Do you know how to use the JavaScript trim whitespace methods? If not, you are missing out on a simple and powerful way to manipulate strings in JavaScript. Whitespace is any character that creates space or separates words in a text, such as spaces, tabs, newlines, etc. Sometimes, you may want to remove whitespace from a string, either from the ends, from one end, or from anywhere in the string. This can help you validate user input, format output, compare strings, and more.
In this blog post, I will show you:
- What are the whitespace characters?
- Different ways to trim whitespace from a string in JavaScript
- Remove whitespaces from the start and end of a string with JavaScript
- How to remove whitespaces from only one side of a string
- How to eliminate whitespaces from any part of a string
- Comparing trim(), trimStart() and trimEnd() methods in JavaScript
- Practical examples of using trim() methods in JavaScript
By the end of this post, you will be able to trim whitespace from both ends, one end, or anywhere in a string with ease.
What are the whitespace characters in JavaScript?
Whitespace characters in JavaScript are any characters that create space or separate words in a text. They include:
- A space character ( )
- A tab character (\t)
- A carriage return character (\r)
- A new line character (\n)
- A vertical tab character (\v)
- A form feed character (\f)
- A no-break space character (\u00A0)
You can use the \s metacharacter in a regular expression to match any whitespace character. For example, /^\s+$/ matches a JavaScript string that contains only whitespace characters.
JavaScript trim whitespace from both ends of a string
trim()
method. This method removes whitespace from both sides of a string and returns a new string. For example:let name = " John Doe ";
let trimmedName = name.trim(); // Output: "John Doe"
The trim()
method does not change the original string, so you can use it without worrying about modifying your data. Also, the trim()
method considers whitespace as any white space character plus line terminators, such as spaces, tabs, newlines, etc.
However, the trim()
method is not supported by some older browsers, such as Internet Explorer 8 or below. If you need to support these browsers, you can use a polyfill
, which is a piece of code that provides the functionality that is missing in the browser.
How to trim whitespace from one end of a string
Sometimes, you may want to trim whitespace from only one end of a string, either from the beginning or from the end. For this, you can use the trimStart()
and trimEnd()
methods. These methods remove whitespace from the beginning or the end of a string respectively and return a new string.
For example for how to use trimStart() and trimEnd() methods:
let name = " John Doe ";
let trimmedNameStart = name.trimStart(); // Output: "John Doe "
let trimmedNameEnd = name.trimEnd(); // Output: " John Doe"
trimStart()
and trimEnd()
methods do not change the original string, so you can use them without worrying about modifying your data. Also, the trimStart()
and trimEnd()
methods consider whitespace as any white space character plus line terminators, such as spaces, tabs, newlines, etc.However, the trimStart()
and trimEnd()
methods are not supported by some older browsers, such as Internet Explorer or Edge 18 or below. If you need to support these browsers, you can use a polyfill
, which is a piece of code that provides the functionality that is missing in the browser.How to trim whitespace from anywhere in a string
replace()
method with a regular expression to remove whitespace from anywhere in a string and return a new string. For example:let name = " John \n Doe ";
let trimmedName = name.replace(/\s/g, ""); // Output: "JohnDoe"
The replace()
method does not change the original string, so you can use it without worrying about modifying your data. The regular expression /\s/g
matches any whitespace character (\s)
and replaces it with an empty string (”). The g
flag means that the replacement is done globally, that is, for all occurrences of whitespace in the string.
You can modify the regular expression to suit your needs, such as removing only leading or trailing whitespace, or removing multiple consecutive whitespace characters. For example:
let name = " John \n Doe ";
let trimmedNameStart = name.replace(/^\s+/, ""); // Output: "John \n Doe "
let trimmedNameEnd = name.replace(/\s+$/, ""); // Output: " John \n Doe"
let trimmedNameMultiple = name.replace(/\s+/g, " "); // Output: " John Doe "
What is the difference between trim(), trimStart() and trimEnd() methods?
The difference between trim()
, trimStart()
and trimEnd()
methods is that trim()
removes whitespace from both ends of a string, while trimStart()
and trimEnd()
remove whitespace from the beginning and end of a string respectively. For example:
let name = " John Doe ";
console.log(name.trim()); // Output: "John Doe"
console.log(name.trimStart()); // Output: "John Doe "
console.log(name.trimEnd()); // Output: " John Doe"
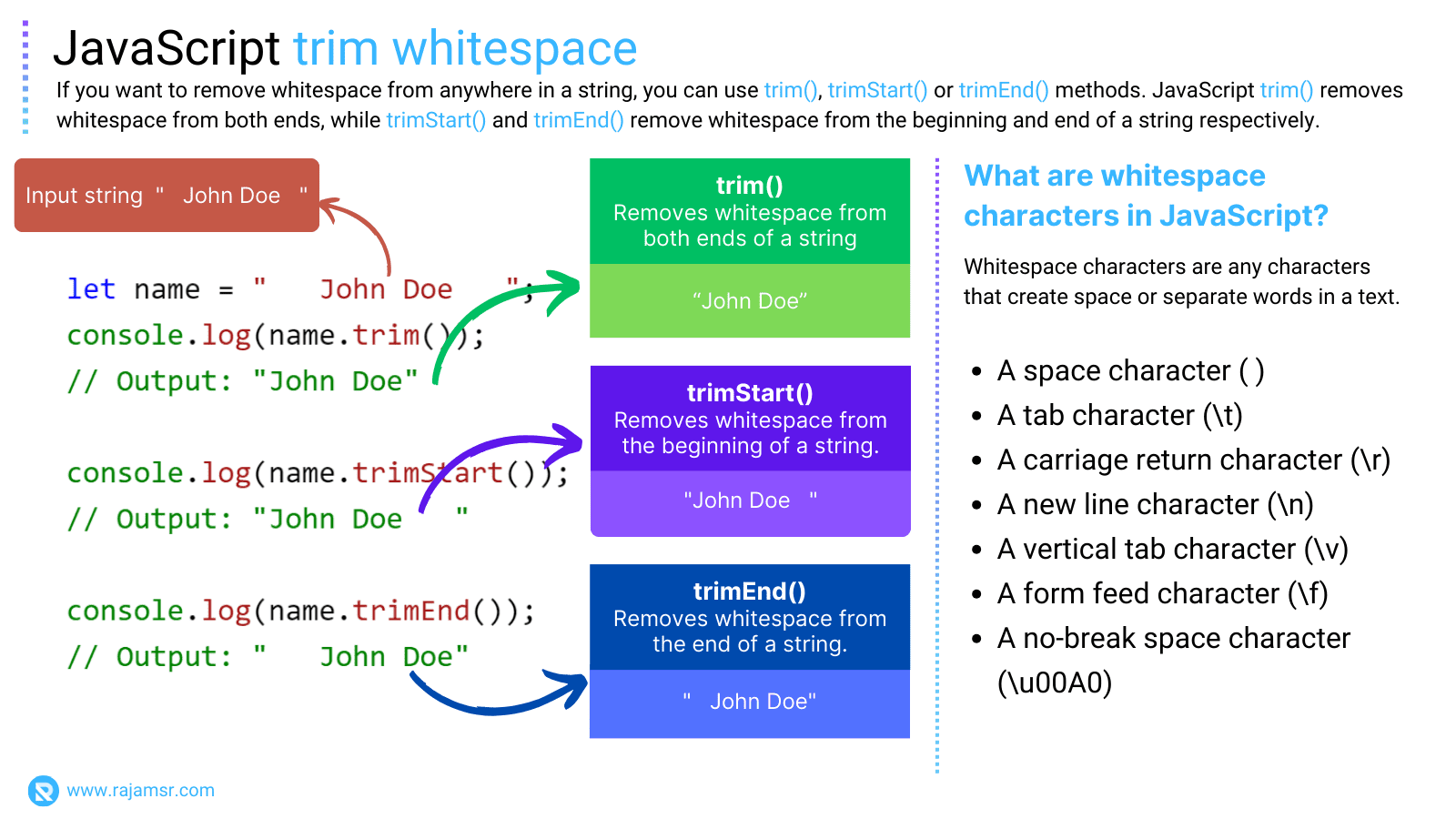
Sometimes you want to get rid of extra spaces or other things in your text. You can use some methods that will give you a new text without those things. But be careful, there are different methods for this, and some of them are better than others.
The best methods are called trimStart()
and trimEnd()
. They work well with other methods that add things to your text, like padStart()
and padEnd()
. But some old browsers don’t know these methods, so they won’t work there. If you want to use them anyway, you need to add some extra code that will teach the old browsers how to do it.
Usage of trim() methods in real time examples
trim()
methods.1. Trim string to length
If the JavaScript string length exceeds the specific length, truncate the remaining characters; otherwise, return the original string.
var originalString = " javascript trim string to length ";
let trimToLength = 25
let trimmedString = originalString.trim();
var truncatedString = trimmedString.length> trimToLength
? trimmedString.substring(0,trimToLength)+'...'
: trimmedString ;
console.log(truncatedString);
// Output: "javascript trim string to..."
2. JavaScript trim characters from a string
There is no built-in method in JavaScript for trimming particular characters or strings. Assume you’re working with comma-separated
values. You may need to remove the comma
at the end of the string. You can accomplish this by writing custom code, as shown in the accompanying example.
var originalString = "First Name, Last Name, Phone Number, ".trim();
var charToRemove = ','
// Trim last character if comma
var commaRemovedString = (originalString[originalString.length - 1] === charToRemove)
? originalString.substring(0, originalString.length - 1)
: originalString;
console.log(commaRemovedString)
// Output: "First Name, Last Name, Phone Number"
In this way, you can remove a specific character from the end of the string.
3. Trim all whitespaces
The built-in trim() method does not remove whitespace
between words. You can use the string method replaceAll() as shown in the following example, to remove whitespace
characters between words.
let message = 'This is JavaScript string.'
console.log(message.replaceAll(' ',''))
// Output: ThisisJavaScriptstring
4. Trim leading zeros
Assuming that you are reading data from an external source, such as a CSV file
, everything is treated as a string
, including numbers
. In some cases, people use numerals with leading zeros. If you want to remove leading zeros from a string, use the built-in Number
object, as shown in the following code example:
let message = '000182787.98'
console.log(Number(message))
// Output: 182787.98
Conclusion
In this blog post, I have shown you how to trim whitespace from a string in JavaScript using the trim(), trimStart(), trimEnd(), and replace() methods with regular expressions. These methods are easy to use and can help you handle whitespace in your strings more efficiently.
Here are some tips or best practices for trimming whitespace from strings in JavaScript:
Use the trim() method before comparing strings, as it can help you avoid false negatives or positives due to whitespace differences.
Use the replace() method with a global flag (g) to remove all whitespace occurrences in a string, not just the first one.
Test your regular expressions with online tools, such as [RegExr] or [Regex101], to make sure they work as expected.
I hope you found this blog post helpful and informative