Are you looking for a quick way to check whether all elements in an array satisfy a certain condition? You can accomplish this by using the JavaScript every() array function.
The every() method is a built-in array method in JavaScript that returns a boolean value indicating whether all elements in an array pass a test implemented by a provided function. This method is extremely useful for validating arrays and for making complex conditions for arrays.
In this blog post, we will look into:
- What is JavaScript’s every() function?
- How to use every() method in JavaScript
- Top 5 real-time use-cases using every() method
- Benefits of using every() method
What is JavaScript array.every() method?
The every() method in JavaScript is a built-in array method that tests whether all elements pass a test in an array implemented by a provided function. The array.every() in the JavaScript method returns true if all elements in the array pass the test. If any one of the elements fails the test, it will return false.
The syntax for the every() method is as follows:
array.every(callback(currentValue[, index[, array]])[, thisArg])
Parameter | Description |
---|---|
callback | The function to test for each element in an array. The callback function takes three arguments: currentValue, index, and array. |
thisArg | Optional. Object to use as this when executing callback. |
The use case of the every() method is to validate arrays and make complex conditions for arrays. It is useful for checking if all elements in an array meet certain criteria. It is often used in combination with other JavaScript array methods such as filter() and map().
How to use .every() method in JavaScript?
The JavaScript array every() method is straightforward to use. Let’s start with a basic example.
const numbers = [2, 4, 6, 8, 10];
const isEven = (num) => {
return num % 2 === 0;
};
const result = numbers.every(isEven);
console.log(result);
// Output: true
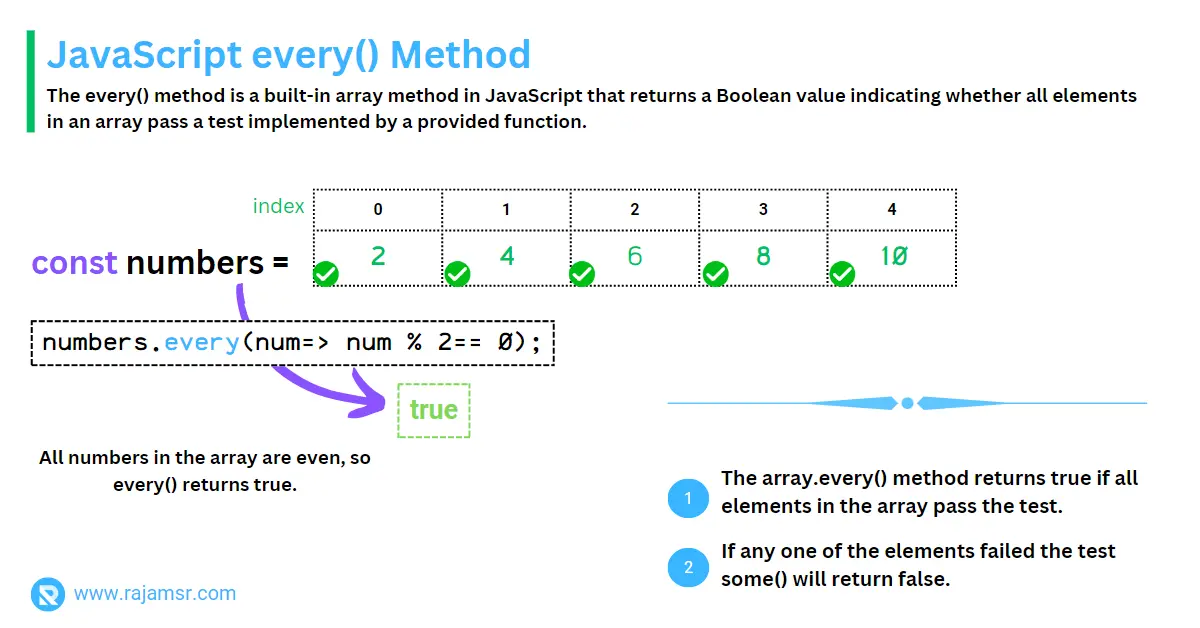
In this example, we have an array of numbers and a callback function called the isEven() function that checks if a number is even using the JavaScript modulus operator %. We pass the isEven() arrow function as a parameter to the every() method, and the method returns true because all elements in the array are even.
Let’s look at a more advanced example of the array every() method.
const numbers = [2, 4, 6, 8, 10];
const limit = 5;
const isLessThanLimit = (num) => {
return num < limit;
};
const result = numbers.every(isLessThanLimit);
console.log(result);
// Output: false
In this example, we have an array of numbers and a callback function called LessThanLimit(). It will check if a number is less than a specified limit.
We pass the isLessThanLimit() function as a parameter to the every() method. The every() method returns false because not all elements in the array are less than the limit of 5.
In JavaScript, if you want to ensure at least one element meets certain conditions instead of all, you can use the some() method. JavaScript some() method will return true only if at least one element in an array satisfies a condition.
Top 5 real-time use cases of JavaScript every() method
1. Validating form inputs
Consider using an HTML form to collect user feedback. To make sure that all mandatory input fields are filled by the user, you can use the every() method as shown in the following code.
const formInputs = document.querySelectorAll('input');
const allInputsValid = Array.from(formInputs).every(input => input.value !== '');
if (allInputsValid) {
// Submit the form
} else {
// Show an error message
}
2. Checking if all objects in an array meet a condition using every() in JavaScript
Assume you have a roster of students with grades. If you want to confirm that everyone scored higher than the passing mark of 70, use the following code:
const students = [
{ name: 'Alice', grade: 85 },
{ name: 'Bob', grade: 92 },
{ name: 'Charlie', grade: 79 }
];
// Check whether all the students scored above 70
const allStudentsPassed = students.every(student => student.grade >= 70);
if (allStudentsPassed) {
console.log('All students passed!');
} else {
console.log('Some students failed.');
}
When working with complex logic, it is always best to write JavaScript comments explaining why you are doing so. Most importantly, you have to keep it up-to-date to make it relevant to the current code.
3. Testing if all elements of an array are unique
This is a common use-case scenario. Assume you’re reading student registration numbers from external data and want to ensure there are no duplicate entries.
To verify if there is any duplicate element in an array, you can use the following code block:
const registrationNumbers = [1001, 1002, 1003, 1004, 1005];
const allUnique = registrationNumbers
.every((value, index, self) => self.indexOf(value) === index);
if (allUnique) {
console.log('All numbers are unique.');
} else {
console.log('There are duplicate registration number(s).');
}
// Output: "All numbers are unique."
Note that this method will help you check whether or not there is a duplicate in an array. However, it will not tell you which is a duplicate element.
4. Checking if an array of numbers is sorted in ascending order
Before processing, you may require data in sorted order. Using the JavaScript every() method, you can check whether all of the elements in the sorted sequence are present. Logic is very simple: compare the current element with the previous one; if it is greater than the current, then return false.
const numbers = [1, 2, 3, 4, 5];
const isSorted = numbers
.every((value, index, self) => index === 0 || value >= self[index - 1]);
if (isSorted) {
console.log('The array is sorted in ascending order.');
} else {
console.log('The array is not sorted in ascending order.');
}
// Output: "The array is sorted in ascending order."
5. Verifying if an array of strings contains a particular substring
Sometimes you need to look for a specific JavaScript string that appears in all array elements. In this scenario, you can use the every() method, as shown in the example below:
const searchTerm = 'ar'
const months = ['January', 'February', 'March', 'April'];
const containsSubstring = months.every(str => str.includes(search));
if (containsSubstring) {
console.log(`All strings contain the substring'${search}'.`);
} else {
console.log(`Some strings do not contain the substring '${searchTerm}'.`);
}
// Output: "Some strings do not contain the substring 'ar'."
Except for “April,” the other months in the preceding example have the text “ar,” so the every() method returns the text “Some strings do not contain the substring ‘ar’.” JavaScript includes() array method that will return true if a specified string exists.
Benefits of using JavaScript every() method
The array every() method has several benefits for JavaScript developers. Some of the key benefits include:
Readability: The every() method is a clear and concise way to test whether all elements in an array meet a certain condition. It improves the readability of code by making it easier to understand what the code is doing.
The following method finds whether all elements in the numbers array are even numbers using a traditional for loop.
function areAllEvenNumbers(array) {
for (let i = 0; i < array.length; i++) {
if (array[i] % 2 !== 0) {
return false;
}
}
return true;
}
const numbers = [2, 4, 6, 8, 10];
const isAllEven = areAllEvenNumbers(numbers);
console.log(isAllEven);
13 lines of code simplified to 3 lines using the array every() method:
const numbers = [2, 4, 6, 8, 10];
const isAllEven = numbers.every(e => e % 2 === 0);
console.log(isAllEven);
Faster Execution Time: The every() method is optimized for performance, and it can often be faster than manually iterating through an array and checking each element individually. This can be especially beneficial for large arrays, where the performance gains can be significant.
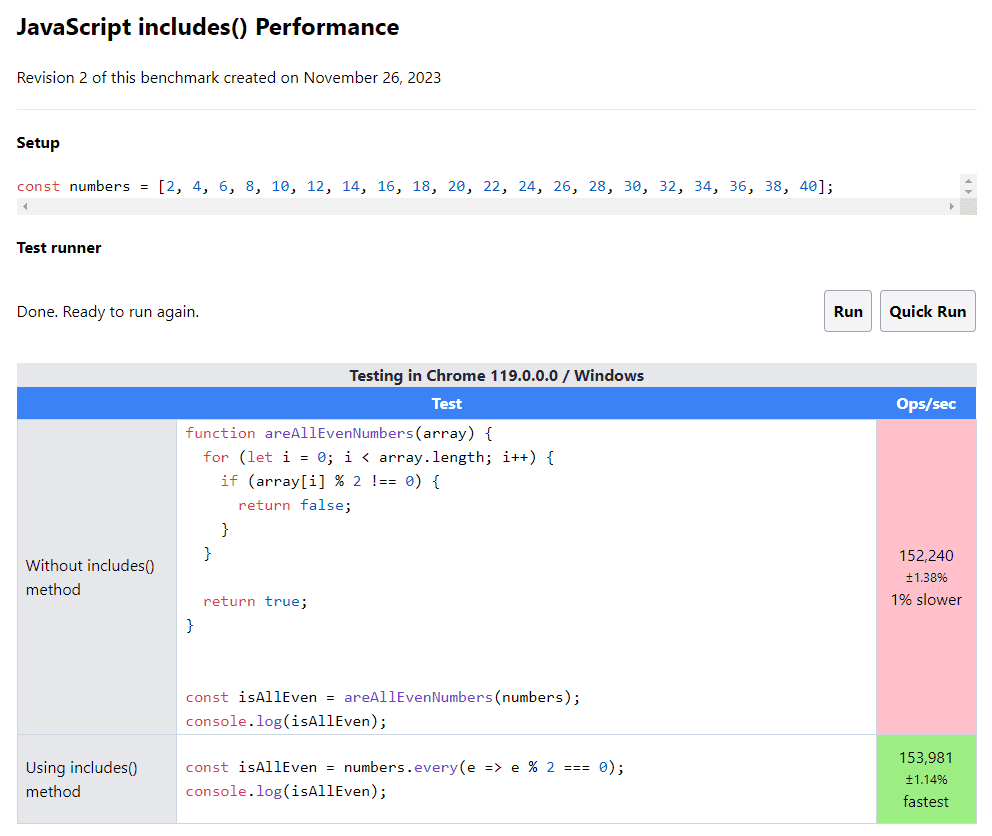
I performed a performance test on an array of 20 even numbers. I compared the array includes() method with a manual operation. The results showed that the array includes() method was slightly faster (153,981 operations per second) than the manual operation (152,240 operations per second), as expected.
Conclusion
In conclusion, the JavaScript every() method provides a clear and concise way to validate arrays and make complex conditions for arrays.
You can use JavaScript every() method to check whether all elements in an array meet a certain condition in a simple way.
In this blog post, we discussed 5 different real-time use cases of how to use every() function. In addition to these use cases, discussed the three benefits of using the every() function.