Are you trying to convert a JavaScript array to a string? Look no further. JavaScript arrays let you work with many values at once. But what if you need to convert the array to a string? Maybe you want to send some data to a server for persistence or display it on the screen.
In this article, we will explore the following:
- Convert JavaScript arrays to strings in three different ways
- Convert to string with comma
- Convert to string without comma
- Convert array to string with newline as a delimiter
- Convert string to an array
Let’s see it in detail.
Convert JavaScript array to string in three different ways
Sometimes, you need to convert the array to a string in JavaScript. In this post, let’s see three different approaches.
1. JavaScript array to string using join() method
This is one of the simple methods. Using the built-in join() JavaScript array methods you can convert an array to a string. Array elements are enclosed in square brackets. For example:
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday'];
const daysString = days.join();
console.log(daysString)
// Output: "Sunday,Monday,Tuesday,Wednesday"
JavaScript string concatenation is the process of joining one or more strings into a single string. In this example, the join()
method is used to concatenate the elements of the array into a single JavaScript string.
By default, the join()
method uses a comma as an array element separator. The problem with this default parameter is that there won’t be any space after the comma. But this can be easily fixed by passing parameters explicitly.
You can also use other characters as a delimiter for the join()
method; you will see more details in later sections.
2. Array to string using toString() method
The JavaScript toString() method converts the array object into a string representation of the array. This method is similar to join()
, but the only difference is that it does not allow you to provide a separator. For Example:
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday'];
console.log(days.toString())
// Output: "Sunday,Monday,Tuesday,Wednesday"
JavaScript object toString()
method will work only if array elements are primitive data types, such as number
, string
, and boolean
.
One disadvantage of using toString()
on an array of objects is that it will return [object Object]
instead of a string representation.
3. Using the JSON.stringify() method
The JSON.stringify()
method converts any JavaScript object to a JSON
format string. You can pass an array to the JSON.stringify()
method, which will return the JSON
string representation of the array.
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday'];
const daysString = JSON.stringify(days);
console.log(daysString)
// Output: ["Sunday","Monday","Tuesday","Wednesday"]
The resulting string is a JSON string. It can be parsed back to an array using the JSON.parse()
method. One of the main advantages of this approach is that it is not just a string or number array; it will convert any JavaScript object to a string.
Converting JavaScript arrays into strings with comma
The simplest way to convert a JavaScript array to a string separated by a comma delimiter
between each element. You can achieve this by using the join()
method with ", "
as a delimiter.
For example:
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday'];
const daysString = days.join(', ');
console.log(daysString)
// Output: "Sunday, Monday, Tuesday, Wednesday"
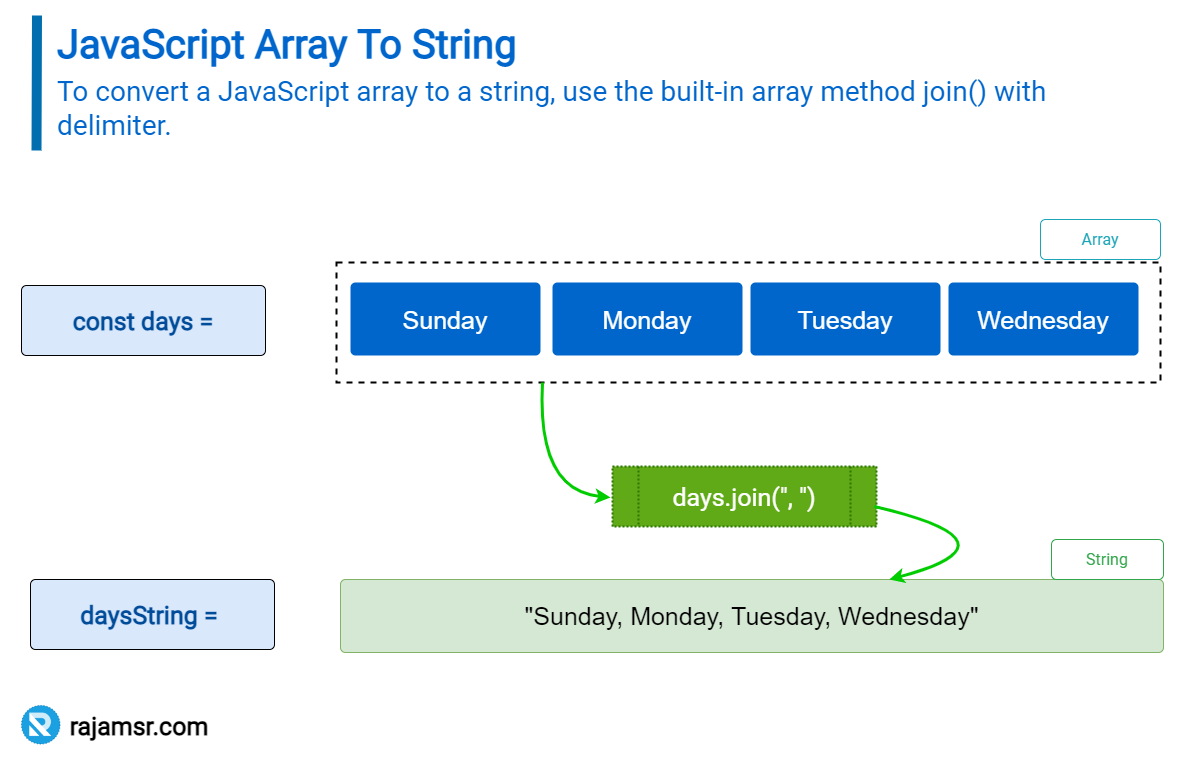
The benefit of using custom commas (', ')
as separators is that it will allow you to add a space after the comma. It makes array-to-string
conversion easy to use.
However, there is a limitation to using commas as the delimiter in some scenarios. For example, using commas as a delimiter may not be suitable when you are handling strings that already contain commas.
For example:
const personalInfo = ['John Doe', '25, Main St, New York', 'johndoe@example.com'];
const personalInfoString = personalInfo.join();
console.log(personalInfoString)
// Output: "John Doe,25, Main St, New York,johndoe@example.com"
How to convert array to string in JavaScript without commas
If you prefer to convert a JavaScript array to a string without using default commas, you can do this by specifying an empty string as a join()
method delimiter.
For Example:
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday'];
const daysString = days.join(' ');
console.log(daysString)
// Output: "Sunday Monday Tuesday Wednesday"
The benefit of using no separator
is that it provides a more natural flow of the elements in the array. It may be more suitable for certain situations. However, there is a limitation. It is difficult to parse the string back into an array object. Especially when the array elements are not of the same data type.
Converting JavaScript arrays to strings with delimiter
What if you wanted to use a delimiter other than a comma
? In JavaScript, the JavaScript join() method accepts any string as a delimiter. For example, you can use a delimiter of your preference, such as a hyphen
, pipe
, or colon
.
The following example uses pipe (|)
as a delimiter:
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday'];
const daysString = days.join('| ');
console.log(daysString)
// Output: "Sunday| Monday| Tuesday| Wednesday"
Converting JavaScript arrays to strings with line breaks
Sometimes you need to display array data in a readable format. You can use line breaks instead of commas or other delimiters to display array elements in a readable format. There are different ways to create JavaScript multiline strings.
The following example uses a newline (\n)
as a delimiter to display array elements in a more readable format:
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday'];
const daysString = days.join('\n');
console.log(daysString)
// Output:
"Sunday"
"Monday"
"Tuesday"
"Wednesday"
The benefit of using line breaks is that it can make the data more readable for humans. Especially when dealing with large arrays. However, a limitation is that it may not be suitable when dealing with structured data that requires consistent formatting.
Till now, we have seen different use cases for how to convert an array to a string and how to use different delimiters. But what if you want to convert a string to an array? Let’s see how to convert a string to an array.
Convert string to array in JavaScript
The JavaScript split()
string method allows you to convert a JavaScript string into an array of substrings. Optionally, you can specify a separator.
For example:
const message = 'JavaScript is a loosely typed language.'
const words = message.split(' ')
console.log(words)
// Output: ["JavaScript", "is", "a", "loosely", "typed","language."]
In the above example, empty space (' ')
is used as a delimiter for the split()
method. So each word is converted into an array element.
Conclusion
In conclusion, there are different ways to convert a JavaScript array to a string. In this article, we explored three different approaches.
You can use the join()
, toString()
, or JSON.stringy()
methods to convert a JavaScript array to a string, depending on your needs and requirements.
You can use commas as separators. Concatenate the array elements without any separators, use any custom delimiter, or separate the elements with line breaks using newline characters. The JavaScript string split()
method allows you to convert a string into an array of substrings.
Which approach are you going to follow to convert an array into a string?