Have you ever wondered how to check if a key exists in JavaScript? A key is a property name or an index that identifies a value in a data structure. Checking for key existence is a common task in JavaScript programming, as it can help you avoid errors, validate data, and perform conditional logic.
In this blog post, you will learn:
- Check if key exists in object
- Finding a key in an array with JavaScript
- Checking for a key in a JSON object or array
- Searching for a key in an array of objects with JavaScript
- Testing if a key exists in a Dictionary or a Map
- Accessing localStorage to check for a key with JavaScript
- Using ES6 syntax to check for a key with JavaScript
- Frequently asked questions
By the end of this blog post, you will be able to confidently check if a key exists in JavaScript and write more robust and efficient code. Let’s get started!
JavaScript check if key exists in object
Objects are like containers for data. They have keys and values. Keys are words, values are anything. For example, this is an object for a person:
const person = {
firstName: 'John',
lastName: 'Doe',
age: 30,
city: 'New York'
};
person
object, firstName
, lastName
, age
, and city
are keys, and "John"
, "Doe"
, 30
, and "New York"
values. To check if a key exists in an object, you can use one of the following methods:1. Check if key exists using hasOwnProperty() method
When working with objects, the JavaScript hasOwnProperty() method proves invaluable. This method checks if an object has a specified property and returns a boolean value.
Let’s see an example of how to check if a key exists in an object:
console.log(person.hasOwnProperty('age')); // Output: true
console.log(person.hasOwnProperty('gender')); // Output: false
console.log(person.hasOwnProperty('toString')); // Output: false
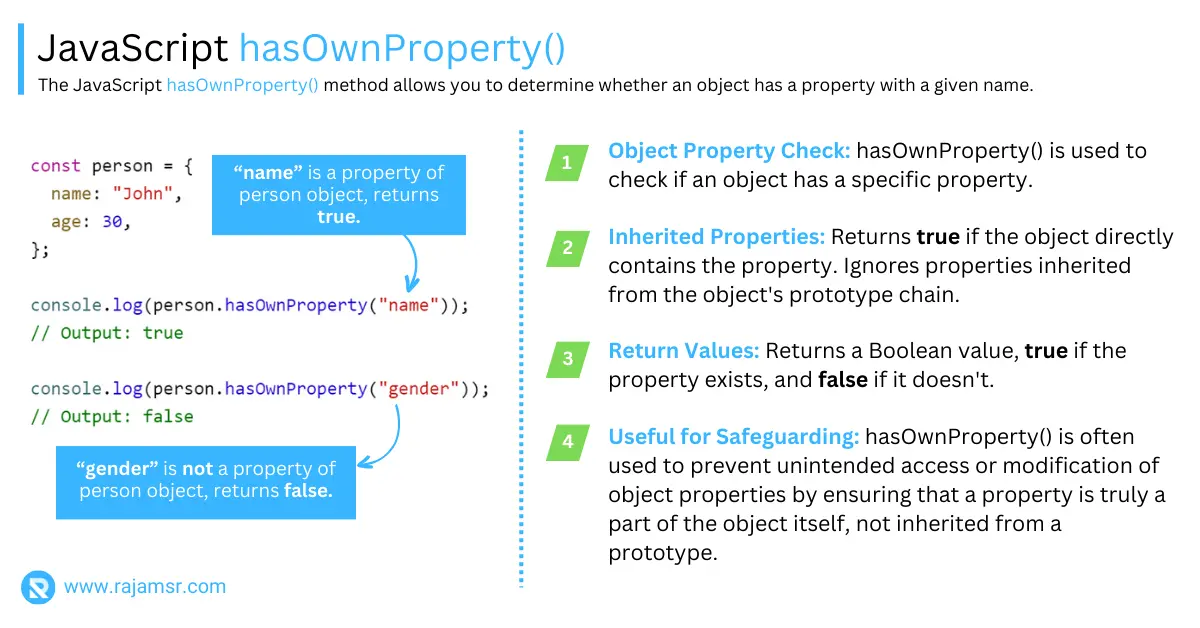
hasOwnProperty()
method. It only looks for keys that belong to the object. This way, you can avoid some mistakes or problems that might happen because of inherited keys. The only downside is that it takes more words and space than the in
operator.2. Check if key exists in object using Object.keys() static method
You can also use JavaScript Object.keys() ES6 method to check whether an object has specific keys or not. Let’s see an example of how to use Object.keys()
method to check if the key exists in an object:
console.log(Object.keys(person).find(k => k === 'age') !== undefined);
// Output: true
console.log(Object.keys(person).find(k => k === 'gender') !== undefined);
// Output: true
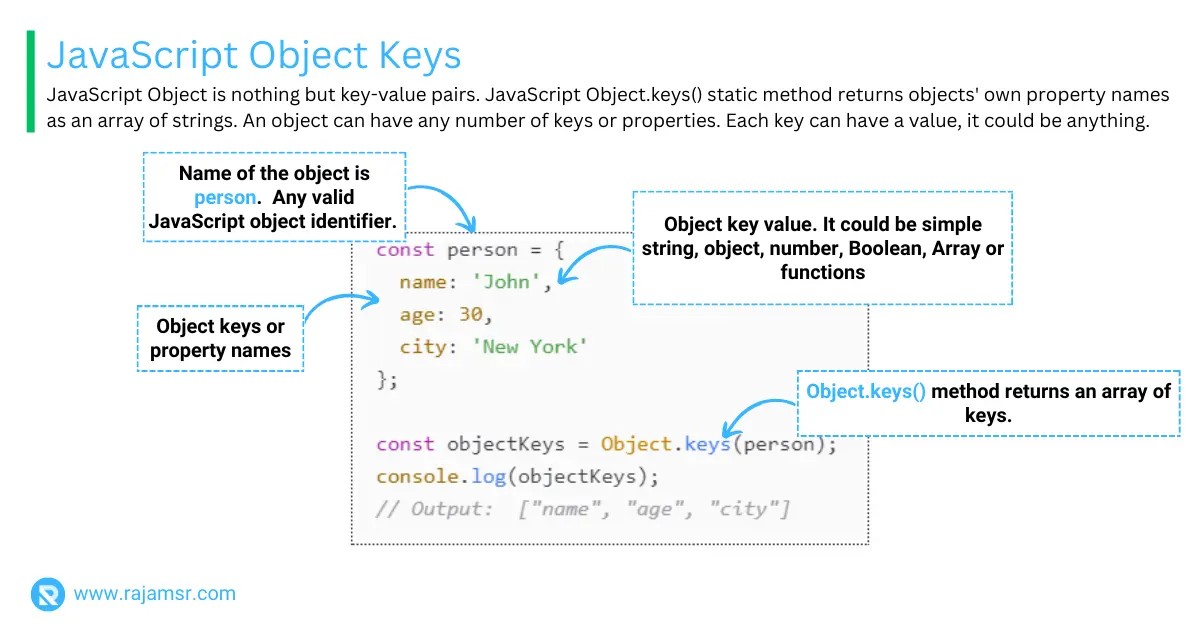
JavaScript Object.keys()
static method will return all keys from an object. JavaScript array find() method used to check the key "age"
exists in the object. The key name exists in the person object, so it returns true.
3. Using the in operator
in
operator returns true if a key exists in an object, and false otherwise. For example, you can check if the key name exists in the person
object like this:console.log("age" in person); // Output: true
console.log("gender" in person); // Output: false
in
operator to find keys that are not only in the object itself, but also in its prototype chain. That means the keys that the object inherited from another object. For example, let’s see if the person
object has the toString
key. You can do this with the in operator like this:console.log("toString" in person); // Output: true
Every object in JavaScript has a toString
key that comes from the Object prototype. This is the basic model for all objects. The in
operator is a quick and easy way to check if an object has a key. But be careful! It can’t tell the difference between keys that belong to the object and keys that come from the prototype. This might lead to some mistakes or surprises.
Check if key exists in an array
index
, that shows its position in the array. The index starts from zero. For example, look at this array of severities
:const severities = ['bocker', 'critical', 'major', 'minor'];
In this array, 0, 1, 2, and 3 are keys, and blocker
, critical
, major
, and minor
are values. To check if a key exists in an array, you can use one of the following methods:
1. Using the indexOf() method
critical
is in the numbers array:console.log(severities.indexOf('critical') !== -1);
// Output: true
critical
in the severities
array. It’s at position 1, so indexOf()
gives you 1. That means the expression is true. You can also look for the key "info"
in the same array.console.log(severities.indexOf('info') !== -1);
// Output: false
indexOf()
to look for info
in the numbers array, you get -1. That means info
is not there. But -1 is also not there, so the expression is false. The good thing about indexOf()
is that it works on old browsers too. The bad thing is that it only finds values that are exactly the same. So it won’t work for things like objects or arrays, which are not simple values.2. Using the includes() method
For arrays, the JavaScript includes() method is the go-to option. Want to find a value in an array? Use the includes()
method! It will tell you if the value is there or not. Just give it the value as an argument. For example, let’s see if critical
is in the severities
array:
console.log(severities.includes('critical')) // Output: true
console.log(severities.includes('info')) // Output: false
severities
array has info
in it. You can use the includes()
method for that. It gives you false, meaning info
is not there. This method is easy and clear, but it has some drawbacks. It doesn’t work on old browsers or on non-primitive values like objects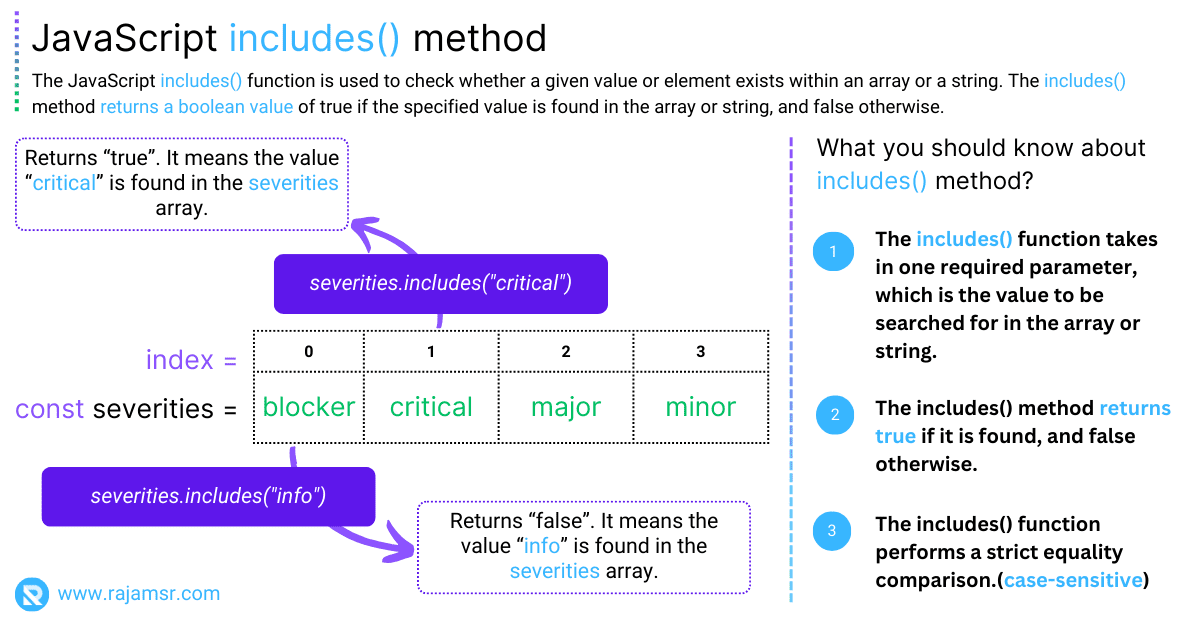
3. Using the find() method
Do you want to find a value in an array that meets a certain condition? You can use the JavaScript find() method! It will give you the first value that matches, or undefined
if nothing does. You can also use it to see if a key is in an array. Just give it a function that returns true or false for each value and key.
For example, this is how you can check if the key critical
is in the severities
array:
console.log(severities.find(value => value === "critical") !== undefined);
// Output: true
find()
method to look for critical
in the severities
array. It will give you the first match it finds. If it doesn’t find anything, it will give you undefined
. So, if you get critical
back, that means you found a match. Another thing you can do is check if the severities array has a key called info
. Here’s how:console.log(severities.find(value => value === "info") !== undefined);
// Output: false
When you use find()
on severities array, you get undefined. That means none of the items in severities is info
. So, undefined === undefined
is false. JavaScript find()
is awesome because it can do anything you want. It can check any condition and any data type. But it has some drawbacks too. Some old browsers don’t like it.
How to check if a key exists in a JSON object or array
JSON
is a way to save and share data in JavaScript. You can use JSON
to store any kind of data, like text, numbers, true/false, nothing, or even more JSON! For example, you can make a JSON object with the details of a book
, like this:{
"title": "The Hitchhiker's Guide to the Galaxy",
"author": "Douglas Adams",
"genre": "Science Fiction",
"published": 1979
}
Or, you can create a JSON array
to store a list of books
, like this:
[
{
"title": "The Hitchhiker's Guide to the Galaxy",
"author": "Douglas Adams",
"genre": "Science Fiction",
"published": 1979
},
{
"title": "The Lord of the Rings",
"author": "J.R.R. Tolkien",
"genre": "Fantasy",
"published": 1954
},
{
"title": "Harry Potter and the Philosopher's Stone",
"author": "J.K. Rowling",
"genre": "Fantasy",
"published": 1997
}
]
JSON
data in JavaScript. JSON is a text format that can store any kind of data. You can turn a JSON text into a JavaScript object or array with the JSON.parse() method. It takes the JSON text as an input and gives you back a JavaScript object or array.Let’s try it with a JSON text that has some information about a book. Here’s the code:let book = JSON.parse(`{"title": "The Hitchhiker\'s Guide to the Galaxy",
"author": "Douglas Adams",
"genre": "Science Fiction",
"published": 1979}`);
Or, you can parse the JSON array that stores a list of books like this:
let books = JSON.parse(`[{"title": "The Hitchhiker\'s Guide to the Galaxy",
"author": "Douglas Adams", "genre": "Science Fiction", "published": 1979},
{"title": "The Lord of the Rings", "author": "J.R.R. Tolkien",
"genre": "Fantasy", "published": 1954}, {"title": "Harry Potter and
the Philosopher\'s Stone", "author": "J.K. Rowling",
"genre": "Fantasy", "published": 1997}]`);
book
object:console.log("title" in book); // Output: true
console.log(book.hasOwnProperty("title")); // Output: true
console.log(Object.keys(book).includes("title")); // Output: true
books
array like this:console.log(0 in books); // Output: true
console.log(books.indexOf(books[0]) !== -1); // Output: true
console.log(books.includes(books[0])); // Output: true
JSON.parse()
is awesome! It lets you use JSON data in JavaScript easily. But be careful, it needs a valid JSON string. If the string is bad or has wrong characters, it will give you an error.How to check if a key exists in an array of objects
students
and give them names
, grades
, hobbies, etc. Like this:let students = [
{
name: "Bob",
age: 18,
grade: 12
},
{
name: "Alice",
age: 17,
grade: 11
},
{
name: "Charlie",
age: 19,
grade: 12
}
];
name
, age
, and grade
, and each key has a different value for each object. To check if a key exists in an array of objects, you can use one of the following methods:1. Using the filter method() to check if exists in array of objects
You can use the JavaScript array method filter() to create a new array with only the items that match a condition. For example, let’s say you have an array of students
and you want to see which ones have a name key. You can write a function that returns true if the object has a name key and false otherwise. Then you can pass this function to filter()
and get a new array with only the students
that have a name key. Isn’t that awesome?
console.log(students.filter(student => "name" in student).length > 0);
// Output: true
filter()
method. It looks for objects with the name key in the students
array. Then it puts them in a new array. If the new array has any objects, it means true. You can also do the same thing with the score
key. Here’s how:console.log(students.filter(student => "score" in student).length > 0);
// Output: false
filter()
to find objects with a score
key. But it gives us an empty array here, so it’s false. JavaScript filter()
is good if we want to use the objects later, like sort them or show them. But it’s not simple or fast. It makes a new array and checks every element.2. Using the some() method to check if key exists
Do you want to know if an array of objects has a certain key? You can use the some()
method in JavaScript! This method is awesome because it returns true as soon as it finds one element that matches your condition. Otherwise, it returns false.
Let’s see how it works with an example. Suppose you have an array called students
that contains some objects. You want to check if any of these objects has the key name. You can do this by writing a function that takes an object and a key as parameters and returns true if the object has that key. Then, you can pass this function to the some()
method like this:
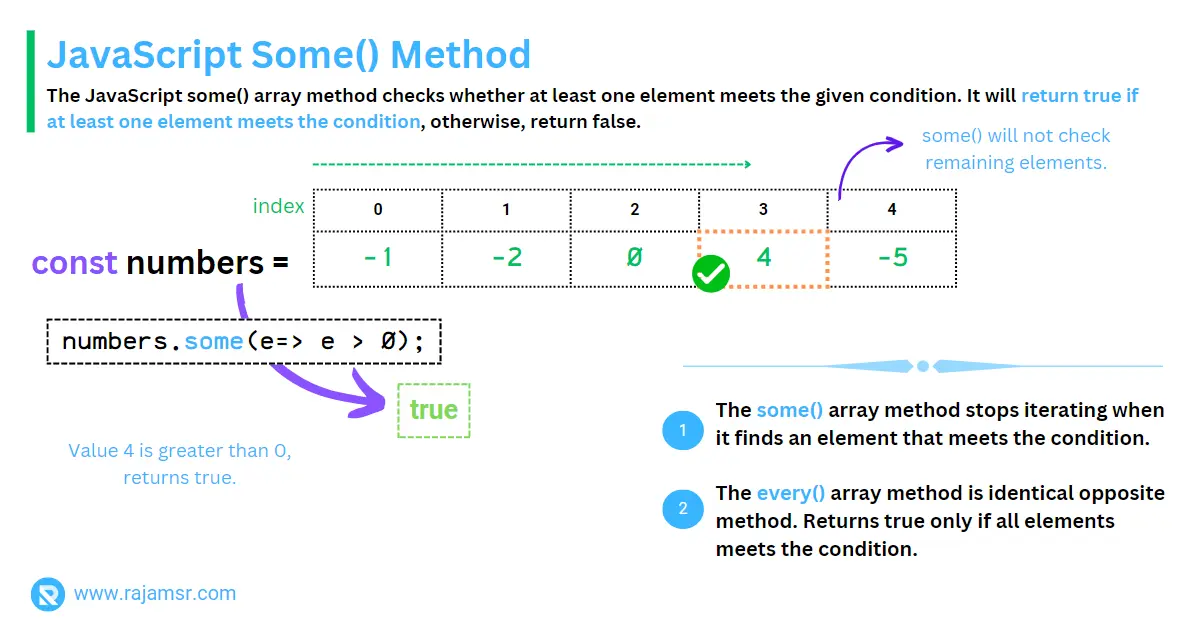
console.log(students.some(student => "name" in student));
// Output: true
some()
! It will give you true if any object has it. You can also look for score
like this:console.log(students.some(student => "score" in student));
// Output: false
some()
method is so cool! It can check if any object in an array has a certain key. For example, if we want to see if any student has a score, we can use some()
. But it will only give us true or false, not the actual objects. That’s okay, because sometimes we just need a quick answer. And some()
is faster than filter()
, because it stops when it finds a match.3. Using the every() method
Do you want to check if all objects in an array have a certain key? You can do that with the JavaScript every() method in JavaScript! This method takes a function that returns true or false for each object. For example, let’s say you have an array of students
and you want to see if they all have a name. You can write a every()
method like this:
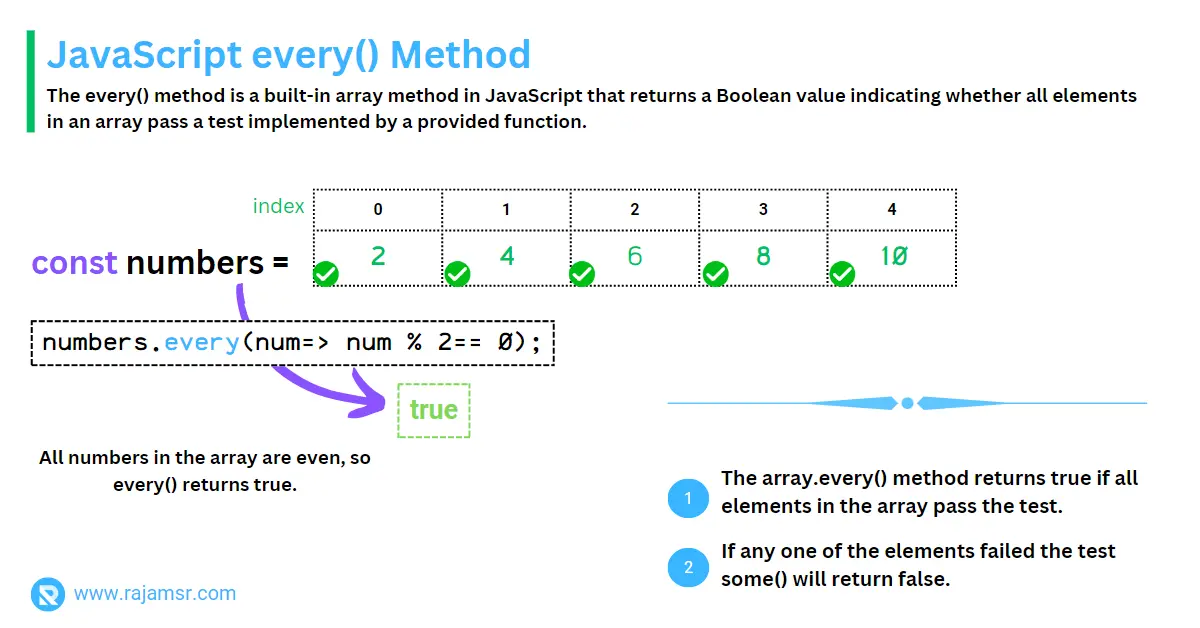
console.log(students.every(student => "name" in student));
// Output: true
every()
method correctly. It returns true because all the students have a name key. Want to try another challenge? See if you can find out if every student
has a score
key too. Here’s a hint:console.log(students.every(student => "score" in student));
// Output: false
every()
method! It’s very precise and strict. It won’t return true unless all the elements have the key score
. But be careful, it’s also more complex and less efficient. It has to loop through all the elements, even if it finds a false one.Check if a key exists in a Dictionary or a Map
JavaScript dictionaries or maps
are awesome! They let you store key-value pairs, where each key is unique and each value can be anything you want. They are like objects, but better, because they have some cool features, such as:
- A dictionary or a map can have any data type as a key, not just strings.
- A dictionary or a map preserves the insertion order of the key-value pairs, unlike an object.
- A dictionary or a map has some built-in methods and properties that make it easier to work with, such as size, get, set, has, delete, and clear.
A dictionary or a map is a great way to store data in JavaScript! You can make one with the Map
constructor, and pass it some data to start with. Let’s see how to make a map of colors
and their hex codes
.
let colors = new Map([
["red", "#FF0000"],
["green", "#00FF00"],
["blue", "#0000FF"]
]);
In this dictionary or map, red
, green
, and blue
are keys and #FF0000
, #00FF00
, and #0000FF
are values.
To check if a key exists in a dictionary or a map, you can use one of the following methods:
1. Using the get() method
The get()
method is awesome! It lets you find the value of a key in a dictionary or a map. If the key is not there, it gives you undefined
. You can use this to see if a key is in a dictionary or a map.
For example, you can see if green
is in the colors
dictionary or map like this:
console.log(colors.get("green") !== undefined);
// Output: true
You can get the value for a key in a dictionary or map. For example, colors.get("green")
gives you #00FF00
. That’s not undefined, so it’s true. You can also see if white
is a key in colors like this:
console.log(colors.get("white") !== undefined);
// Output: false
get()
method to look for the key white
in the colors dictionary. But what happens if the key is not there? You get undefined, right? And undefined
is not equal to undefined
, so the expression is false. The get()
method is great because it’s simple and neat. But it has a downside too.Sometimes, you might have keys that have undefined
as their value. Then, the get()
method can’t tell the difference and might give you wrong results.2. Using the has() method
Do you want to know if a dictionary or a map has a certain key? Just use the has()
method! It’s super easy and fast. Just give it the key you want to check and it will tell you if it’s there or not. For example, let’s say you have a colors
dictionary or map and you want to see if it has green
. Just do this:
console.log(colors.has("green"));
// Output: true
has()
method to see if a key is in a dictionary or map. For example, has("green")
returns true because green
is in the colors dictionary. What about has("white")
? Try it and see what happens!console.log(colors.has("white"));
// Output: false
white
in the colors map? No, right? That’s why has()
gives false. It’s a great method to check if a key exists or not. It’s better than get()
because it doesn’t care about the value of the key. The only downside is that it takes more words to write than get()
.JavaScript check if localStorage key exists
JavaScript localStorage
introduces its challenges. Verifying if a key exists can be accomplished by checking if the stored value is not null:
const key = 'myKey';
if (localStorage.getItem(key) !== null) {
console.log('Key exists in local storage!');
} else {
console.log('Key does not exist.');
}
This approach ensures you handle localStorage
specific scenarios, offering a reliable method for checking key existence.
JavaScript check if key exists ES6
ES6 brings a concise syntax to the table. The in
operator, when used with objects, provides a clean and efficient way to check for key existence:
const person = {
firstName: 'John',
lastName: 'Doe',
age: 30,
city: 'New Work'
};
console.log('firstName' in person); // Output: true
console.log('gender' in person); // Output: false
firstName
property. It uses the in
operator to do that. The person
object has a firstName
, so it says true. But if you look for gender
, it says false. That’s because the person
object doesn’t have that property.Frequently asked questions
Validate if a key exists in a JSON string object.
const personJsonString = `{ "firstName": "John", "lastName": "Doe", "age": 30, "city": "New Work" }` const keyToFind = 'age'; const jsonObject = JSON.parse(personJsonString); const keyExists = keyToFind in jsonObject; console.log(keyExists); // Output: true
const person = new Map([['firstName', 'John'], ['lastName', 'Doe'], ['city', 'New Work']]); const keyToFind ='city' const keyExists = person.has(keyToFind); console.log(keyExists); // Output: true
Conclusion
In this blog post, you learned how to check if a key exists in various data structures in JavaScript, such as objects, arrays, JSON, dictionaries, and maps. You also learned the pros and cons of different methods and techniques to check for key existence, and saw some code examples and output for each method.
I hope this blog post has helped you understand how to confidently check if a key exists in JavaScript.