Do you want to learn how to use JavaScript endsWith() method to manipulate strings in your code? If you are a web developer, you know how important it is to work with strings efficiently and effectively. Strings are everywhere in web development, from URLs to user input to JSON data. But sometimes, you need to check the end of a string for a specific value or pattern. For example, you might want to validate an email address, a file extension, or a date format. How can you do that easily and quickly with JavaScript? The answer is JavaScript endsWith(), a built-in string method that returns true or false depending on whether a string ends with a given value.
In this blog, I will show you:
- The endsWith() method and its syntax
- How to Use endsWith() method
- Real-world use cases with examples
- Alternatives to endsWith() method
- JavaScript endsWith() case insensitive
- Support for old browsers
Let’s get started!
JavaScript string endsWith() method
The endsWith()
string method is used to determine whether a JavaScript string ends with a specified string or character. The syntax for endsWith()
is straightforward and consists of the optional length of the string parameters, as shown in the following:
string.endsWith(searchString[, length])
Parameter | Description |
---|---|
string | The input string you want to check. |
searchString | String or characters to be searched for. |
length | Optional. specifies the from where to start the string search. If no length is provided, the full string will be searched. |
endsWith()
JavaScript method:endsWith()
is one of the JavaScript String methods. It returns true if the specified string ends with a character or substring.- It’s important to note this
endsWith()
is case-sensitive. It will do a strict JavaScript string equality comparison and return false if the search string and the string being searched are not the same.
For example, if you have a string like 'Hello, JavaScript'
and you want to check if the string ends with "JavaScript"
, you can use the endsWith()
method like this:
const greet = 'Hello, JavaScript';
console.log(greet.endsWith('JavaScript'));
// Output: true
console.log(greet.endsWith('javascript'));
// Output: false
endsWith()
in the example verifies if the string "Hello, JavaScript"
has "JavaScript"
as its last part. The result is returning true because that is the case for the string.The second statement in the above code example returns false because the string does not end with the lowercase string "javascript"
.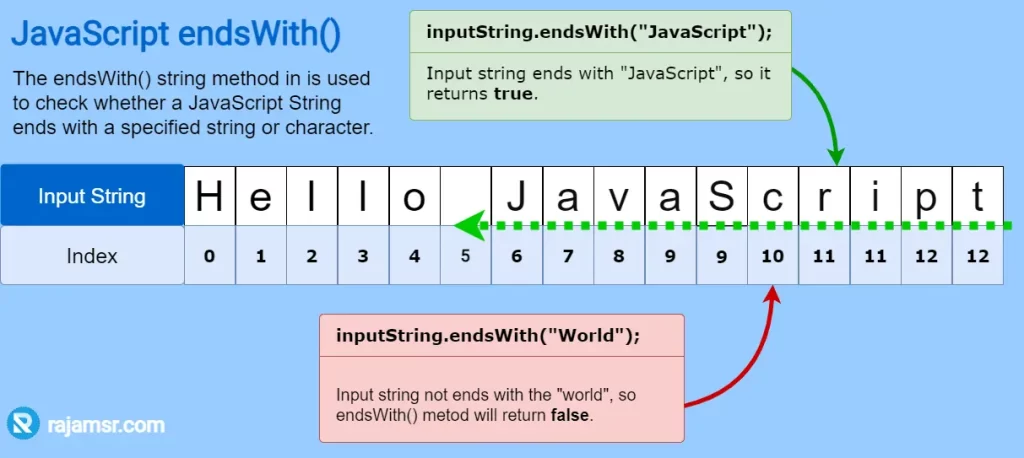
In JavaScript, there is an identical opposite method to the endsWith()
method. The JavaScript startsWith() method can be used to check whether a string begins with a specific substring or character.
Using endsWith() JavaScript method in real-world scenarios
1. File name validation using JavaScript string endsWith() method
You can use the JavaScript string endsWith()
method to check whether a file name ends with a specific file type or extension, such as .jpg
, or .pdf
. This can be useful for validating user input in a form or ensuring that links are pointing to the correct resources.
const fullName = "C:\\windows\\pictires\\image.jpg";
if (fullName.endsWith(".jpg")) {
console.log('File format is jpg.')
}
2. Text filtering with endsWith() in JavaScript
You can use endsWith()
to filter out text that does not end with a specific pattern, such as filtering out all lines of text that do not end with a period. The JavaScript array methods filter()
is used to filter the result.
const lines = [
"This is first line.",
"This is just another line.",
"Line with no period"
];
const filteredLines = lines.filter(line => !line.endsWith("."));
console.log(filteredLines);
// Output: ["Line with no period"]
3. Filter by file type (.jpg or .jpeg)
Assume you are reading all files from a directory and want to filter by a particular file extension. JavaScript endsWith()
can be used to find files in a directory that have a particular extension. This can be helpful for tasks like batch renaming or deleting files.
const files = [
"C:\\Images\\file1.bmp",
"C:\\Images\\file2.png",
"C:\\Images\\file3.jpg",
"C:\\Images\\file4.jpeg"];
const jpegFiles = files.filter(file => file.endsWith(".jpg") || file.endsWith(".jpeg"));
console.log(jpegFiles);
// Output: ["C:\Images\file3.jpg", "C:\Images\file4.jpeg"]
In this example, the OR condition (||)
is combined with multiple endsWith()
methods to select files with the extension .jpg
or .jpeg
. In JavaScript, an escape character is required for a backslash, so a double backslash (\\)
is used in the file name.
4. Formatting file name using string endswith() method
Assume you’re merging a directory and a file name. It will not work in some cases if the file name contains multiple directory separators. You can guarantee that the directory name ends with a backslash by using the endsWith()
method. This method will ensure that if the directory name ends with a backslash, ignore it; otherwise, add it to the end to create the correct file name.
function combinePath(dir, fileName)
{
return dir.endsWith('\\')
? `${dir}${fileName}`
: `${dir}\\${fileName}`
}
console.log(combinePath('C:\\Docs', 'images.jpg'))
console.log(combinePath('C:\\Docs\\', 'images.jpg'))
// Output: C:\Docs\images.jpg
// Output: C:\Docs\images.jpg
JavaScript string endsWith() case insensitive
The endsWith()
method is case-sensitive by default. In some cases, check whether the string ends with a specific substring; regardless of the case,
Either you can convert the input and search string to lowercase using toLowerCase()
or JavaScript Uppercase using the toUpperCase()
method before calling the endsWith()
method to ensure that it works properly regardless of the case. Here is an example of how to use endsWith() case insensitive manner:
const endsWithIgnoreCase = (inputString, searchString) =>
{
const loweredInputString = inputString.toLocaleLowerCase();
return loweredInputString.endsWith(searchString.toLocaleLowerCase())
}
console.log(endsWithIgnoreCase('Hello, JavaScript', 'JavaScript'));
// Output: true
console.log(endsWithIgnoreCase('HELLO, JAVASCRIPT', 'javajcript'));
// Output: true
JavaScript endsWith() alternative methods
The endsWith()
method in JavaScript is used to determine whether a string ends with a specified substring. If you’re looking for alternative methods to achieve the same result, here are a three options:
1. Using regular expression test() as endsWith() alternative
You can use regular expressions to check if a string ends with a particular pattern. Here’s an example:
const greet = 'Hello, JavaScript!';
const pattern = /JavaScript!$/;
const endsWithPattern = pattern.test(greet);
console.log(endsWithPattern);
// Output: true
In this example, you create a regular expression pattern /JavaScript!$/
which matches the substring "JavaScript!"
at the end of the string. The regular expression test()
method is used to check if the pattern matches the string.
2. Using substring() as endsWith() alternative
You can combine the JavaScript substring() and lastIndexOf()
methods to check if a string ends with a specified substring. Here’s an example:
const greet = 'Hello, JavaScript!';
const pattern = 'JavaScript!';
const endsWithSubstring = greet.substring(greet.lastIndexOf(pattern)) === pattern;
console.log(endsWithSubstring);
// Output: true
In this example, you use lastIndexOf()
JavaScript array method to find the index of the last occurrence of the substring "JavaScript!"
in the string. Then, you extract a substring from that index to the end using substring()
and compare it with the search string to check if they are equal.
3. Using slice() as endsWith() alternative
You can use the JavaScript slice() method along with length comparison to check if a string ends with a specific substring. Here’s an example:
const greet = 'Hello, JavaScript!';
const pattern = 'JavaScript!';
const endsWithSlice = greet.slice(-pattern.length) === pattern;
console.log(endsWithSlice);
// Output: true
In this example, you use the slice()
method with a negative index to extract a substring from the end of the string. You can extract a substring of the same length as the search string and compare it with the search string to check if they are equal.
These are some alternative methods you can use to achieve similar functionality to endsWith()
. Each method has its own advantages and may be more suitable depending on the specific use case.
JavaScript endsWith() alternative methods performance
I conducted a performance test for the endsWith()
method and its alternative solutions. The results showed that the endsWith()
method performed better than the others. It achieved 158,697
operations per second, while the alternative methods were slower.
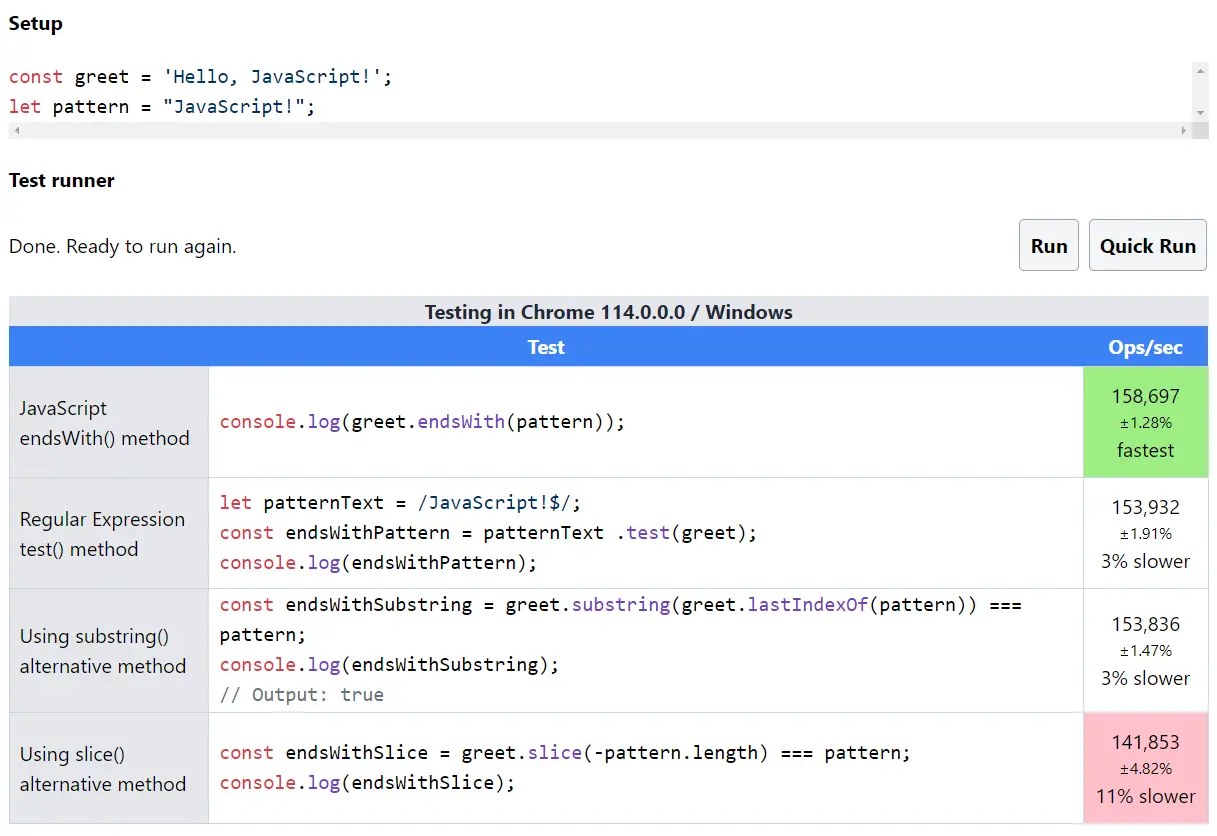
JavaScript string endswith() regex
You can’t use regex (regular expressions)
in the endsWith()
method to check whether the string ends with the specified string.
let text = "Hello, JavaScript!";
let pattern = /JavaScript/g;
console.log(text.endsWith(pattern))
// TypeError: First argument to String.prototype.endsWith must not be a regular expression
If you want to use a regex to confirm the ending of a string, you can use the following steps:
- Create a variable adding the “$” at the end of the “target” argument, using the concat() method in this case.
- Use the RegExp constructor and the “new” operator to create the right RexExp with the above variable.
- Return the result of the test() function.
Here is an example of how to use string endsWith() regex to check if a string ends with specific string:
function isEndsWith(string, target) {
// Step 1 - Create a variable adding the "$" at the end of the "target" argument
var pattern = target.concat("$");
// Step 2 - Use the RegExp constructor and the "new" operator
// to create the right RexExp
var regex = new RegExp(pattern);
// Step 3 - Return the result of the test() function
return regex.test(string);
}
console.log(isEndsWith("Hello, JavaScript", "Script")); // true
console.log(isEndsWith("Hello, JavaScript", "script")); // false (case-sensitive)
console.log(isEndsWith("Hello, JavaScript", "Hello")); // false
How to implement endsWith() in older browsers
Modern browsers enabled the .endsWith()
method, which was introduced in ECMAScript 6. However, if you need to support older browsers, this approach may not be supported. In that situation, a polyfill
function can be used to provide endsWith()
support for those browsers.
Here is an example of a polyfill function for endsWith()
:
if (!String.prototype.endsWith) {
String.prototype.endsWith = function (searchString, position) {
if (position === undefined || position > this.length) {
position = this.length;
}
return this.substring(position - searchString.length, position) === searchString;
};
}
JavaScript endsWith() is not a function
endsWith()
is a string function. If you call it on another type of string, it will throw an "endswith is not a function"
error message.To avoid this error, you can use the JavaScript typeof() operator to determine the type before calling the endsWith()
method, as shown in the following example:const greet = 'Hello, World!';
if (typeof (greet) === 'string') {
console.log(greet.endsWith('World!'))
}

Conclusion
In conclusion, JavaScript endsWith()
is a perfect choice to check whether the string ends with a specific substring or character. It is used to check whether a string ends with a specified suffix, and it can be combined with other string methods.
- You can use the
endsWith()
method to verify file types, filter text that ends with a particular character, and format file names. - The
endsWith()
function is case-sensitive by default. You must take extra steps to operate in case-insensitive mode, either by using string lowercase or uppercase methods. - You can use the substring() method to create an alternate method for
endsWith()
. - The
endsWith()
function does not support regular expressions. - If your user is using an older version of a browser, such as Internet Explorer 11 or earlier, use the polyfill to support the
endsWith()
method.
Although it may seem like a small method for JavaScript, this method can greatly simplify certain programming tasks.
For which use case are you going to use the endsWith()
method?