JavaScript string concatenation is the process of joining two or more strings together to form a new string. This feature of JavaScript is very useful and powerful and can help you manipulate text data in various ways. String concatenation can help you add some flair to your web page, format your data for display, or build complex queries for your database.
In this post, we will explore:
- What is string concatenations?
- Concatenate strings with six different methods
- How to handle string concatenation null issue
- String concatenation time complexity
By the end of this post, you will be able to choose the best method for your needs and write cleaner and faster code.
What is JavaScript string concatenation?
String concatenation in JavaScript is the process of joining two or more strings into one. In JavaScript, there are several ways to concatenate strings, such as:
- Using the concatenation operator (+)
- The string concat() function
- Template literals.
- Concatenate strings using the join() method
- Using the += operator to concatenate string
- Using reduce() method
Let’s see how to concatenate strings in JavaScript with code examples.
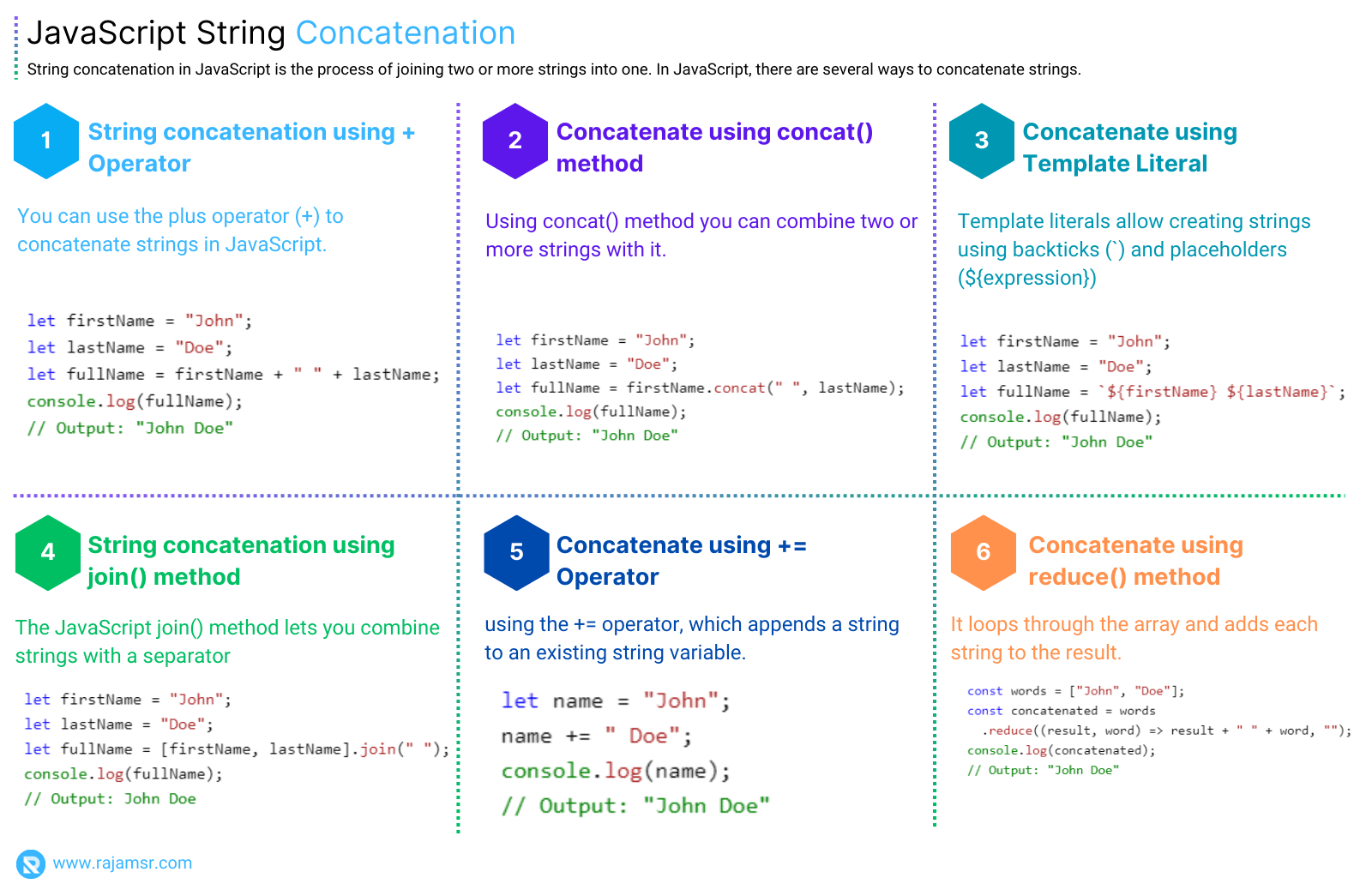
1. JavaScript string concatenation using + with variables
Strings are sequences of characters that we can use to store and manipulate text. One of the coolest things we can do with strings is to combine them. This is called string concatenation.
You can use the plus operator (+)
to concatenate strings in JavaScript. It’s super easy and fun! Just put a plus sign between the strings you want to join, and you’ll get a new string that contains both of them.
Let me show you how to concatenate strings with JavaScript variables. Variables are like containers that hold values. We can assign a string to a variable, and then use the variable name to concatenate it with another string. For example:
let firstName = "John";
let lastName = "Doe";
let fullName = firstName + " " + lastName;
console.log(fullName);
// Output: "John Doe"
Let me show you how to create a full name from two JavaScript variables. We have firstName
and lastName
, and we want to join them with a whitespace in between. One way to do this is to use the + operator. But this is not a good idea if you have many strings to join together. It can make your code slow and hard to read.
But be careful when you use the +
operator with other types of data, such as numbers or booleans. The +
operator will try to convert them to strings before joining them, and this can cause some weird results. The boolean variable isHappy
concatenated with a string variable message
it is converted as string. You can confirm by using the JavaScript typeof operator as shown in the following example:
let age = 25;
let message = "I am " + age + " years old.";
console.log(message); // I am 25 years old.
console.log(typeof message); // string
let isHappy = true;
message = "I am " + isHappy;
console.log(message); // I am true
console.log(typeof message); // string
Advantages and disadvantages of the plus operator
The plus operator is easy to use and understand, and it works well for simple concatenation tasks. However, it also has some drawbacks, such as:
- You might get confused or make mistakes when mixing strings with other data types. Sometimes, the types don’t match and you get weird results or errors.
- You have to type a lot and repeat yourself when joining multiple strings or variables. This is boring and wastes your time.
- You end up with long and messy code when joining long or complex strings. This makes it hard to read and edit your code later.
2. JavaScript concatenate strings using concat() method
You can also use the JavaScript string concat() method to join strings in JavaScript. It’s a handy function of the String object. You can combine two or more strings with it, like this:
let firstName = "John";
let lastName = "Doe";
let fullName = firstName.concat(" ", lastName);
console.log(fullName);
// Output: "John Doe"
To build the absolute filename, the concat()
function combines the fisrtName
and lastName
variables into a single string.
You can use concat()
to join strings with other things, like numbers or true/false values. But be careful! Sometimes this can cause problems, because the other things will change into strings before they join. Look at this example:
let age = 25;
let message = "I am ".concat(age, " years old.");
console.log(message); // I am 25 years old.
console.log(typeof message); // string
let isHappy = true;
message = "I am ".concat(isHappy);
console.log(message); // I am true
console.log(typeof message); // string
Advantages and disadvantages of the concat() method
concat()
method is similar to the plus operator, but it has some differences, such as:- You can easily see that you are joining strings, because
concat()
is made for that. - You can join as many strings or variables as you want, without worrying about adding separators between them.
- You can join strings with other data types, without getting unexpected results because of the order or precedence of the operands.
concat()
method also has some drawbacks, such as:- It’s slower and less efficient than the plus operator. It needs to call a function and make a new string every time.
- It’s less compatible and portable than the plus operator. Some old browsers or environments don’t support it.
- It’s less intuitive and natural than the plus operator. It has a different syntax and style from the rest of JavaScript.
3. JavaScript string concatenation using template literal
Another way to concatenate strings in JavaScript is by using template literals (also known as template strings). Template literals are a new feature introduced in ES6, and they allow creating and formatting strings using backticks (`) and placeholders (${expression}). For example:
let firstName = "John";
let lastName = "Doe";
let fullName = `${firstName} ${lastName}`;
console.log(fullName);
// Output: "John Doe"
template literals
are used to join two strings fistName
, and lastName
variables into a single string to build full name.But that’s not all! Template literals can also mix strings with other data types, like numbers or booleans. And you don’t have to worry about type conversion, because the placeholders can handle any valid JavaScript expression. For example: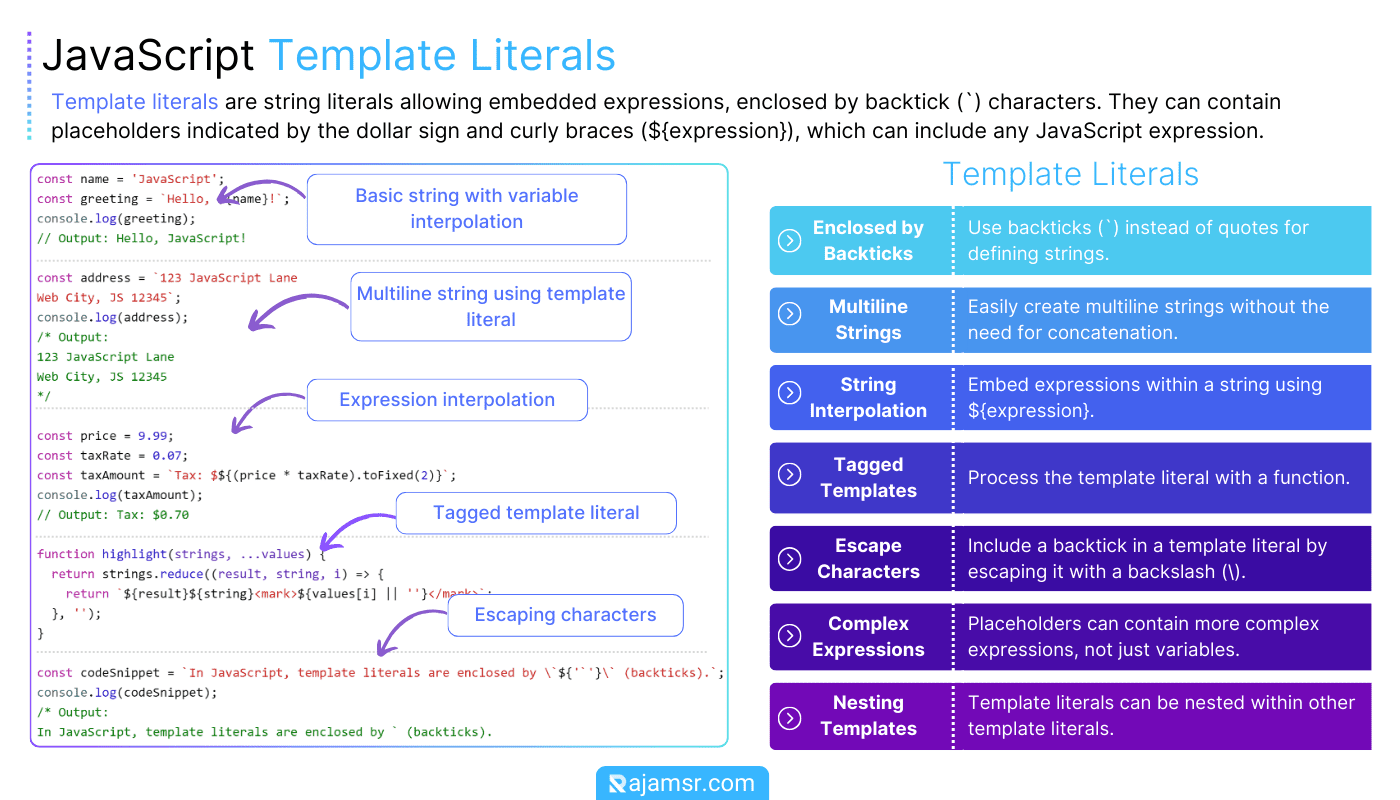
let age = 25;
let message = `I am ${age} years old.`;
console.log(message); // I am 25 years old.
console.log(typeof message); // string
let isHappy = true;
message = `I am ${isHappy ? "happy" : "sad"}.`;
console.log(message); // I am happy
console.log(typeof message); // string
Advantages and disadvantages of template literals
Template literals are the most advanced and powerful way to concatenate strings in JavaScript, and they have many advantages, such as:
- Template literals make concatenation easy and fun. You’ll love how simple they are to use and understand.
- Template literals are super fast and efficient. No more function calls or type conversions slowing you down.
- Template literals work everywhere. Most browsers and environments support them, so you don’t have to worry about compatibility issues.
- Template literals fit right in with JavaScript. They use the same syntax and style as the rest of the language, so you can write them naturally and intuitively.
- Template literals give you lots of flexibility and convenience. You can put any expression or value inside the placeholders, and you can also write them on multiple lines and include special characters.
However, template literals also have some disadvantages, such as:
- Some old browsers or environments don’t support them. You may need to transpile or polyfill them to make them work.
- Backticks or placeholders can be tricky and buggy. You may need to escape or nest them to avoid problems.
- Complex expressions or values inside the placeholders can make your code hard to read and maintain.
4. Concatenate strings in JavaScript using join() method
Do you want to combine all the items in an array into one string? You can do that with the JavaScript Array method join()! Just use it like this:
The JavaScript join() method lets you combine strings with a separator. You can choose the separator by passing it as a parameter. If you don’t, it will use a comma (','
) as a separator by default.
You can also use join()
to combine strings with other things, like numbers or booleans. But be careful, because this can cause some problems. The other things will be changed to strings before they are combined. For example:
let firstName = "John";
let lastName = "Doe";
let fullName = [firstName, lastName].join(" ");
console.log(fullName);
// Output: John Doe
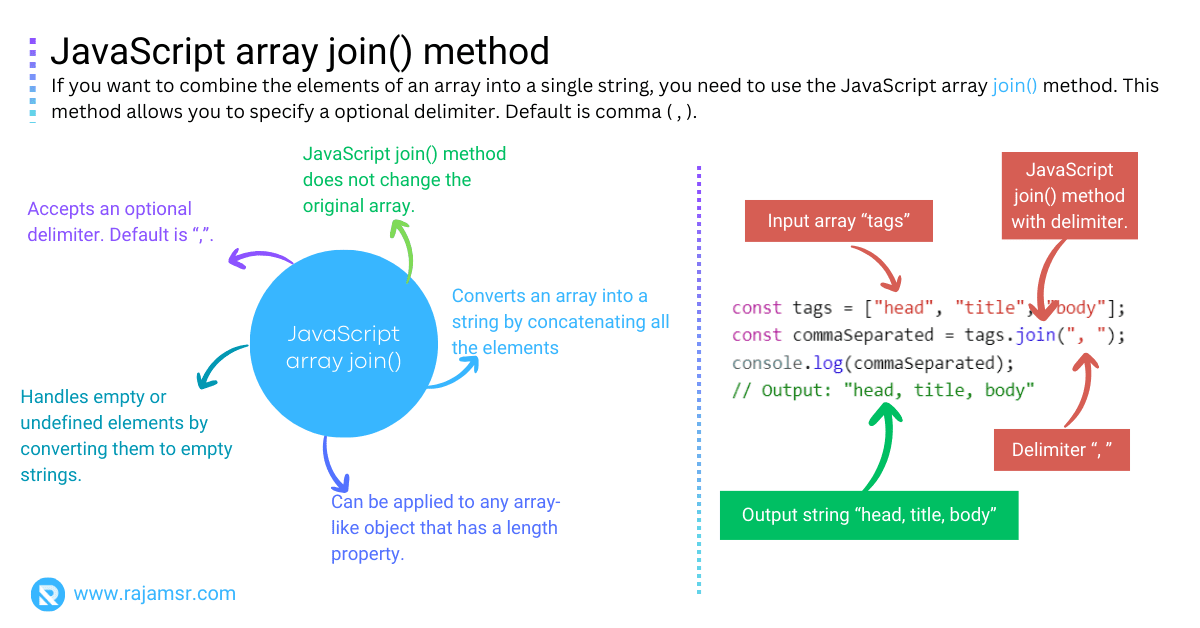
let age = 25;
let message = ["I am", age, "years old."].join(" ");
console.log(message); // I am 25 years old.
console.log(typeof message); // string
let isHappy = true;
message = ["I am", isHappy].join(" ");
console.log(message); // I am true
console.log(typeof message); // string
Advantages and disadvantages of the join() method
join()
method is different from the previous methods, as it requires an array as the input and returns a string as the output. This has some advantages and disadvantages, such as:- You get a faster and smoother performance. No need to create many strings, just one!
- You have more flexibility and convenience. You can choose any separator or delimiter you want.
- You have more readability and maintainability. You can use line breaks and indentation to make your code look nice.
- This method needs more work. You have to make an array and call a function every time.
- This method may not work everywhere. Some old browsers or environments don’t support it.
- This method is different from the rest of JavaScript. It uses a different type and structure of data.
5. JavaScript append string using the += operator
Another approach is to use the +=
operator, which appends a string to an existing string variable. This method is useful for gradually building up a string:
let name = "John";
name += " Doe";
console.log(name);
// Output: "John Doe"
Advantages and disadvantages of the join() method
+=
operator requires a string as the input and returns a string as the output. This has some advantages and disadvantages, such as:- Use
+=
to join strings. It’s simple and clear! - This way, you don’t need new variables every time. That saves memory and makes your code faster.
- You can create strings on the fly, depending on what you need or want.
- Strings are immutable in languages. You can’t change them, only make new ones.
- The
+=
operator looks like it changes a string, but it makes a new one each time. This can slow down your code if you use it a lot with big strings. - The
+=
operator is short, but not always clear. It can be hard to follow if you have many things to join together.
6. Using array reduce() for concatenation
reduce()
method on an array! It loops through the array and adds each string to the result. Here’s how it works:const words = ["John", "Doe"];
const concatenated = words.reduce((result, word) => result + " " + word, "");
console.log(concatenated);
// Output: "John Doe"
Advantages and disadvantages of the join() method
The reduce()
method requires an array as the input and returns a string as the output. This has some advantages and disadvantages, such as:
reduce()
is awesome for many kinds of aggregations, not just string concatenation.- You can customize how you want to join the strings with a callback function.
- You can do it all in one line, no loops or ifs needed.
However, the join method also has some drawbacks, such as:
- reduce() is powerful, but it can slow down your code for simple tasks like joining strings.
- Be careful with the accumulator (result) in the callback function. It can cause unexpected changes in your code.
- If you don’t know functional programming,
reduce()
might be hard to understand for simple string operations. You need to learn more. - The code is short, but
reduce()
adds more complexity. It can be tricky for beginners.
JavaScript concat string with separator (custom delimiter)
Let’s see how to join two or more strings with a separator. You can use any valid string parameter or character as a separator.
In the following example, the semicolon (;) is used as a separator.
let fileTypes = ["*.jpeg", "*.bmp", "*.txt", "*.docx"];
let fileTypesString = fileTypes.join(';');
console.log(fileTypesString);
// Output: "*.jpeg;*.bmp;*.txt;*.docx"
String concatenation result is null
JavaScript string concatenation will throw cannot read properties of null if you are using the concat()
method on uninitialized string values. If you concatenate the string with a null value, the string result includes null.
let input;
let concatenatedString = input.concat("Hello");
console.log(concatenatedString)
// Output: Cannot read properties of null (reading 'concat')
You can rewrite the above code as shown below to safely handle null value.
let input;
let concatenatedString = (input) ? input.concat("Hello") : "Hello";
console.log(concatenatedString)
JavaScript string concatenation time complexity
Let’s talk about how to join strings in JavaScript. There are different ways to do it, but the simplest one is to use the +
operator. For example, "Hello" + "World"
gives us "HelloWorld"
. Easy, right?
But there is a catch. JavaScript strings cannot be changed once they are created. So every time we use the +
operator, we actually make a new string with the contents of both strings. This takes some time and memory.
How much time and memory? Well, it depends on how long the strings are. If we have two strings of length n
, then joining them with the +
operator takes O(n^2)
time. That means the time grows very fast as the strings get longer. This is not very efficient.
So what can we do to avoid this problem? We will see some other methods to concatenate strings in JavaScript in the next section.
For example:
let contents = '';
for (let index = 0; index < n; index++) {
contents += index.toString();
}
This is because the loop creates a new string in each iteration that contains the current number converted to a string using the JavaScript toString() method, as well as the contents of the existing string.
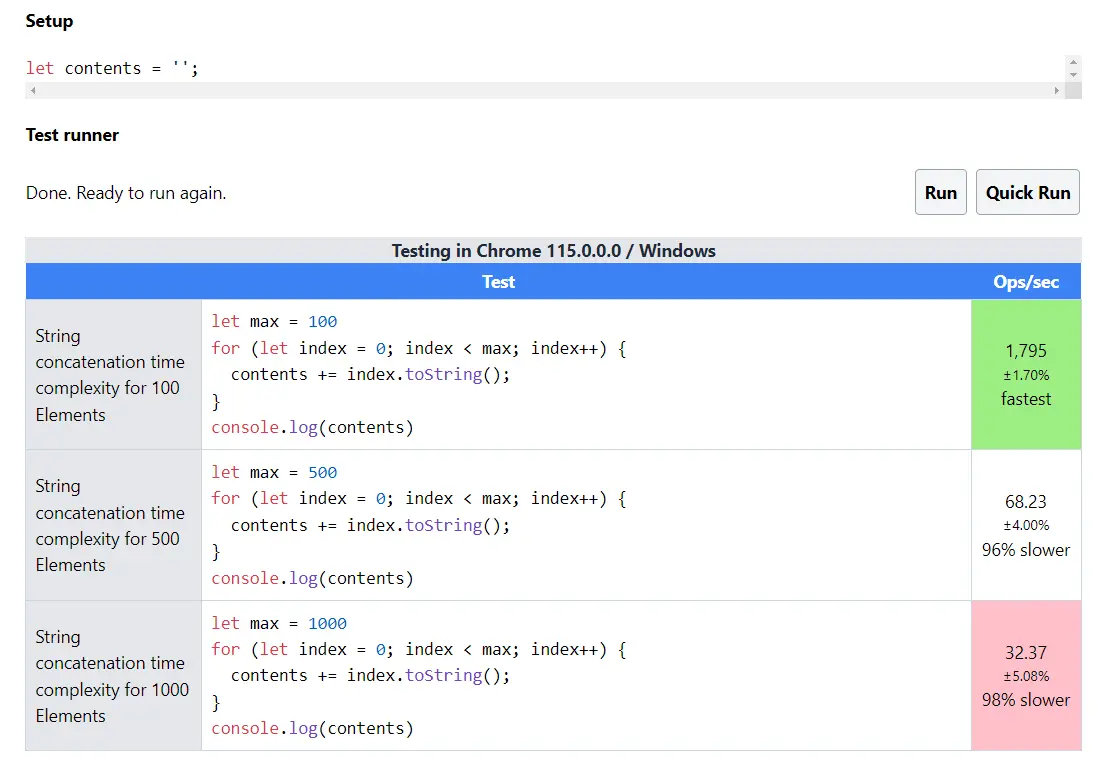
100
to 500
and 1000
. The number of operations per second has been drastically reduced to 98%
drastically.Efficient way to combine strings
Here’s a great tip for joining strings in JavaScript: use the join()
method! It’s super fast and easy. It only takes O(n)
steps, where n is the total length of the strings. How cool is that? All you need to do is pass an array of strings and a separator to the join()
method, and it will create a new string with all the elements joined by the separator.
For example, you can join strings like this with the join()
method:
let contents = [];
for (let index = 0; index < n; index++) {
contents.push(index.toString());
}
let str = contents.join('');
join()
method! It’s fast and easy. It loops over the array once and adds an empty string between each element. The time it takes is O(n), which means it depends on the number of elements in the array.Conclusion
In this blog post, you have learned six different ways to concatenate strings in JavaScript, as well as their advantages and disadvantages. You have also learned about a modern and convenient way to create and format strings using template literals.
All these techniques have their use cases, and depending on your use case, you can choose the one that best suits your needs.
The concatenation operator (+)
is the most common and straightforward way, while the template literals
and join()
method provide additional functionality and readability.
Now that you know how to concatenate strings in JavaScript, you can choose the best method for your needs and write cleaner and faster code. You can also experiment with different methods and see how they affect the performance and readability of your code.
If hope, you learned something new from this post. Over to you: Which method you are going to use to concatenate strings?