Are you looking for ways to add elements to the beginning of the array? Look no further than the JavaScript unshift() method! This built-in function is a must-know for manipulating arrays. With JavaScript unshift()
, you can easily add elements to the beginning of an array.
In this blog post, we will look at the following:
- The basic syntax and usage of the unshift() method
- The concept of array-like objects and how to use unshift() with them
- The behavior and return value of the unshift() method
- The performance comparison of the unshift() method
- Compare
shift()
andunshift()
methods - Alternative methods to unshift()
Let us see this in detail.
JavaScript unshift() method syntax and usage
unshift()
method is as follows:array.unshift(element1, element2, ..., elementN)
Do you want to add new elements to the start of an array? Use the unshift() JavaScript array method! It takes any number of elements and puts them at the beginning. The elements keep their order. The first element becomes the first in the array, the second element becomes the second, and so on.
The unshift()
method changes the array and gives you the new JavaScript array length. Here is an example:
let array = ['Yes', 'No'];
array.unshift('Select');
console.log(array);
// Output: ["Select", "Yes", "No"]
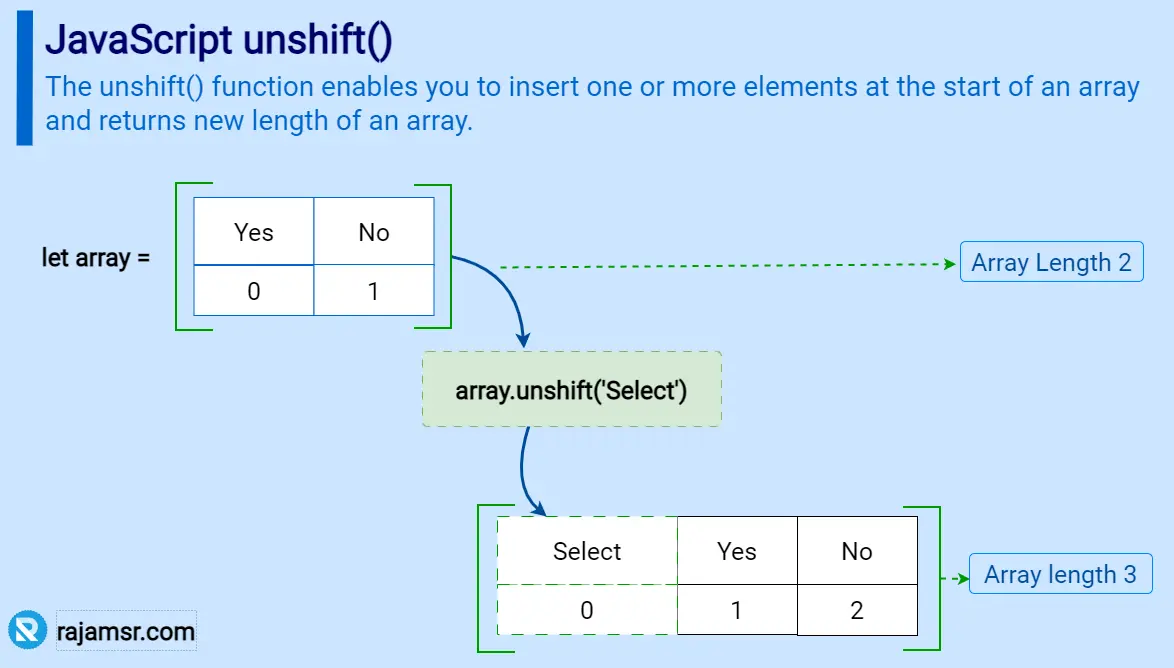
Array-like objects
unshift()
method with them, by using the call()
or apply()
method of the Function object. Here is how:function showArguments() {
console.log(arguments);
// Output: {0: "arg1", 1: "arg2", 2: "arg3"}
Array.prototype.unshift.call(arguments, "x", "y");
console.log(arguments);
// Output: {0: "x", 1: "y", 2: "arg1", 3: "arg2", 4: "arg3"}
}
The unshift() JavaScript method behavior and return value
unshift()
method.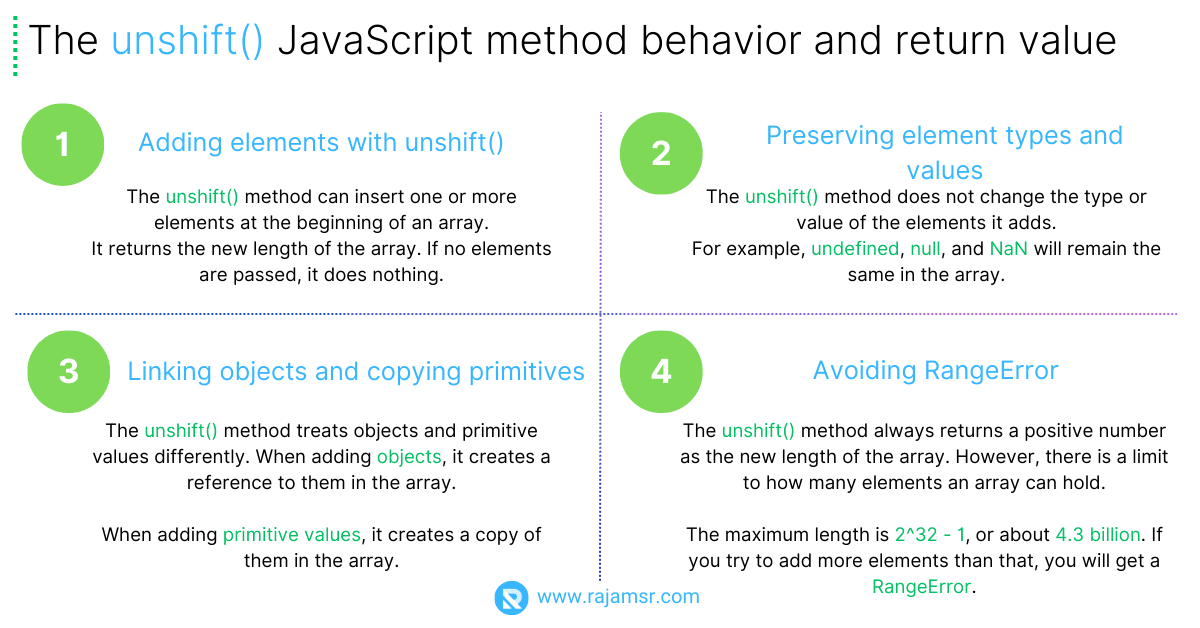
Add to the beginning of array using .unshift() JavaScript method
1. Simple examples of using unshift() array elements
Here’s a simple example of using the array unshift()
JavaScript method to add an element to the beginning of an array:
let array = [1, 2, 3];
array.unshift(0);
console.log(array);
// Output: [0, 1, 2, 3]
2. Adding multiple elements using array.unshift() JavaScript method
You can also add multiple elements
to the beginning of an array using the unshift method. The unshift()
function accepts any number of elements separated by commas. To insert each element as a new element at the beginning of the array, use the unshift method. This method adds one or more elements to the start of the array and returns the new length of the array.
Here’s an example of adding multiple elements:
let array = [1, 2, 3];
array.unshift(-2, -1, 0);
console.log(array);
// Output: [-2, -1, 0, 1, 2, 3]
3. Comparison of unshift() with push() method
It’s worth noting that the array unshift()
in JavaScript is the opposite of the push()
method. The push()
method inserts elements at the end of an array, whereas the unshift()
method inserts elements at the beginning.
Here’s an example of JavaScript unshift() vs. push()
:
let array = [1, 2, 3];
array.unshift(0);
array.push(4);
console.log(array);
// Output: [0, 1, 2, 3, 4]
Comparison of shift() and unshift() in JavaScript
The shift()
and unshift()
are two important JavaScript array methods in JavaScript that allow you to manipulate arrays.
let array = [1, 2, 3];
let shiftedElement = array.shift();
console.log(shiftedElement);
// Output: 1
console.log(array);
// Output: [2, 3]
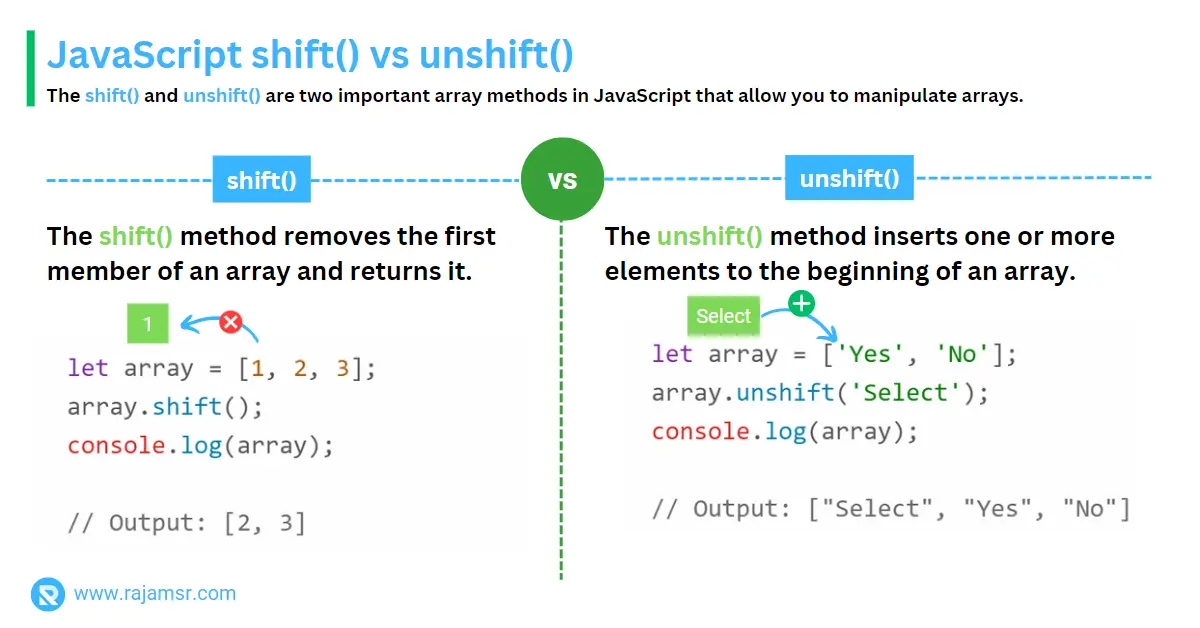
JavaScript array.unshift() method performance comparison
unshift()
method is a convenient and easy way to add elements to the beginning of an array, but it comes with a cost. In terms of time complexity, the unshift()
method has a linear time complexity of O(n)
.Here’s the reasoning behind this time complexity:- To make space for the new element, all the existing elements must shift to the right when
unshift()
adds an element to the beginning of the array. - Shifting the elements requires iterating through the existing elements and moving them in one position to the right. Perform this operation for each element in the array.
- As the number of elements in the array increases, the time required to shift all the elements to the right also increases linearly. If there are
'n'
elements in the array, the unshift() method will take approximately O(n) time to complete.
const numbers = [1, 2, 3, 4, 5];
numbers.unshift(0);
console.log(numbers)
// [0, 1, 2, 3, 4, 5]
In this example, the unshift()
method adds the element 0 at the beginning of the array. To do this, we shift all the existing elements [1, 2, 3, 4, 5]
in the array one position to the right, and we get the updated array [0, 1, 2, 3, 4, 5]
.
As you can see, the time required to operate increases linearly with the size of the array. Hence, the time complexity of the unshift()
method is O(n)
.
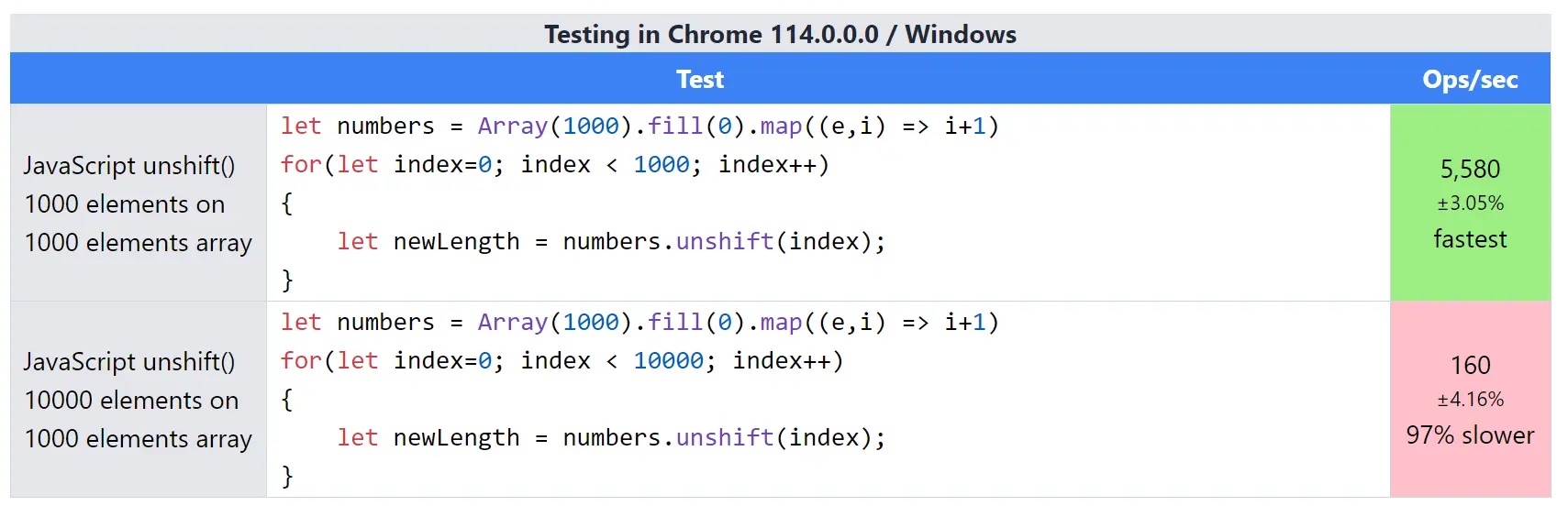
In comparison to unshifting 1000
elements on 1000
elements array, unshifting 10,000
elements on 1000
elements is 97% slower
.
JavaScript unshift() alternative methods
unshift()
alternative methods:Alternate 1: Using JavaScript spread operator (...)
For example, you can make a new array by concatenating the new elements with the old array using the spread operator
.
let numbers = [1, 2];
let newNumbers = [...numbers, 3, 4, 5];
// The original array is not changed
console.log(numbers); // Output: [1, 2]
console.log(newNumbers); // Output: [1, 2, 3, 4, 5]
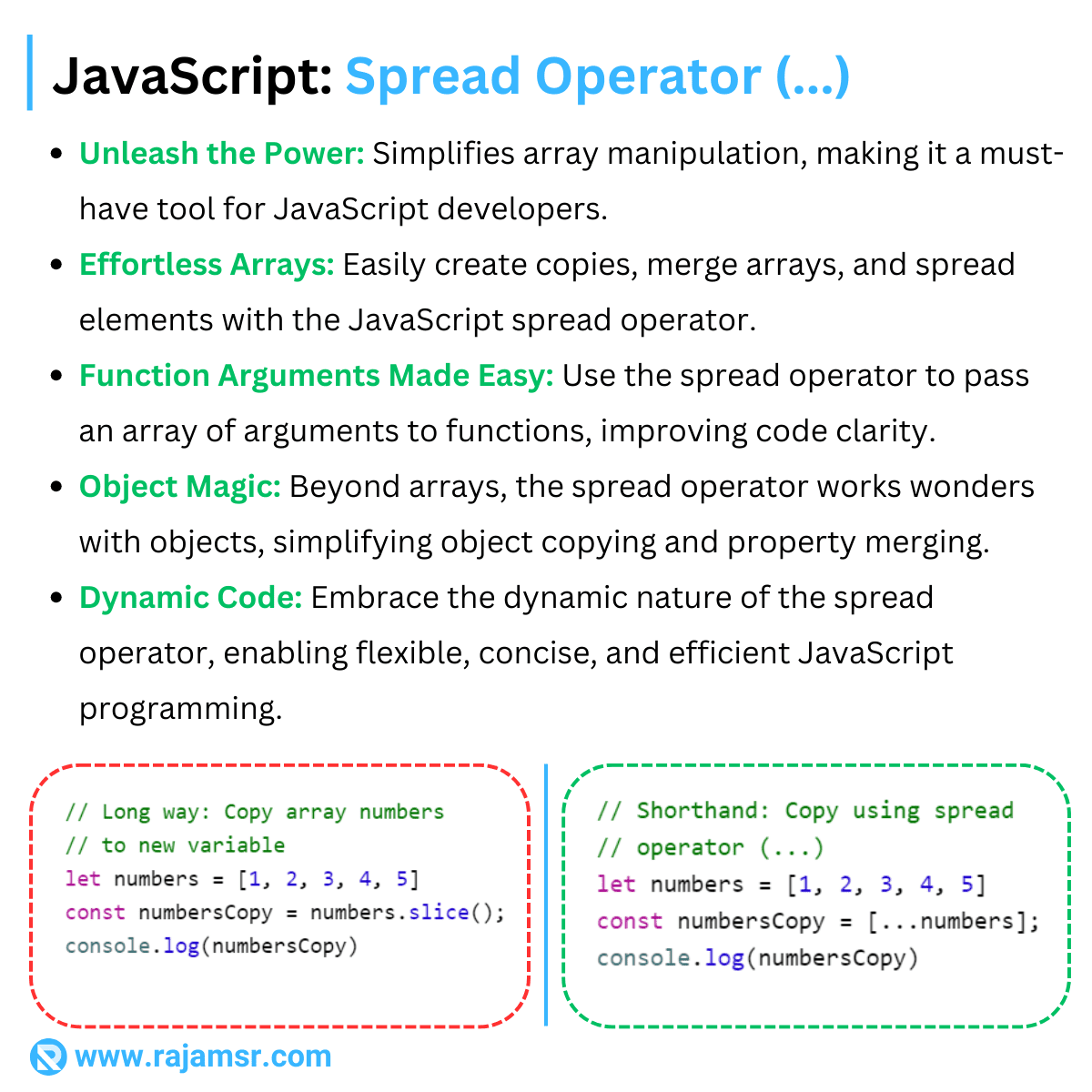
A new array, newNumbers
, is created by spreading the elements of the original array numbers and adding 3, 4,
and 5
. The original array remains unchanged.
Alternate 2: Using concat() method
You can add elements to the start of an array without changing the original array. Use the concat()
array method. This method makes a new array. The JavaScript concat()
joins two or more arrays.
Look at an example to see how it works:
let numbers = [3, 4, 5];
// New array with elements added to the beginning
let newNumbers = [1, 2].concat(numbers);
// The original array is not changed
console.log(numbers); // Output: [3, 4, 5]
console.log(newNumbers); // Output: [1, 2, 3, 4, 5]
Unshift() in JavaScript - Frequently asked questions
What is unshift() in JavaScript?
unshift()
is a JavaScript array method that adds one or more elements to the beginning of an array, effectively modifying the original array. It shifts the existing elements to higher indexes and increases the length of the array.const array = [2, 3, 4]; array.unshift(1); // Result: [1, 2, 3, 4]
What does unshift() do in JavaScript?
unshift()
in JavaScript is used to prepend one or more elements to the beginning of an array. It modifies the original array, shifting existing elements to higher indexes and increasing the array length.const htmlTags = ['head', 'title']; htmlTags.unshift('meta', 'body'); console.log(htmlTags) // Output: ["meta", "body", "head", "title"]
How to use unshift() in JavaScript without modifying the array?
If you want to add elements to the beginning of an array without modifying the original array, you can use the concat() method to create a new array with the added elements.
const originalArray = [2, 3, 4]; const newArray = [1].concat(originalArray); // newArray: [1, 2, 3, 4] // Original array remains unchanged: [2, 3, 4]
Why can't I unshift() and pop() at the same time in JavaScript?
You can’t unshift and pop at the same time on the same array because these operations work on opposite ends of the array. The unshift()
add elements to the beginning while pop()
removing elements from the end. Attempting both operations simultaneously would have unpredictable and undesired effects.
const numbers = [1, 2, 3]; numbers.unshift(0); // Adds 0 to the beginning: [0, 1, 2, 3] numbers.pop(); // Removes 3 from the end: [0, 1, 2] // Attempting to unshift and pop simultaneously may lead to unexpected results.
Conclusion
unshift()
method to add elements to the beginning of an array. However, it is not without drawbacks, and it is critical to comprehend the unshift()
method’s performance and memory constraints.If you are dealing with a large array, you can try with an alternate method involving the spread operator
or using concat()
method.So the next time you find yourself needing to add new elements to the beginning of an array, remember to use the unshift()
method!