If you are a web developer, you have probably used the JavaScript join()
method at some point. But do you know what it does and how it works?
The JavaScript join()
method is a built-in array method in JavaScript. The join()
method joins all elements of an array into a single string. A specified separator separates the resulting string.
In this article, we will explore:
- The basics of the JavaScript
join()
method - How it can help you manipulate arrays and strings
- Advanced usage of
join()
methods - Common use cases and examples.
Whether you are a beginner or an experienced developer, you will find something useful and interesting in this article.
Definition of the join() method
Join()
is one of the JavaScript array methods that takes an array and converts it into a string. One optional argument can be passed to the join()
method. The separator that should be used to separate the elements in the resulting string is this argument. The default separator is a comma (,)
.
For example, you can use the join()
method to format data for output, concatenate strings, or create delimited strings for data storage.
Here is the basic syntax of the JavaScript join()
method:
array.join(separator);
To convert an array into a string, use the name of the array, followed by a separator. The separator is optional
and specifies how to separate the elements in the resulting string.
Joining arrays into strings
1. Basic usage of the join() method
Here is a simple example of using the join()
method to convert an array into a string:
let days = ["Sunday", "Monday", "Tueday", "Wednesday"];
let daysString = days.join();
console.log(daysString);
// Output: "Sunday,Monday,Tueday,Wednesday"
In this example, we have an array of day names
that we want to convert into a string.
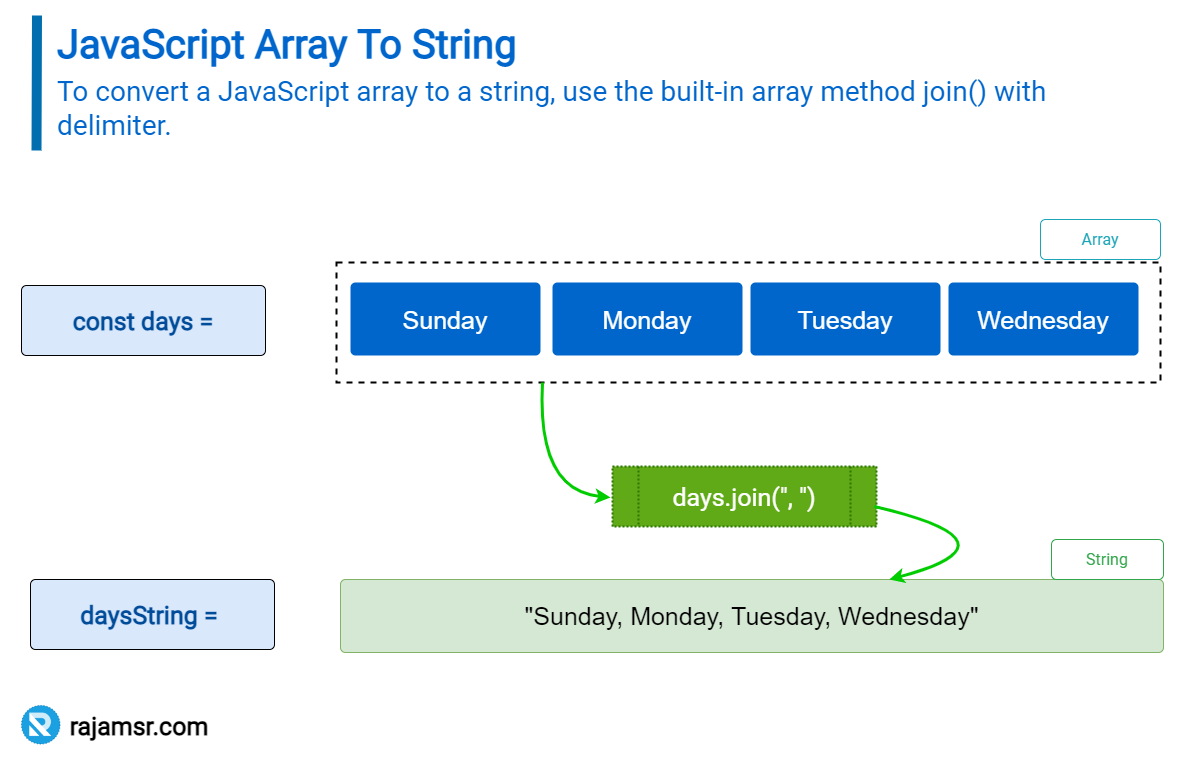
2. Custom separators in the join() method
You can also use a custom separator in the resulting string. Here is an example that uses a custom separator
:
let months = ["Jan", "Feb", "Mar", "Apr"];
let result = months.join(' | ');
console.log(result);
// Output: "Jan | Feb | Mar | Apr"
We use the " | "
string as the separator in this example. We log the resulting string to the console.
3. Examples of using the join() method to join arrays into strings
Here is an example of how to convert an array of numbers into a string using the join()
method:
let numbers = [1, 2, 3, 4, 5];
let result = numbers.join('');
console.log(result);
// Output: "12345"
Advanced usage of the join() method
Join method with nested arrays
We can use the join()
method with nested arrays as well. Here is an example:
let nestedArray = [
["Jan", "Feb"],
["Mar", "Apr"]
];
let result = nestedArray.join(", ");
console.log(result);
// Output: "Jan,Feb, Mar,Apr"
In this example, we have a nested array
, which is an array of arrays. We use the join()
method to convert the nested array into a string. Then, we log the resulting string to the console.
Join method with objects
The join()
method can also be used with arrays of objects. However, since objects do not have a default string representation, you need to specify how the objects should be represented in the resulting string.
Here is an example:
let people = [{
name: "John",
age: 30
},
{
name: "Jane",
age: 25
},
{
name: "Jim",
age: 35
}
];
let result = people.map(person => person.name).join(", ");
console.log(result);
// Output: "John, Jane, Jim"
In this example, we have an array of objects that represent people
. We use the map()
method to extract the 'name'
property of each person object and then use the join()
method to convert the resulting array of names into a string. The resulting string is printed to the console.
Performance considerations of the join() method
It is important to note that the join method is an efficient way to manipulate arrays. However, you should know the performance implications of using the join()
method, especially when working with large arrays.
The join()
method has a linear time complexity, which means that it takes longer to process as the size of the array increases.
If you need to work with vast arrays, you should consider using alternative methods, such as String.concat(), with constant time complexity.
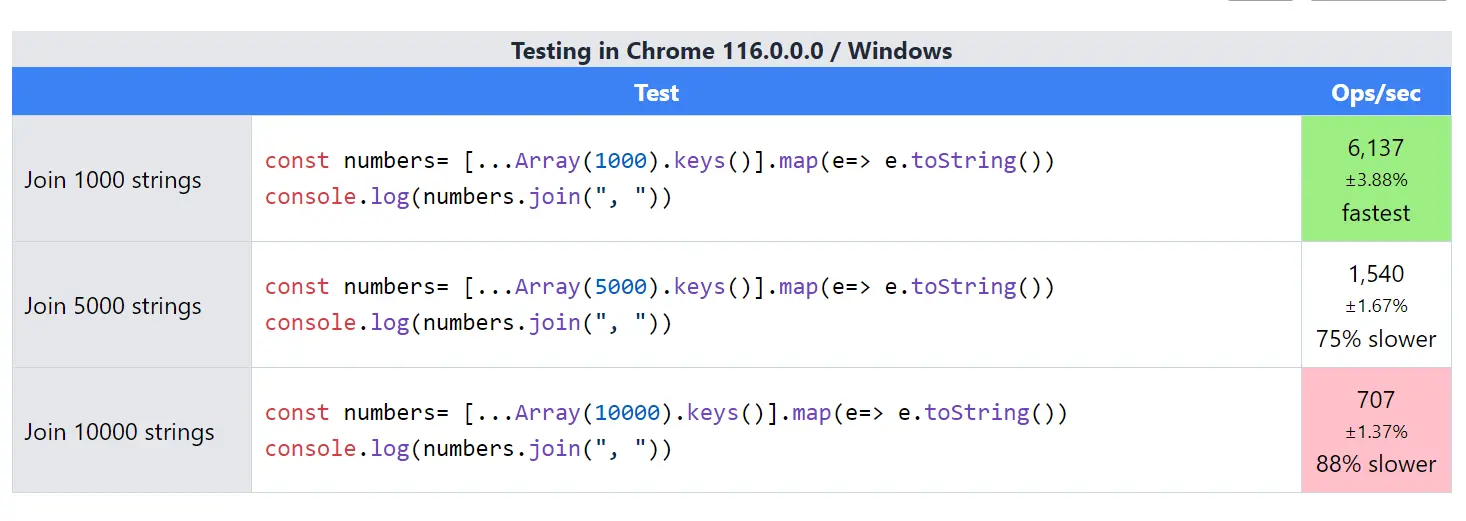
The results show that the performance of the JavaScript join()
method decreases as the size of the array increases.
For 1000 elements
, the performance achieved 6137
operations per second, but for 5000
and 10000
elements, it only achieved 1540
and 707
operations per second, respectively.
Common use cases for the join() method
1. Formatting data for output
One of the most common use cases for the join()
method is to format data for output. For example, you might want to convert an array of names into a comma-separated string that can be displayed on a web page.
Here is an example:
let names = ["Jan", "Feb", "Mar"];
let result = names.join(", ");
document.getElementById("output").innerHTML = result;
In this example, we use the join()
method to convert an array of names into a string, and then use the "innerHTML"
property to insert the string into an HTML element with the 'id'
of 'output'
.
2. Concatenating strings
join()
method is to concatenate strings. For example, you might want to concatenate a list of names into a single string that represents a greeting.Here is an example:let names = ["John", "Jane", "Jim"];
let result = "Hello, " + names.join(", ") + "!";
console.log(result);
// Output: "Hello, John, Jane, Jim!"
In this example, we use the join()
method to convert an array of names into a string, and then use string concatenation to create a greeting. The resulting string is logged to the console.
JavaScript join() frequently asked questions
concat()
method to join multiple strings.const str1 = "Hello"; const str2 = " "; const str3 = "World"; const result = str1.concat(str2, str3); console.log(result); // Output: Hello World
Yes, you can merge objects using the Object.assign() method.
const obj1 = { a: 1, b: 2 }; const obj2 = { b: 3, c: 4 }; const mergedObject = Object.assign({}, obj1, obj2); console.log(mergedObject); // Output: { a: 1, b: 3, c: 4 }
You can use the join method to concatenate the elements of an array into a single string.
const stringArray = ["Hello", " ", "World"]; const result = stringArray.join(""); console.log(result); // Output: Hello World
Conclusion
In conclusion, the JavaScript join()
method is an incredibly useful method for working with arrays. It allows you to convert arrays into strings, which can be useful for formatting data for output, concatenating strings, and creating delimited strings for data storage.
With its linear time complexity, the join()
method is efficient for most arrays, but it is important to be aware of performance implications when working with large arrays.
If you want to convert an array into a string, the join()
method is an excellent option that is easy to use and can help you simplify your code.
Whether you’re formatting data for output, concatenating strings, or creating delimited strings for data storage, the join()
method is the best option.