Have you ever wondered how to JavaScript loop over object properties and values? JavaScript objects are awesome! They let you store different kinds of data in a single variable. But sometimes, you might want to do more with them. For example, you might want to loop through all the keys and values of an object and use them for something. How can you do that? Well, there is no easy way to do that with objects. Unlike arrays, they don’t have a built-in method for looping. But don’t worry, there are some tricks you can use to make it work.
In this blog post, I will show you:
- Three different ways to loop over JavaScript objects
- The advantages and disadvantages of each method
- Loop through array of objects
- Loop through JSON object in JavaScript
In this post, I will show you how to iterate over any object in JavaScript with ease. You will learn the basics and the best practices of looping. You will also see some examples and tips to make your code more readable and efficient. Let’s dive in!
Looping over object keys
JavaScript object keys or properties are the names or identifiers of the values stored in an object. JavaScript object is one of the non-primitive data types in JavaScript. They can be strings, symbols, or numbers, and they are used to access or modify the object’s values. For example, person.name is a property that returns the name value of the person object.
const person = {
name: "John",
age: 30,
favoriteColors: ["Red", "Green"]
};
We are going to use the same person object throughout the post.
Method 1: JavaScript loop over object keys using Object.keys()
The JavaScript Object.keys() method returns an array of all the keys (or property names) of an object. You can then use a for loop or a forEach() method to iterate over the array and access the keys. To loop over the keys of the person object, you can use the Object.keys() static method like this:
const keys = Object.keys(person);
// Output: ["name", "age", "favoriteColors"]
// 1. Using a for() loop
for (let i = 0; i < keys.length; i++) {
console.log(keys[i]);
}
// Output: "name", "age", "favoriteColors"
// 2. Using a forEach() method
keys.forEach(key => {
console.log(key);
});
// Output: "name", "age", "favoriteColors"
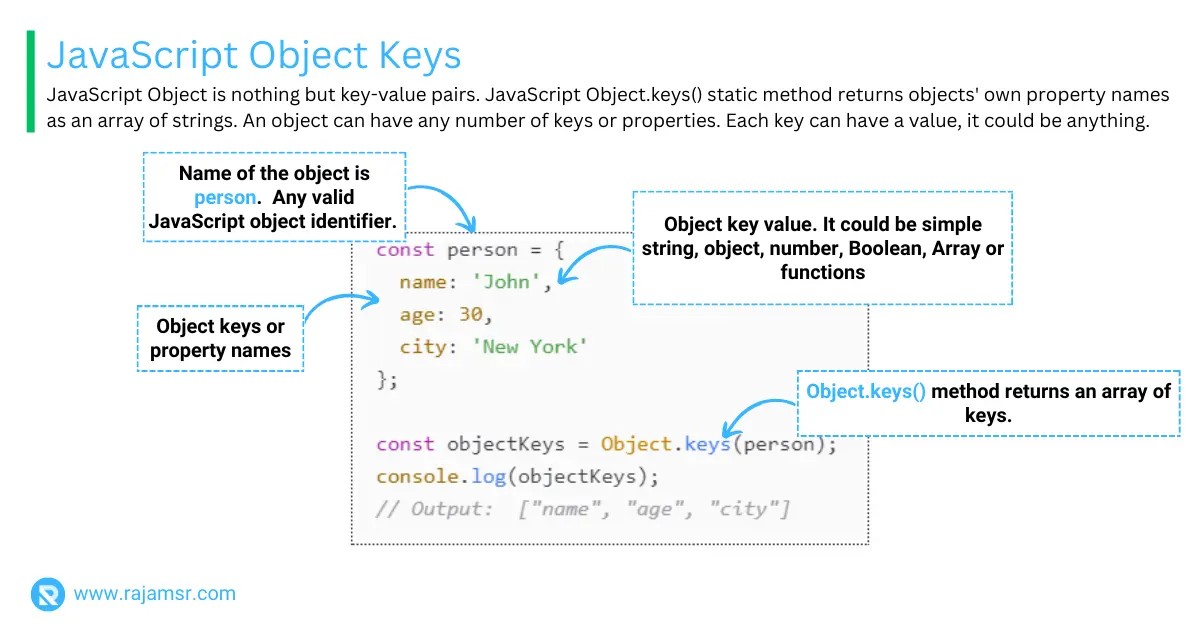
The pros of this method are:
- It is simple and easy to use.
- It works with any object, regardless of its structure or complexity.
- It is compatible with older browsers, as it is part of the ECMAScript 5 standard.
The cons of this method are:
- This method gives you the object’s own keys. It ignores the keys it got from its prototype chain. Sometimes, that’s exactly what you need. Other times, you might want to include those keys too.
- Objects don’t have a fixed order for their keys. That means you can’t always expect them to show up in the same sequence. Be careful if your logic depends on the key order, because it might not work as you expect.
- This code makes another array. That can slow down your code and use more memory. This is worse if the object is big or has many parts.
Method 2: Looping over object values using Object.values()
Object.values() gives us an array with all the values of an object. You can loop over the array and get the values easily. For example, look at this person object. You can use Object.values() like this:
const values = Object.values(person);
// Output: ["John", 40, ["Red", "Green"]]
// 1. Using a for() loop
for (let index = 0; index < values.length; index++) {
console.log(values[index]);
}
// Output: ["John", 40, ["Red", "Green"]
// 2. Using a forEach() method
values.forEach(key => {
console.log(key);
});
// Output: ["John", 40, ["Red", "Green"]
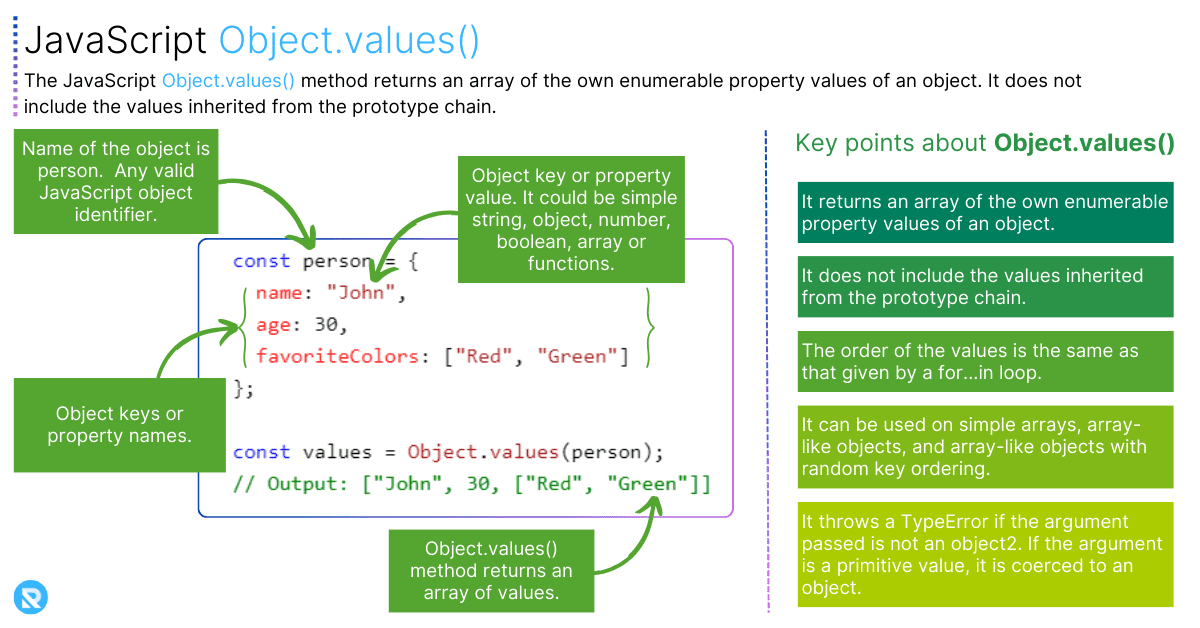
The pros of this method are:
- It is simple and easy to use.
- It works with any object, regardless of its structure or complexity.
- It is part of the ECMAScript 2017 standard, which means it is a modern and widely supported feature.
The cons of this method are:
- It only returns the own values of the object. Meaning it does not include the values inherited from the object’s prototype chain. This may or may not be what you want, depending on your use case.
- Object.values() doesn’t care about the order of the values, because objects are not ordered. That can be a problem if your code depends on the order.
- This is a new feature, so some old browsers don’t support it. You may need to use some tricks to make it work in those cases.
- Object.values() creates a new array with the values, which can affect how fast and how much memory your code uses. This can be an issue if your object is big or complex.
Method 3:Looping over object entries using Object.entries()
Do you want to get the key-value pairs of an object? You can use Object.entries()! It gives you an array of arrays, where each subarray has a key and a value. Then you can loop over the array with for or forEach and use the entries however you want.
For example, using the same person object as before, you can use the Object.entries() method like this:
const entries = Object.entries(person);
// 1. Using a for() loop
for (let index = 0; index < entries.length; index++) {
console.log(entries[index]);
}
// Output: ["name", "John"], ["age", 30], ["favoriteColors", ["Red", "Green"]]
// 2. Using a forEach() method
entries.forEach(entry => {
console.log(entry);
});
// Output: ["name", "John"], ["age", 30], ["favoriteColors", ["Red", "Green"]]
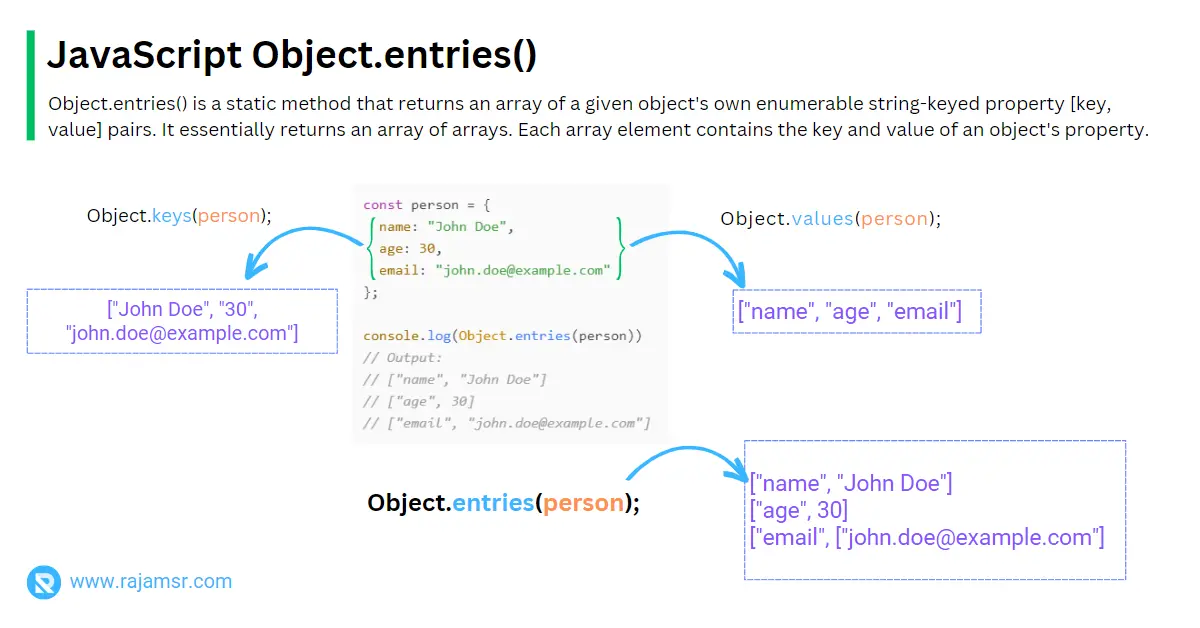
The pros of this method are:
- It is simple and easy to use.
- It works with any object, regardless of its structure or complexity.
- It is part of the ECMAScript 2017 standard, which means it is a modern and widely supported feature.
- It returns both the keys and the values of the object, which can be useful if you need to access both in your logic.
The cons of this method are:
- It only returns the own entries of the object, meaning it does not include the entries inherited from the object’s prototype chain. This may or may not be what you want, depending on your use case.
- It does not guarantee the order of the entries, as objects are unordered by nature. This may cause some issues if you rely on the order of the entries for your logic.
- It creates an extra array, which may affect the performance and memory usage of your code, especially if the object is large or nested.
- It is not compatible with older browsers, as it is a relatively new feature. You may need to use a polyfill or a transpiler to make it work in older environments.
Method 4: JavaScript loop over object properties using for...in
With the for…in loop, you can go through all the properties of an object. It gives you a variable that holds the property name. Then you can use the variable with a dot or brackets to get the property value.
For example, using the same person object as before, we can use the for…in statement like this:
const person = {
name: "John",
age: 30,
favoriteColors: ["Red", "Green"]
};
// Using a for...in statement
for (let key in person) {
console.log(key); // Output: "name", "age", "favoriteColors"
console.log(person[key]); // Output: "John", 30, ["Red", "Green"]
}
The pros of this method are:
- It is simple and easy to use.
- It does not create an extra array, which may improve the performance and memory usage of your code, especially if the object is large or nested.
- It returns both the keys and the values of the object, which can be useful if you need to access both in your logic.
The cons of this method are:
- It returns all the enumerable properties of the object, meaning it includes the properties inherited from the object’s prototype chain. This may or may not be what you want, depending on your use case. If you only want to loop over the own properties of the object, you need to use the hasOwnProperty() method to filter out the inherited properties.
- It does not guarantee the order of the properties, as objects are unordered by nature. This may cause some issues if you rely on the order of the properties for your logic.
- It is not compatible with some newer features of JavaScript, such as Symbol properties or Object.defineProperty(). These features can create non-enumerable properties that are not visible to the for…in statement.
Comparison of different looping over object properties
Here is a quick comparison table of the four methods:
Method | Returns | Own/All | Order | Performance | Compatibility |
---|---|---|---|---|---|
Object.keys() | Keys | Own | No | Low | High |
Object.values() | Values | Own | No | Low | Medium |
Object.entries() | Key-values | Own | No | Low | Medium |
for...in | Properties | All | No | High | Low |
Loop through array of objects
An array of objects is a data structure that stores multiple objects in a single variable. Each object can have different properties and values, and can be accessed by using an index or a key. For example, we can create an array of objects that represents some books:
var books = [
{ name: "JavaScript Ultimate", price: 10.2 },
{ name: "JS for Kids", price: 2.2 },
{ name: "JS Reference Guide", price: 15.2 }
];
You can access the first object in the array by using books[0], and you can access the name property of the first object by using books[0].name or books[0][“name”].
There are several ways to loop through an array of objects in JavaScript, such as:
- Using a for loop
- Using a for…of loop
- Using a forEach method
- Using a map method
- Using a filter method
- Using a reduce method
for (var index = 0; index < books.length; index++) {
console.log(`${books[index].name} costs ${books[index].price}$.`);
}
/*
JavaScript Ultimate costs 10.2$.
JS for Kids costs 2.2$.
JS Reference Guide costs 15.2$.
*/
Loop through JSON object in JavaScript
A JSON object is a data format that is commonly used for exchanging data between web servers and clients. JSON stands for JavaScript Object Notation, and it is based on the syntax of JavaScript objects. However, there are some differences between JSON and JavaScript objects, such as:
- JSON keys must be quoted using double quotes, while JavaScript keys can be unquoted or quoted using single or double quotes.
- JSON values can only be strings, numbers, booleans, arrays, objects, or null, while JavaScript values can also be functions, dates, symbols, or undefined.
- JSON does not support comments, while JavaScript does.
A JSON object can be stored in a string variable or a file, and it can be parsed into a JavaScript object by using the JSON.parse() method.
For example, you can create a JSON object that represents some books:
let books = `[{"title": "The Lord of the Rings", "author": "J.R.R. Tolkien", "genre": "Fantasy"},
{"title": "The Hitchhiker\'s Guide to the Galaxy", "author": "Douglas Adams", "genre": "Science Fiction"},
{"title": "Harry Potter and the Philosopher\'s Stone", "author": "J.K. Rowling", "genre": "Fantasy"}]`;
You can parse the JSON string object into a JavaScript object. After that, you can access the title, author, and genre properties of the book object. For example:
let jsonBooks = JSON.parse(books);
for (var index = 0; index < jsonBooks.length; index++) {
console.log(jsonBooks[index].title);
}
/* Output:
The Lord of the Rings
The Hitchhiker's Guide to the Galaxy
Harry Potter and the Philosopher's Stone
*/
Conclusion
In this blog post, you have learned how to loop over objects in JavaScript using four different methods: Object.keys(), Object.values(), Object.entries(), and for…in. Each method has its own advantages and disadvantages, depending on your use case and preferences.
The best way to loop over objects in JavaScript depends on what you want to achieve and how you want to write your code. However, a general rule of thumb is to use the Object methods if you want to access the keys, values, or entries of the object, and use the for…in statement if you want to access the properties of the object and its prototype chain.
I hope you found this blog post helpful and informative. Over to you: Which method you are going to use to loop through object properties?