Do you know how to use JavaScript comments in your code? Comments are one of the most important and useful features of any programming language. They allow you to write notes, explanations, and instructions for yourself and others who read your code. They also help you to debug, test, and improve your code quality.
In this blog, I will show you:
- How to use comments in JavaScript
- Writing single-line comments in JavaScript
- Creating a multi-line comment in JavaScript
- Different kinds of comments in JavaScript
- A quick way to comment in JavaScript
- Commenting in JavaScript with Visual Studio Code
- Adding comments to a JavaScript function
- Commenting on an object in JavaScript
- How to comment on the DOM in JavaScript
- Documenting your code with JavaScript comments
- Can you comment in JSON with JavaScript?
Let’s dive in.
Comments in JavaScript
JavaScript comments are like notes that you write for yourself and other programmers. They help you remember what your code does and why. There are two kinds of comments:
- Single-line and multi-line. Single-line comments are for short notes. They start with two slashes (//) and end when the line ends.
- Multi-line comments are for longer notes. They start with a slash and a star (/) and end with a star and a slash (/). They can go over many lines.
Look at these examples of how to use comments in JavaScript code:
// This is a single-line comment
console.log("Hello, world!");
/* This is a multi-line comment
You can write as many lines as you want
Until you close the comment with */
console.log("Goodbye, world!");
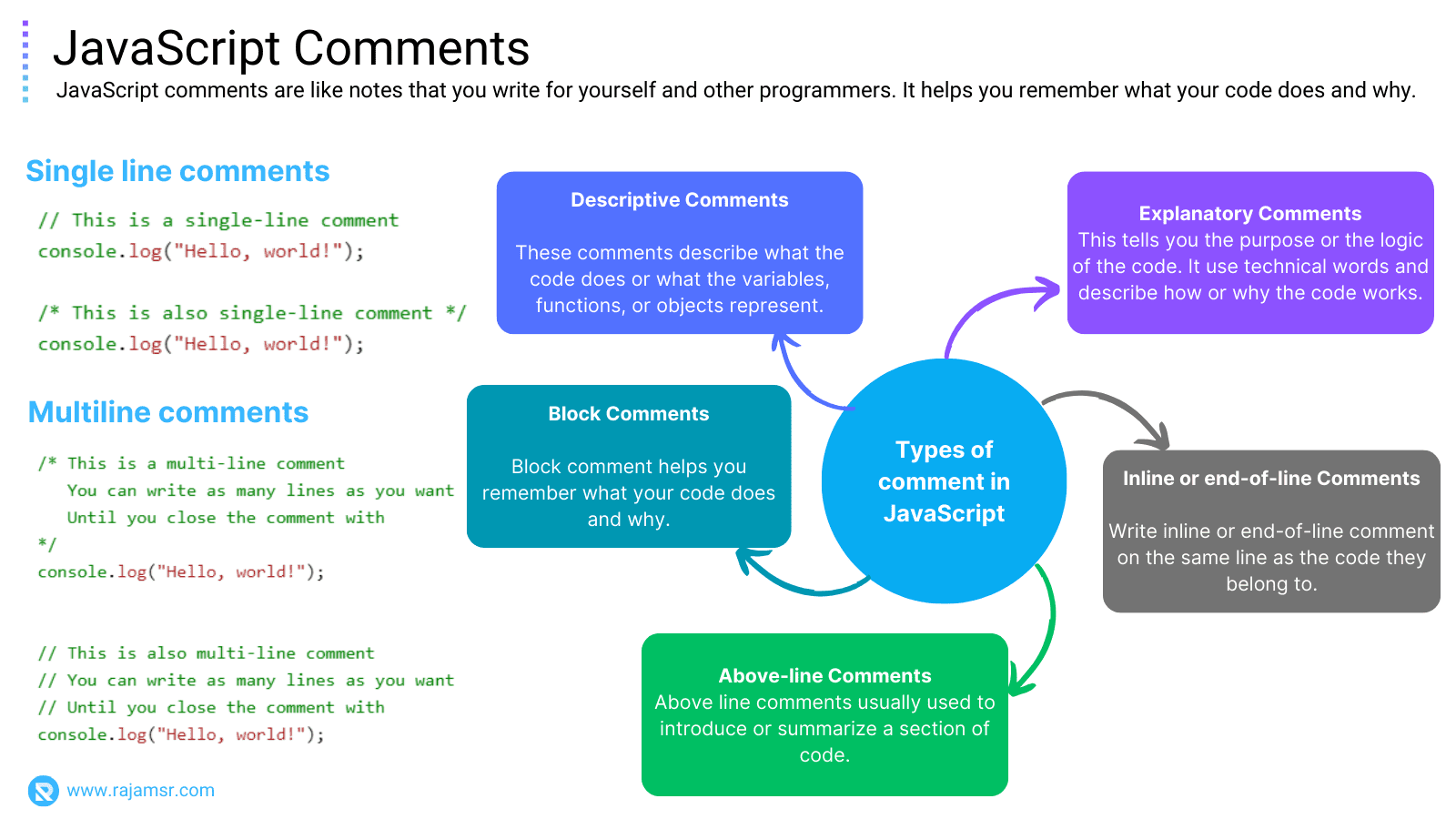
Single line JavaScript comments
Single-line
JavaScript comments start with two slashes (//)
and can be placed anywhere in your code. Everything after the slashes on a line will be ignored by the JavaScript interpreter.
Let’s take a look at how to write a single line comment in JavaScript:
// This is a single-line comment
console.log("Hello, world!");
Multi-line JavaScript comments
Do you like writing code in JavaScript? Then you should know how to use multi-line comments. They are awesome! You can write as many lines as you want inside them. They won’t affect your code at all. You can use them for different things.
/* This is a multi-line comment
You can write as many lines as you want
Until you close the comment with */
console.log("Hello, world!");
// This is a multi-line comment
// You can write as many lines as you want
// Until you close the comment with
console.log("Hello, world!");
Preventing code execution using JavaScript comments
Sometimes you want to try different things with your code and see what happens. Or maybe you have a problem and you need to find out where it is. In these cases, you can use multi-line comments to turn off some parts of your code for a while. This way, you can focus on the parts that matter and ignore the rest. For example:
/* This code will not run
console.log("This is a test");
alert("This is an alert");
*/
// This code will run
console.log("This is not a test");
Documenting code
Sometimes it can be hard to remember what each piece of code does, or to explain it to others. That’s why I use multi-line comments to document my code. They let me write longer descriptions of my functions or objects, like what they do, what they take as input, what they give as output, and how to use them. This makes my code easier to understand and reuse.
Here’s an example of how I write multi-line comments:
/* This function calculates the area of a circle
It takes one parameter, r, which is the radius of the circle
It returns the area as a number
Example: areaOfCircle(5) returns 78.53981633974483 */
function areaOfCircle(r) {
return Math.PI * r * r; // Use the formula for the area of a circle
}
Type of comments in JavaScript
There are different types of comments that serve different purposes and have different styles. Here are some of the common types of comments in JavaScript:
Descriptive comments
These comments describe what the code does or what the JavaScript variables, functions, or JavaScript objects represent. They are usually written in plain English and use nouns and adjectives. For example:
// This is a descriptive comment
let name = "John";
Explanatory comments
Sometimes we need to explain our code to other programmers or ourselves. You can use explanatory comments to do that. Explanatory comments tell you the purpose or the logic of the code. They use technical words and describe how or why the code works. For example:
// We use a number instead of a string to perform calculations
let age = 25;
Inline or end-of-line comments
inline
or end-of-line
comments on the same line as the code they belong to. They are short and clear, and they end with a period or other punctuation. For example:let x = 10; // Initialize x with value 10
x++; // Increment the value of x
Block comments
Sometimes you want to group some statements and run them as one. You can do that with blocks in JavaScript. Blocks are like containers for your code. You can make a block with curly braces ({}) or words like if, else, for, while, and so on. Blocks can also have other blocks inside them.
But blocks can also be tricky and hard to understand, especially if they have many code, layers, or complicated logic. That’s why you should use comments to explain your blocks in JavaScript.
Here is an example of block level comment:
// This block defines a function
function sumOfSquares(a, b) {
// This block declares and initializes two variables
var x = a * a;
var y = b * b;
// This block returns the sum of the squares
return x + y;
}
var result = sumOfSquares(3, 4);
console.log(result);
Above-line JavaScript comments
These comments are written on the line above the code they refer to. They are usually used to introduce or summarize a section of code. For example:
// Declare and assign variables
var z = 30;
var w = 40;
// Perform some operations
z += w; // Add w to z
w -= z; // Subtract z from w
// Print the results
console.log(z); // Print z
console.log(w); // Print w
JavaScript Comments Shortcut
Writing comments sometimes can be a hassle, especially when I have to write many of them or update them often. That’s why I use keyboard shortcuts to make my life easier. They let me comment and uncomment code in JavaScript with just a few keystrokes.
Here are some of the shortcuts I use for different editors and IDEs:
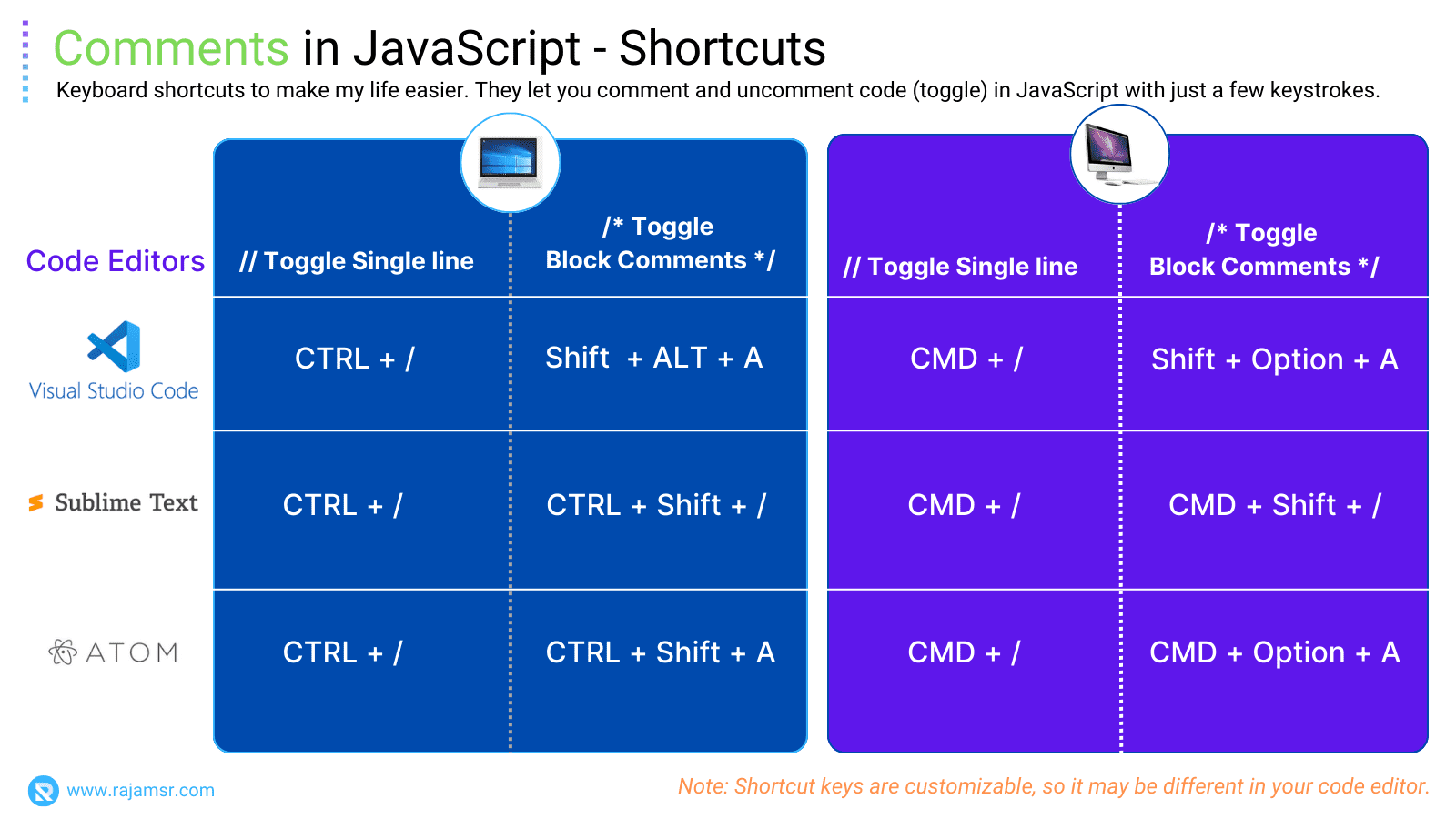
// Press Ctrl + / or Cmd + / to toggle this line comment
console.log("Hello, world!");
/* Press Ctrl + Shift + / or Cmd + Shift + / to toggle this block comment
console.log("Goodbye, world!");
*/
Comment in JavaScript Visual Studio Code
Visual Studio Code is one of the most popular and powerful editors for JavaScript development. It has many features and extensions that can help you comment and uncomment code in JavaScript more efficiently and effectively. Here are some of them:
Key Binding | Description |
---|---|
CTRL+K+C | Add line comment. |
CTRL+K+U | Remove line comment. |
Shift+ALT+A | Toggle block comment. |
CTRL+/ | Toggle line comment. |
Extensions
Visual Studio Code
has some amazing extensions to make your comments more fun and effective. Some of the extensions are:Better Comments: This extension lets you use different colors and styles for different kinds of comments. You can write alerts
, questions
, todos
, highlights
, and more. Here’s an example of how it works:// ! This is an alert comment
// ? This is a question comment
// TODO: This is a todo comment
// * This is a highlight comment
Comment Anchors: Do you want to make your comments more organized and fun? Try this extension! It lets you add anchors to your comments that you can jump to easily. You can also customize your anchors with different icons
and colors
. Here’s how it works.
// ANCHOR: This is an anchor comment
// FIXME: This is a fixme comment
// NOTE: This is a note comment
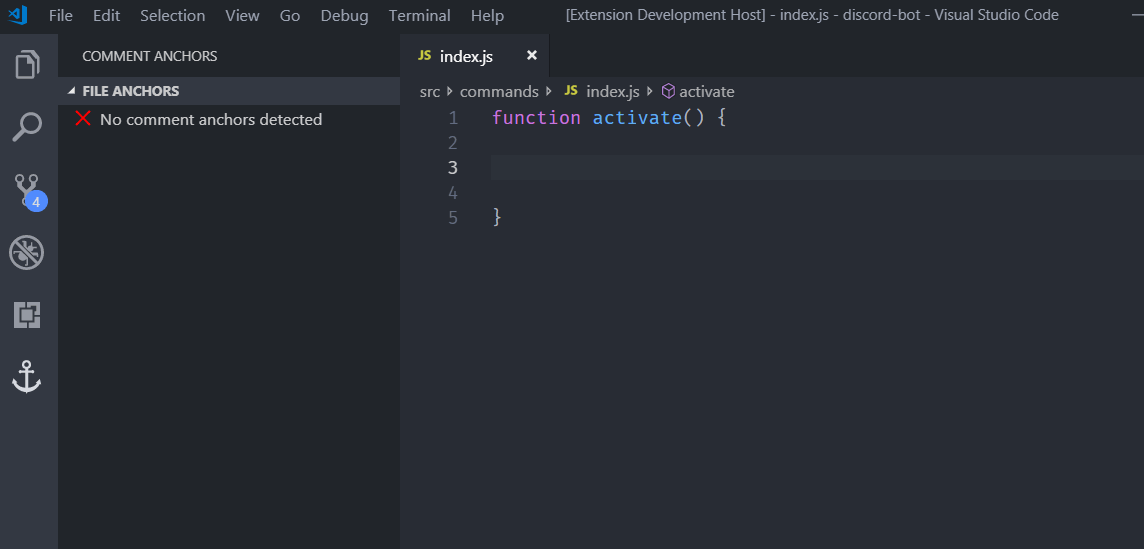
Comment in JavaScript function
Functions are one of the non-primitive data types in JavaScript. Functions are like little blocks of code that do something for you. You can give them some inputs, and they will give you some outputs. You can also use them over and over again, and put them in different places in your code.
But how do you remember what each function does, and how to use it? That’s where comments come in handy. They explain what your code does, and why you wrote it that way. For example:
/* This function calculates the factorial of a number
It takes one parameter, n, which is a positive integer
It returns the factorial as a number
Example: factorial(5) returns 120 */
function factorial(n) {
// Base case: factorial of 0 or 1 is 1
if (n === 0 || n === 1) {
return 1;
} else {
// Recursive case: n! = n * (n-1)!
return n * factorial(n - 1);
}
}
// Example: Calculate factorial of 5
let result = factorial(5);
console.log(result);
Comments in JavaScript Object
JavaScript object lets you keep and change many values and functions together. You can also use them to make your code look like the things and ideas you want to work with. But sometimes objects can be hard to understand, especially when they have a lot of stuff inside them. That’s why you should always write comments to explain your objects in JavaScript.
/* This object represents a person
It has three properties: name, age, and gender
It has two methods: greet and birthday
*/
var Person = {
name: "John",
age: 30,
gender: "male",
greet: function() {
console.log("Hello, my name is " + this.name);
},
birthday: function() {
this.age++;
console.log("Happy birthday, I am now " + this.age + " years old");
}
};
Comment in JavaScript DOM
The DOM lets you change the web page with JavaScript. You can access and change the parts of the HTML document, like elements, attributes, styles, and events. But the DOM can be hard to work with if you have a lot of things in your web page. So you should use comments to help you with the DOM in JavaScript. Here are some ways to do that:
JavaScript comments to create, access, modify, and remove elements and attributes in the DOM
Comments are useful for writing and reading code. They can explain what the code does and why. They can also turn on or off some code that changes the DOM. For example:
// Create a new element
var div = document.createElement("div");
// Access an existing element
var p = document.getElementById("paragraph");
// Modify the element's style
p.style.color = "red";
// Remove the element from the DOM
// document.body.removeChild(p);
// Add an attribute to the element
div.setAttribute("id", "container");
// Remove an attribute from the element
// div.removeAttribute("id");
Difference between HTML comments and JavaScript comments in the DOM
HTML comments go inside the HTML document, and JavaScript comments go inside the script tag or the external file. Both types of comments can help you change the web page with JavaScript, but they have different rules. For example:
<!-- This is an HTML comment -->
<html>
<head>
<script>
// This is a JavaScript comment
console.log("Hello, world!");
</script>
</head>
<body>
<p id="paragraph">This is a paragraph</p>
<script>
// This is another JavaScript comment
var p = document.getElementById("paragraph");
p.innerHTML = "This is a new paragraph";
</script>
</body>
</html>
Documentation using JavaScript comments
You want your code to be clear and easy for yourself and others. That’s why you need documentation. Documentation tells what your code does, how it works, and how to use it.
But writing documentation can be hard and boring. You don’t want to waste time or repeat yourself. So, use comments to make documentation for your JavaScript code.
You can use different tools to make docs for your JavaScript code. For example, JSDoc, ESDoc, Docco, and more. These document generator tools need you to write comments specially. They can read your comments and make docs from them. They also need you to use tags, like @param
, @return
, @example
, and so on. These tags tell the tools about your code.
They can make docs in many formats, like HTML, PDF, Markdown, and so on. Here is an example JavaScript string reverse example with documentation tags:
/**
* This function reverses a string
* @param {string} originalString - The string to reverse
* @return {string} The reversed string
* @example
* reverseString("hello") returns "olleh"
*/
function reverseString(originalString) {
var reversed = "";
for (var index = originalString.length - 1; index >= 0; index--) {
reversed += originalString[index];
}
return reversed;
}
Comments in JavaScript JSON
JSON lets you store and share data easily. But it has a catch: no comments. That’s right, you can’t write anything to explain your data to yourself or others. That can be frustrating and confusing. But don’t worry, there are solutions! You can use some tricks to add comments to your JSON data in JavaScript.
Let’s see how!
Use a JavaScript object instead of a JSON string
Here’s a simpler way to work with JSON data in JavaScript: use a JavaScript object! You can write comments with // or /* */ to explain your data. But there are some things to watch out for:
- You need to change your object to a JSON string when you send or save it and change it back when you get or load it.
- You need to follow the rules of JavaScript syntax, like using quotes and commas correctly.
- You need to protect your object from being changed or seen by other parts of your code.
// This is a JavaScript object that represents a person
var person = {
// This is a single-line comment
"name": "Alice", // The name of the person
/* This is a multi-line comment
It explains the age and gender of the person */
"age": 25,
"gender": "female"
};
// This is a JSON string that represents the same person
var personJSON = '{"name":"Alice","age":25,"gender":"female"}';
Use a JSON parser or serializer that supports comments
If you want to add comments to your JSON data in JavaScript, you can use a special JSON parser or serializer. This lets you write your JSON data with the normal rules, like double quotes and colons, but also with comments. But be careful, because this method has some problems:
- You need to use an extra library or tool that can handle comments, like JSON5, JSONC, HJSON, and so on.
- You need to check that the library or tool works well with your code and your system and that it is safe and trustworthy.
- You need to learn the specific syntax and rules of each library or tool, because they may differ in how they deal with comments and other JSON features.
// This is a JSON5 string that represents a person
var personJSON5 = '{
// This is a single-line comment
name: "Alice", // The name of the person
/* This is a multi-line comment
It explains the age and gender of the person */
age: 25,
gender: "female"
}';
// This is a JSON5 parser that supports comments
var person = JSON5.parse(personJSON5);
Conclusion
In this blog, you have learned how to use JavaScript comments to explain, document, and test your code. Comments are lines of code that don’t run. They help you and others understand your code better.
You can use single-line
or multi-line
comments to explain your code or stop some code from running. You also learned about different types of comments and how to write them well. For example, descriptive comments
tell what your code does, explanatory comments
tell why your code does something, and inline comments
tell how your code does something.
You also learned how to use tools and extensions to comment and uncomment code easily in JavaScript. For example, you can use keyboard shortcuts, Visual Studio Code
commands and extensions, and common formats and standards, such as JSDoc
, ESDoc
, etc. You also learned how to use comments for other purposes, such as documenting and manipulating the DOM
, generating documentation, creating code blocks, and annotating JSON data in JavaScript. You can use some common solutions, such as JSON5
, JSONC
, HJSON
, etc.
Comments are awesome and powerful in JavaScript. They help you write notes, explanations, and instructions for yourself and others. They also help you debug, test, and improve your code quality and performance. Comments are not only for humans but also for machines. They can help you generate documentation, validate data, and optimize performance.
Comments are not a waste of time, but a valuable investment for your code quality and productivity.
Over to you: How you are going to use JavaScript comments?