JavaScript optional parameters are a powerful feature that can make your functions more flexible, concise, and adaptable. But sometimes, they can also be tricky to work with. For example, how do you handle different numbers and types of arguments? How do you make some parameters optional, so that you can skip them or use a default value?
Optional parameters are a great solution for this problem. They let you write functions that can adapt to different situations and needs. They make your code more concise and flexible. But they also have some rules and pitfalls that you need to know. Otherwise, you may end up with errors, bugs, or surprises in your code.
In this blog, I will cover the following topics:
- Definition of optional parameters in JavaScript
- How to skip optional parameters in JavaScript using the undefined value
- How to use objects as optional parameters in JavaScript to group related values and avoid order dependency
- How to use optional parameters in constructors in JavaScript to create objects with different properties
- Best practices for using optional parameters in JavaScript
Optional parameters in JavaScript
Optional parameters in JavaScript are parameters that are not required to be passed when calling a function. They allow you to define default values for parameters, making them optional for the caller.
For example, you can create a function that says hello to someone with their name and title. But what if you don’t know their title? No problem! You can use the = operator to give them a default one. Look at this code:
function greet(name, title) {
console.log(`Hello, ${title} ${name}!`);
}
greet("Alice", "Ms.")
, which prints Hello, Ms. Alice!
. That’s nice, right? But what if you don’t know their title, or they don’t have one? No problem! You can make the title parameter optional by giving it a default value of "Sir/Madam"
using the =
operator. That way, if you don’t provide a title, the function will use the default one and print something like Hello, Sir/Madam John!
. How simple is that?function greet(name, title = "Sir/Madam") {
console.log(`Hello, ${title} ${name}!`);
}
Now, you can call the function with or without the title argument, and it will work as expected:
greet("Alice", "Ms."); // Output: "Hello, Ms. Alice!"
greet("Bob"); // Output: "Hello, Sir/Madam Bob!"
Multiple optional parameters
multiple optional parameters
with default values, as long as they are at the end of the parameter list. For example, let’s say you want to write a function that calculates the area of a rectangle. You need to give it a length and a width, but you can also choose a unit. If you don’t specify a unit, it will use the default one.Here’s how you can write such a function with multiple optional:function area(length, width = 1, unit = "cm") {
console.log(`The area is ${length * width} ${unit}^2.`);
}
This function is super flexible! You can pass different arguments to it and it will work fine. If you don’t give it a unit, it will use the default one.
area(10, 5, "m"); // Output: "The area is 50 m^2."
area(10, 5); // Output: "The area is 50 cm^2.
area(10); // Output: "The area is 10 cm^2" (width defaults to 1)
Skip optional parameters in JavaScript
Here’s a way to make your code more readable and flexible. Imagine you have a function that lets you format a date with different parts: year
, month
, day
, and separator. But what if you don’t want to use the separator? You can make it optional by using a default value. That way, you can pass only the parameters you need and get the desired output.
function formatDate(year, month, day, separator = "-") {
console.log(`${year}${separator}${month}${separator}${day}`);
}
formatDate(2024, 2, 5, "/")
gives you 2024/2/5
. But what if you only want to show the year and the month? No problem! Just use undefined for the parameters you don’t need:formatDate(2024, 2, undefined, undefined); // 2024-2-undefined
2024/2/undefined
, which is not what you want. To fix this, you need to use the undefined value for the separator as well, and let the function use the default value:formatDate(2024, 2, undefined, "/"); // 2024/2/undefined
2024-2-undefined
when you print dates. That’s not good, right? You need to use a different way to write optional parameters. We’ll learn that soon.But first, let’s talk about undefined
and null
. They are different things in JavaScript. Undefined
means you didn’t give a value to a JavaScript variable or a parameter. Null
means you gave an empty or non-existent value on purpose.For example, look at this function that checks if a parameter is undefined or null:function check(param) {
if (param === undefined) {
console.log("The parameter is undefined.");
} else if (param === null) {
console.log("The parameter is null.");
} else {
console.log("The parameter is neither undefined nor null.");
}
}
You can call this function with different values and see the difference:
check(); // Output: "The parameter is undefined."
check(undefined); // Output: "The parameter is undefined."
check(null); // Output: "The parameter is null."
check(0); // Output: "The parameter is neither undefined nor null."
check(""); // Output: "The parameter is neither undefined nor null."
Use objects as optional parameters in JavaScript
- Organizing related values into one object makes your code more elegant and readable.
- The order of the parameters doesn’t matter. Just make sure to use the correct property names.
- You have the flexibility to pass any number of optional parameters. No need to fill in the gaps with undefined.
subject
, a body
, a recipient
, and some optional options. The options are cc
, bcc
, attachments
, and priority
:function sendEmail(subject, body, recipient, options) {
// ...
}
spread operator (...)
to copy the existing options. Then you can change only the ones you want. This way, you don’t have to worry about undefined values.Look how neat and easy it is:function sendEmail(subject, body, recipient,
options = {
cc: "",
bcc: "",
attachments: [],
priority: "normal"
}) {
// ...
}
sendEmail("Hello", "How are you?", "alice@example.com",
{ cc: "bob@example.com", priority: "high" }); // OK
sendEmail("Hello", "How are you?", "alice@example.com", undefined, "high");
// Error: too many arguments
sendEmail("Hello", "How are you?", "alice@example.com",
{ cc: undefined, bcc: undefined, attachments: undefined, priority: "high" });
// OK, but ugly
Using the object as an optional parameter method, you can simplify the code and make it more flexible:
// Using object as an optional parameter
function sendEmail(subject, body, recipient,
{ cc = "",
bcc = "",
attachments = [],
priority = "normal" } = {}) {
// ...
}
sendEmail("Hello", "How are you?", "alice@example.com",
{ cc: "bob@example.com", priority: "high" }); // OK
sendEmail("Hello", "How are you?", "alice@example.com",
{ priority: "high" });
// OK
sendEmail("Hello", "How are you?", "alice@example.com"); // OK
You use object destructuring
to get the options object properties. You also give them default values with the =
operator. If you don’t pass any options, you use an empty object by default.
You can also use the JavaScript double question mark ?? operator to give default values. This is a new JavaScript feature that returns the right value if the left value is null or undefined. Otherwise, it returns the left value. Here’s how you can use it in the sendEmail()
function:
function sendEmail(subject, body, recipient,
{ cc, bcc, attachments, priority } = {}) {
cc = cc ?? "";
bcc = bcc ?? "";
attachments = attachments ?? [];
priority = priority ?? "normal";
// ...
}
This is equivalent to the previous version, but it may be more concise and clear in some cases.
JavaScript optional parameters in constructor
Optional parameters let you create objects with different properties in JavaScript. For example, you can make a person
object with a name
, an age
, and maybe an occupation
. You use a special function called a constructor to do this.
Here is an example of constructor with an optional parameter:
// Using optional parameter in constructors
function Person(name, age, occupation = "unemployed") {
this.name = name;
this.age = age;
this.occupation = occupation;
}
This is how you make person
objects with a constructor. The this
keyword links the parameters to the object. You can also give a default value to a parameter with the =
sign. Look at these examples of person
objects with different arguments:
let alice = new Person("Alice", 25, "teacher");
let bob = new Person("Bob", 30);
let charlie = new Person("Charlie", 35, "engineer");
person
objects using the dot notation:console.log(alice.name); // Output: "Alice"
console.log(bob.occupation); // Output: "unemployed"
console.log(charlie.age); // Output: 35
Optional parameters are awesome! They let you make objects with different properties easily. You don’t need to write many constructors or use if-else
statements. Just pass the values you want and you’re done!
Best practices for using optional parameters in JavaScript
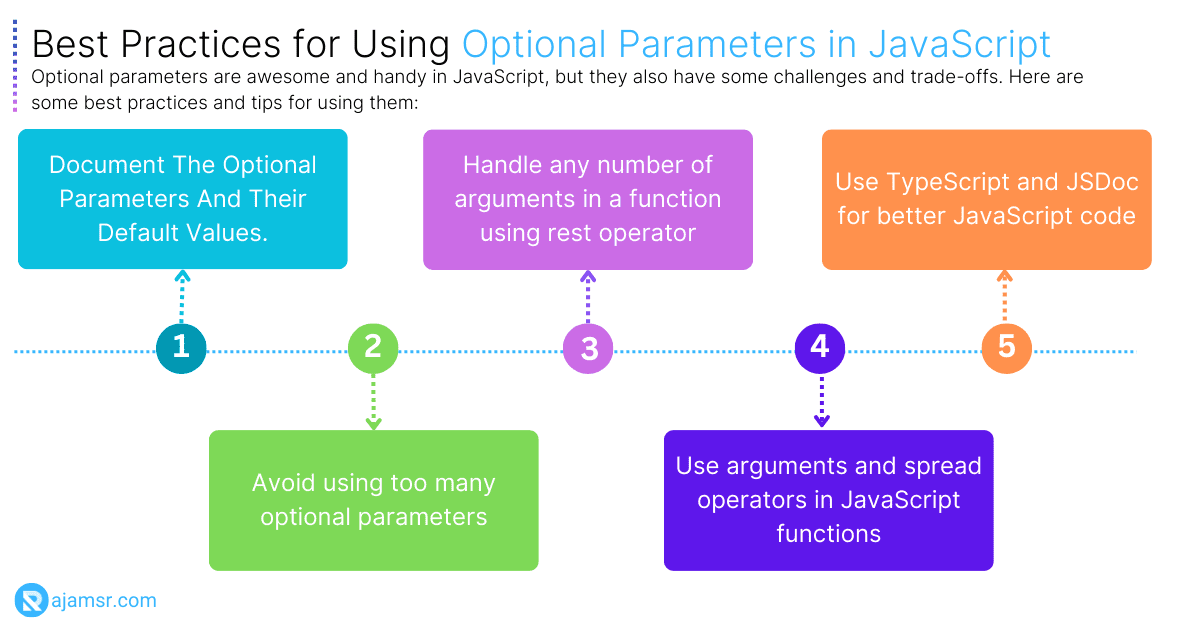
Optional parameters are awesome and handy in JavaScript, but they also have some challenges and trade-offs. Here are some best practices and tips for using them:
1. Document the optional parameters and their default values
This will help others understand how to use your functions and what they do. You can use JavaScript comments, JSDoc
, TypeScript
, or other tools for this.
2. Avoid using too many optional parameters
They can make your code hard to read, maintain, and test. They can also cause errors and bugs. Try to use a reasonable number of optional parameters, and use objects or arrays to group related values if needed.
3. Handle any number of arguments in a function using rest operator
Sometimes you don’t know how many arguments a function will get. Don’t worry, JavaScript has you covered! You can use the arguments object or the … rest operator to get all the arguments easily. The arguments object is a special thing that holds all the arguments in a function.
The … operator is a cool new ES6 feature that lets you spread an array or an object into separate pieces. Both of them can help you work with any number of arguments in a function, even if they are not in the parameters.
4. Use arguments and spread operators in JavaScript functions
Sometimes you want to use all the arguments in a function, even if you don’t know how many there are. You can do that with the arguments object or the spread operator (…). The arguments object is a special thing that has all the arguments inside it.
The … operator is a cool new thing in JavaScript that lets you spread an array or an object into separate pieces. Both of them can help you get and change all the arguments in a function, no matter what parameters you have.
5. Use TypeScript and JSDoc for better JavaScript code
Want to write better code with fewer errors? Try TypeScript
or JSDoc
! TypeScript adds types to JavaScript, so you can catch bugs before they happen. JSDoc creates docs from your comments, so you can document your functions easily. Both TypeScript and JSDoc let you use the ? operator for optional parameters. This way, you can show which parameters are optional and what types they have. It’s simple and useful!
Conclusion
In this blog, You have learned how to use optional parameters in JavaScript, and what are the benefits and best practices of doing so. You have seen how to:
- Define optional parameters with the = operator
- Skip optional parameters with the undefined value
- Group optional parameters into objects to avoid order issues
- Use optional parameters in constructors to make different objects
- Document, check, and mark optional parameters with various tools and operators
Optional parameters let you write flexible and elegant JavaScript code. But they also have some pitfalls and drawbacks, so use them with caution and care.
Thank you for reading this blog and I hope you found it useful. Feel free to experiment with the examples and share your thoughts.