Do you know how to get the JavaScript object length? It’s not as simple as using the length property, because objects don’t have one by default.
Object length represents the number of properties or keys in an object. To get the object length or size, you need to use a loop or a JavaScript built-in static method like Object.keys() or Object.values() to count the number of properties or values in an object.
In this blog post, we will explore:
- The length property of JavaScript objects
- The number of properties
- How to check for empty objects
- Objects with the size of 0
- Length limits
- Handling object length undefined error
What is JavaScript Object?
Before checking the object length, let’s see what an Object
in JavaScript is. In JavaScript, an object is a collection of key-value
pairs, where the keys are strings. The values can be any valid JavaScript data type, including other objects. Objects
are one of the non-primitive data types in JavaScipt.
In JavaScript, you can create an object using object literal notation by enclosing the key-value
pairs in curly braces, as shown in the following example:
const book = {
title: "JavaScript for Beginners",
price: 19.99,
isbn: "978-0-123456-78-9"
};
In the above book
object, title
, price
, and ISBN
represent the JavaScript object keys or properties. "JavaScript for Beginner"
, 19.99
and 978-0-123456-78-9
represent its corresponding values.
Check object length in JavaScript or number of properties
The JavaScript length of an object represents the number of properties or keys
in the object. Here is an example of how to check object length in JavaScript:
const book = {
title: "JavaScript for Beginners",
price: 19.99,
isbn: "978-0-123456-78-9"
};
console.log(Object.keys(book).length)
// Output: 3
In the code above, we created an object book
with three properties, title
, price
, and ISBN number
. We then used the Object.keys()
static method to get the object length as an array of the property names. Then we used the JavaScript array length property to get the number of keys or properties in the object. The length of the object in this case is 3
.
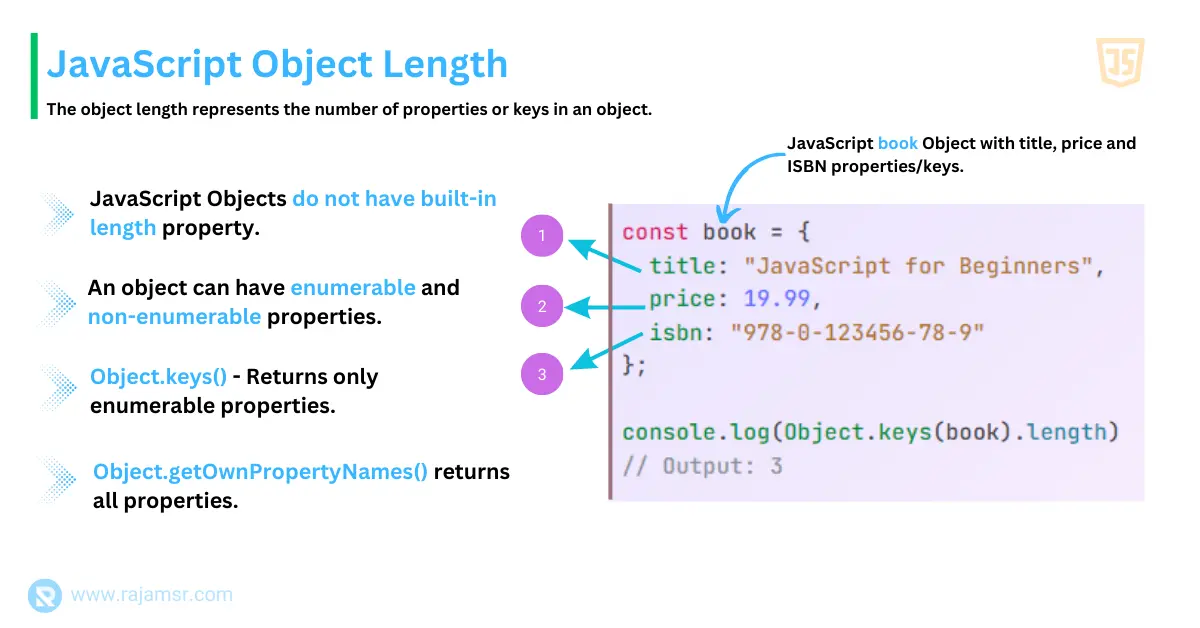
JavaScript check object length using Object.keys() method
To check if an object is empty, we can use the Object.keys()
static method. It will return object property names as an array. If the resulting array length is 0
then we can decide the object is empty.
Here is an example:
const book = {};
if (Object.keys(book).length === 0) {
console.log('Object is empty.')
}
else {
// Do object manipulation
}
// Output: "Object is empty."
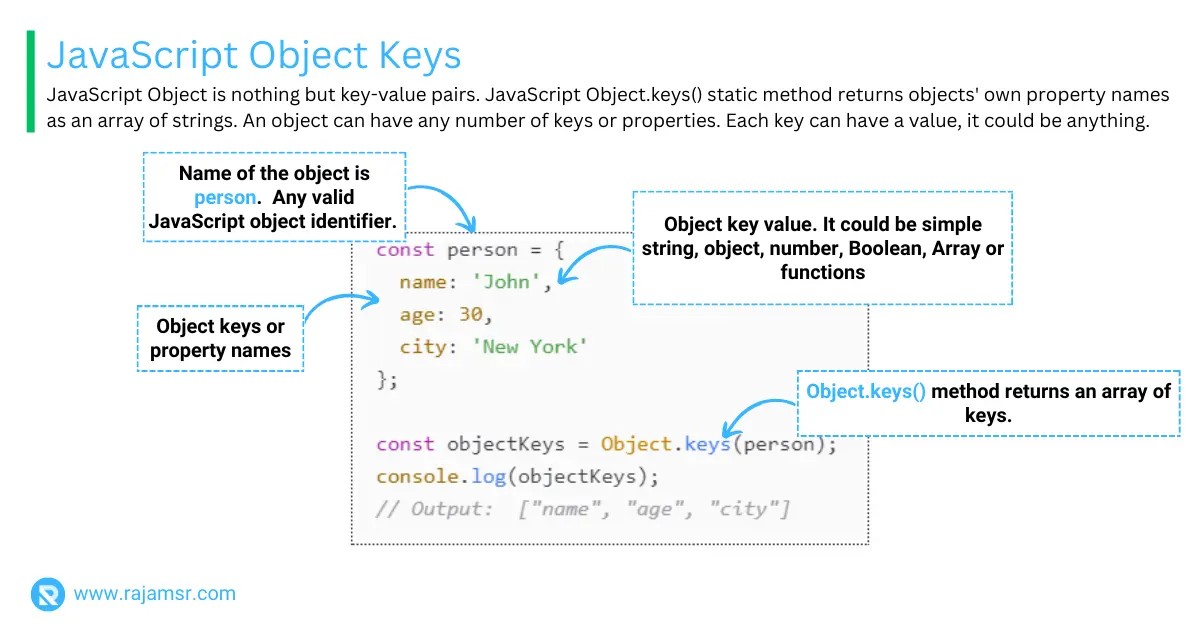
In this code, we created an empty object, book
. We then used the Object.keys()
method to get the length of object property names, and we checked if the length of the array was equal to 0
. Since the book
object does not have any properties, the message "Object is empty"
will be printed in the browser console.
JavaScript object length is 0
Sometimes, the length property of an object might return 0
. This can happen when the object has no enumerable properties. An object can have properties and still have a length of 0
. If all of its properties are non-enumerable,
So, how do you make sure objects have properties? To differentiate between an empty object and an object with a length of 0, we can use the Object.getOwnPropertyNames()
method instead of Object.keys()
. Here is an example:
let book = Object.create({}, { prop:
{ title: "JavaScript for Beginners",
enumerable: false
} });
console.log(Object.getOwnPropertyNames(book).length);
// Output: 1
console.log(Object.keys(book).length);
// Output: 0
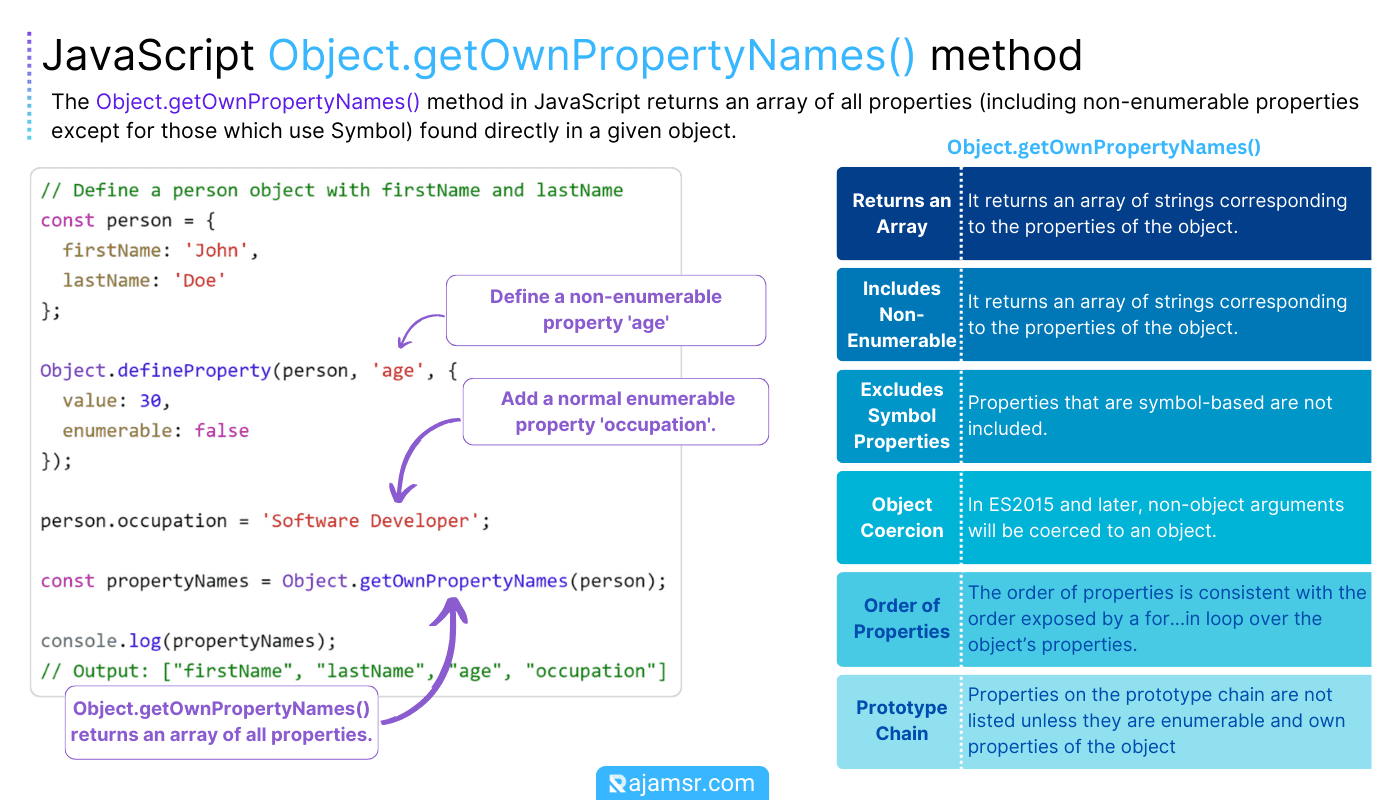
book
with a single non-enumerable
property prop
. We then used the Object.getOwnPropertyNames()
method to get an array of the object’s property names, and we checked the length. Since the book object has a non-enumerable property, the output, in this case, is 1.We then used the Object.keys()
method to get an array of the object’s enumerable property names, and we checked the length of the array. Since the book object has no enumerable properties, the output, in this case, is 0.JavaScript object key max length
There is a limit to the maximum length that the length property of an object can return. This limit is 2^53 - 1
, which is the maximum safe integer in JavaScript. If an object has more properties than this limit, the length property will not work correctly.
To check if an object has exceeded the length limit, we can use the Object.getOwnPropertySymbols()
method instead of Object.keys()
or Object.getOwnPropertyNames()
.
Here is an example:
let largeObject = {};
for (let index = 0; index < Math.pow(2, 53); index++) {
largeObject["key" + index] = "value" + index;
}
console.log(Object.getOwnPropertySymbols(largeObject).length > 0);
// Output: true
In this code, we created an object largeObject
with more properties than the maximum safe integer using the JavaScript math function Math.pow()
. We then used the Object.getOwnPropertySymbols()
method to get an array of the object’s symbol properties. After that, we checked if the length of the array was greater than 0. Since largeObject
has symbol properties, the output is true. It indicates that the object has exceeded the length limit.
JavaScript object length undefined
Object.length undefined
JavaScript objects do not have a length property like arrays and strings. So if you call the object.length
property on the object, it will return undefined
, as shown in the following example:
let book = { };
console.log(book.length);
// output: undefined
Safely call length property to avoid object length undefined
In some cases, you have to call the length
property on the dynamic parameter. To handle cases where the length of the object property returns undefined, we can use the JavaScript typeof operator to check if the length
property exists and is a number.
Here is an example of how to avoid object length undefined error:
let book = { };
console.log(typeof book.length === "number");
// output: false
let bookTitle = 'JavaScript for Beginners'
console.log(typeof bookTitle.length === "number");
// output: true

In this code, we created an empty object book that does not have a length
property. We then used the typeof operator
to check if the book.length
exists and is a number. Since book.length
does not exist, the output, in this case, is false.
At the same time, a string variable bookTitle
was declared with the value "JavaScript for Beginners"
. Since a JavaScript String has a length property, it returns true.
Conclusion
In this blog post, we explored how to get JavaScript object length using the Object.keys()
static method.
Covering topics such as how to check for empty objects, objects with a length of 0, object length limits, and cases where the length property might return undefined
. Understanding the behavior of the length property is essential for working with JavaScript objects effectively.
By using the methods described in this post, you can avoid common issues that can arise when working with the length property of objects.