When it comes to printing an array in JavaScript, having the right methods at your disposal can make your code more efficient and readable. As JavaScript arrays are at the core of handling collections of data, knowing how to print them effectively is crucial for displaying information to users or for debugging purposes.
In this ultimate guide, we will explore:
- Various approaches to printing arrays in JavaScript
- Printing array elements to the console
- Converting arrays to strings
- Generating comma-separated values
- Printing arrays of objects
- Print array without using loop
Let’s dive in.
JavaScript print array to the console
The array is one of the non-primitive data types in JavaScript. Printing array elements to the console is a common debugging technique. Let’s dive into the code and see how it’s done:
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
codeSeverities.forEach((element) => {
console.log(element);
});
// Output:
"Blocker"
"Critical"
"Major"
"Minor"
We can use the forEach()
method to go through every item in the array. Then we can print each item to the console. That’s what we do in the example above.
Run the code in your browser’s developer console, and you’ll see each element printed on a separate line.
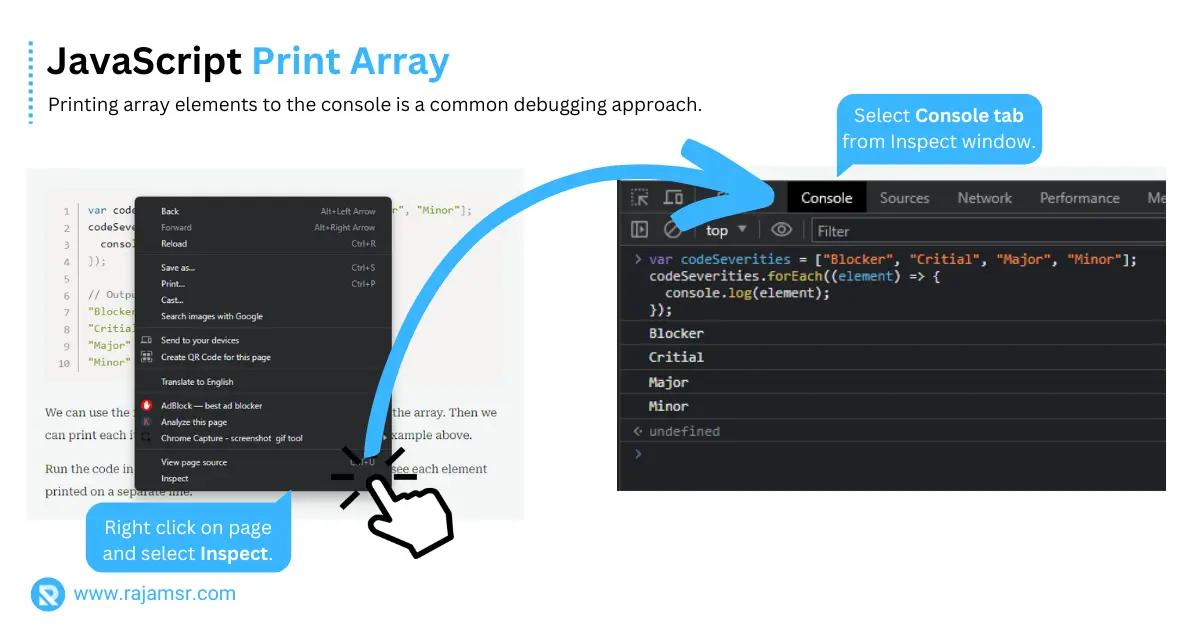
Print array of objects to the console
Printing an array of objects can be particularly useful when working with complex data structures. Let’s see how to print an array of objects to the console:
const users = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
{ name: 'Mark', age: 35 },
];
console.log(users);
// Output:
// {name: "John", age: 30}
// {name: "Jane", age: 25}
// {name: "Mark", age: 35}
In the code snippet above, we simply pass the array of objects to console.log()
, and the console will display the array’s contents.
Printing an array using the console.table() in JavaScript is the best way to visualize the data in JavaScript compared to using the console.log()
method.
If the above "users"
object array is printed using the console.table()
method will neatly print the values in table format.
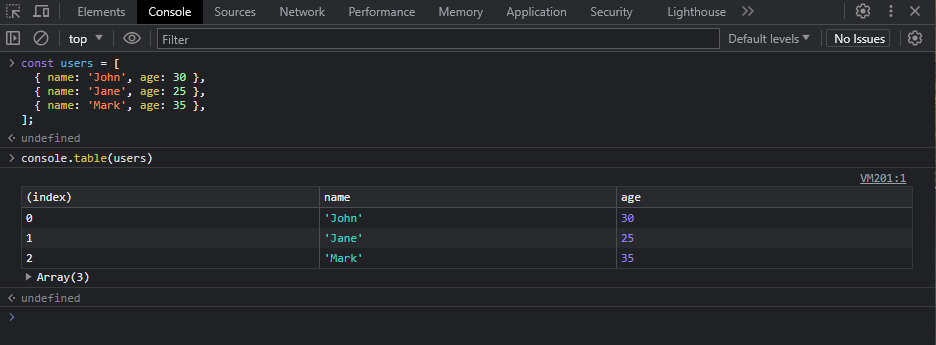
Print array of objects to HTML
Printing an array of objects in an HTML
format can be achieved by dynamically generating HTML
elements. Here’s an example:
const users = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
{ name: 'Mark', age: 35 },
];
const outputDiv = document.getElementById('output');
users.forEach((obj) => {
const p = document.createElement('p');
p.textContent = `Name: ${obj.name}, Age: ${obj.age}`;
outputDiv.appendChild(p);
});
In the code snippet above, we assume you have an HTML element with the ID "output"
to which we append dynamically created <p>
elements. Each <p>
element contains the name and age properties of each object in the array.
JavaScript print array values comma separated
If you want to display array values separated by commas, use the following approach:
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
const commaSeparated = codeSeverities.join(', ');
console.log(commaSeparated);
// Output: "Blocker, Critical, Major, Minor"
By using the join()
array method with a comma and space as the separator, you can transform your JavaScript array to a comma-separated string: "Blocker, Critical, Major, Minor"
.
Printing array values without commas
In certain scenarios, you may prefer printing array values without any separators. Let’s explore how to achieve this:
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
const commaSeparated = codeSeverities.join('');
console.log(commaSeparated);
// Output: "BlockerCriticalMajorMinor"
We replace the join()
method with an empty string without spaces in the code snippet above. The result is a string where array values are concatenated without any separators: "BlockerCriticalMajorMinor."
Print array elements on separate lines
When printing array elements on separate lines, you have a couple of options. One way is to use the join()
method with a new line separator:
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
const commaSeparated = codeSeverities.join('\n');
console.log(commaSeparated);
// Output:
// "Blocker"
// "Critical"
// "Major"
// "Minor"
By iterating through the array using a forEach()
loop, we can print each element on a separate line.
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
codeSeverities.forEach((element) => {
console.log(element);
});
// Output:
// "Blocker"
// "Critical"
// "Major"
// "Minor"
Both methods print each element on a new line.
Printing array elements as a string
Sometimes, you may need to convert an array into a single-string representation. Here’s a simple method to achieve that:
const words = ['JavaScript', 'runs', 'everywhere', 'on','everything!'];
const sentence = words.join(' ');
console.log(sentence);
// Output: "JavaScript runs everywhere on everything!"
You can make it one string from all the items in the array. You can do this with the join()
method by concatenating an array of strings. It puts a space
between each item when it joins them.
JavaScript print array with spaces
A simple way to turn an array into a string is to use join()
. This method lets you combine all the elements of the array with a separator of your choice. Here’s an example:
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
const commaSeparated = codeSeverities.join(' ');
console.log(commaSeparated);
// Output: "Blocker Critical Major Minor"
This code uses the join()
method to create a single string from the array elements. It adds a space between each element in the string.
How to print an array in JavaScript without a loop?
In JavaScript, you can use the console.log()
method directly on the array to print its elements without using a loop. Here’s an example:
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
console.log(...codeSeverities);
// Output: "Blocker"
// "Critical"
// "Major"
// "Minor"
The spread operator (...)
is used to spread the elements of the array as individual arguments toconsole.log()
.
Alternatively, you can use JSON.stringyfy()
method to print JavaScript array elements without using a for loop.
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"];
console.log(JSON.stringify( codeSeverities));
// Output: ["Blocker","Critical","Major","Minor"]
The JSON.stringyfy()
method will print an array object in string representation.
Frequently asked questions
Iterate through the array using forEach()
and log each element with its index.
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"]; codeSeverities.forEach((element, index) => { console.log(`Index ${index}: ${element}`); }); // Output: /* Index 0: Blocker Index 1: Critical Index 2: Major Index 3: Minor */
Use the JavaScript slice(0, -1) method to create a new array excluding the last element and log it.
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"]; const elementsExceptLast = codeSeverities.slice(0, -1); console.log(elementsExceptLast); // Output: ["Blocker", "Critical", "Major"]
Use the JSON.stringify()
method to convert the array into a JSON-formatted string.
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"]; const jsonArray = JSON.stringify(codeSeverities); console.log(jsonArray); // Output: ["Blocker","Critical","Major","Minor"]
Reverse the array using reverse() and log the reversed array.
var codeSeverities = ["Blocker", "Critical", "Major", "Minor"]; const reversedArray = codeSeverities.reverse(); console.log(reversedArray); // Output: ["Minor", "Major", "Critical", "Blocker"]
Conclusion
You have now mastered the art of printing arrays in JavaScript. I have covered various techniques, including printing elements to the console, creating strings, generating comma-separated values, and pretty much printing arrays of objects. With these skills in your arsenal, you can effectively display an array of data to meet your specific needs.
Keep experimenting and exploring additional JavaScript array printing techniques to enhance your coding abilities.