- Using the
string.reverse()
method - Using a
for loop
to iterate over the characters - Using the
reduce()
method to accumulate the reversed string - Splitting the string into words and reversing each word
- Using
recursion
to reverse the string from the end - Concatenating the characters in reverse order without a function
- Converting the string to a number and reversing it
- Converting the string to an array and reversing it
1. Reverse string using the string.reverse() JavaScript method
The reverse()
method is one of the simplest methods to reverse a JavaScript string. The reverse()
method is one of the built-in array methods. It reverses the order of the original array’s elements.
To use this method in JavaScript to reverse a string, first split the string into an array of characters. Here’s an example of how to reverse a string in JavaScript:
let originalString = 'JavaScript runs everywhere!';
// split reverse and join
let reversedString = originalString
.split('')
.reverse()
.join('');
console.log(reversedString);
// Output: "!erehwyreve snur tpircSavaJ"
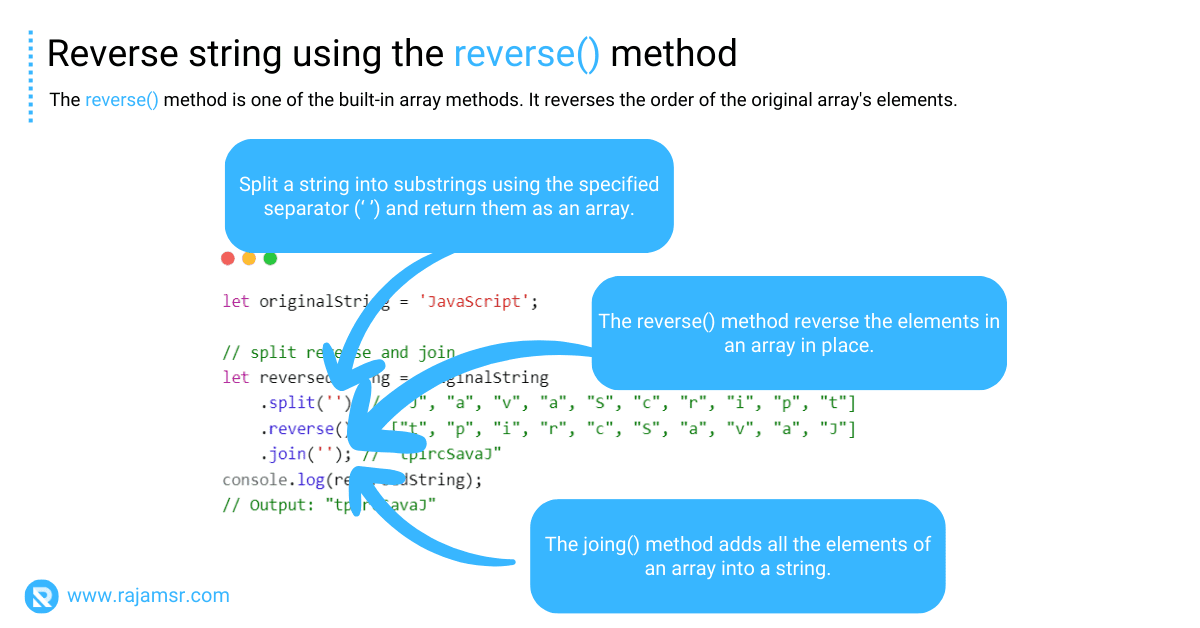
To reverse a string, you can use one of the JavaScript array methods reverse(). The array join()
method is used to join the reversed array of strings.
Reverse string without using reverse() method
If you want to reverse a string without using the built-in reverse()
array method, you can use either a for loop (method 2)
or a reduce() (method 5)
or recursive (method 5)
method based solution.
2. Reverse a string in JavaScript using a for loop
Using a loop is another method to reverse a string in JavaScript. When using a for loop, you must iterate through the string’s characters from the last index
to the first index
. Retrieve the characters using the charAt()
method. Finally, using JavaScript string concatenation, we can create a new string.
Here’s an example of how to reverse a string without using the reverse()
method:
let originalString = 'Hello World';
let reversedString = '';
for (let index = originalString.length - 1; index >= 0; index--) {
reversedString += originalString.charAt(index);
}
console.log(reversedString);
// Output: "dlroW olleH"
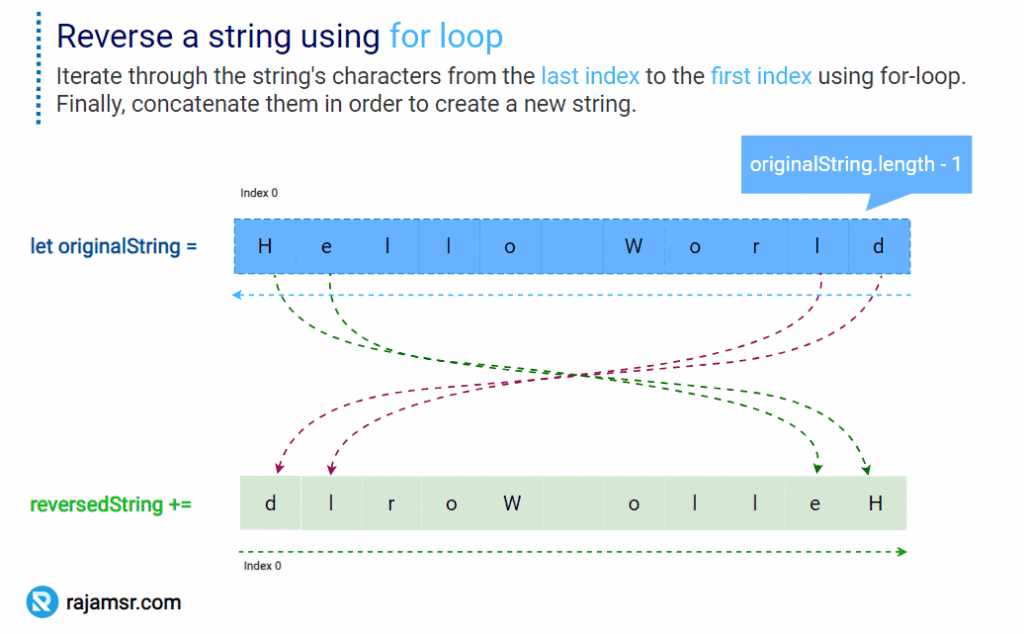
The for loop
approach is not only traditional but also allows for customization, making it suitable for specific use cases.
3. Reverse string using the reduce() method
The reduce()
array method is a more elegant way of reversing a string in JavaScript. This method takes each array element and applies a function to it, reducing the array to a single value.
When using this method to reverse a string, use the reduce()
function to loop through the characters and concatenate them to a new string in reverse order.
Here’s an example of how to reverse a string using the split()
and reduce()
methods:
let originalString = 'Hello World';
let reversedString = originalString
.split('')
.reduce((acc, char) => char + acc, '');
console.log(reversedString);
// Output: "dlroW olleH"
reduce()
method iterates over each character in the string. Builds a new string by adding each character to the accumulated result acc
in reverse order. The initial value of acc
is a JavaScript empty string, and the result is the reversed string.4. Reverse each word in a string in JavaScript
In certain scenarios, the requirement may not be to reverse the entire string but to reverse each word
in a sentence individually.
Here’s a solution to reverse each word in a string:
// Reverse each word in a string
function reverseStringBasic(inputString) {
return inputString
.split('')
.reverse()
.join('');
}
function reverseWordsInSentence(inputString) {
return inputString
.split(' ')
.map(word => reverseStringBasic(word))
.join(' ');
}
let originalString = 'JavaScript runs everywhere!';
let reversedString = reverseWordsInSentence(originalString);
console.log(reversedString)
// Output: "tpircSavaJ snur !erehwyreve"
This method is particularly useful when dealing with sentences and the need to maintain word order while reversing individual words.
5. JavaScript reverse a string using recursion
You can use recursive function
to reverse a string. Let’s see an example:
function reverseStringRecursive(inputString) {
if (inputString === '') return inputString;
return reverseStringRecursive(inputString.substr(1)) + inputString[0];
}
let originalString = 'JavaScript runs everywhere!';
let reversedString = reverseStringRecursive(originalString);
console.log(reversedString)
// Output: "!erehwyreve snur tpircSavaJ"
The function recursively reverses a string by taking the JavaScript substring starting from the second character. Appending the first character at each step, until the base case (empty string) is reached, creating the reversed string.
This is an excellent demonstration of the power of recursive functions.
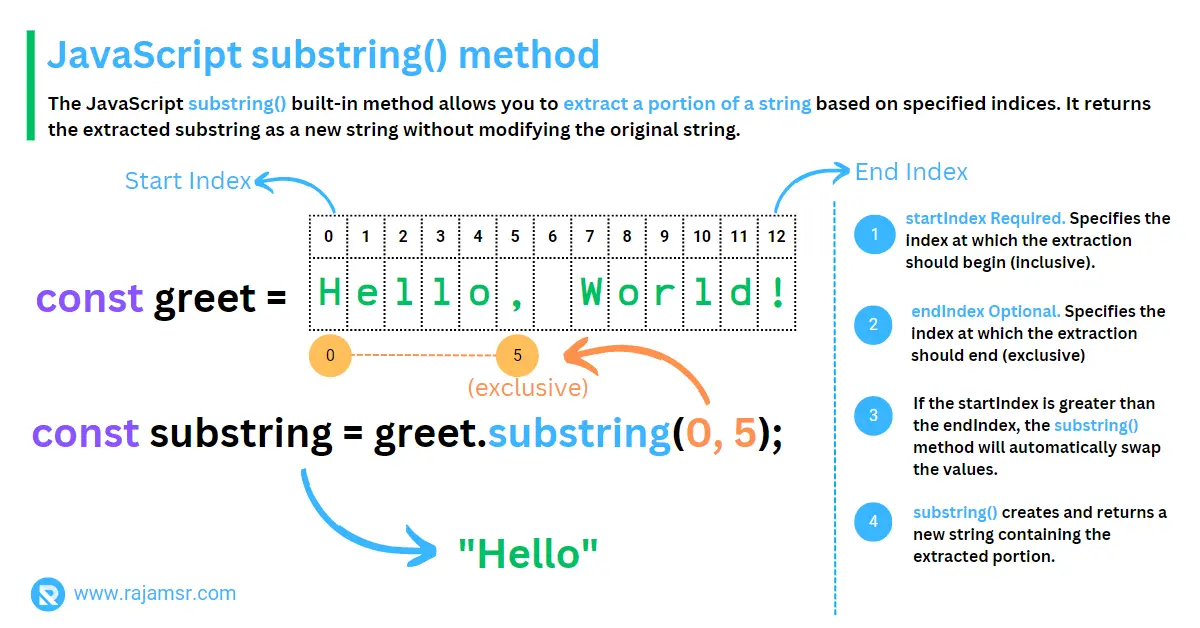
6. Reverse a string without function
If you are looking for a concise solution without explicitly defining a function, consider using an immediately invoked function expression (IIFE):
let originalString = 'JavaScript runs everywhere!';
const reversedString = (s => s.split('').reverse().join(''))(originalString);
console.log(reversedString)
// Output: "!erehwyreve snur tpircSavaJ"
This one-liner is both efficient and suits scenarios where a reusable function is not necessary.
Reverse number using JavaScript
To reverse a string, you can use one of the methods listed above. What if you need to reverse a number? The solution is straightforward.
- Use the JavaScript toString() function to convert any number, not only an integer, to a string.
- To reverse a string, use one of the methods listed above in this article.
- Using the JavaScript parseFloat() or JavaScript parseInt() methods, depending on the data type, convert the generated string to a number.
const number = -12345.67;
const reversedString = number
.toString()
.split('')
.reverse()
.join('');
let reversedNumber = parseFloat(reversedString) * Math.sign(number);
console.log(reversedNumber);
// Output: -76.54321
The toString()
method was used to transform the input number into a string in the above code. The string is then split into an array of characters using the split method with the empty string (‘ ‘) delimiter. We next reverse the array’s order using the reverse()
method and then join the array into a string using the join(' ')
method, with the empty string ' '
serving as the separator once more.
The resulting reversed string is then converted back to a number using the Number()
object constructor.
With this approach, you can easily reverse any number in JavaScript.
Reverse array using JavaScript
Reversing an array is simple and straightforward because reverse()
is a built-in array method.
const numbers = [10, 20, 30, 40, 50]
let reversedNumbers = numbers.reverse();
console.log(reversedNumbers)
// Output: [50, 40, 30, 20, 10]
The JavaScript reverse()
method will update the original array. You can use the reverse()
method to reverse object arrays as well as numeric arrays.
const books = [
{ bookdId: 100, title: 'Mastering JS' },
{ bookdId: 200, title: 'JS for WebDev' },
{ bookdId: 300, title: 'Pactical JS' }
]
let reversedBooks = books.reverse();
console.log(reversedBooks)
/* Output:
[
{ bookdId: 300, title: 'Pactical JS' },
{ bookdId: 200, title: 'JS for WebDev' },
{ bookdId: 100, title: 'Mastering JS' }
]
*/
Conclusion
In this article, we have explored three different methods to reverse a string in JavaScript. To reverse a string using the reverse()
method is simple.
If you want to reverse a string without using the built-in reverse()
method, you can use the for loop or reduce()
methods. Compared to the different reverse methods, every reverse method has its own set of benefits and drawbacks.
In addition to the string reverse method, you learned how to reverse a number and an array of numbers. Regardless of the method chosen, it is important to understand the underlying logic and principles involved in reversing a string.
Which method are you going to use to reverse a string?