join()
method is working? This is a common task that can be tricky to accomplish without the right method.In this blog post, I will show you how to:- Concatenate arrays with various separators in JavaScript
- Converting numeric arrays into strings
- Stringifying arrays in JavaScript
- Inserting spaces between array elements
- Using newline characters to join arrays
- Concatenating arrays of objects in JavaScript
- Using dynamic values as separators for array join
- Improving performance for large arrays
- Creating custom string templates
JavaScript array
A JavaScript array is one of the non-primitive data types, that is used to store data. An array can hold values of mixed types and use numbered indices starting from 0 to access specific items.
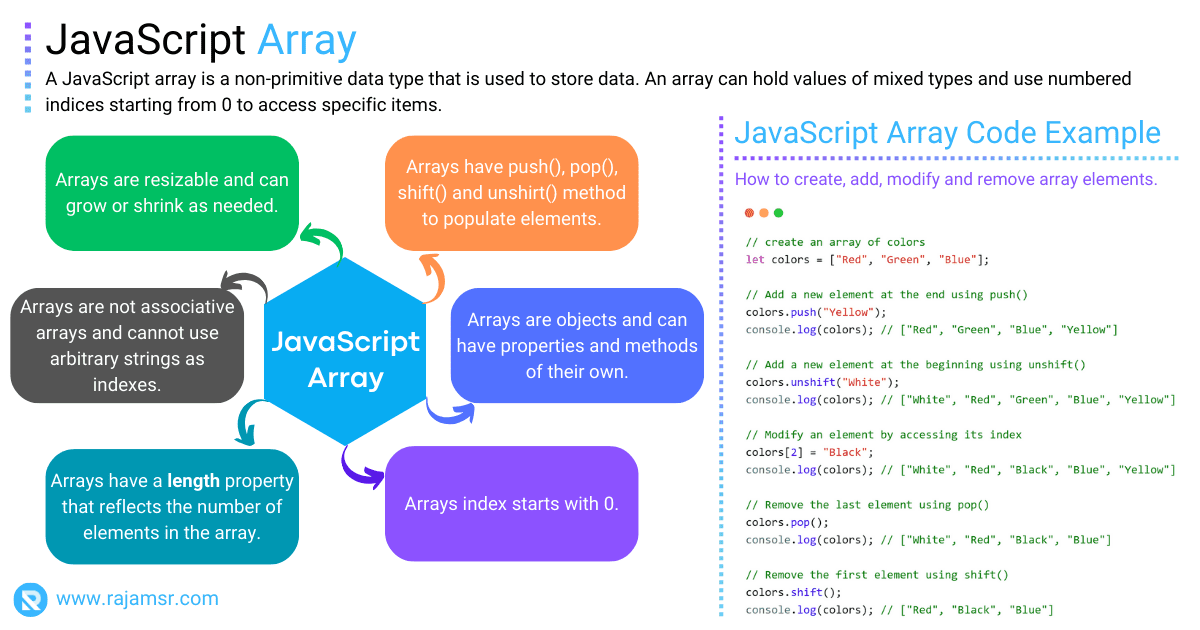
JavaScript array join() method
To begin, let’s explore the basics of the join()
method.
const tags = ["head", "title", "body"];
const commaSeparated = tags.join();
console.log(commaSeparated);
// Output: "head,title,body"
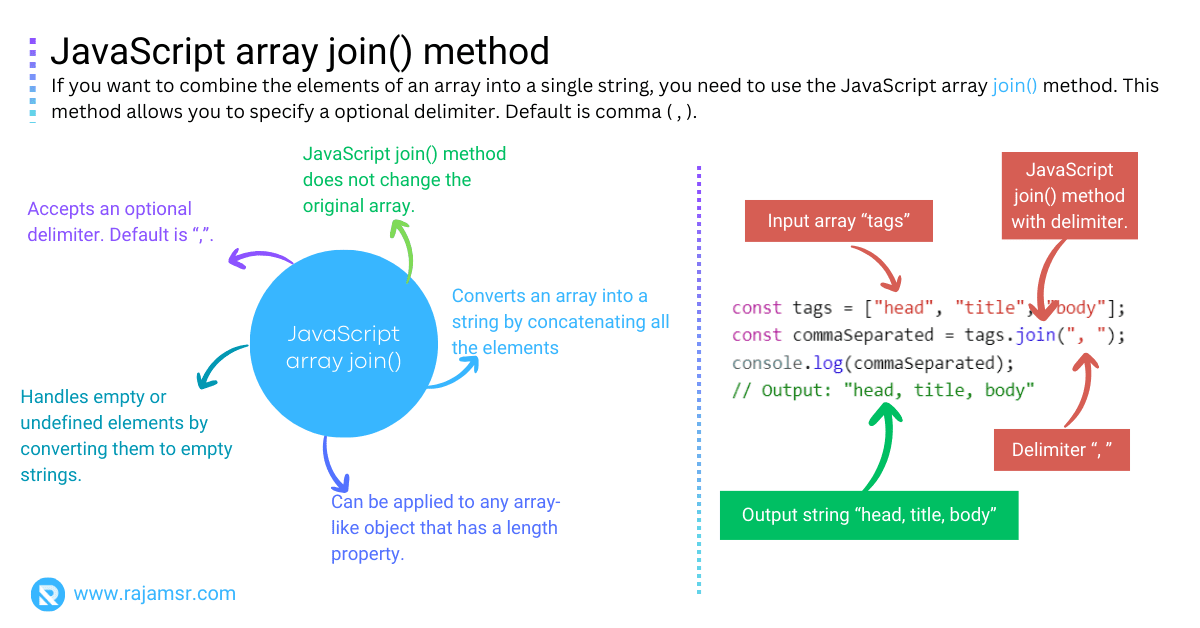
This code snippet is used join()
without specifying a separator. Default delimiter is comma
. The output is a string: "head,title,body"
. Simple, right?
Joining arrays with different delimiters
1. JavaScript array join with comma
If you want to combine the elements of an array into a single string, you need to use the JavaScript array join() method. This method allows you to specify a separator, such as a comma, that will be inserted between each element of the array.
const tags = ["head", "title", "body"];
const commaSeparated = tags.join(',');
console.log(commaSeparated);
// Output: "head,title,body"
Here, we use join(',')
to concatenate array elements with commas. Resulting in "head,title,body"
. Ideal for creating CSV
like strings.
2. Join number arrays to string
You can convert an array of numbers to a string using the join()
method. Here is an example:
const numbers = [1, 2, 3];
const joinedString = numbers.join('');
console.log(joinedString);
// Output: 123
By using join('')
, we create a string without any separators. For this numbers
array, the output is "123"
. Useful when a concatenated string without spaces or characters is needed.
3. JavaScript array join to string
One of the most useful features of the join()
method is the ability to customize the separator between the elements. This allows you to create different kinds of strings from the same array, depending on your needs and preferences.
const tags = ["head", "title", "body"];
const commaSeparated = tags.join('-');
console.log(commaSeparated);
// Output: "head-title-body"
The join('-')
method lets us customize the separator. In this case, the output is "head-title-body"
. You can customize it to your specific needs.
Adding spaces in array join
1. Joining arrays with space separator
space separator
is a common task in many programming languages. It allows you to combine multiple elements into a single string, with a space character between each element.const tags = ["head", "title", "body"];
const commaSeparated = tags.join(' ');
console.log(commaSeparated);
// Output: "head title body"
join(' ')
allows us to include spaces between array elements. Resulting in the string "head title body"
. Useful for natural language outputs.2. Handling various scenarios with space-separated array join
const cities = ["New York", "San Francisco", "Los Angeles"];
const complexSeparated = cities.join(', ');
console.log(complexSeparated);
// Output: "New York, San Francisco, Los Angeles"
join(', ')
combines array elements with a comma and a space
, resulting in "New York, San Francisco, Los Angeles"
. Perfect for list formatting.Array join with newlines
join()
method with a newline character (\n)
as the separator, and then add another newline character at the end of the string. This way, you can create a clear output that displays each element of the array on a separate line.const pageStructure = ["Header", "Body", "Footer"];
const newlineSeparated = pageStructure.join('\n');
console.log(newlineSeparated);
/* Output:
Header
Body
Footer
*/
Ideal for formatting text blocks or generating JavaScript multiline strings.
Joining arrays of objects
join()
method can still be your ally in such scenarios.const employees = [
{ name: 'Alice', role: 'Developer' },
{ name: 'Bob', role: 'Designer' },
{ name: 'Charlie', role: 'Manager' }
];
const employeeString = employees.map(e => `${e.name} - ${e.role}`).join('\n');
console.log(employeeString);
/* Output:
Alice - Developer
Bob - Designer
Charlie - Manager
*/
Here, we use map()
to transform each object into a string and then employ join('\n')
to create a multiline string. This is a powerful technique for generating reports or string logs.
Joining arrays with dynamic separators
What if you need to handle scenarios where the separator changes dynamically? JavaScript’s flexibility comes to the rescue.
const tags = ["head", "title", "body"];
const delimiter = (Math.random() > 0.5) ? ',' : '-';
const commaSeparated = tags.join(delimiter);
console.log(commaSeparated);
// Output: "head-title-body"
join()
method to meet dynamic requirements.Optimizing for large arrays
Handling large arrays efficiently is crucial for performance. JavaScript join()
method is well-suited for such scenarios.
const largeArray = new Array(1000000).fill('item');
const startTime = performance.now();
const largeString = largeArray.join(',');
const endTime = performance.now();
console.log(`Time taken: ${endTime - startTime} milliseconds`);
// Output: "Time taken: 27.799999982118607 milliseconds"
Here, we create a large array and measure the time it takes to join its elements with a comma. JavaScript array join() method excels in these situations, providing optimized performance.
Creating custom string templates
join()
is not limited to simple separators; you can create custom string templates
tailored to your needs.const users = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
{ name: 'Doe', age: 22 }
];
const userTemplate = users
.map(u => `Name: ${u.name}, Age: ${u.age}`)
.join('\n');
console.log(userTemplate);
/* Output:
Name: John, Age: 30
Name: Jane, Age: 25
Name: Doe, Age: 22
*/
In this example, we construct a custom string template for each user and then use join('\n')
to create a formatted multiline string.
Conclusion
As you can see, JavaScript array join() is a powerful method that allows you to manipulate and combine arrays in different ways.
You can use it to create strings from arrays, join arrays with different separators, or even create nested arrays. Whether you are working on web development or data analysis JavaScript array join() method can help you achieve your goals faster and easier
Over to you: how you are going to use array join() method?