If you want to know how to JavaScript get last element of array, you are not alone. Many developers face this common problem when working with arrays in JavaScript. How can you access the last element of an array without knowing its length or index? Is there a simple and elegant way to do it?
In this blog post, you will learn:
- How to use the length property to get the last element in an array
- Using pop() method remove the last array element
- Get the last array element with slice() without deleting it
- Filter an array and get the last element that meets a condition
- Use the spread operator to get the last array element
- Get the first and last array elements with destructuring assignment
- Write a custom function to get the last n elements of an array
These methods will help you manipulate arrays in JavaScript more easily and efficiently. You will also improve your coding skills and knowledge by learning different ways to solve the same problem.
1. Use the length property to get the last element of an array
How do you find the last item in a JavaScript array? Easy! Just use the JavaScript array length property. It tells you how many items are in the array. But remember, the last item’s index is always one less than the length. So, to get the last item, use this code:
// Get last element using array length property
let numbers = [1, 2, 3, 4, 5];
let lastElement = numbers[numbers.length - 1];
console.log(lastElement);
// Output: 5
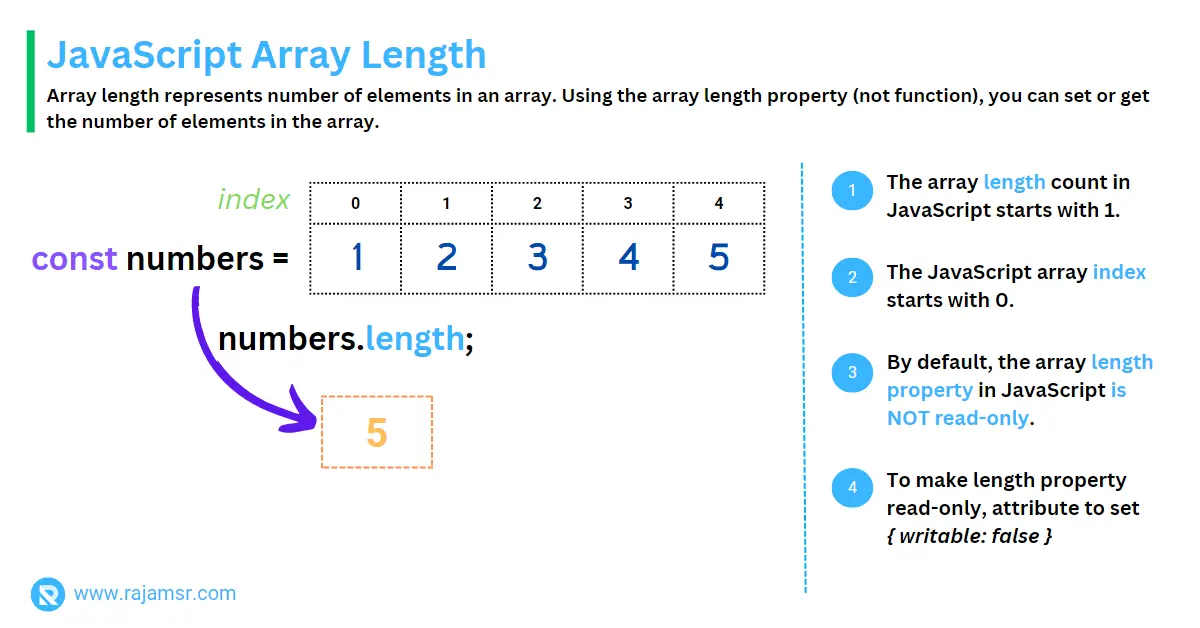
Getting the last element of an array is easy with the length property. You just need to access the element at the index of length minus one. But this method has some limitations:
- It won’t work for empty arrays, because the index will be negative and cause an error.
- It won’t change the original array, so you can’t use it to delete the last element.
- And it’s not very clear or elegant, because you have to repeat the array name and do some math.
Here are some examples of how to use the length property to get the last element of an array in JavaScript:
Get the last element of an array of strings
let colors = ["Red", "Green", "Blue", "White"];
let lastColor = colors[colors.length - 1];
console.log(lastColor);
// Output: "White"
Get the last element of an array of sorted numbers
let scores = [85, 90, 95, 100, 105];
let highestScore = scores[scores.length - 1];
console.log(highestScore);
// Output: 105
Get the last element of an array of objects
let books = [
{name: "Javascript For Kids", price: 1.9},
{name: "Javascript Cookbook", price: 4.99},
{name: "JavaScript Reference", price: 10.40}
];
let lastBook = books[books.length - 1];
console.log(lastBook);
// Output: {name: "JavaScript Reference", price: 10.40}
2. Use the pop() method to get and remove the last element of an array
Here’s a cool trick to get the last item in a JavaScript array. Just use the JavaScript array method pop()! It takes out the last item and gives it back to you. Like this:
// Get and remove last element using pop() method
let numbers = [1, 2, 3, 4, 5];
let lastElement = numbers.pop();
console.log(lastElement); // Output: 5
console.log(numbers); // Output: [1, 2, 3, 4]
The pop() method is a handy way to get the last item in any array. It doesn’t matter what the JavaScript array contains – numbers, strings, objects, anything! But be careful, because the pop() method also removes the last item from the array. That means you can’t use it if you want to keep the array intact. Also, the pop() method won’t work if the array is empty. It will give you undefined, which is not what you want.
Here are some examples of how to use the pop() method in JavaScript. You can see how it returns the last item and changes the array. You need to use a variable to store the result of the pop() method, because it won’t show up otherwise.
Get and remove the last element of an array of strings
let colors = ["Red", "Green", "Blue", "White"];
let lastColor = colors.pop();
console.log(lastColor); // Output: "White"
console.log(colors) // Output: ["Red", "Green", "Blue"]
Get and remove the last element of an array of numbers
let scores = [85, 90, 95, 100, 105];
let highestScore = scores.pop();
console.log(highestScore); // Output: 105
console.log(scores); // Output: [85, 90, 95, 100]
Get and remove the last element of an array of objects
let books = [
{name: "Javascript For Kids", price: 1.9},
{name: "Javascript Cookbook", price: 4.99},
{name: "JavaScript Reference", price: 10.40}
];
let lastBook = books.pop();
console.log(lastBook);
// Output: {name: "JavaScript Reference", price: 10.40}
console.log(books);
/* Output: [{name: "Javascript For Kids", price: 1099},
{name: "Javascript Cookbook", price: 4.99},]
*/
3. Use the slice() method to get the last element of an array without removing it
Do you want to learn a cool trick to access the last item of an array in JavaScript? You can use the JavaScript slice() method, which gives you a new array with some elements from the original array. The best part is that the original array stays the same, so you don’t lose any data. To get the last item of an array with slice(), you just need to write this:
// Get the last element of the array without removing it
let numbers = [1, 2, 3, 4, 5];
let lastElement =numbers.slice(-1)[0];
console.log(lastElement); // Output: 5
console.log(numbers); // Output: [1, 2, 3, 4, 5]
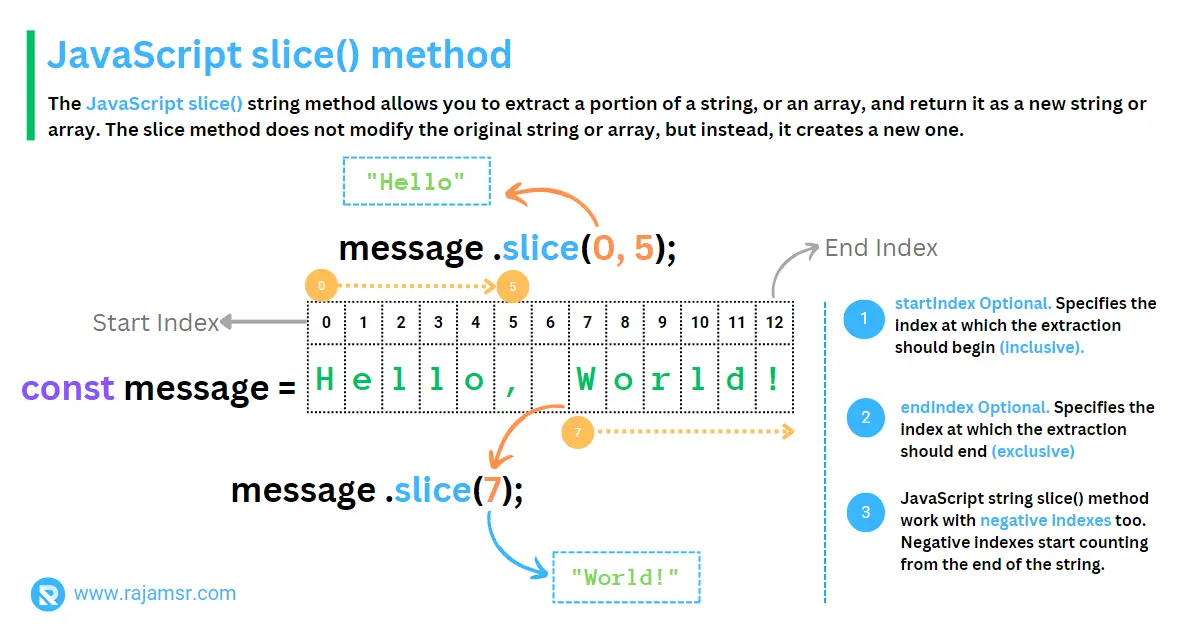
This is a way to get the last item in any array. It works no matter what the array contains. But this way has some problems:
- It does not work for empty arrays. If the array is empty, the slice() method gives back an empty array. And an empty array has no items, so we cannot get the last one.
- Another problem is that it makes a new array. This can use up more memory and make the code slower.
- It is not very clear or easy to read. We have to use a negative number and get the first item of the new array.
Here are some examples of how to use this way in JavaScript:
Get the last element of an array of strings
let colors = ["Red", "Green", "Blue", "White"];
let lastColor = colors.slice(-1)[0];
console.log(lastColor); // Output: "White"
console.log(colors); // Output: ["Red", "Green", "Blue", "White"]
Get the last element of an array of numbers
let scores = [85, 90, 95, 100, 105];
let highestScore = scores.slice(-1)[0];
console.log(highestScore); // Output: 105
console.log(scores); // Output: [85, 90, 95, 100, 105]
Get the last element of an array of objects
let books = [
{name: "Javascript For Kids", price: 1099},
{name: "Javascript Cookbook", price: 4.99},
{name: "JavaScript Reference", price: 10.40}
];
let lastBook = books.slice(-1)[0];
console.log(lastBook);
// Output: {name: "JavaScript Reference", price: 10.40}
console.log(JSON.stringify(books))
/*
[{"name":"Javascript For Kids","price":1099},
{"name":"Javascript Cookbook","price":4.99},
{"name":"JavaScript Reference","price":10.4}]
*/
4. Use the filter() method to get the last element of an array that meets a certain condition
Sometimes you want to find the last item in an array that matches a certain rule. For example, maybe you have an array of numbers and you want to get the last even number. How can you do that in JavaScript? One option is to use the filter() method. This method lets you create a new array with only the items that pass your rule. The original array stays the same. To get the last item of the new array, you can use the length property and subtract one.
Here is how it looks in code:
let numbers = [1, 2, 3, 4, 5];
let condition = (element) => element % 2 === 0;
let lastElement = numbers.filter(condition).slice(-1)[0];
console.log(lastElement); // Output: 4
console.log(numbers); // Output: [1, 2, 3, 4, 5]
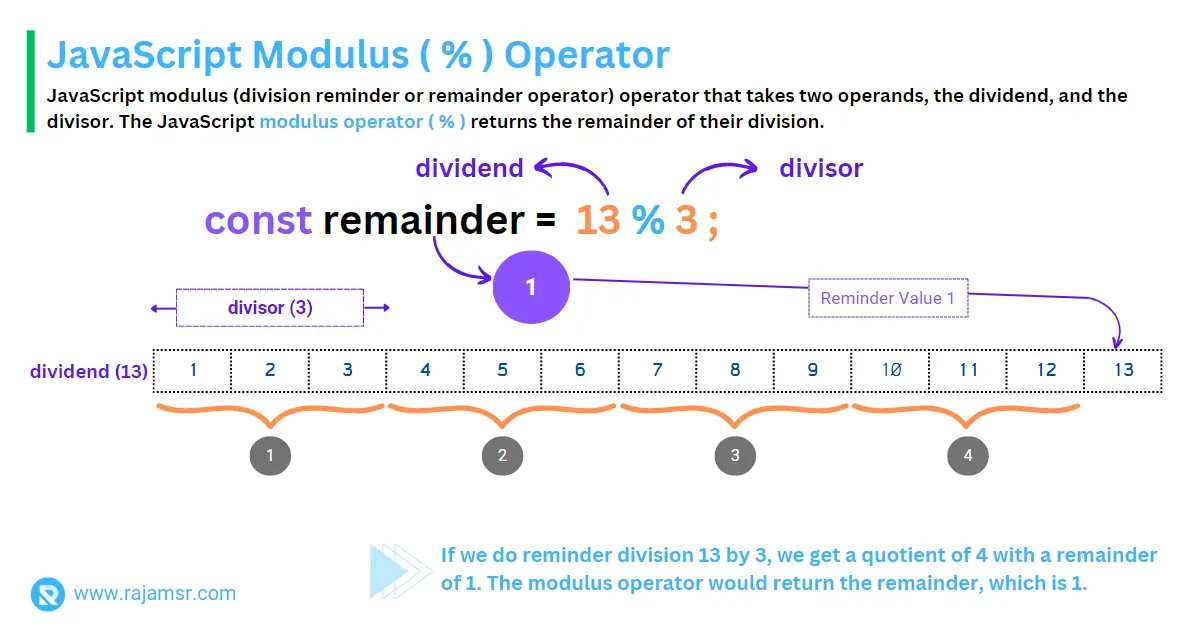
The code defines an array of numbers. A condition function checks for even numbers using the JavaScript modulus operator (%) and then filters the array based on the condition. It extracts the last element of the filtered array (the last even number) and prints it, along with the original array.
Sometimes you want to find the last item in an array that matches a certain condition. You can use the filter() method for this, but it has some problems:
- It won’t work if the array is empty or none of the items match the condition. It will give you an empty array, and you can’t get the last item from that.
- It makes two new arrays, one with the filter() method and one with the slice() method. This can use up more memory and slow down your code.
- This approach is not very clear or easy to read, because you have to write a function, use a negative index, and get the first item of the new array.
Here are some examples of how to use the filter() method to get the last item that matches a condition in an array in JavaScript:
Get the last element of an array of strings that starts with a vowel
let colors = ["Red", "Green", "Blue", "Earth yellow"];
let condition = (color) => /^[aeiou]/i.test(color);
let lastName = colors.filter(condition).slice(-1)[0];
console.log(lastName); // Output: "Earth yellow"
console.log(colors); // Output: ["Red", "Green", "Blue", "Earth yellow"];
Get the last element of an array of numbers that is divisible by 3:
// A function that tests if a score is divisible by 3
let scores = [85, 90, 95, 100, 105];
let condition = (score) => score % 3 === 0;
let highestScore = scores.filter(condition).slice(-1)[0];
console.log(highestScore); // Output: 105
console.log(scores); // Output: [85, 90, 95, 100, 105]
Get the last element of an array of objects that has a price less than 1
let books = [
{name: "Javascript For Kids", price: 1.9},
{name: "Javascript Cookbook", price: 4.99},
{name: "JavaScript Reference", price: 10.40}
];
let condition = (product) => product.price < 5;
let lastBook = books.filter(condition).slice(-1)[0];
console.log(lastBook);
// Output: {name: "Javascript Cookbook", price: 4.99}
5. Use the spread operator to get the last element of an array
Sometimes you want to get the last item from an array in JavaScript. There are many ways to do this, but one of them is using the ES6 feature spread operator. The spread operator looks like three dots (…), and it lets you turn an array into a list of values. You can use this list in different places, like when you call a function, or when you create a new array or object. To get the last item from an array with the spread operator, you can write something like this:
// Get the last element of the array using the spread operator
let array = [1, 2, 3, 4, 5];
let lastElement = Math.max(...array);
console.log(lastElement); // Output: 5
Let’s say you have an array and you want to get the last element of it. One way to do that is to use the spread operator (…) and the Math.max() function. But this way has some problems.
- It doesn’t work for empty arrays. Because, the spread operator gives nothing and that makes an error.
- For another thing, it uses the Math.max() function, which gives the biggest number from the array, but that might not be the same as the last element.
- And also, it is not very clear or easy to read, because you have to use a different function and the spread operator to get the element.
Here are some examples of how you can use the spread operator and the Math.max() function to get the last element of an array in JavaScript:
Get the last element of an array of strings
// Math.max() will not work with string array, it will return NaN
let colors = ["Red", "Green", "Blue", "White"];
let lastColor = Math.max(...colors);
console.log(lastColor); // Output: NaN
Get the last element of an array of numbers
// Get the highest score in the array using the spread operator
let scores = [85, 90, 95, 100, 105];
let highestScore = Math.max(...scores);
console.log(highestScore); // Output: 105
6. Use the destructuring assignment to get the first and last element of an array
If you want to get the first and last item of an array in JavaScript, try this trick. It’s called a destructuring assignment. It lets you take values from arrays or objects and put them into separate variables. You can write less code and make it easier to read. To use a destructuring assignment for the first and last item of an array, you can write something like this:
// Get the first and last element of the array using the destructuring assignment
let numbers = [1, 2, 3, 4, 5]; // an example array
let [firstElement, , , , lastElement] = numbers;
console.log(firstElement); // Output: 1
console.log(lastElement); // Output: 5
If you have an array with many elements, you can use a special technique to get the first and last one. This technique is called destructuring assignment. It lets you assign some elements of the array to variables, and ignore the rest. But this technique has some limitations:
- You can only use it if the array has at least two elements. Otherwise, it will give you an error.
- It does not change the original array. So you cannot use it to remove the first and last element from the array. And it is not very clear or easy to understand.
- You have to use commas to skip the elements you don’t want.
Here are some examples of how to use destructuring assignments to get the first and last element of an array in JavaScript:
Get the first and last element of an array of strings
let colors = ["Red", "Green", "Blue", "White"];
let [firstColor, , , lastColor] = colors;
console.log(firstColor); // Output: "Red"
console.log(lastColor); // Output: "White"
Get the first and last element of an array of numbers
// Get the lowest and highest score in the array using the destructuring assignment
let scores = [85, 90, 95, 100, 105];
let [lowestScore, , , , highestScore] = scores;
console.log(lowestScore); // Output: 85
console.log(highestScore); // Output: 105
Get the first and last element of an array of objects
let books = [
{name: "Javascript For Kids", price: 1.9},
{name: "Javascript Cookbook", price: 4.99},
{name: "JavaScript Reference", price: 10.40}
];
let [firstBook,,lastBook] = books;
console.log(firstBook); // Output: {name: "Javascript For Kids", price: 1.9}
console.log(lastBook); // Output: {name: "JavaScript Reference", price: 10.40}
7. Use the custom function to get the last n elements of an array
One more way to get the last n elements of an array in JavaScript is to write your own function for this task. A function is a piece of code that you can use many times, and you can decide what it does. So, to write a function that gets the last n elements of an array, you can use this code:
// Get the last n elements of the array using a custom function
function getLastElements(array, n) {
// Check if the array is empty or n is zero
if (array.length === 0 || n === 0) {
// Return an empty array
return [];
}
// Check if n is greater than the array length
if (n > array.length) {
// Return the whole array
return array;
}
// Use the slice() method to get the last n elements of the array
return array.slice(-n);
}
You can call this getLastElements() with an array and number of elements to get from last as shown below:
let array = [1, 2, 3, 4, 5];
let numberOfElementToGet = 2;
let lastElements = getLastElements(array, numberOfElementToGet);
console.log(lastElements); // Output: [4, 5]
Sometimes we want to get the last few items from an array. We can do this with a custom function that takes an array and a number as arguments. The function uses the slice() method to create a new array with only the last n items. This works for any kind of array, no matter what it contains. But this custom function has some problems:
- You need to write more code to make and use it, which can make our code harder to read and change.
- It also uses more memory and time, because it makes a new array with the slice() method.
Let’s see how the custom function works with some examples. We have different arrays with different types and values of items. We use the custom function to get the last n items from each array.
Get the last 2 elements of an array of strings
let colors = ["Red", "Green", "Blue", "White"];
let numberOfElementToGet = 2;
let lastElements = getLastElements(colors, numberOfElementToGet);
console.log(lastElements); // Output: ["Blue", "White"]
Get the last 4 elements of an array of numbers
let scores = [85, 90, 95, 100, 105]; // an array of scores
let numberOfElementToGet = 4;
let lastElements = getLastElements(scores, numberOfElementToGet);
console.log(lastElements); // Output:[90, 95, 100, 105]
Get the last 1 element of an array of objects
let books = [
{name: "Javascript For Kids", price: 1.9},
{name: "Javascript Cookbook", price: 4.99},
{name: "JavaScript Reference", price: 10.40}
];
let numberOfElementToGet = 1;
let lastElements = getLastElements(books, numberOfElementToGet);
console.log(lastElements);
// Output: {name: "JavaScript Reference", price: 10.40}
Conclusion
In this blog post, we have shown you how to get the last element of an array in JavaScript using different methods and scenarios. We have covered the following methods:
- Use the length property to get the last element of an array
- Use the pop() method to get and remove the last element of an array
- Use the slice() method to get the last element of an array without removing it
- Use the filter() method to get the last element of an array that meets a certain condition
- Use the spread operator to get the last element of an array
- Use the destructuring assignment to get the first and last element of an array
- Use the custom function to get the last n elements of an array
I hope you have learned something new and useful from this blog post. If you have any questions or feedback, please leave a comment.