Are looking to check whether a value exists in a string or array? you can use the JavaScript indexOf()
method. The index of the first occurrence will be returned for the specified value in a string or array. JavaScript indexOf()
method is commonly used to find the index of a specific value within an array or string.
In this blog post, we’ll dive into the details of:
- The overview
indexOf()
method - How to use with strings
IndexOf()
works with arrays- Works with an array of objects
- How to use the
indexOf()
method with regular expressions - Comparison of
indexOf()
vsincludes()
- Comparison of
search()
vsincludes()
- How to use the
indexOf()
method in a case insensitive. - Compare indexOf() with includes() method and discuss some potential pitfalls.
Let’s get started.
JavaScript IndexOf() method
The indexOf()
method is a built-in JavaScript method that works with arrays and JavaScript Strings. It gives the index of the first occurrence of a given value or substring in an array or string.
The syntax for the indexOf()
method is as follows:
array.indexOf(searchElement[, fromIndex])
string.indexOf(searchValue[, fromIndex])
Parameter | Description |
---|---|
array | Refers to the input array you want to search for a specific value or object. The array is one of the non-primitive primitive data types in JavaScript. |
searchElement | The value or object you want to search for in the array |
string | Refers to the input string that you want to search for a specific substring |
searchValue | The substring you want to search for. |
fromIndex | An optional parameter that specifies the starting index for the search. If no fromIndex is specified, the search starts at index 0. |
Now that we’ve defined the indexOf()
method, let’s explore how it works with different data types.
The indexOf() JavaScript string example
indexOf()
works in JavaScript:const greet = 'Hello, JavaScript!';
let index = greet.indexOf('Script');
console.log(index);
// Output: 11
index = greet.indexOf('script');
console.log(index);
// Output: -1
In this example, we’re searching for the substring 'Script'
within the string 'Hello, JavaScript!'
. The indexOf()
method gives the index of the first occurrence of the substring,'Script,'
which is 11
in this example.
If we look for 'script'
the same input string, the indexOf()
method returns -1
. Surprised? Because JavaScript indexOf()
in a string works in a case-sensitive way.
In the later section, we’ll dive into some tips and tricks to help you apply this method in a more thoughtful and considerate way.
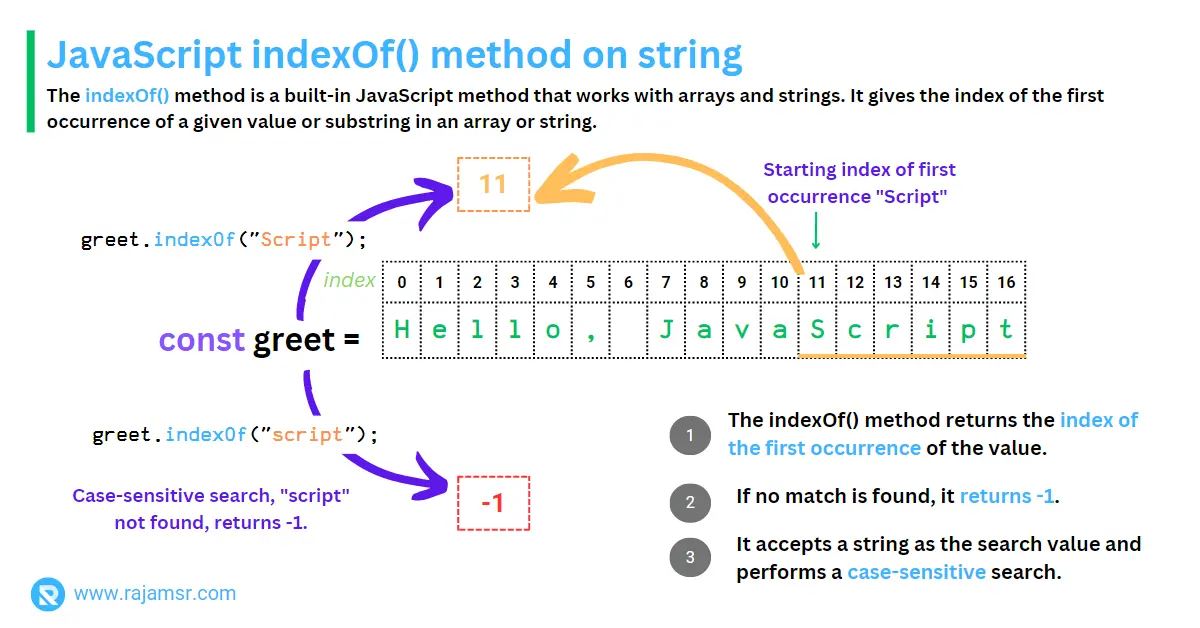
JavaScript indexOf() array example
Now let’s move on to searching for a specific value within an array. Here’s an example of how indexOf()
works on JavaScript array:
const numbers = [10, 20, 30, 40, 50];
const numberToSearch = 40;
if (numbers.indexOf(numberToSearch) !== -1) {
console.log(`Number ${numberToSearch} exists in the array.`);
}
// Output: "Number 40 exists in the array."
In this example, we’re searching for the value 40
within the array [10, 20, 30, 40, 50]
. The indexOf()
array method returns the index of the first occurrence of the value. In this case, value 40 exists in the array, so it will print "Number 40 exists in the array."
If we search for a value 45
that is not found in the array, the indexOf()
method returns -1
.
const numbers = [10, 20, 30, 40, 50];
index = numbers.indexOf(45);
console.log(index);
// Output: -1
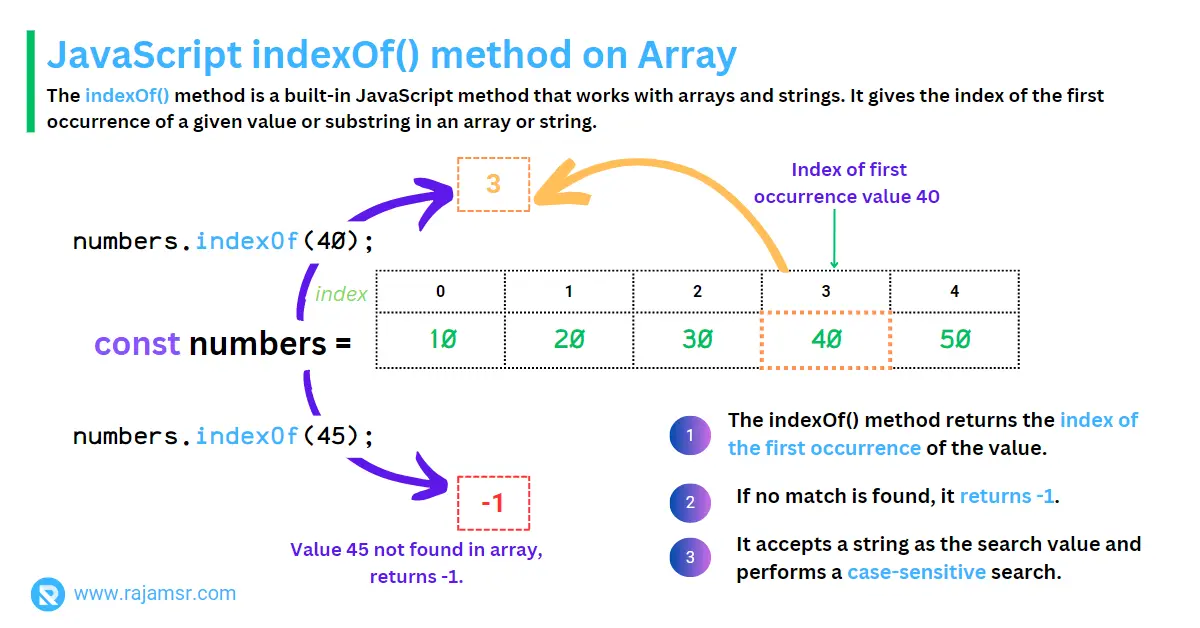
IndexOf() JavaScript array of objects example
Imagine you have a collection of objects, and you’re trying to find a specific object. But hold on, it won’t be simple! This is because searching through an array of objects can be more challenging than searching through a regular array.
Here’s an example of how to use the indexOf()
method to find a person object in an array of objects:
const people = [
{ id: 1, name: 'Scott' },
{ id: 2, name: 'Jane' },
{ id: 3, name: 'Bob' },
];
let person = people[1]
const index = people.indexOf(person);
console.log(index);
// Output: 1
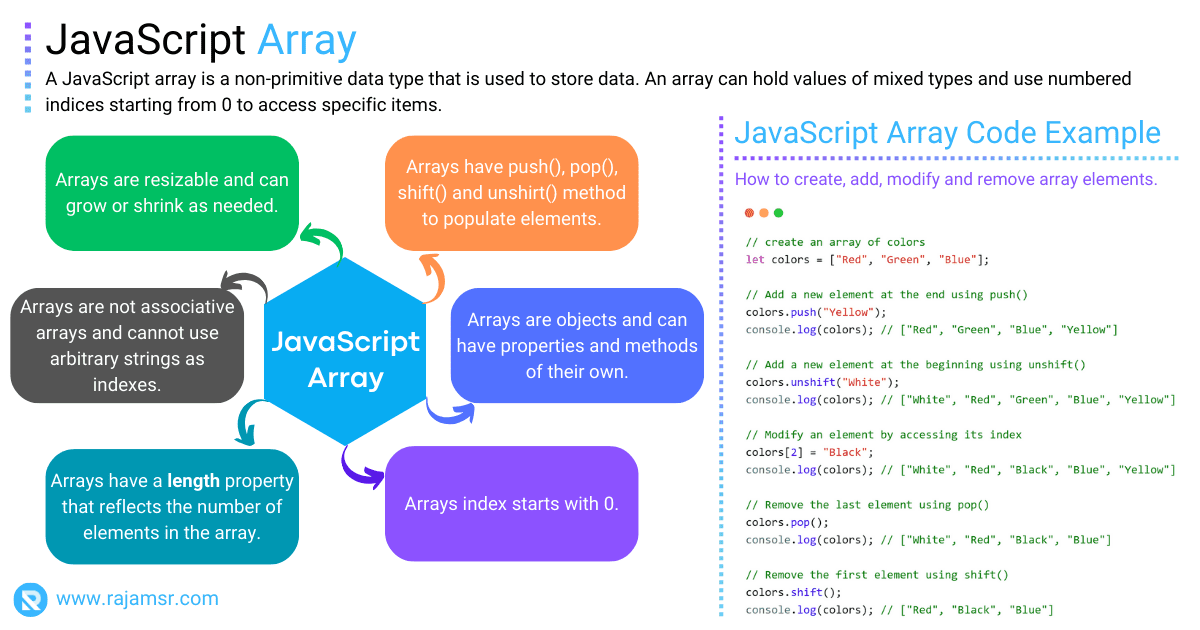
In this example, we’re searching for the object retrieved from array index 1. The index of the first instance of the object that matches the criteria will be returned from the JavaScript indexOf()
method. If the object cannot be found within the input array, the indexOf()
JavaScript method will return -1.
JavaScript indexOf() regex example
You may want to use regular expressions to look for a value within a string in some scenarios instead of using other approaches. Unfortunately, the indexOf() method does not support regular expression
. We can use the regular expression match()
method along with indexOf()
to find the first occurrence of the value.
Here’s an example of using a regular expression with indexOf()
method:
const inputText = 'JavaScript is the worlds most popular programming language';
var match = inputText.match(/popular/gi);
var firstIndex = match ? inputText.indexOf(match[0]) : -1;
console.log(firstIndex)
// Output: 30
Using a case-insensitive regular expression, we look for the substring 'popular'
within the string 'JavaScript is the world's most popular programming language'
. The match()
method returns an array of values that match. If there is no match, the number -1 is returned. If there are any matches, the indexOf()
method uses the first matching value.
Find multiple occurrences of value using IndexOf() method
What if you want to identify every instance of a substring within a string or every instance of a value within an array? Unfortunately, the indexOf()
method only returns the index of the first occurrence.
To find all occurrences, you’ll need to use a loop or a regular expression. Here’s an example using a loop to find all occurrences:
const numbers = [100, 200, 300, 400, 200, 500, 600];
const valueToSearch = 200;
const indices = [];
let index = numbers.indexOf(valueToSearch);
indices[0] = index
while (index !== -1) {
index = numbers.indexOf(valueToSearch, index + 1);
if (index !== -1)
indices.push(index)
}
console.log(indices);
// Output: [1, 4]
In this example, we’re searching for all occurrences of the value 3
within the array [100, 200, 300, 400, 200, 500, 600]
. With a while loop
, you can easily iterate over every element in the array and do something with each one. In this case, we want to push the index of each occurrence using the JavaScript Array push() method into a new array called "indices"
.
Finally, we log the indices array to the console, which contains the indices of all occurrences of the value 200
.
Comparison of indexOf() vs includes() methods
The includes()
method is a newer addition to JavaScript. The JavaScript includes() method provides a more intuitive way of checking if a value exists within an array or string. Here’s an example:
const numbers = [100, 200, 300, 400, 200, 500, 600];
console.log(numbers.includes(200));
// Output: true
console.log(numbers.indexOf(200));
// Output: 1
In this example, we’re checking if the value 3 exists within the array [100, 200, 300, 400, 200, 500, 600]
. Neither includes()
nor indexOf()
return the data from array or string.
includes() method | indexOf() method |
---|---|
The includes() method returns a boolean value, indicating whether or not the value exists within the array. If the value exists, it returns true; otherwise, it returns false. | The indexOf() method also returns the index of the first occurrence of the value instead of a boolean value. |
If you want to know if the value exists within the array, use the includes() method. | Use the indexOf() method if you want to know only the index of the value. |
Comparison of JavaScript search() vs indexOf() methods
The search()
and indexOf()
methods in JavaScript are used to search for a specified substring within a string. While both methods serve a similar purpose, they have some differences in terms of functionality and usage. Here’s a comparison of the JavaScript string search()
vs indexOf()
methods:
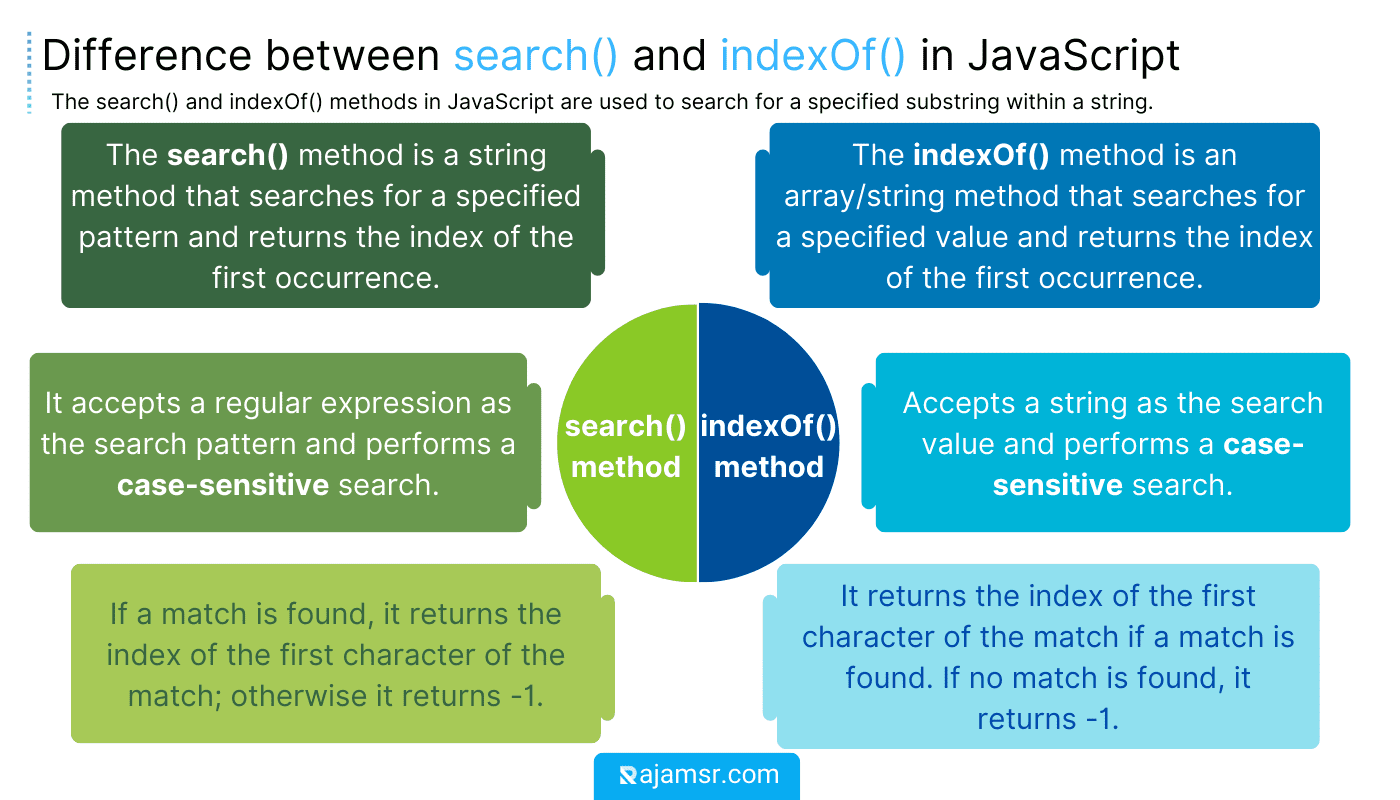
const message = 'JavaScript Runs Everwhere!';
const pattern = /Everwhere/i; // Case-insensitive search for 'Everywhere'
let searchIndex1 = message.search(pattern);
console.log(searchIndex1);
// Output: 16
let searchIndex2 = message.indexOf('Everwhere');
console.log(searchIndex2);
// Output: 16
In summary, search()
provides more powerful searching capabilities with regular expressions, while indexOf()
is a simpler method for basic string matching.
How to use JavaScript IndexOf() case insensitive manner
By default, the indexOf()
method is case-sensitive, meaning it will only find exact matches of a string or value.
Did you know that you can easily make your text case-insensitive? That’s right! You only need to use the toLowerCase()
or toUpperCase()
methods. These handy methods will convert all the characters in your text to either lowercase or uppercase in JavaScript uppercase making it easier for you to compare strings without worrying about case sensitivity.
Here’s an example of indexof()
with an ignore case:
const inputText = 'The quick brown fox jumps over the lazy dog';
const index = inputText.toLowerCase().indexOf('fox');
console.log(index);
// Output: 16
In this example, we’re searching for the substring 'brown'
within the string 'The quick brown fox jumps over the lazy dog'
, but we’re making the search case-insensitive by converting it to JavaScript lowercase using the toLowerCase()
method. Now, the indexOf()
method will find the substring 'fox'
regardless of whether it’s capitalized or not.
If you wish to make an array’s search case-insensitive, use the map()
JavaScript array methods to iterate over all elements to convert all members to lowercase or uppercase, and then use the indexOf()
method:
const months = ['January', 'February', 'March', 'April','May'];
const searchValue = 'april';
const index = months.map(m => m.toLowerCase())
.indexOf(searchValue.toLowerCase());
console.log(index);
// Output: 3
In this example, we’re searching for the value 'april'
within the array ['January', 'February', 'March', 'April', 'May']
, but we’re making the search case-insensitive by converting all elements in the array to lowercase using the map()
method. Finally, we use the indexOf()
method to find the index of the value 'april'
, regardless of whether it’s capitalized or not.
How to fix indexof() is not a function error
The indexOf()
in JavaScript is a string and array function. If you use it on a type other than a string or array, it will throw an "indexOf is not a function"
error.
Use the Array.isArray()
function to check whether the input is an array. To identify the type of a string, use the JavaScript typeof keyword. You can use both of the conditions to make sure the input is an array or string, as shown in the following code:
const message = 'JavaScript Runs Everwhere!';
if (Array.isArray(message) || typeof message === 'string') {
console.log(message.indexOf('Runs'))
}
else {
console.log(`Input is not type of string or array.`)
}
Conclusion
In conclusion, the indexOf()
method in JavaScript is an incredibly powerful method for searching for values within strings, arrays, and arrays of objects.
By using regular expressions, you can even search for values using more complex patterns. However, keep in mind that indexOf()
will only return the index of the first occurrence of a value, and you may need to use loops or regular expressions to find all occurrences.
Additionally, if you simply want to check if a value exists within an array or string, the includes()
method is generally a better option.
Lastly, don’t forget that you can make your search case-insensitive by converting strings or array elements to lowercase or uppercase using the toLowerCase()
or toUpperCase()
method.