Do you want to learn how to sum an array in JavaScript? If you are a web developer, you probably encounter this problem frequently, as arrays are one of the most common and useful data structures in JavaScript. However, unlike some other programming languages, JavaScript does not have a built-in method to sum an array of numbers. So, how can you perform this basic arithmetic operation on arrays in JavaScript?
In this blog post, I will show you:
- Calculate the sum of a JavaScript array with the reduce() method
- Compute the array sum in JavaScript using a for-loop
- Determine the sum of an array with a for…of loop
- Retrieve the sum of an array using the forEach() method
- Calculate the array sum using the map() and reduce() methods
- Use recursion to find the sum of an array
- Evaluate the Pros and Cons of each method for array summation
- Assess the Performance Benchmark for array summation methods
I will also show you the performance comparison and readability of each approach. By the end of this blog post, you can sum an array in JavaScript like a pro. Let’s get started!
1. JavaScript array sum using the reduce() method
One simplest and most elegant way to sum an array is to use the JavaScript array method reduce() method. This method takes a callback function and an initial value as arguments, and applies the callback function to each element of the array, accumulating the result in a single value.
The callback function takes two parameters: the accumulator and the current element. The accumulator is the value returned after each iteration, and the current element is the value being processed. The initial value is the starting point for the accumulator, and it is optional.
If you omit the initial value, the first element of the array will be used as the accumulator, and the second element will be the current element.
Array sum values
To sum an array of numbers using the JavaScript array method reduce(), you can write something like this:
// An array of numbers
let numbers = [1, 2, 3, 4, 5];
// Sum the array using reduce()
let sum = numbers.reduce((accumulator, current) => accumulator + current);
// Print the result
console.log(sum);
// Output: 15
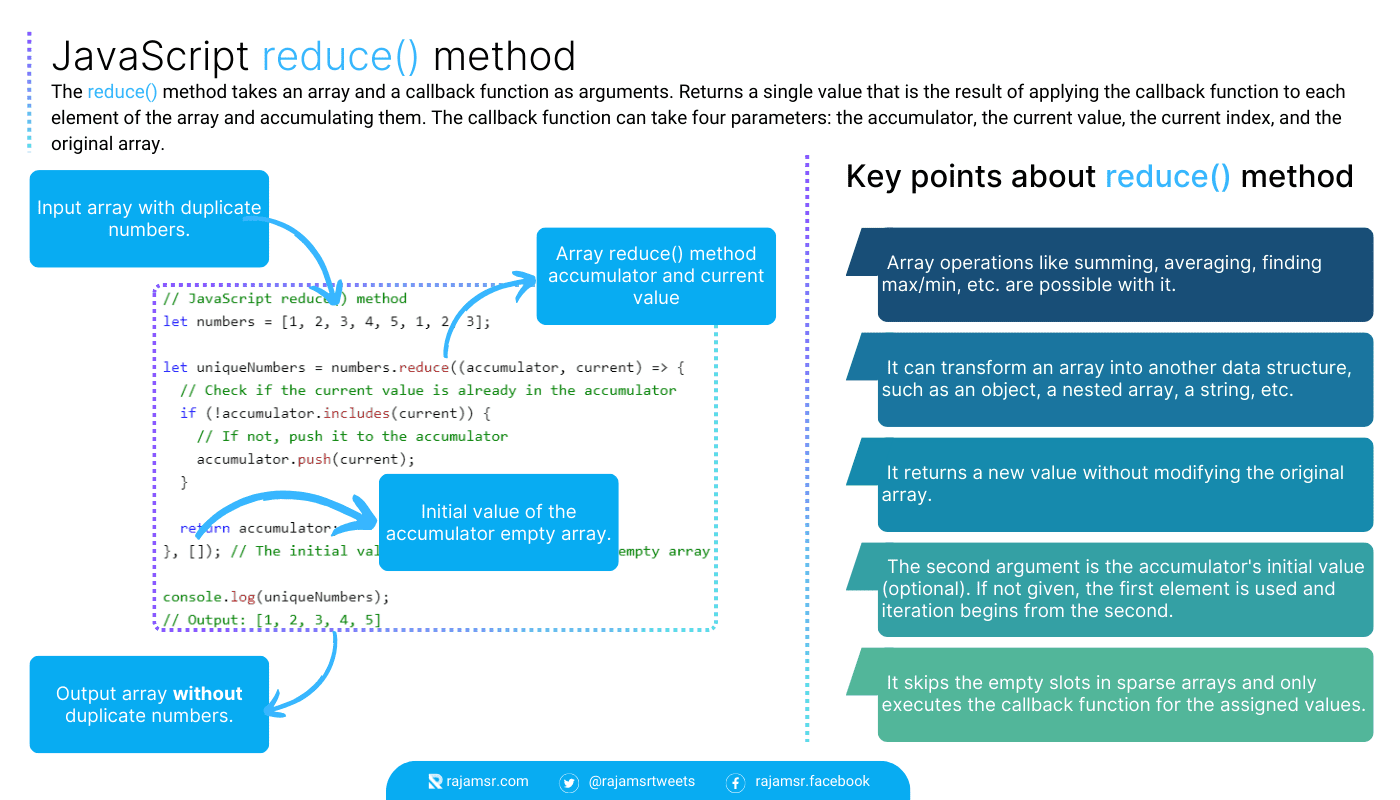
In this code, an array of numbers is defined. The reduce() method is used to sum up all the elements in the array. The result is stored in the variable sum.
Array sum of property
For example, you can sum an array of objects by accessing a specific property of each object, such as:
// An array of book objects
let books = [
{ name: "JavaScript Guide", price: 5 },
{ name: "JS Reference Book", price: 10 },
{ name: "JS Ultimate Guide", price: 20 },
];
// Sum the price property of each object using reduce()
let total = books.reduce((accumulator, current) => accumulator + current.price, 0);
// Print the result
console.log(total);
// Output: 35
This JavaScript code defines an array of book objects with names and prices. It then uses the reduce() function to calculate the total price of all books and print the result, which is 35, to the console.
Note that in this case, you have to provide an initial value of 0 for the accumulator, otherwise, the first element of the array will be used as the accumulator, and the result will be incorrect.
The reduce() method is one of the most popular and recommended ways to sum an array in JavaScript, as it is concise, expressive, and functional. However, it may not be the most intuitive or familiar way for beginners, and it may have some performance drawbacks compared to other methods.
2. Array sum using a for-loop
Another common way to sum an array in JavaScript is to use a for-loop. This is a basic and imperative way of iterating over an array, using a counter variable, a condition, and an increment expression. Inside the loop, you can access each element of the array by its index, and add it to a JavaScript variable that holds the sum. For example:
// An array of numbers
let numbers = [1, 2, 3, 4, 5];
// Initialize a variable to store the sum
let sum = 0;
// Loop through the array using a for loop
for (let index = 0; index < numbers.length; index++) {
// Add the current element to the sum
sum += numbers[index];
}
// Print the result
console.log(sum);
// Output: 15
The for-loop is a classic and familiar way of working with arrays, and it gives you full control over the iteration process. You can also use a for-loop to sum an array of objects, by accessing specific keys of each object, such as:
// An array of book objects
let books = [
{ name: "JavaScript Guide", price: 5 },
{ name: "JS Reference Book", price: 10 },
{ name: "JS Ultimate Guide", price: 20 },
];
// Initialize a variable to store the sum
let total = 0;
// Loop through the array using a for loop
for (let i = 0; i < books.length; i++) {
// Add the price property of the current object to the total
total += books[i].price;
}
// Print the result
console.log(total);
// Output: 60
The for-loop is a simple way to sum an array in JavaScript, and it may have some performance advantages over other methods. However, it may also be more verbose, error-prone, and less readable than other methods.
There are many other ways to sum an array in JavaScript, using different methods and techniques. Some of them are listed below sections.
3. The sum of an array using a for…of loop
This is a modern and concise way of iterating over an array, without using a counter variable or an index. For example:
// An array of numbers
let numbers = [1, 2, 3, 4, 5];
// Initialize a variable to store the sum
let sum = 0;
// Loop through the array using a for...of loop
for (let number of numbers) {
// Add the current element to the sum
sum += number;
}
// Print the result
console.log(sum);
// Output: 15
4. Get the sum of an array using a forEach() method
The forEach() is a built-in method that executes a callback function for each element of the array. For example:
// An array of numbers
let numbers = [1, 2, 3, 4, 5];
// Initialize a variable to store the sum
let sum = 0;
// Loop through the array using the forEach() method
numbers.forEach(number => {
// Add the current element to the sum
sum += number;
});
// Print the result
console.log(sum);
// Output: 15
5. The sum of an array using a map() and reduce() method
The map() and reduce() are two built-in methods that can be chained together to transform and aggregate an array.
The map() method creates a new array by applying a callback function to each element of the original array.
The reduce() method reduces the new array to a single value by applying another callback function. For example:
// An array of numbers
let numbers = [1, 2, 3, 4, 5];
// Sum the array using map() and reduce()
let sum = numbers.map(number => number * 2)
.reduce((accumulator, current) => accumulator + current);
// Print the result
console.log(sum);
// Output: 30
6. Find the sum of an array using a recursion method
This technique calls a function from within itself until a base case is reached. For example:
// An array of numbers
let numbers = [1, 2, 3, 4, 5];
// Define a recursive function to sum an array
function sumArray(array) {
// Base case: if the array is empty, return 0
if (array.length === 0) return 0;
// Recursive case: return the first element
// of the array plus the sum of the rest of the array
return array[0] + sumArray(array.slice(1));
}
// Call the recursive function and print the result
console.log(sumArray(numbers));
// Output:15
This JavaScript code defines an array of numbers and a recursive function called sumArray() to calculate the sum of the array elements. JavaScript slice(1) returns all array elements starting from index 1. It then calls the function with the array [1, 2, 3, 4, 5] and prints the result, which is 15.
These are just some of the many possible ways to sum an array in JavaScript, and each one has its pros and cons. Depending on your preference, use case, and performance requirements, you may choose the one that suits you best.
Pros and Cons of each sum method
Table that summarizes the main advantages and disadvantages of each method:
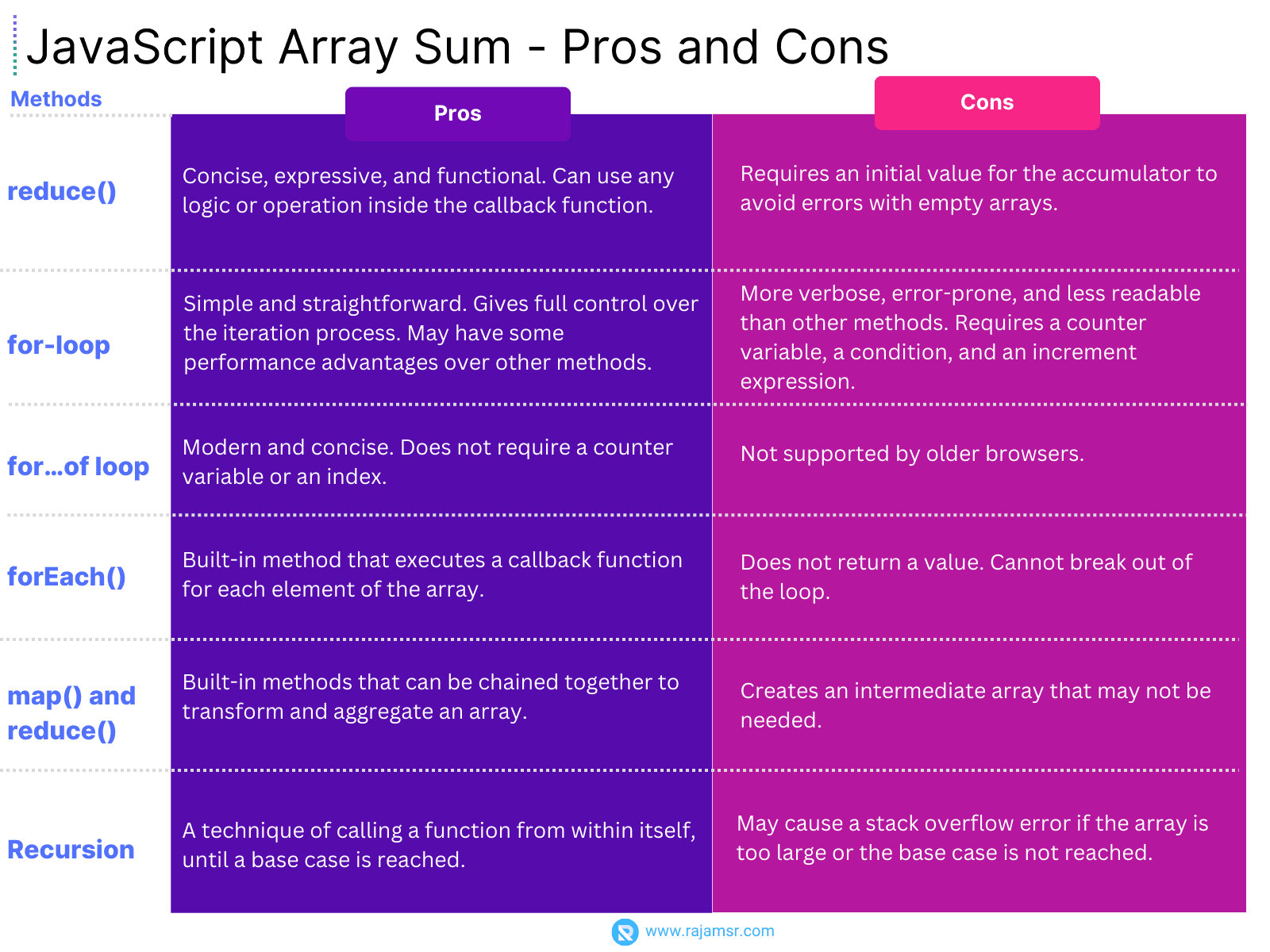
Performance Benchmark
I did a performance benchmark with all these methods using the JsPerf tool. I used the same input for all the methods.
let numbers = [1, 2, 3, 4, 5];
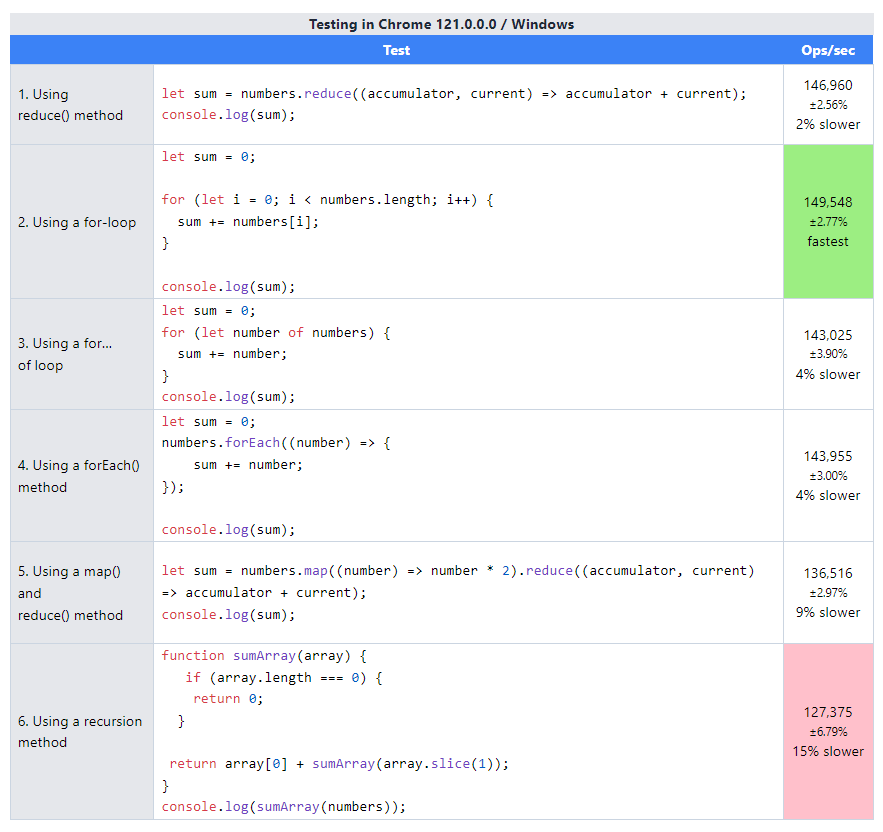
From the result, you can see that for-loop is doing better compared to other methods. Use the recursion method only if there are no other options because of its poor performance.
Conclusion
In this blog post, we have learned how to sum an array in JavaScript, using different methods and techniques. We have seen how to use the reduce() method, the for loop, the for…of loop, the forEach() method, the map() and reduce() method, and the recursion. We have also compared the performance and readability of each approach and provided some tips and best practices for working with arrays in JavaScript.
I hope you have found this blog post helpful and informative. Over to you, which method you are going to use sum an array elements?