Have you ever wondered how to create a JavaScript multiline string? Do you want to write a string that has more than one line in JavaScript? It’s not as easy as you might think. JavaScript doesn’t like line breaks in strings. It thinks they are mistakes and gives you errors. So, how can you write a long and beautiful string without any problems? You need to use some special tricks to make it work.
In this blog post, I will show you:
- Different ways to create a multiline string in JavaScript
- How to use the new line character ( \n) for multiline strings in JavaScript
- How to write a multiline string without any newlines in JavaScript
- How to convert a multiline string into a single line in JavaScript
- How to escape the backtick (`) character in a multiline string in JavaScript
- How to remove the indentation from a multiline string in JavaScript
- How to fix the issue of new line not working in JavaScript
By the end of this blog post, you will be able to choose the best way to format multiline strings in your JavaScript code.
JavaScript multiline string literal
A string literal is a sequence of characters enclosed in quotes. You can use single quotes (‘), double quotes (“), or backticks (`) to create a string literal in JavaScript. For example:
let singleQuote = 'This is a string literal with single quotes';
let doubleQuote = "This is a string literal with double quotes";
let backtick = `This is a string literal with backticks`;
To create a multiline string in JavaScript, you can use a string literal with backticks (`). This is also known as a template literal. For example:
let multiline = `This is a multiline
string with backticks`;
The benefits of using a string literal with backticks are:
- You don’t need to escape special characters, such as quotes, newlines, tabs, etc. You can write them as they are in your code.
- You can use tagged templates, which allow you to customize the string output with a function.
- You can use template literals, which allow you to embed expressions and variables inside the string. For example:
let name = 'John';
let age = 25;
let message = `Hello, ${name}. You are ${age} years old.`;
The drawbacks of using a string literal with backticks are:
- They are not supported by older browsers or versions of JavaScript (ES5 or lower). You may need to use a transpiler or a polyfill to make them work.
- They may cause syntax errors or unexpected behavior if the string contains unescaped backticks or template literals.
JavaScript multiline string in different ways
There are several ways to break long JavaScript strings into multiline strings. Let’s explore five different methods for making a multiline string in JavaScript:
- Using new line characters
- Template literals
- The backslash character
- The string concatenation operator
- Using ASCII characters.
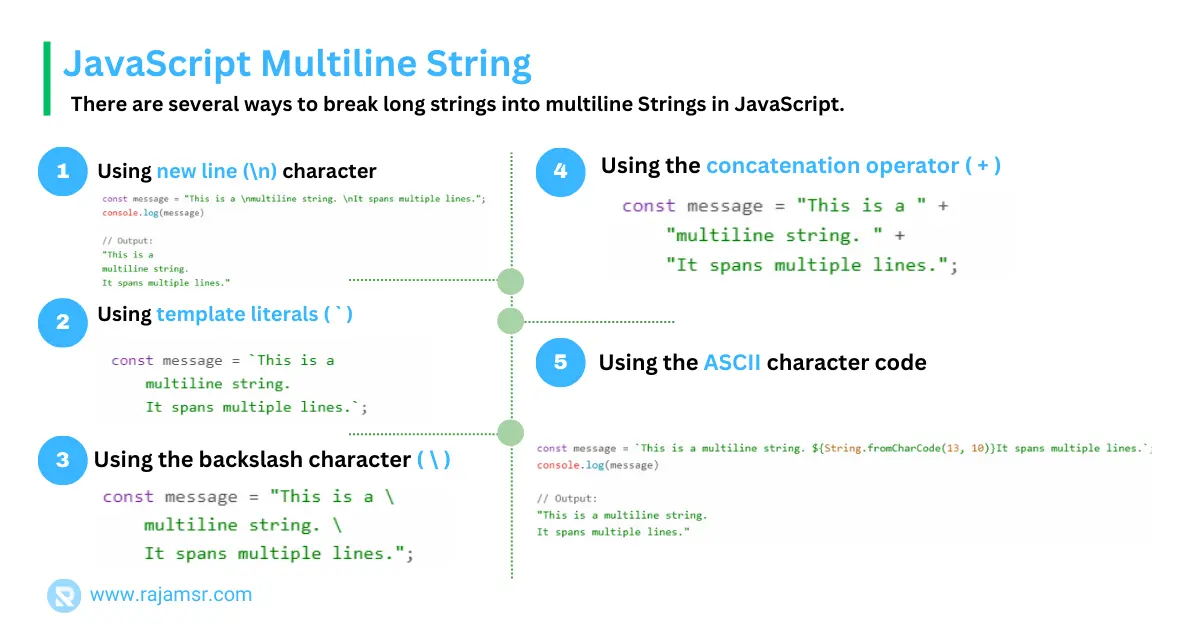
1. Using new line character ( \n) to create multiline string in JavaScript
Using the new line character \n
is the traditional way to create a new line in JavaScript. To create multi-line strings, you have to include newline characters as part of the string, which will display a multiline string.
Here is an example of creating a multiline string using the new line \n character:
const message = "This is a \nmultiline string. \nIt spans multiple lines.";
console.log(message)
// Output:
"This is a
multiline string.
It spans multiple lines."
If you want to show multiline text on an HTML page, you must use the line break <br>
tag instead of the new line \n character, as shown in the following example code:
const message = "This is a <br>multiline string. <br>JavaScript string multiple lines.";
document.getElementById('header').innerHTML = message
JavaScript multiline strings without newlines
In JavaScript multiline string without newlines characters is also possible using the following methods.
2. Using template literals ( ` ) to create multiline string
The template literals were introduced in ES6. The use of template string literals in JavaScript allows for the easy creation of multiline strings. They are enclosed in backticks ( ` ) instead of single quotes or double quotes. However, there is no difference between ‘ and ” in JavaScript string creation.
Here’s an example of creating multiline strings using a template literal:
const message = `This is a
multiline string.
It spans multiple lines.`;
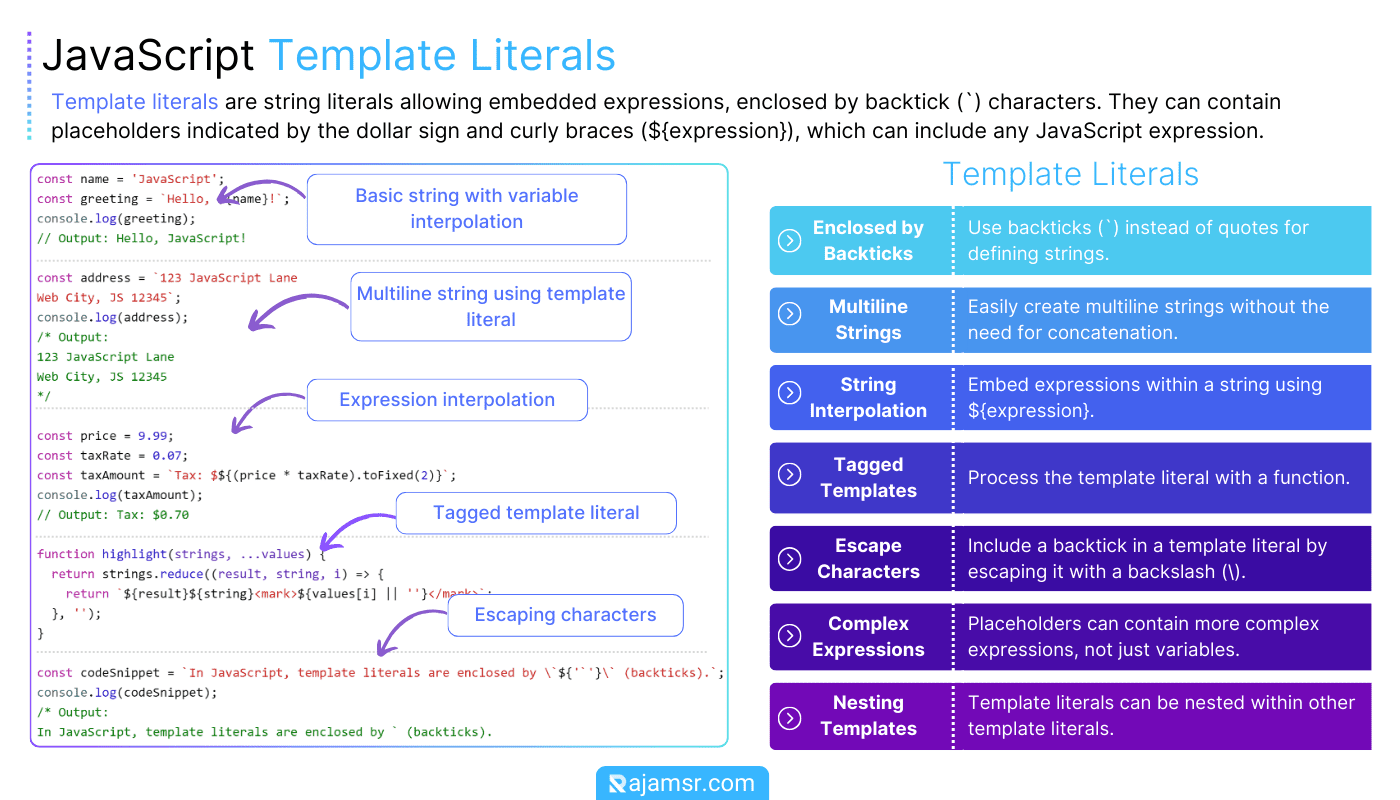
In the example above, we created a string that spans multiple lines using a template literal
. We used the backticks to enclose the string.
3. Using the backslash character ( \ )
Another way to create multiline strings in JavaScript is to use the backslash \ character. The backslash character is used to escape or ignore special characters in strings. The \ operator can be used to create multiline strings by adding a \ at the end of each line, which tells JavaScript to ignore the line break and continue the string on the next line.
Here’s an example of creating a multiline string using a backslash:
const message = "This is a \
multiline string. \
It spans multiple lines.";
This code will also run without any errors and will produce the same output as the template literal. However, there are also some drawbacks of using the \ operator to create multiline strings, such as:
- It is not very intuitive or clear to read or write
- It may cause confusion or errors if used incorrectly or inconsistently
- It does not support placeholders for variables or expressions
- It may not work in some environments or editors that do not support it
Therefore, the \ operator is also not the best option to create multiline strings in JavaScript, unless you have a specific reason to use it.
What if you want to include a backslash (\) in a multiline string using this method? You must take extra precautions by including an escape symbol (\\).
const message = "This is a \
\\multiline\\ string. \
It spans multiple lines.";
console.log(message)
// Output: "This is a \multiline\ string. It spans multiple lines."
In this way, you can assign JavaScript string variable value to multiple lines.
4. Using the concatenation operator
In JavaScript, the concatenation operator (+) can also be used to create multiline strings. We can split the string into several parts and then concatenate them.
Here’s an example of creating a multiline using the JavaScript string concatenation (+) operator:
const message = "This is a " +
"multiline string. " +
"It spans multiple lines.";
This code will also run without any errors and will produce the same output as the template literal. However, there are some drawbacks of using the + operator to create multiline strings, such as:
- Writing and reading it takes more time and effort
- Each line needs extra quotes and + signs to join them
- Variables or expressions cannot be inserted with placeholders
- It can slow down the performance if overused
Therefore, the + operator is not the best option to create multiline strings in JavaScript, unless you need to support very old browsers or environments that do not support template literals.
5. Using the ASCII character code
fromCharCode()
method converts numbers to an equal ASCII character. This function can accept multiple numbers, join all the characters then return the string. Here’s an example of creating a multiline string using ASCII characters:const message = `This is a multiline string. ${String.fromCharCode(13, 10)}It spans multiple lines.`;
console.log(message)
// Output:
"This is a multiline string.
It spans multiple lines."
In the above example, number 13 represents carriage return (\r) and 10 represents line feed (\n).
JavaScript multiline string with variables
What if you want to use a JavaScript variable in a multiline string? Using string interpolation you can create a JavaScript multiline string with variables as shown in the below example:
let newLine ='\n'
const message = `This is a multiline string. ${newLine}It spans multiple lines.`;
console.log(message)
// Output:
"This is a multiline string.
It spans multiple lines."
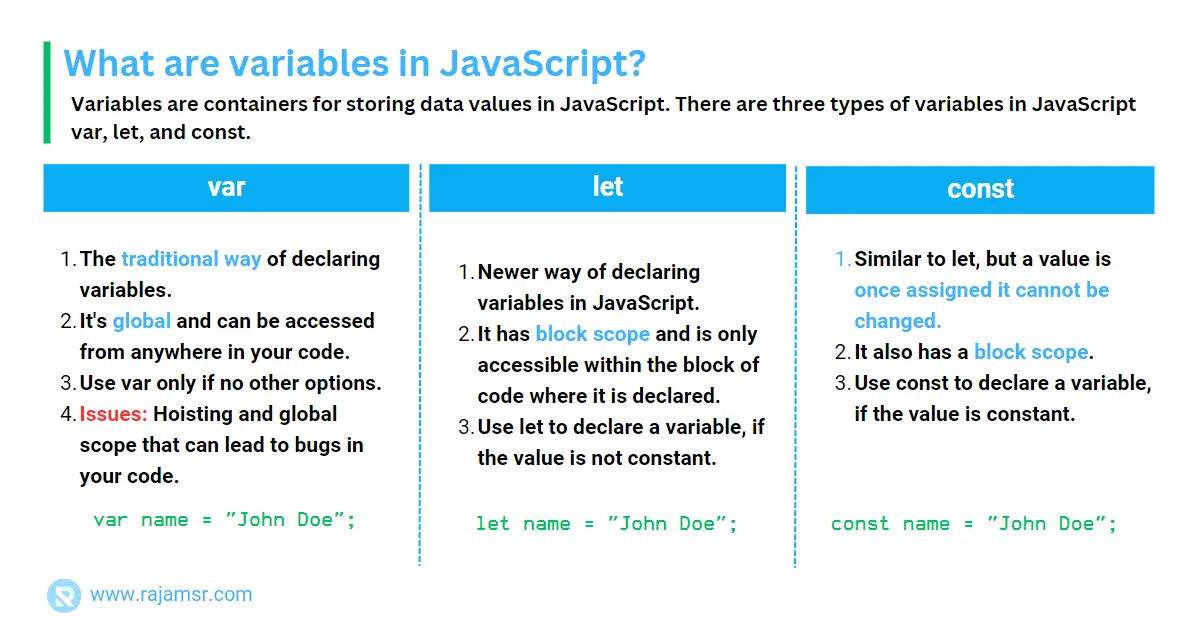
Converting multiline string to single line
You can create a multiline string using the methods mentioned above. You may need to remove a new line character from a string at times.
To remove new line characters, use the following code:
const message = "This is a \n multiline string. \n"+
"It spans multiple lines.";
let singleLine = message.replaceAll('\n','')
console.log(singleLine)
// Output: "This is a multiline string. It spans multiple lines."
You can easily remove all new line characters from a string using the JavaScript string replaceAll()
method.
If the new line is at the beginning or end of the string, merely use the JavaScript trim() method to remove all whitespace characters.
JavaScript multiline string escape backtick (`)
Backticks allow for easier multiline string creation, interpolation of variables, and the avoidance of escaping characters within the string. To escape a literal backtick within such a string, you can use a backslash (\) before it.
Here’s how to use it:
// multiline string backtick escaped
const escapedBacktickString = `
This is a multiline string
using backticks (\`) in JavaScript.
It can span across multiple lines.
`;
console.log(escapedBacktickString);
JavaScript multiline string without indentation
While template literals (template strings) offer an easy solution, they can result in unintended indentation. Let’s see how to create clean multiline strings without indentation.
Remove indentation using regex
To eliminate indentation, you can use regular expressions:
function stripIndent(strings, ...values) {
const result = strings
.map((str, i) => str.replace(/^\s+/gm, '') + (values[i] || ''))
.join('');
return result.trim();
}
const content = stripIndent`
This is a multiline
string without indentation.
`;
console.log(content);
// Output:
This is a multiline
string without indentation.
In this approach, the stripIndent()
function removes leading spaces on each line, creating a clean multiple line string.
Remove indentation from multi line string using array Join() method
By creating an array of lines and joining them with newline characters, indentation is avoided.
The code creates a multiple line string with an indentation called content
and then removes the indentation by breaking the string into lines, trimming each line, and concatenating them. The final content is printed to the console without leading whitespace.
const content = `
This is a multiline
string without indentation.
`;
let indentRemovedContent = content.split('\n')
.map(s => s.trim())
.join('\n');
console.log(indentRemovedContent);
// Output:
This is a multiline
string without indentation.
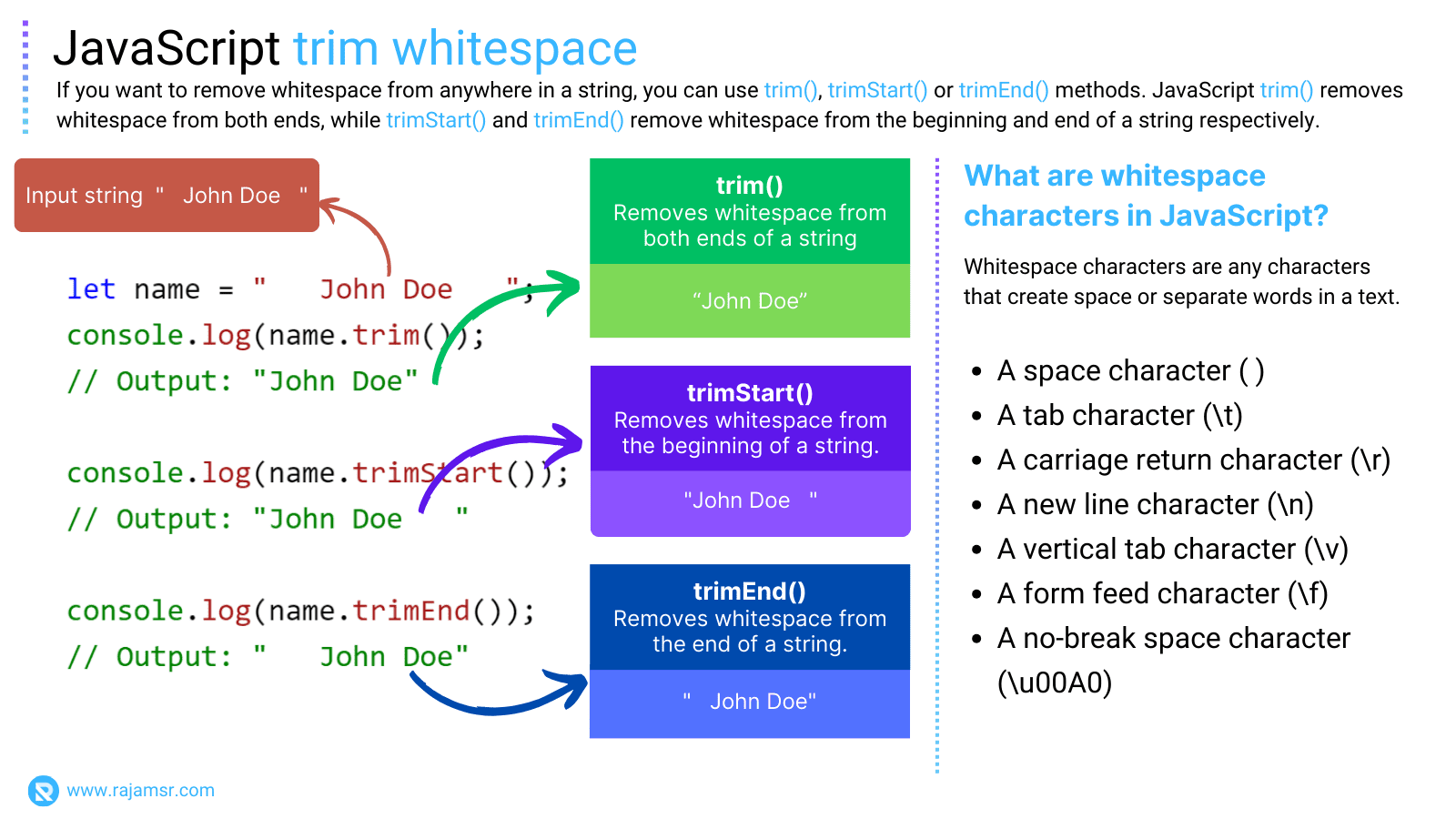
JavaScript new line not working
In JavaScript, you can use the methods described above to insert a new line. However, if you are manipulating text for an HTML page, it will not work.
If you are going to display content on an HTML page, you must use the line break tag (<br>) as shown in the following example:
document.getElementById('header').innerHTML = 'This is multiline <br> String'
Frequently asked questions
You can use the replace() method with a regular expression to escape quotes.
const multilineString = `This is a multiline string with 'single' and "double" quotes`; const escapedQuotesHandled = multilineString.replace(/\\(['"])/g, '$1'); console.log(escapedQuotesHandled); // Output: This is a multiline string // with 'single' and "double" quotes
const multilineStringWithTabs = ` Tabbed line 1 Tabbed line 2 Tabbed line 3`; const tabsRemoved = multilineStringWithTabs.replace(/^\s+/gm, ''); console.log(tabsRemoved);
const person = { address: `910 Main St Westbrook, Massachusetts Zipcode-04092` }; console.log(person.address);
Conclusion
In conclusion, multiline strings in JavaScript are a handy way to write code that is more readable and easy to maintain. We discussed five different techniques to create strings that span multiple lines in JavaScript:
- Using the new line character (\n) to insert line breaks.
- Using template literals (` `) to write strings that preserve the formatting of the source code
- Using the backslash character (\) at the end of each line to escape the new line character.
- Using the concatenation operator (+) to join strings that are on separate lines.
- Using ASCII characters (\r\n) to represent carriage return and line feed
I hope this blog post has helped you understand how to create multiline strings in JavaScript and how to choose the best method for your needs.