One of the common challenges that JavaScript developers face is how to conditionally add keys to objects in JavaScript. This means adding new properties to your objects only when some criteria are met. For instance, imagine you’re creating a web app that displays user profiles. You have some basic details for each user, but you also want to include a new property to show if the user is verified or not. How can you achieve this without changing each object by hand?
The solution is to use ES6
the latest version of JavaScript, which brings many new features, such as the spread operator (...)
and object destructuring
.
In this blog post, we’ll explore:
- How to use
ES6
to conditionally add keys to objects in JavaScript? - Spread operator and object destructuring
- Conditionally adding properties to objects in arrays.
- How to dynamically add nested properties to objects
- How to add properties to
JSON
objects - Adding properties to lists of objects
Let’s get started.
Conditionally add key to object in JavaScript
The object is one of the non-primitive data types in JavaScript. Conditionally adding key-value pairs to JavaScript objects is a common task in web development.
When you want to add a new property to an object conditionally, the traditional approach involves using an if statement. For instance, let’s say we have an object representing a person
.
let person = {
name: "John",
age: 30,
};
If you want to conditionally add a property hasJob
only if the person has a job, you can do it like this:
if (hasJob) {
person.hasJob = true;
}
However, ES6 introduced a more concise way to conditionally add a key to an object using object destructuring:
const updatedPerson = {
...person,
...(hasJob && { hasJob: true }),
};
In this example, the spread operator ...
copies all existing properties from the person object to the updatedPerson
object. The second part of the expression, hasJob && { hasJob: true }
, only adds the hasJob
property if the hasJob
variable is true.
This approach makes the code cleaner and more readable, especially when you have multiple properties to conditionally add.
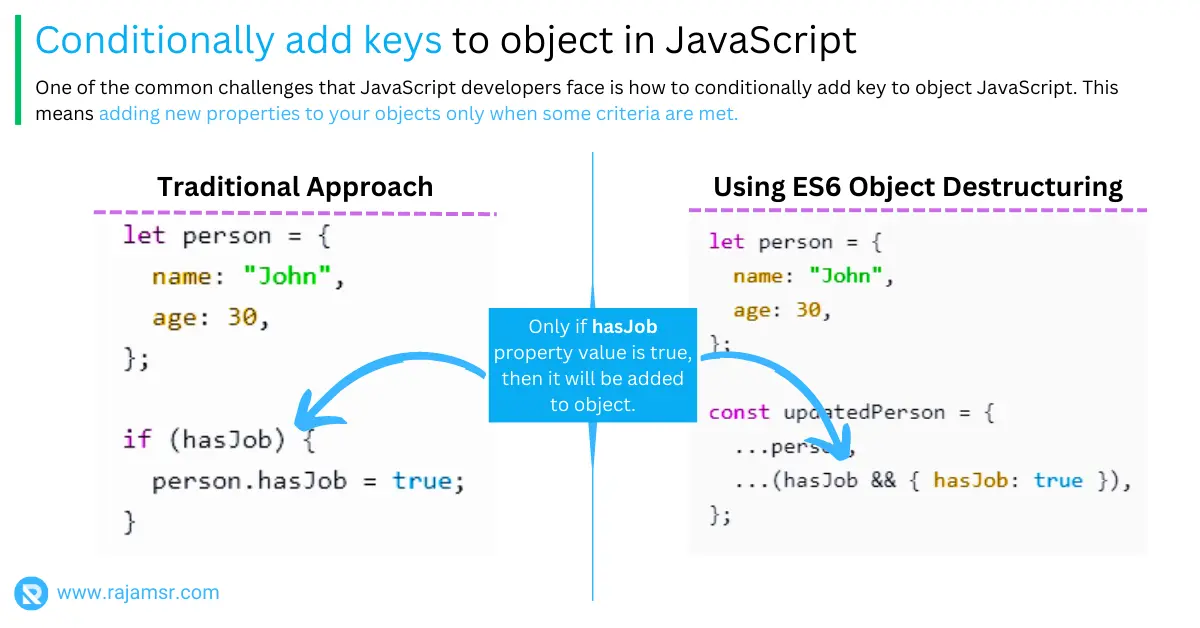
Optionally add key to object using ES6 spread operator
The ES6 spread operator is a powerful option in JavaScript that can be used for various operations, including conditionally adding properties to objects. Let’s explore how to utilize the spread operator
to add properties to objects conditionally.
Imagine you have an object representing a shopping cart.
let shoppingCart = {
items: [],
total: 0,
};
Now, let’s say you want to add an additional discount
property to the shopping cart object if the user is eligible for a discount
:
const eligibleForDiscount = true;
const updatedCart = {
...shoppingCart,
...(eligibleForDiscount && { discount: 10 }),
};
In this example, we’re using the spread operator to create a copy of the shoppingCart
object and adding a discount property conditionally based on the value of eligibleForDiscount
.
The spread operator’s concise syntax simplifies the process of adding properties conditionally and enhances the readability of your code.
Adding properties to objects in arrays with multiple conditions
In JavaScript, you often encounter scenarios where you need to add properties to objects within an array conditionally. Let’s explore how to achieve this by leveraging JavaScript array methods and the spread operator.
Consider an array of student
objects:
let students = [
{ name: "Alice", grade: "A" },
{ name: "Bob", grade: "B" },
// ...
];
Suppose we want to add a property isPassing
to each student based on their grade. Here’s how you can conditionally add the key to an object in JavaScript using the map()
function:
const updatedStudents = students.map(student => ({
...student,
isPassing: student.grade === "A" || student.grade === "B",
}));
In this example, we’re using the map()
function to iterate over each student
object, and for each student, we’re adding the isPassing
property based on their grade.
By combining the power of the array method map()
and the spread operator, you can efficiently add properties to objects within an array conditionally.
Using the spread operator, you can merge JavaScript objects.
Dynamically adding nested properties to objects
user
object:let user = {
name: "Alice",
info: {},
};
Suppose you want to add information to the property dynamically. You can use dot notation
or compute property names:
const key = "age";
const value = 30;
user.info[key] = value;
const updatedUser = {
...user,
info: {
...user.info,
[key]: value, // Using computed property name
},
};
In the second example, we’re using the spread operator
to maintain the existing properties of the user object while adding a new property to the nested info object using a computed property
name.
The dynamic addition of nested properties
enhances the flexibility of your code and accommodates varying data structures.
Adding properties to JSON objects dynamically
While JavaScript objects and JSON
objects are related, there are differences in their usage. Let’s explore how to dynamically add properties to JSON
objects while adhering to the JSON
format.
Consider a JSON object representing a book
:
let bookJSON = `{
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald"
}`;
Suppose we want to add a property called year
to indicate the publication year. To achieve this, we’ll need to parse the JSON
, add the property, and then stringify
it back:
const book = JSON.parse(bookJSON);
book.year = 1925;
const updatedBookJSON = JSON.stringify(book);
In this example, we’re using JSON.parse()
to convert the JSON
string to a JavaScript object, adding the year property, and then using JSON.stringify()
to convert it back to a JSON string.
Dynamic property addition to JSON
objects requires a combination of parsing and stringifying to maintain the JSON
format.
Conditionally add key to lists of objects
map()
function and the spread operator.Consider an array of products
:let products = [
{ name: "Shirt", price: 25 },
{ name: "Jeans", price: 50 },
// ...
];
Suppose we want to add a property called isAffordable
based on the price of each product. We can use the map()
function to achieve this:
const affordableThreshold = 30.00;
const updatedProducts = products.map(product => ({
...product,
isAffordable: product.price <= affordableThreshold,
}));
In this example, we’re using the map()
function to iterate over each product, adding the isAffordable
property based on the comparison with the affordableThreshold
.
By using array methods and the spread operator
, you can efficiently add properties to a list of objects and enhance the data representation.
Adding entries to JavaScript objects
The concept of object entries provides an interesting way to dynamically add key-value pairs
to objects. Let’s explore how to do this using the Object.entries()
method.
Consider an object representing a user
:
let user = {
name: "John",
age: 30,
};
Suppose we want to conditionally add an entry for the user’s address
. We can achieve this using the Object.entries()
method:
const addressKey = "address";
const addressValue = "123 Main St";
if (shouldAddAddress) {
user = {
...user,
...Object.fromEntries([[addressKey, addressValue]]),
};
}
In this example, the Object.fromEntries()
method is used to convert an array of key-value pairs
into an object. We spread this newly created object into the existing user object conditionally.
Adding entries using Object.entries()
can be a flexible way to dynamically extend objects with new key-value pairs.
Best practices for dynamic property addition
When dynamically adding properties to objects conditionally, following best practices ensures clean, efficient, and maintainable code.
- Use Immutability: Avoid modifying objects directly. Create new objects with the required properties to ensure immutability.
- Conditional Logic Clarity: Write clear and concise conditional logic to ensure that properties are added only when necessary.
- Error Handling: Account for cases where properties may not exist. Use optional chaining or provide default values.
- Readability Matters: Prioritize code readability over brevity. Clear code is easier to understand and maintain.
- Leverage ES6 Features: Utilize ES6 features like object destructuring and the spread operator for elegant property addition.
- Performance Optimization: Be mindful of performance implications, especially with deeply nested objects or large data sets.
- Consistency: Maintain a consistent coding style and approach across your codebase to enhance code maintainability.
By adhering to these best practices, you’ll ensure that your dynamically added properties enhance your codebase without introducing unnecessary complexities.
Conclusion
In JavaScript, dynamically adding properties to objects conditionally is a fundamental skill. By utilizing ES6
features like object destructuring
, the spread operator and array methods, you can elegantly extend objects with new key-value pairs.
Additionally, it covered best practices to be followed to ensure clean, efficient, and maintainable code.
These methods let you work with different kinds of data structures, such as nested objects, JSON
or arrays
of objects. You can use them to conditionally add keys to objects in JavaScript
which can improve your applications.