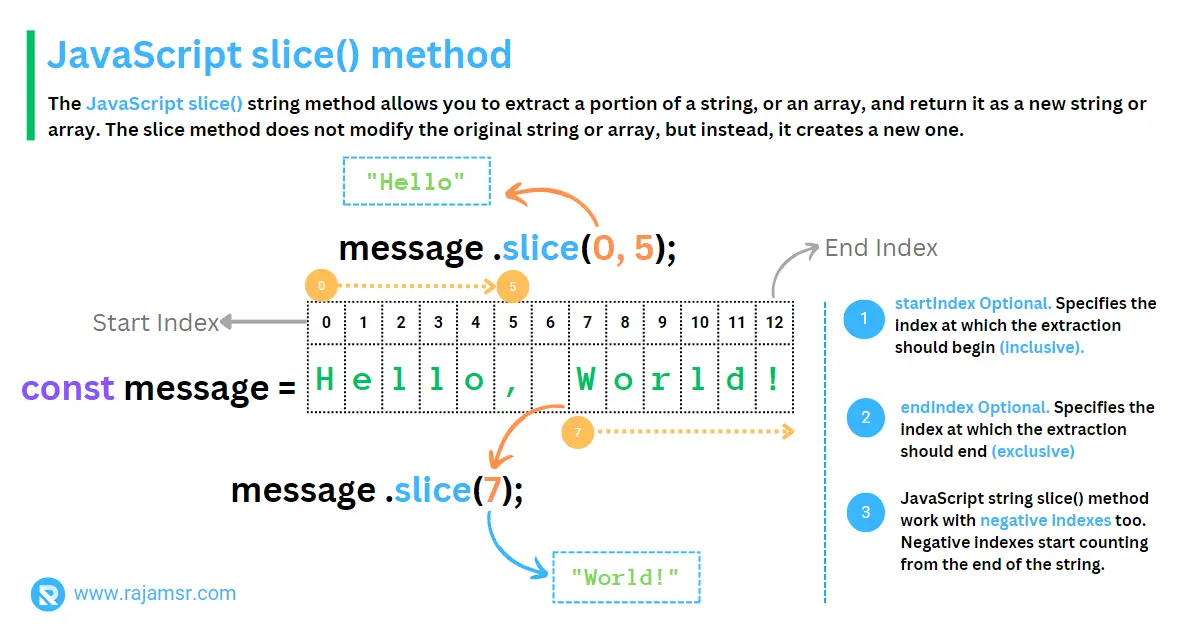
The slice()
method can handle negative numbers as well. A negative index is treated as an offset from the end of the string.
JavaScript substr() method
The JavaScript substr()
method is similar to the substring()
method, but it takes two parameters: the start index
and the length
of the substring.
Let’s see an example of how to use the substr() method.
let message = "JavaScript runs everywhere on everything!";
console.log(message.substr(0, 10)); // Output: "JavaScript"
console.log(message.substr(11, 15)); // Output: "runs everywhere"
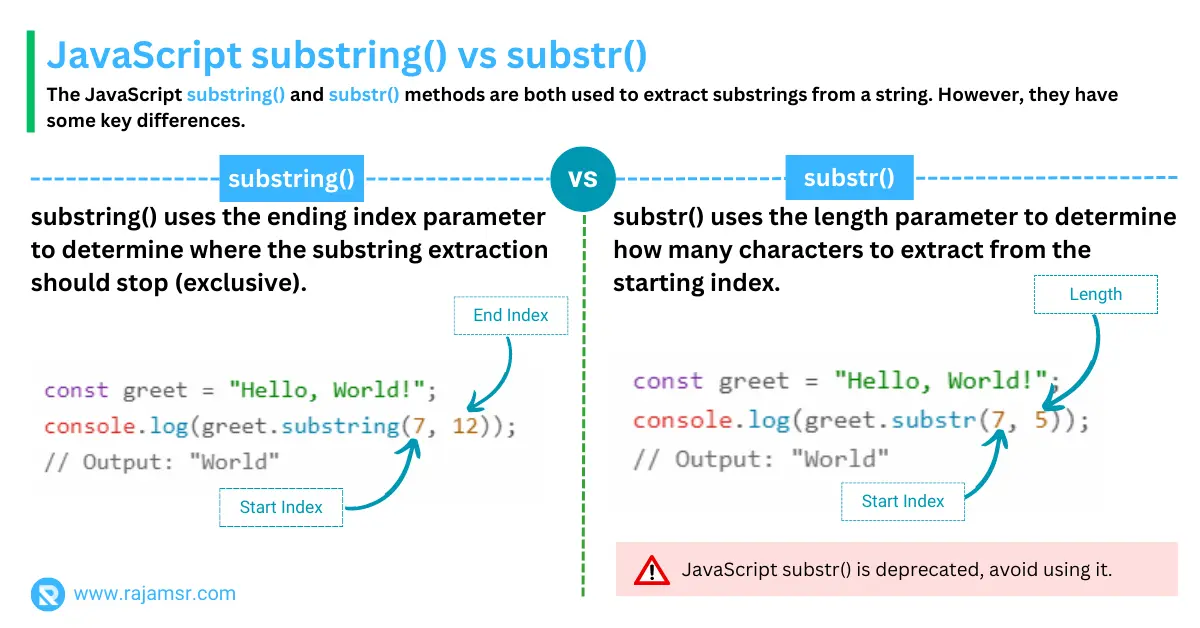
Difference between JavaScript substring vs slice methods
In many ways, the substring and slice string methods are similar, but there are some significant differences between substring()
and slice()
in JavaScript.
Here is difference between slice and substring in JavaScript:
JavaScript substring() | JavaScript slice() |
---|---|
The substring() method does not handle negative indexes. The system treats a negative index as zero. | The slice() method can handle negative indexes in parameters.For example, slice(-3) extracts the last three characters of the string. |
The The method does not modify the original string. | The JavaScript The method does not modify the original string. |
If startIndex is greater than endIndex , substring() swaps the arguments and proceed with the extraction. | If startIndex is greater than endIndex , slice() returns an empty string. |
We can use the JavaScript substring() method without specifying any index parameters. | We can use the JavaScript slice() method without any index parameters. |
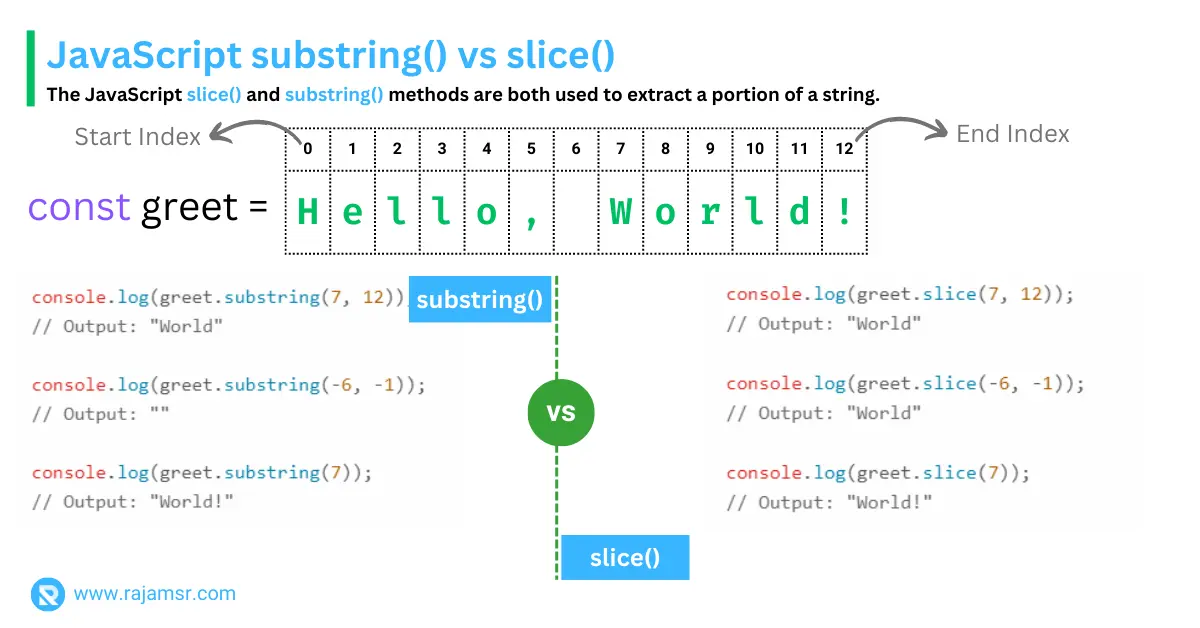
Overall, the choice between slice()
and substring()
depends on the specific requirements of your code. If you need to handle negative indices or prefer a more flexible behavior when the arguments are swapped, slice()
is a suitable choice.
You can use substring()
if you prefer automatic argument adjustment and don’t need negative indexing.
JavaScript slice vs substring performance comparison
Both substring() and slice() methods have a time complexity of O(n)
, where 'n'
is the length of the substring they extract. I performed benchmark testing for both substring() and slice() methods using the jsPerf online tool.
let greet = "Hello World";
console.log(greet.slice(0, 5))
console.log(greet.substring(0, 5))
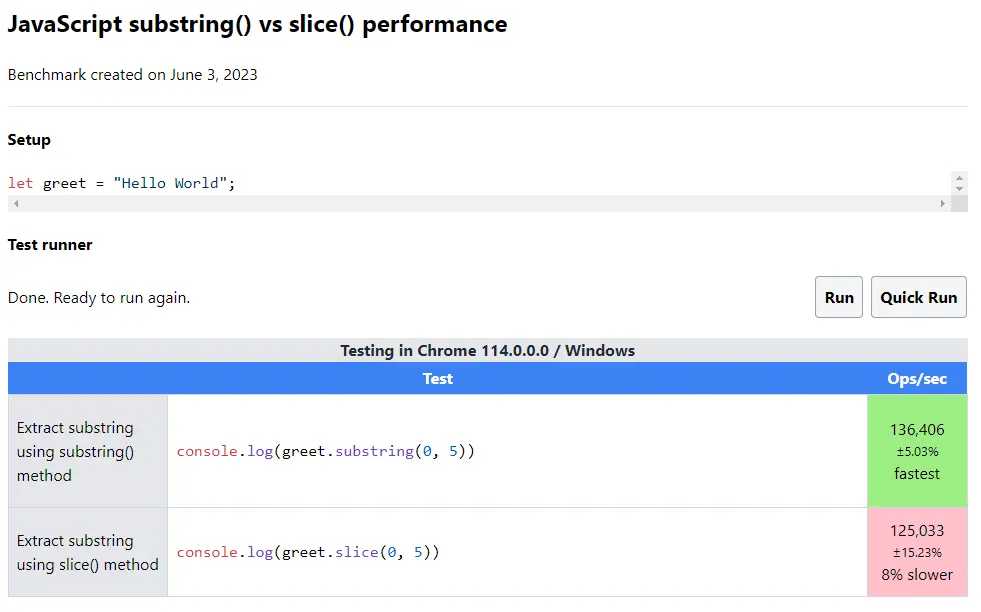
The slice() method is 8% slower than the substring() method, as shown in the above performance test snapshot.
We were able to perform 136,406 operations per second using substring(), but only 125,033 operations per second using the slice()
method.
However, you will notice performance issues only when doing substring extraction operations on large data sets.
Frequently asked questions
Use substring when you want to ensure that the provided indices are valid. Use slice when you want to handle negative indices or extract a substring up to the end of the string.
const message = "Hello, JavaScript"; const substringResult = message.substring(7, 17); // "JavaScript" const sliceResult = message.slice(7, -1); // "JavaScrip"
JavaScript slice()
and substring()
methods are used to extract substrings without modifying the original string. Whereas, splice()
is used to add or remove elements from an array. These methods have different purposes and behaviors.
const message = "Hello, World"; const numbers = [1, 2, 3, 4, 5]; const sliceResult = message.slice(0, 5); // "Hello" const spliceResult = numbers.splice(1, 2); // Removes [2, 3] and modifies 'numbers' const substringResult = message.substring(0, 5); // "Hello"
Conclusion
In conclusion, the JavaScript substring
and slice
methods are both useful for extracting a part of a string, but they have different rules for handling negative and out-of-range parameters.
The substr()
method is similar to the substring
method, but it takes the length of the substring instead of the end index. However, the substr()
method is not recommended to use, as it is a legacy feature and may be removed in the future. You should always use the slice()
or substring()
methods instead, as they are more consistent and reliable.
In general, slice()
is more flexible and easier to use, but substring()
is also a good option to extract substrings.
Over to you: which method will you use to extract the substring?