JavaScript is a programming language that is widely used for creating interactive web applications. One of the essential concepts in JavaScript is string equality. String equality is the process of checking whether two JavaScript strings are equal or not.
In this article, we will explore various aspects of:
- JavaScript string equality
- How to check string equality in JavaScript
- The string equality operator
- Ignoring case in string comparison
- Common issues that cause string equality not to work and string equality testing
Let’s see each topic in detail.
JavaScript string equals
String equality in JavaScript means comparing two strings to see if they are equal or not. There are two types of string equality:
- Strict equality
- Loose equality
Strict equality
is when two JavaScript strings are identical in terms of their content and type. Loose equality
is when two strings are equal in terms of their content but not necessarily their type.
Here is an example of comparing strings using strict and loose equality:
let string1 = "Hello World";
let string2 = "Hello World";
console.log(string1 === string2);
// Output: true (strict equality)
console.log(string1 == string2);
// Output: true (loose equality)
Compare string in JavaScript using equality operator
The string equality operators are ==
and ===
. The ===
operator checks if the two strings are equal in both value and type, while the ==
operator checks if the two strings are equal in value only.
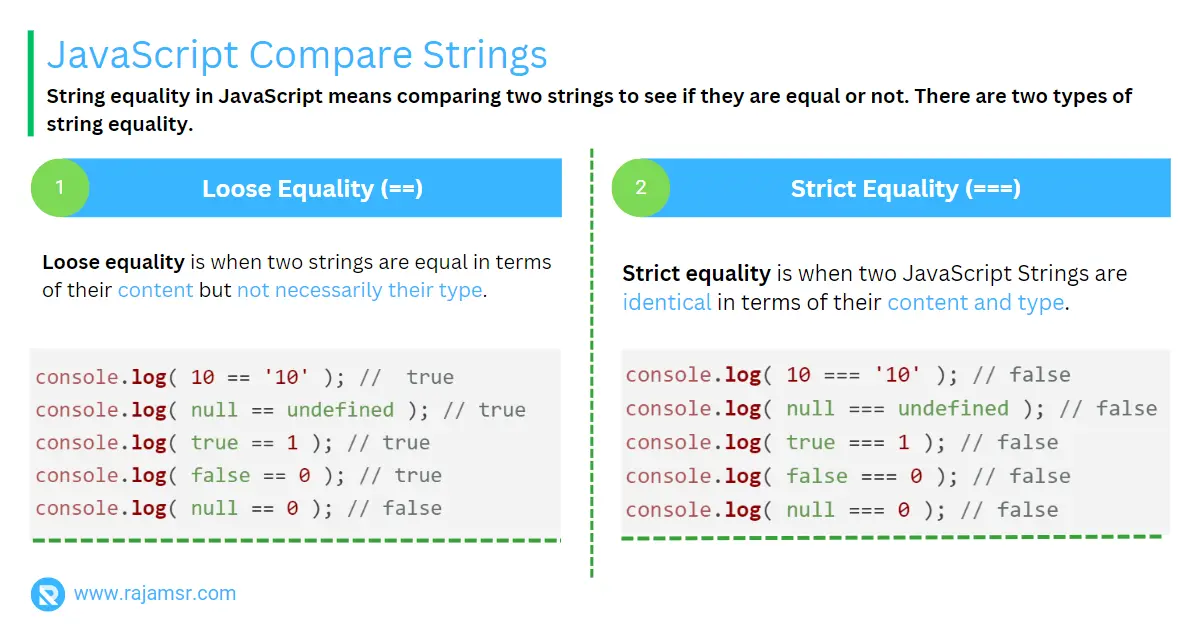
Compare string equals using loose equality (==)
==
operator to compare two values. It performs type coercion if the two values have different data types before the comparison.Let’s see how to compare strings in JavaScript using the loose equality operator:console.log( 10 == '10' ); // true
console.log( null == undefined ); // true
console.log( true == 1 ); // true
console.log( false == 0 ); // true
console.log( null == 0 ); // false
Compare string equals using strict equality (===)
Strict equality, also known as identity equality, uses the triple-equals ===
operator to compare two values. It compares the values and their data types without performing any type of coercion.
For example, JavaScript string equality checks using string equal (===
):
console.log( 10 === '10' ); // false
console.log( null === undefined ); // false
console.log( true === 1 ); // false
console.log( false === 0 ); // false
console.log( null === 0 ); // false
JavaScript string comparison
There are several methods for checking string equality in JavaScript. The most common method is the ===
or ==
operator to compare strings in JavaScript. The ===
operator checks if the two strings are equal in both value and type, while the ==
operator checks if the two strings are equal in value only.
Here is a string comparison example of using the ===
and ==
operators:
console.log("Hello" === "Hello"); // true
console.log("Hello" == "Hello"); // true
console.log("Hello" === "World"); // false
console.log("Hello" == "World"); // false
Compare string localeCompare() method
Another method for checking string equality is using the localeCompare()
method. The localeCompare() method compares two strings based on the current locale settings and returns a number.
The localeCompare()
method returns a number indicating if a reference string comes before
, after
, or is the same
as the given string in sort order.
Here is an example of string comparison using localeCompare()
:
// Negative when the reference string occurs before compare string.
// The letter "a" is before "b", so returning a negative value
console.log("a".localeCompare("b"));
// Output: -1
// Returns 0 if both are equivalent.
// The word "Hello" and "Hello" are equivalent, returning a neutral value of 0
console.log("Hello".localeCompare("Hello"));
// Output: 0
// Positive when the reference string occurs after compare string.
// Alphabetically the word "World" comes after "Hello" returning a positive value
console.log("World".localeCompare("Hello"));
// Output: 1
Note that localeCompare()
method will throw a “cannot read properties of undefined (reading ‘localecompare’)” exception if you apply it to a null
value.
const compareText = (message) => {
console.log(message.localeCompare("Hello"));
}
compareText()
// TypeError: Cannot read properties of undefined (reading 'localeCompare')
You can easily fix this by checking the input message variable value, as shown below:
const compareText = (message) => {
if (message)
console.log(message.localeCompare("Hello"));
}
String equality not working
Sometimes, string equality does not work due to several issues. The most common issue is that the strings are not the same.
It is essential to check the strings’ values and make sure that there are no extra spaces
or characters that could affect the equality check.
Another issue is the difference in character encoding
between the two strings, which can cause the equality check to fail. It is also possible that the variables holding the strings are not properly initialized or defined.
Here is an example of a common mistake that can cause string equality not to work:
let string1 = "JavaScript";
let string2 = "javascript";
console.log(string1 === string2);
// Output: false
String equality ignore case
Sometimes, it is necessary to ignore the case when checking for string equality. The toLowerCase()
method can be used to convert both strings to JavaScript lowercase before checking for equality.
Alternatively, you can convert both strings to JavaScript uppercase using the toUpperCase()
method.
Here is an example:
let string1 = "hello";
let string2 = "HELLO";
let string3 = "HeLlO";
console.log(string1.toLowerCase() === string2.toLowerCase()); // true
console.log(string1.toLowerCase() === string3.toLowerCase()); // true
console.log(string2.toLowerCase() === string3.toLowerCase()); // true
Equality check use case
String equality checks are essential in JavaScript applications. They are used to compare user input, validate passwords, and check if two values are equal. Here is an example of a string equality check:
let password = "password";
let confirmPassword = "password";
if (password === confirmPassword) {
console.log("Passwords match");
} else {
console.log("Passwords do not match");
}
In this example, we are checking if the password
and confirmPassword
JavaScript variables are equal. If they are equal, we log "Passwords match."
Otherwise, we log "Passwords do not match."
Conclusion
String equality is an essential concept in JavaScript. It is used to check if two strings are equal or not. There are several methods for checking string equality in JavaScript, such as using the ===
or ==
operator and the localeCompare()
method. It is also possible to ignore the case when checking for string equality using the toLowerCase()
method.
However, string equality can sometimes not work due to issues such as differences in character encoding, extra spaces or characters, and uninitialized variables.
String equality checks and tests are crucial in JavaScript applications to ensure the correctness of implementations.