If you want to learn how to clear a JavaScript array, you’ve come to the right place! In this article, I will show you three different ways to clear an array using the splice()
, length property
, and pop()
methods. Arrays are an important data structure in JavaScript that allows you to store and manipulate collections of data. However, at times, it may be necessary to clear the contents of an array in order to optimize memory usage or prepare the array for new data.
In this blog post, we will explore:
- Different ways to clear arrays in JavaScript
- How to clear array entries
- JavaScript clears arrays after loops
- Create empty arrays of a certain size
- JavaScript clear const array
- Clear multiple specific elements from arrays
Let’s dive in.
JavaScript clear array
Arrays do not have an array.clear() method. However, there are multiple ways to clear an array. In this article, I will show you how to clear an array or reset an array in three ways:
- Using the length property of an array
- Clear array using splice() method
- Remove array elements using the pop() method
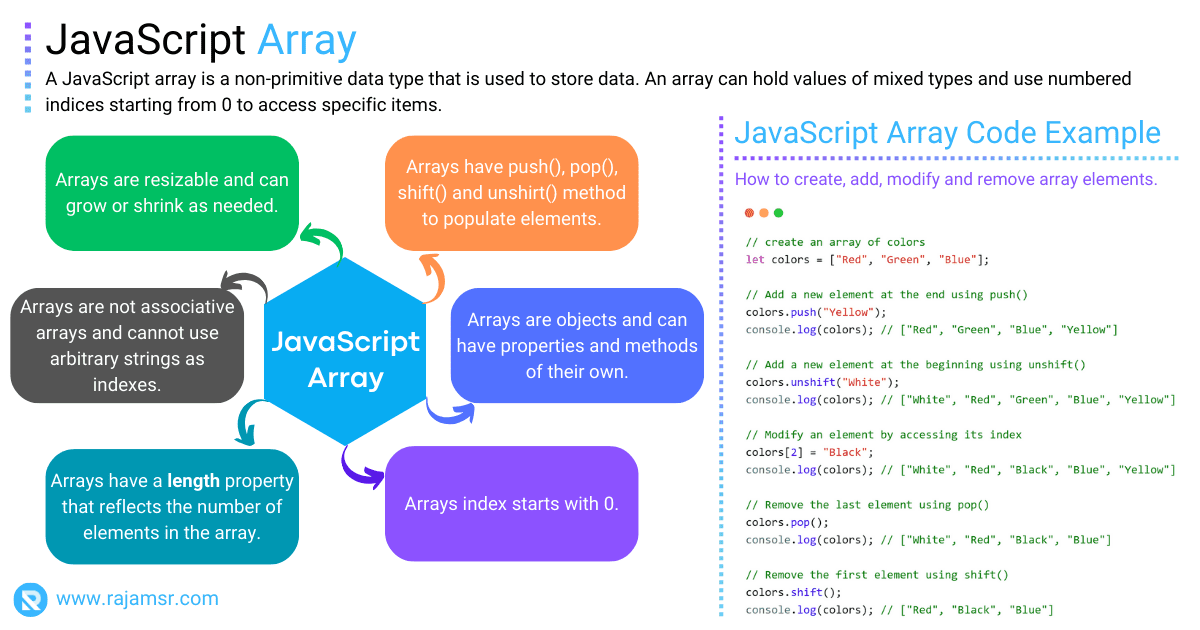
Method 1. How to clear an array in JavaScript using length property
The array is one of the non-primitive data types in JavaScript. The easiest way to clear an array in JavaScript is to set the length of the array to 0. This will remove all the elements in the array and set its length to 0, effectively clearing it.
Let’s take a look at how to clear an array with an example:
let numbers = [1, 3, 5, 7];
console.log(numbers);
// Output: [1, 2, 3, 4]
numbers.length = 0;
console.log(numbers);
// Output: []
In this example, we first create an array called numbers with a few numbers in it. We then log the original array to the console to confirm its contents. We then set the JavaScript array length property to 0 to reset the array, which clears all the elements in the array. Finally, we log the array to the console again to confirm that it has been cleared.
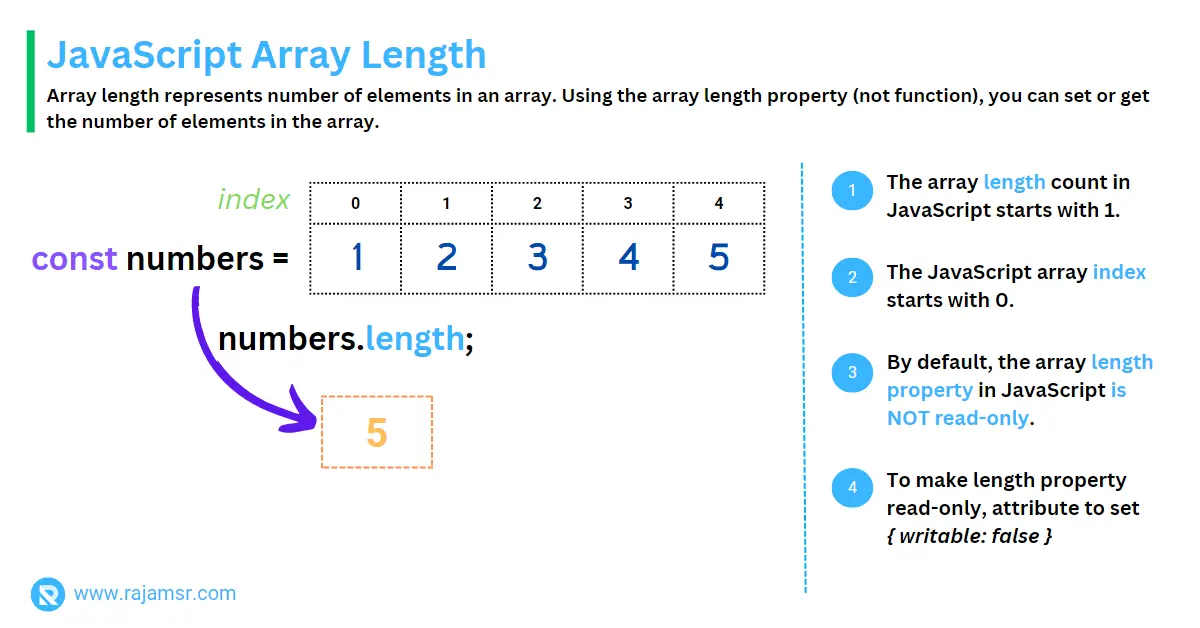
Method 2. JavaScript array clear using splice() method
Using the array splice()
method, you can remove array elements. It accepts the starting index and the number of elements to be removed as parameters. We want to clean the entire array, so we can specify 0 as the start index. Using the JavaScript indexof() method, you can search an array of elements.
The array length property returns the number of elements in an array. We can pass this as a second parameter. However, the second parameter is optional.
let numbers = [1, 3, 5, 7];
console.log(numbers);
// Output: [1, 2, 3, 4]
numbers.splice(0, numbers.length)
console.log(numbers);
// Output: []
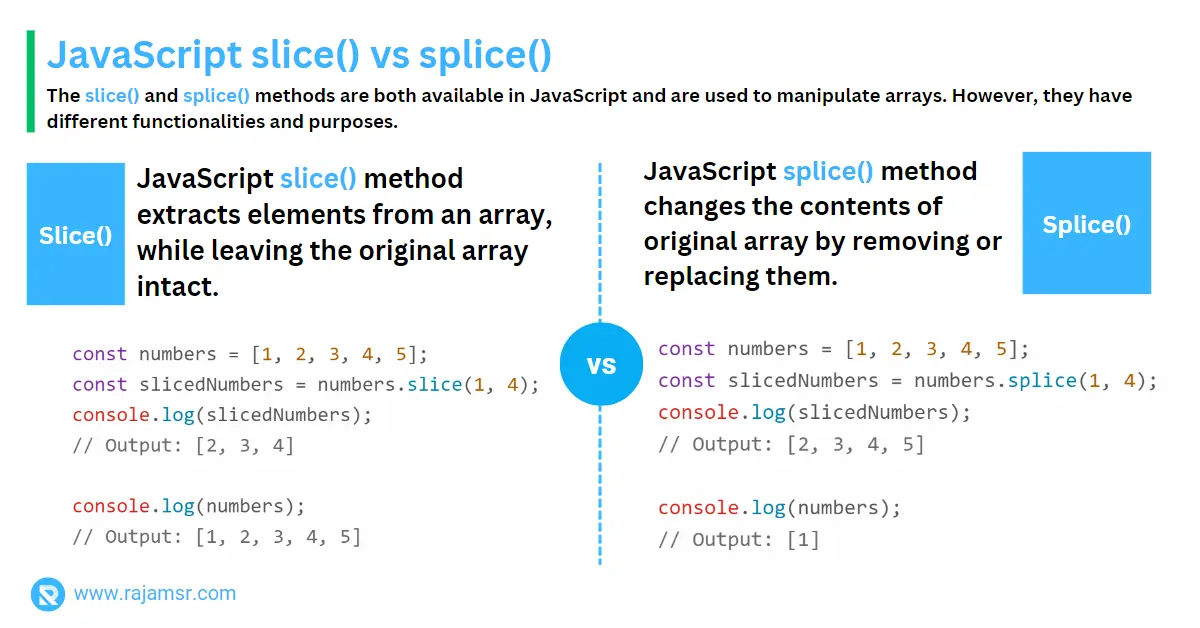
Method 3. Clear array in JavaScript using pop() method
The array pop()
method will remove an element from the end of the array. Using a for loop, we can iterate through an array from end to end by reducing the index value.
Here is an example of a clear JavaScript array using the pop()
method:
let numbers = [1, 3, 5, 7];
console.log(numbers);
// Output: [1, 2, 3, 4]
for (let index = numbers.length; index>= 0; index--) {
// pop method removes the last element from an array
numbers.pop(index);
}
console.log(numbers);
// Output: []
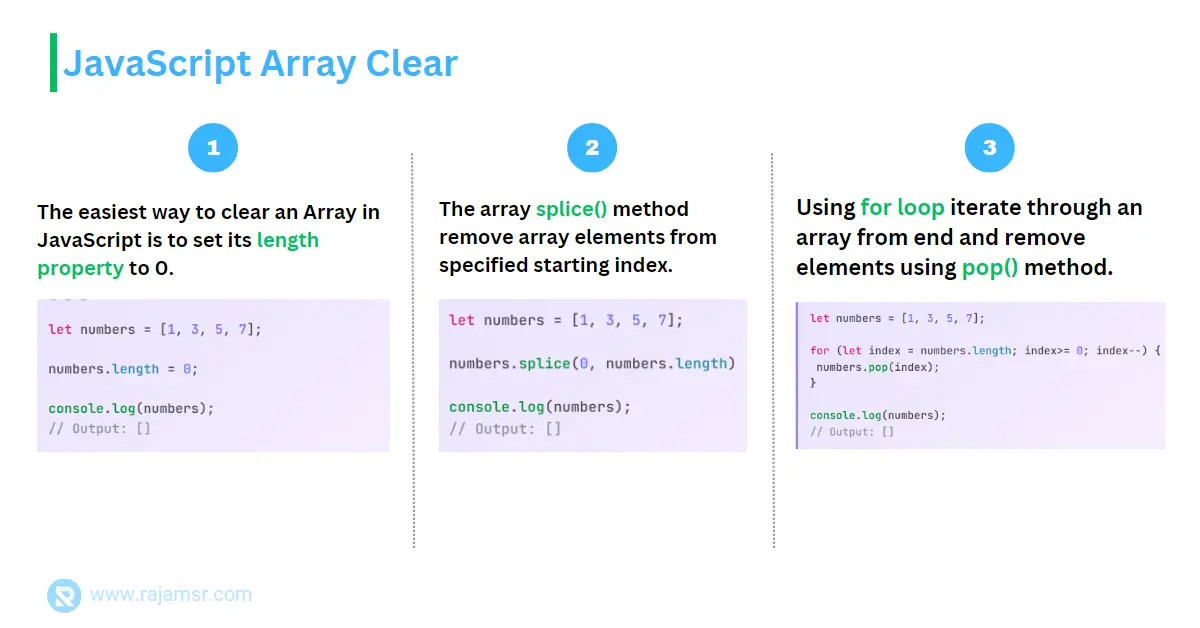
How do you clear an array entry?
Sometimes, you may want to clear a specific element in an array rather than clearing the entire array. To do this, you can simply assign undefined to the element’s index.
Let’s take a look at an example:
let numbers = [1, 3, 5, 7];
console.log(numbers);
// Output: [1, 2, 3, 4]
const numberToRemove = 5
numbers = numbers.filter(e => e !== numberToRemove)
console.log(numbers);
// Output: [1, 3, 7]
In this example, we first create an array called numbers with some elements. We then log the numbers, which are references to the original array, to the console to confirm its contents.
Let’s assume we have to remove the value 5 from the number array. Using the filter()
JavaScript array method, we can filter all elements other than 5 and then assign the result to the same variable numbers to override the initial value. For this to work, the array should be declared using either var or let. It will throw an error if the array is declared with a const.
Finally, we log the array to the console again to confirm that the element has been cleared.
JavaScript array clear after loop
Sometimes, you may want to clear an array after looping through it in order to free up memory or prepare the array for new data. To do this, you can simply set the array’s length property to 0 after the loop has been completed.
Let’s take a look at the JavaScript clear array after the loop:
let numbers = [1, 3, 5, 7];
for (let index = 0; index < numbers.length; index++) {
console.log(numbers[index]);
}
numbers.length = 0;
console.log(numbers);
// Output: []
In this example, we first create an array called a number with a few numbers. We then log the original array to the console to confirm its contents. We then loop through the array using a for loop and log each element to the console. After the loop has been completed, we set the array’s length property to 0, effectively clearing it. Finally, we log the array to the console again to confirm that it has been cleared.
Instead of removing elements after looping through them, if you want to remove them immediately after accessing the elements, you can use the while loop and array pop()
methods, as shown in the following example:
let numbers = [1, 3, 5, 7];
while (numbers.length > 0) {
let num = numbers.pop()
// Process num value
}
console.log(numbers);
// Output: []
Creating an empty array of JavaScript with size
Creating an empty array
You can create an empty array like this with empty square bracket.
// Creating an empty array using literal notation
let numbers = [];
// Creating an empty array using Array() object
let numbers = new Array();
Creating an empty array with specific size
Sometimes, you may want to create an empty array object of a certain size in JavaScript. To do this, you can use the Array()
constructor and pass in the desired size of the array as an argument. Let’s take a look at an example:
const numbers = Array(5);
console.log(numbers);
// Output: [undefined, undefined, undefined, undefined, undefined]
console.log(numbers.length);
// Output: 5
In this example, we use the Array()
constructor to create an array with a size of 5. Since we did not pass any values to the constructor, each element in the array is initialized as undefined.
Clear const array in JavaScript
In JavaScript, you can declare arrays using the const keyword, which makes the variable immutable. However, this does not mean that the contents of the array are immutable. You can still clear the contents of a const array by setting its length property to 0.
Let’s take a look at an example of how to clear a const array in JavaScript:
const numbers = [1, 3, 5, 7];
console.log(numbers);
// Output: [1, 3, 5, 7]
numbers.length = 0;
console.log(numbers);
// Output: []
"Cannot assign to the number because it is a constant."
const numbers = [1, 3, 5, 7];
numbers = [2, 4, 6, 8]
// Cannot assign to "numbers" because it is a constant
If you find that you need to modify an array frequently, you should consider using a variable declared with let
instead of a const
(constant) to store the array.
Re-assigning value to a const variable defeats the purpose of a constant.
Clear multiple specific elements
There are multiple ways to clear specific elements in a JavaScript array. However, we will look into two different approaches:
Clear multiple elements using splice() method
If you need to clear multiple specific elements from an array in JavaScript, you can use the splice()
method. The splice()
method allows you to remove elements from an array by specifying the starting index and the number of elements to remove. Let’s take a look at an example:
const numbers = [1, 3, 5, 7, 9];
console.log(numbers);
// Output: [1, 3, 5, 7, 9]
numbers.splice(1, 2);
console.log(numbers);
// Output: [1, 7, 9]
In this example, we create an array called numbers with some elements. We then use the splice()
method to remove two elements starting from index 1, effectively clearing elements at indexes 1 and 2. Finally, we log the modified array to the console to confirm that the specific elements have been cleared.
Clear multiple elements using filter() method
Let’s assume you have a book array and you want to clear books whose price is less than 30. Filter all the books whose prices are greater than 30 using the array filter() method. Normally, the filter()
method returns a new array, which means the original array will remain unchanged.
Instead of assigning it to a new JavaScript variable, you can reassign it to the same books variable, which will override the existing array by setting filtered values.
let books = [
{ title: "A Modern Introduction to Programming", price: 26.99 },
{ title: "JavaScript: The Definitive Guide", price: 37.99 },
{ title: "You Don't Know JS Yet: Get Started", price: 19.99 },
{ title: "Learning JavaScript Design Patterns", price: 28.99 },
{ title: "Secrets of the JavaScript Ninja", price: 34.99 }
];
books = books.filter(b => b.price > 30)
console.log(books)
// Output: [ { title: "JavaScript: The Definitive Guide", price: 37.99 },
// { title: "Secrets of the JavaScript Ninja", price: 34.99 }]
For this solution to work, the array should be initially declared with the let
or var
keyword. If the same books variable is not used, the original array will remain the same.
Conclusion
In this blog post, we have explored different ways to clear arrays in JavaScript, including how to clear array entries, clear arrays after loops, create empty arrays of a certain size, clear const arrays, and clear multiple specific elements from arrays. By knowing how to effectively clear arrays, you can optimize memory usage and ensure that code runs efficiently.