Do you know how to use the JavaScript modulus operator (%) in your code? If not, you are missing out on one of the most useful and versatile operators in JavaScript. The modulus operator returns the remainder of a division operation, and it can help you solve various problems and tasks, such as checking for divisibility, finding the last digit of a number, or cycling through an array. However, many JavaScript developers are confused about how the modulus operator works, especially when it comes to negative numbers or decimals.
In this blog post, we’ll dive into:
- What is modulus operator?
- How to use modulus in JavaScript?
- How it works with negative and decimal numbers?
- Seven real-time use case example of modulo operator
Let’s dive deep!
What is JavaScript modulus operator (%)?
The modulus operator (%) takes two operands, a dividend and a divisor, and returns the remainder of the division. The JavaScript modulus operator (%)
returns the remainder of its division.
Here is the syntax for using modulus:
result = dividend % divisor;
How to use modulus in JavaScript?
For example, if we divide 13 by 3
we get a quotient of 4 with a remainder of 1. The modulus operator would return the remainder, which is 1.
Here’s how we can use the JavaScript modulus example to calculate the reminder:
let dividend = 13;
let divisor = 3;
let remainder = dividend % divisor;
console.log(remainder);
// Output: 1
// Few more examples
console.log(12 % 5); // Output: 2
console.log(7 % 3); // Output: 1
console.log(4 % 2); // Output: 0
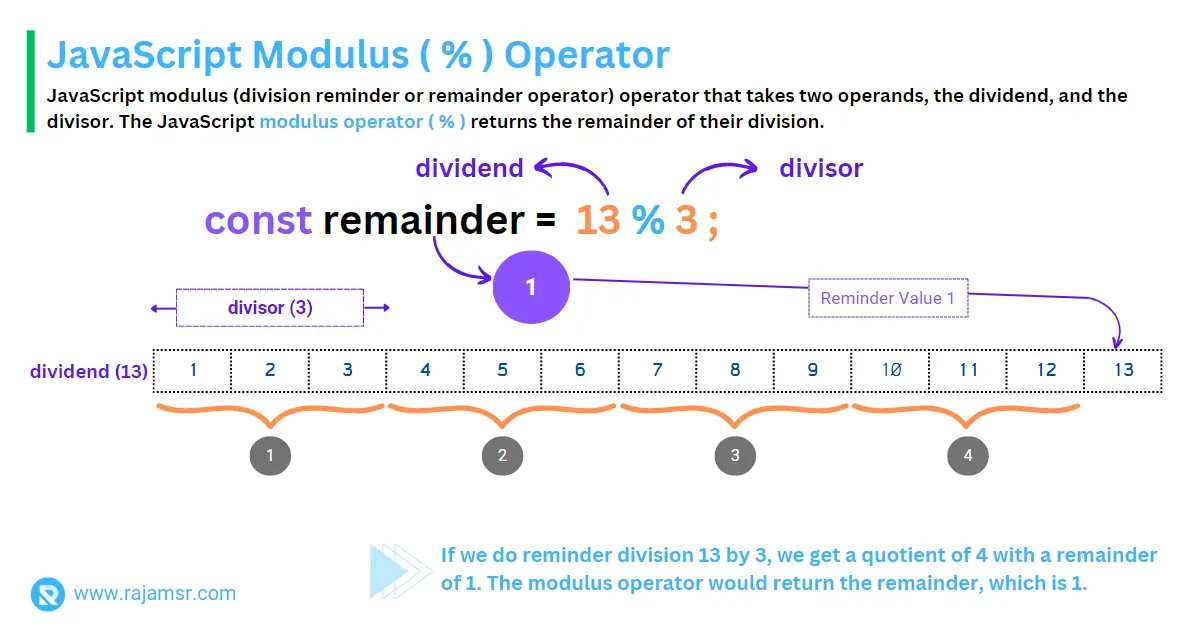
In this example, both the dividend and the divisor are integers. So this is an integer division.
JavaScript modulus operator with negative numbers
The modulus operator can be used with both positive and negative numbers, but the sign of the result depends on the sign of the dividend. Here’s an example with a negative number:
console.log(-12 % 5); // Output: -2
console.log(7 % -3); // Output: 1
console.log(-4 % -2); // Output: 0
JavaScript modulus operator with non-integer numbers
The modulus operator can also be used with non-integer numbers, such as decimals or JavaScript floating-point numbers, but the result may not be what you expect. For example:
console.log(12.5 % 5); // Output: 2.5
console.log(7.5 % 3); // Output: 1.5
console.log(4.5 % 2); // Output: 0.5
This is because the modulus operator does not return the fractional part of the division, but the remainder after rounding the operands to the nearest integer. For example:
console.log(12.5 % 5); // Output: 2.5 (Equivalent to 13 % 5)
console.log(7.5 % 3); // Output: 1.5 (Equivalent to 8 % 3)
console.log(4.5 % 2); // Output: 0.5 (Equivalent to 5 % 2)
If you want to get the fractional part of the division, you can use the JavaScript Math.floor() function to round down the operands before applying the modulus operator. For example:
console.log(Math.floor(12.5) % 5); // Output: 2
console.log(Math.floor(7.5) % 3); // Output: 1
console.log(Math.floor(4.5) % 2); // Output: 0
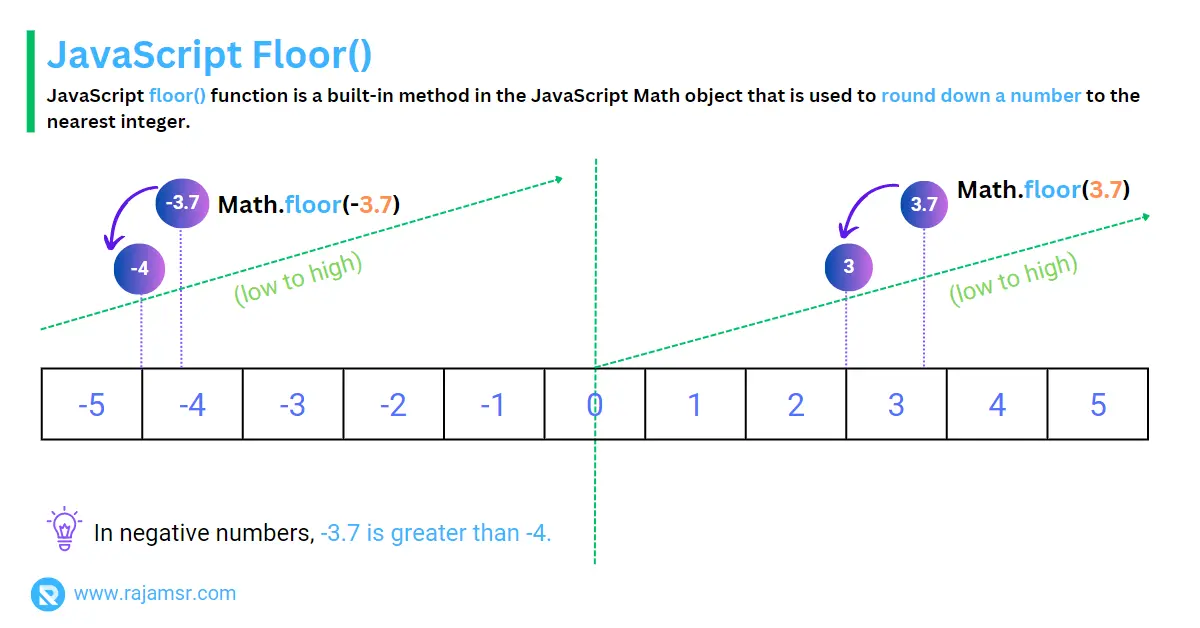
Applications of modulo operator in JavaScript
Modulus has a variety of use cases in programming languages. Here are some of the most common applications of the modulus operator in real-time use cases:
1. Checking if a number is even or odd using modulus operator
Modulus can be used to check if a number is even or odd. If a number is even (divided without remainder), its remainder when divided by 2 will be 0. If a number is odd, its remainder when divided by 2 will be 1.
Here’s an example of using modulus to check if a number is odd or even:
function isEvenNumber(number) {
return number % 2 === 0;
}
console.log(isEvenNumber(8));
// Output: true
console.log(isEvenNumber(9));
// Output: false
2. Finding multiples of a number using mod operator in JavaScript
Modulus in JavaScript can be used to find multiples of a number. If a number is a multiple of another number, its remainder when divided by that number will be 0. Here’s an example of using modulus to find multiples of a number:
function isMultiplesOf(number, divisor) {
return number % divisor === 0;
}
console.log(isMultiplesOf(8, 2));
// Output: true
console.log(isMultiplesOf(5, 2));
// Output: false
In the above example, 8 modulus 2 divides without remainder, so 8 is a multiple of 2.
3. Implementing circular arrays
Modulo (%) can be used to implement circular arrays
. An array is one of the non-primitive data types in JavaScript. A circular array is an array that wraps around when you reach the end of the array. This is useful when you click through a set of values circularly.
Here’s an example of using modulus to implement a circular array:
let severities = ['Blocker', 'Critical', 'Major'];
let index = 0;
function getNextSeverity() {
let severity = severities[index];
index = (index + 1) % severities.length;
return severity;
}
console.log(getNextSeverity());
// Output: "Blocker"
console.log(getNextSeverity());
// Output: "Critical"
console.log(getNextSeverity());
// Output: "Major"
console.log(getNextSeverity());
// Output: "Blocker"
4. Implementing hashing algorithms
Modulus can be used to implement hashing algorithms. A hashing algorithm is a function that takes a piece of data and returns a fixed-size JavaScript string or number. Hashing algorithms are often used to index data in databases or to validate passwords.
Here’s an example of using modulus to implement a hashing algorithm:
function hash(value) {
let hash = 0;
for (let index = 0; index < value.length; index++) {
hash = (hash << 5) - hash + value.charCodeAt(index);
}
return Math.abs(hash) % 10000;
}
console.log(hash("JavaScript Modulus"));
// Output: 2322 (Random integer between 0 and 9999)
In the above example, the left shift (<<) operator, charCodeAt()
and modulus operators are used to compute the hash value. Math.abs()
function used to get the JavaScript absolute value of the hash value.
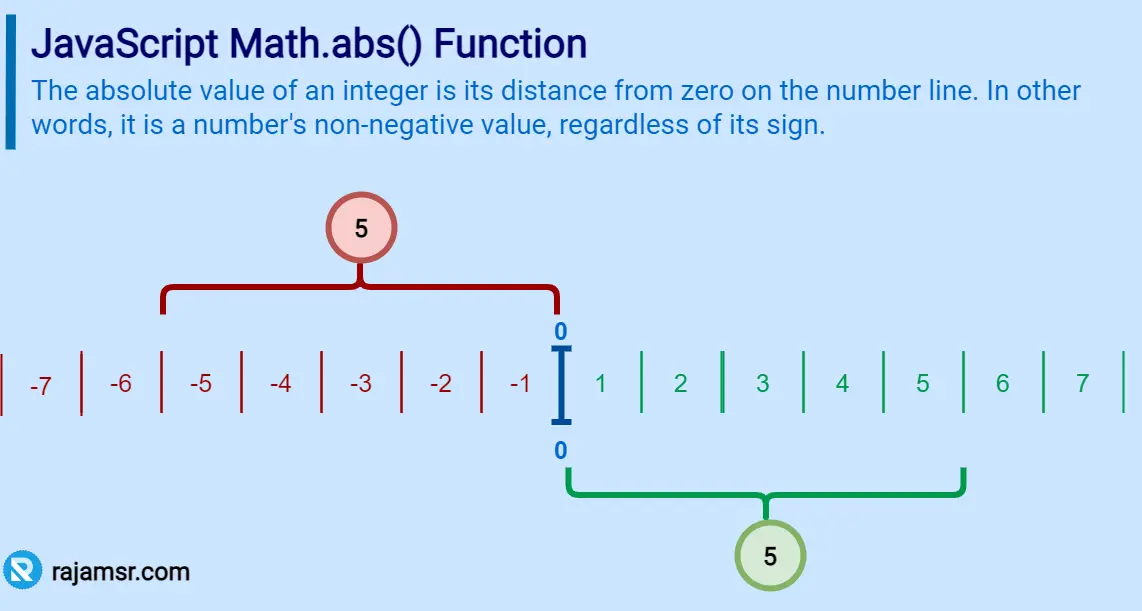
5 JavaScript modulus with two conditions
In some situations, we need to use the modulus operator with multiple conditions. Here’s an example of how you can use the modulus operator with two conditions
:
function checkDivisibility(number) {
switch(number)
{
case (number % 3 === 0 && number % 5 === 0):
console.log(number + " is divisible by both 3 and 5");
break;
case (number % 3 === 0):
console.log(number + " is divisible by 3");
break;
case (number % 5 === 0):
console.log(number + " is divisible by 5");
break;
default:
console.log(number + " is not divisible by either 3 or 5");
break;
}
}
checkDivisibility(15); // Output: "15 is divisible by both 3 and 5"
checkDivisibility(9); // Output: "9 is divisible by 3"
checkDivisibility(10); // Output: "10 is divisible by 5"
checkDivisibility(7); // Output: "7 is not divisible by either 3 or 5"
In this example, we define a function checkDivisibility()
that takes a number as input. We then use the modulus operator to check if num is divisible by 3 and 5, only by 3, only by 5, or by neither.
We use a switch statement to determine the appropriate message to output based on the conditions that are met. Finally, we call the function with different numbers to test the logic.
6. Use of modulus in date and time calculations
The modulus operator (%)
in JavaScript can be used in JavaScript Date and time calculations. For example, if you want to calculate the number of days between two dates
, you can use modulus to get the remainder of the division by 7 (number of days), which gives you the number of days that fall on a particular day of the week.
Here’s an example of using modulus to calculate the number of days between two dates:
function getDaysBetweenDates(startDate, endDate) {
// Hours * minutes * seconds * milliseconds
const milliSecondsPerDay = 24 * 60 * 60 * 1000;
const diffDays = Math.round(Math.abs((startDate - endDate) / milliSecondsPerDay));
const dayOfWeek = diffDays % 7;
return { diffDays, dayOfWeek };
}
const startDate = new Date('2023-01-01');
const endDate = new Date('2023-02-15');
const { diffDays, dayOfWeek } = getDaysBetweenDates(startDate, endDate);
console.log(`There are ${diffDays} days between ${startDate.toDateString()} and ${endDate.toDateString()}`)
// Output: "There are 45 days between Sun Jan 01 2023 and Wed Feb 15 2023"
console.log(`The ${dayOfWeek}rd day of the week.`);
// Output:The 3th day of the week.
7. Data pagination using modulus operator
Using the modulus operator, you can also implement pagination that wraps around the beginning of the data set when the last page is reached. For example, to display items 4-6
of the array after displaying pages 1 (from 1 to 3
), you can use the following code:
const bookTitles = [
"JavaScript - The Good Parts",
"Eloquent JavaScript",
"You Don't Know JS",
"JavaScript and JQuery",
"Learning JavaScript Design Patterns",
"JavaScript -The Definitive Guide",
"JavaScript Pocket Reference"
];
const itemsPerPage = 3;
const currentPage = 2;
const startIndex = ((currentPage - 1) * itemsPerPage) % bookTitles.length;
const endIndex = (startIndex + itemsPerPage) % bookTitles.length;
const currentPageItems = bookTitles.slice(startIndex, endIndex);
console.log(currentPageItems)
// Output: ["JavaScript and JQuery",
"Learning JavaScript Design Patterns",
"JavaScript -The Definitive Guide"]
In the above code, the JavaScript array length property with the modulus operator ensures that the index wraps around the beginning of the array when it reaches the end.
Frequently asked questions
In JavaScript, the modulus (%) operator
returns the remainder when one number is divided by another. It’s often used to check if a number is divisible by another.
Modulus works by dividing one number by another and returning the remainder. Here’s an example:
const result = 10 % 3; console.log(result)
// Output: 1 (10 divided by 3, leaves a remainder of 1)
const dividend = 15; const divisor = 7; const remainder = dividend % divisor; console.log(`The remainder is: ${remainder}`);
const changeCounter = 10 % 5; // changeCounter will be 0 (no change is needed)
You can use modulus in a forEach()
loop to perform actions based on the index. For example, if you want to execute code every 3rd iteration:
const array = [1, 2, 3, 4, 5, 6, 7, 8, 9]; array.forEach((element, index) => { if (index % 3 === 0) { console.log(`Element at index ${index}: ${element}`); } }); /* Output: Element at index 0: 1 Element at index 3: 4 Element at index 6: 7 */
Conclusion
In this blog post, we have learned about the JavaScript modulus operator(%)
and how it can be used to perform various calculations and operations on numbers. We have seen some examples of how the modulus operator can help us find the remainder of a division, check for even or odd numbers, create patterns and cycles, and more.
For which scenario are you going to use the JavaScript modulus operator?