If you are a web developer, you might have wondered how to JavaScript compare dates in your projects. Comparing dates is a common task that can be tricky and frustrating if you don’t know the best practices and methods.
Comparing dates in JavaScript can be tricky. There are many factors to consider, such as the format, the time zone, the accuracy, and the purpose of the comparison. How do you know if two dates are equal, greater than, or less than each other? How do you compare dates without time or by day? How do you compare dates in different time zones?
In this blog post, you will learn:
- JavaScript date comparison methods and tips
- Comparing dates without considering time in JavaScript
- Comparing dates and times in JavaScript
- How to check if two dates have the same day in JavaScript
- Dealing with different time zones when comparing dates in JavaScript
- Using libraries to simplify date comparison in JavaScript
By the end of this post, you will be able to compare dates in JavaScript like a pro!
How to compare dates in JavaScript?
getTime()
method. These are the best tools for working with dates and times in JavaScript. Let me show you why and how to use them.The Date
object is a special kind of object that stores a date and time. You can make a new Date object by giving it a string, a number, or nothing at all. For examplelet date1 = new Date(); // Current date and time
let date2 = new Date("6/01/2022"); // Specific date string
let date3 = new Date(2022, 5, 1); // Specific date (year, month, day)
The getTime()
method is a wonderful way to work with dates in JavaScript. It gives you a number that represents the exact moment in time when a date object was created. This number is the amount of milliseconds that have passed since the start of 1970
, which is a special date in computer science. Why is this number so useful? Because you can easily compare different dates with each other using simple math. You can check if one date is before, after, or equal to another date by comparing their numbers. For example:
let date1 = new Date();
let date2 = new Date("6/01/2022");
let date3 = new Date(2022, 5, 1);
console.log(date1.getTime() > date2.getTime()); // false
console.log(date2.getTime() == date3.getTime()); // true
console.log(date3.getTime() < date1.getTime()); // false
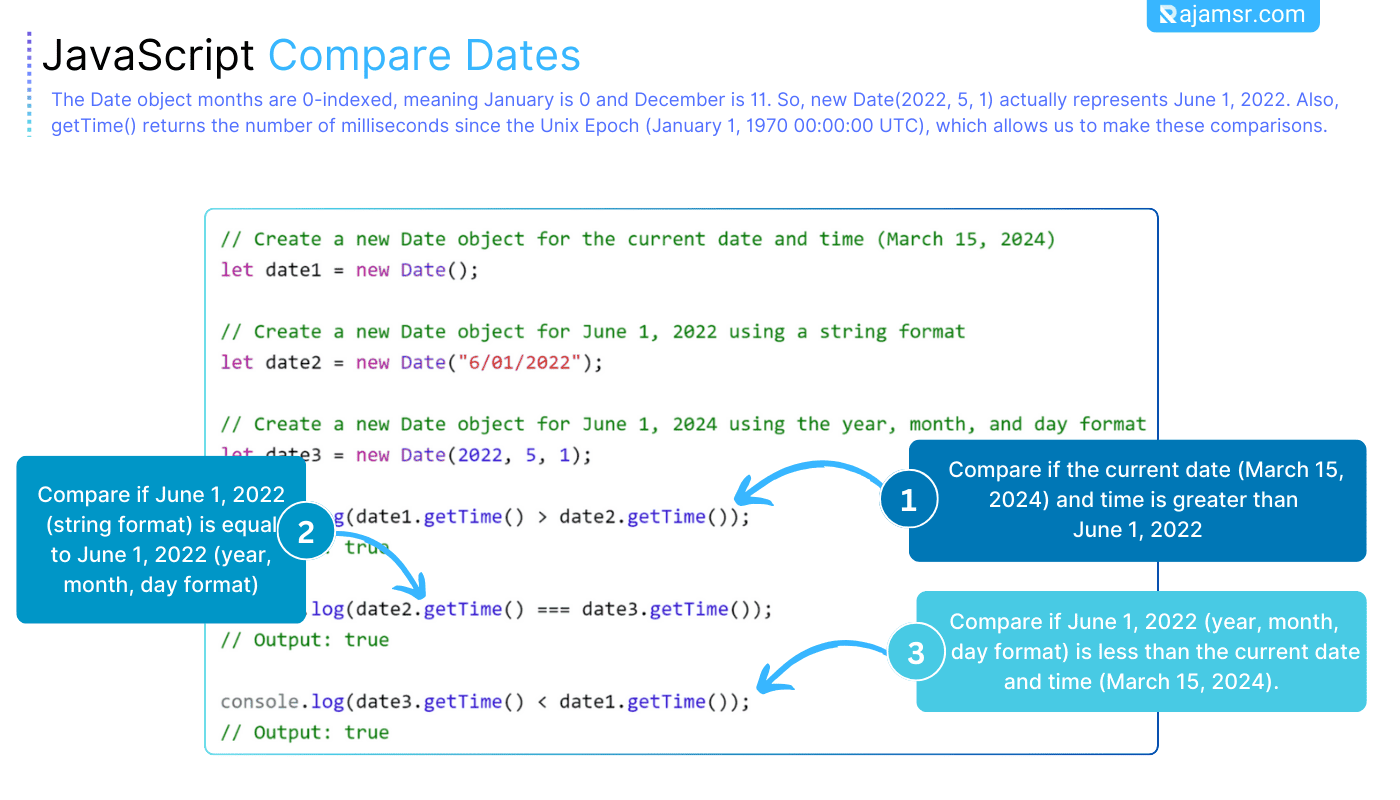
getTime()
method. You can use any format, timezone
, or leap year for the dates. And you don’t need any extra libraries like moment.js
that can make your code heavier.Compare dates without time in JavaScript
How do you compare dates in JavaScript without caring about the time? You might need this for birthdays, coupons, or other things that depend on the date only.
There are two ways to do this in JavaScript: with strings or with numbers. Let me show you how they work.
Compare dates without time using strings
toDateString()
method. It gives you a string like this: “Weekday Month Day Year”
. For example:let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1String = date1.toDateString(); // "Tue Jan 29 2024"
let date2String = date2.toDateString(); // "Tue Jan 29 2024"
Now, you can compare the strings using the comparison operators (<, >, ==, etc.). For example:
console.log(date1String === date2String); // Output: true
console.log(date1String < date2String); // Output: false
console.log(date1String > date2String); // Output: false
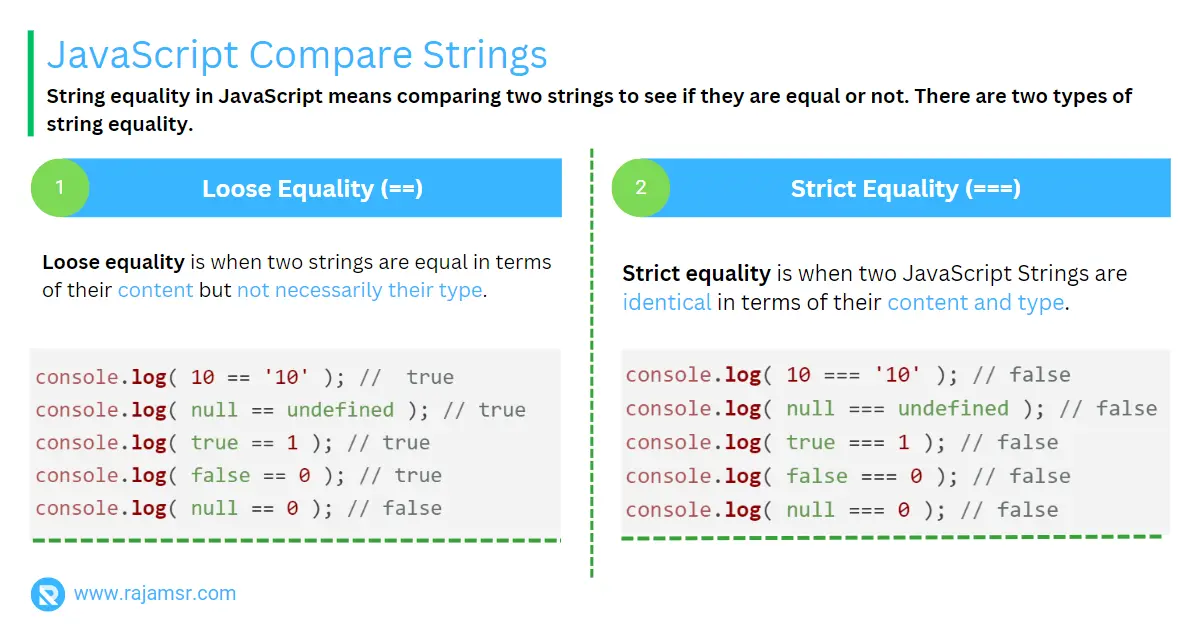
As you can see, the strings are equal, because they have the same date part, even though the time part is different.
Compare dates without time using numbers
getTime()
method. It gives you a number like this: "Milliseconds"
. Here’s an example:let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1Number = date1.getTime(); // 1709286888000
let date2Number = date2.getTime(); // 1709332800000
Now, you can compare the numbers using the comparison operators (<, >, ==, etc.). For example:
console.log(date1Number === date2Number); // Output: false
console.log(date1Number < date2Number); // Output: true
console.log(date1Number > date2Number); // Output: false
Look at these numbers. They are not the same, right? That’s because they have different times, even though the dates are the same.
But how can you compare dates without times using numbers? You can do it by rounding down the milliseconds to the closest day. You can use the JavaScript Math.floor() function for that. It gives you the biggest whole number that is smaller or equal to our number. For example:
let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1Number = date1.getTime(); // 1709286888000
let date2Number = date2.getTime(); // 1709332800000
let numberOfMillisecondsPerDay = (1000 * 60 * 60 * 24);
let date1Day = Math.floor(date1Number / numberOfMillisecondsPerDay); // Output: 19767
let date2Day = Math.floor(date2Number / numberOfMillisecondsPerDay); // Output: 19767
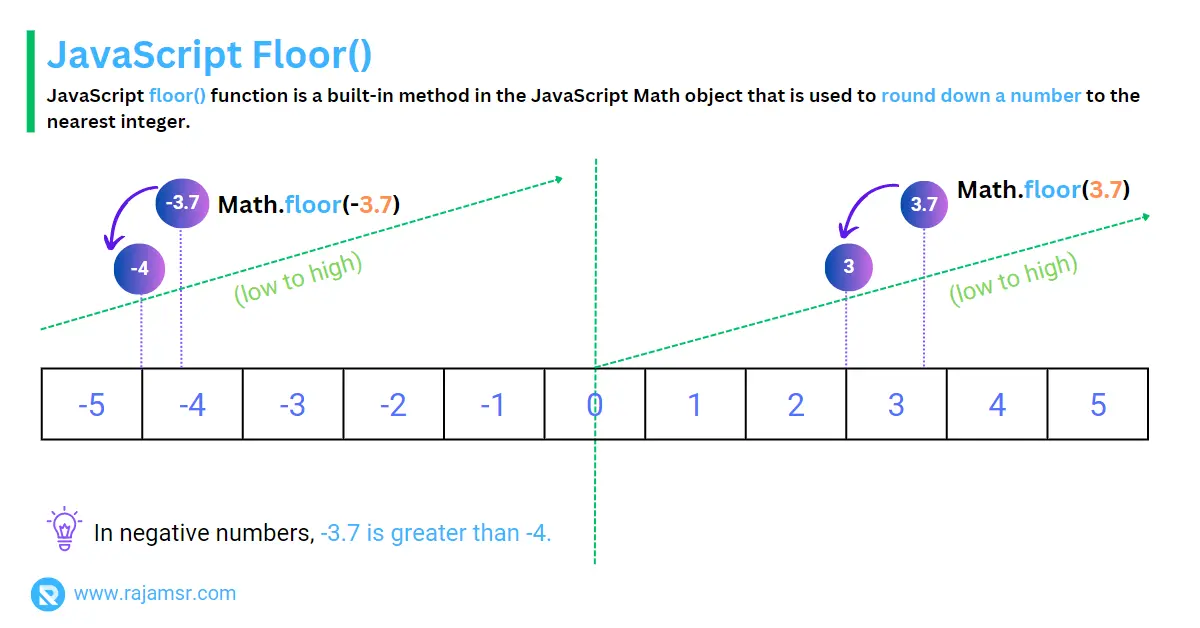
Now, you can compare the numbers using the comparison operators (<, >, ==, etc.). For example:
console.log(date1Day === date2Day); // Output: true
console.log(date1Day < date2Day); // Output: false
console.log(date1Day > date2Day); // Output: false
As you can see, the numbers are equal, because they have the same date part, after rounding down to the nearest day.
JavaScript compare dates with time in JavaScript
Sometimes you need to compare dates and times in JavaScript. This is handy when you care about the exact moment of the date, not just the day. For example, you might want to see if an event has started, ended, or is still going on, or if a deadline is over or coming soon.
You can compare dates and times in JavaScript in two main ways: using strings or using numbers. Let’s learn how they work.
Compare dates with time using strings
You can compare dates with time in JavaScript by using ISO 8601 format strings. They are easy to read and understand. They look like in the date format YYYY-MM-DDTHH:mm:ss.sssZ. Here’s an example:
let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1String = date1.toISOString(); // "2024-01-29T03:34:48.000Z"
let date2String = date2.toISOString(); // "2024-01-29T15:00:00.000Z"
Now, you can compare the strings using the comparison operators (<, >, ==, etc.). For example:
console.log(date1String === date2String); // Output: false
console.log(date1String < date2String); // Output: true
console.log(date1String > date2String); // Output: false
As you can see, the strings are not equal, because they have different time parts, even though the date part is the same.
Compare dates with time using numbers
Here’s a fun way to compare dates in JavaScript: turn them into numbers! How? Just use the getTime()
method. It gives you a number that means "how many milliseconds have passed since January 1, 1970"
. Like this:
let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1Number = date1.getTime(); // 1709286888000
let date2Number = date2.getTime(); // 1709332800000
Now, you can compare the numbers using the comparison operators (<, >, ==, etc.). For example:
console.log(date1Number === date2Number); // Output: false
console.log(date1Number < date2Number); // Output: true
console.log(date1Number > date2Number); // Output: false
As you can see, the numbers are not equal, because they have different time parts, even though the date part is the same.
Compare dates by day in JavaScript
How awesome is it to compare dates by day in JavaScript? You can do amazing things like grouping data by date or showing a calendar of events. You just need to use the date components. Let me show you how.
Using Date Components
getDate()
, getDay()
, getMonth()
, and getFullYear()
methods, which return numbers in the format “Day”, “Weekday”, “Month”, and “Year”. For example:let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1Day = date1.getDate(); // 29
let date1Weekday = date1.getDay(); // 2
let date1Month = date1.getMonth(); // 0
let date1Year = date1.getFullYear(); // 2024
let date2Day = date2.getDate(); // 29
let date2Weekday = date2.getDay(); // 2
let date2Month = date2.getMonth(); // 0
let date2Year = date2.getFullYear(); // 2024
Now, you can compare the numbers using the comparison operators (<, >, ==, etc.). For example:
console.log(date1Day === date2Day); // Output: true
console.log(date1Weekday === date2Weekday); // Output: true
console.log(date1Month === date2Month); // Output: true
console.log(date1Year === date2Year); // Output: true
As you can see, the numbers are equal, because they have the same date components, even though the time part is different.
Compare dates in different time zones in JavaScript
How cool is it to compare dates in different time zones in JavaScript? You can adjust the hours and minutes to match the local time or the universal time. For example, you can show the local time of an event in another country, or make the user’s input consistent with a standard time.
To do this, you need to use the time zone offset. It’s a simple and powerful way to compare dates. Let me show you how.
Using Time Zone Offset
UTC (Universal Time)
. How do you get it? Easy! Just use the getTimezoneOffset()
method. It gives you a number like this: "Minutes"
. For example:let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1Offset = date1.getTimezoneOffset(); // 0
let date2Offset = date2.getTimezoneOffset(); // 0
date1
is in India and date2
is in Japan. India is 5.5 hours ahead of UTC, and Japan is 9 hours ahead. So, the time zone offset for date1 is +05:30
, and for date2 is +09:00
. This means date1 and date2 have different offsets from UTC. Here’s an example: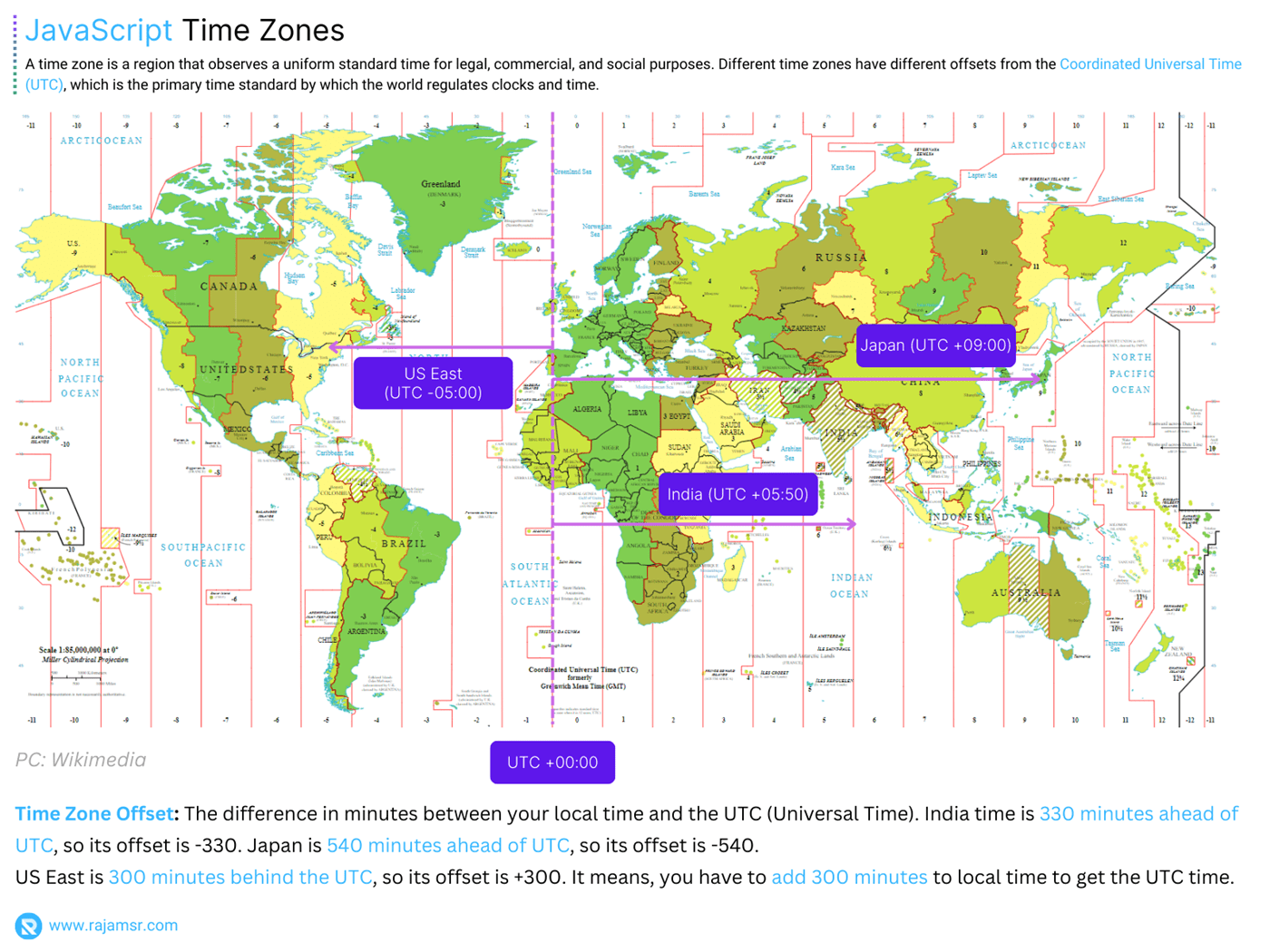
let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
let date1Offset = date1.getTimezoneOffset(); // 0
let date2Offset = date2.getTimezoneOffset(); // 0
// Adjust the dates to the local time zones
date1.setTime(date1.getTime() + date1Offset * 60 * 1000); // Tue Jan 29 2024 09:04:48 GMT+0530
date2.setTime(date2.getTime() + date2Offset * 60 * 1000); // Tue Jan 29 2024 18:00:00 GMT+0900
// Get the new time zone offsets
date1Offset = date1.getTimezoneOffset(); // -330
date2Offset = date2.getTimezoneOffset(); // -540
Date1
is 330
minutes ahead of UTC, so its offset is -330
. Date2
is 540
minutes ahead of UTC, so its offset is -540
.
You can check which date is earlier or later with symbols like <, >, and ==. For example:
console.log(date1 === date2); // Output: false
console.log(date1 < date2); // Output: true
console.log(date1 > date2); // Output: false
As you can see, the dates are not equal, because they have different time zones, even though the UTC time is the same.
Compare dates using libraries in JavaScript
Comparing dates in JavaScript can be tricky. But don’t worry, there are some awesome libraries that make it easy and fun. You can use them to write dates in natural language, relative time, or custom formats. How cool is that?
Some of the best libraries for date comparison are Moment.js
, Day.js
, and date-fns
. Let’s explore how they work and what they can do for you.
Moment.js
"today is Monday"
or "3 hours ago"
or "12/31/2020"
.To start with Moment.js
, you need to install it and import it into your JavaScript project. For example:// Install Moment.js using npm
npm install moment
// Import Moment.js in your JavaScript file
const moment = require("moment");
Moment.js
makes it easy to compare dates. Just create moment objects from whatever you have: date objects, strings, or numbers. Use the moment()
function with any arguments or formats you like. For example:let date1 = new Date("2024-01-29T03:34:48.000Z"); // Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z"); // Tue Jan 29 2024 15:00:00 GMT+0000
// Create a moment object from a date object
let moment1 = moment(date1);
let moment2 = moment(date2);
// Create a moment object from a string in ISO 8601 format
let moment3 = moment("2024-01-29T03:34:48.000Z");
let moment4 = moment("2024-01-29T15:00:00.000Z");
// Create a moment object from a number in milliseconds
let moment5 = moment(1709286888000);
let moment6 = moment(1709332800000);
Moment.js
! You can compare different moments and see which one is earlier, later, or the same as another. You can use simple methods like isBefore()
, isAfter()
, isSame()
, and more. They give you a true or false answer. Here’s an example:console.log(moment1.isBefore(moment2)); // Output: true
console.log(moment1.isAfter(moment2)); // Output: false
console.log(moment1.isSame(moment2)); // Output: false
console.log(moment1.isSameOrBefore(moment2)); // Output: true
console.log(moment1.isSameOrAfter(moment2)); // Output: false
local()
or utcOffset()
. Easy, right?Day.js
Day.js
lets you work with dates and times in JavaScript easily and quickly. It is like Moment.js, but smaller and faster. It also has many plugins that add more features and options. For example, you can say things like "today is before yesterday"
or "3 hours ago"
or "12/31/2020"
with Day.js
.To use Day.js
, you need to download it and add it to your JavaScript project. For example:// Install Day.js using npm
npm install dayjs
// Import Day.js in your JavaScript file
const dayjs = require("dayjs");
dayjs()
function with different inputs and formats. Here’s an example:let date1 = new Date("2024-01-29T03:34:48.000Z");
// Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z");
// Tue Jan 29 2024 15:00:00 GMT+0000
// Create a dayjs object from a date object
let day1 = dayjs(date1);
let day2 = dayjs(date2);
// Create a dayjs object from a string in ISO 8601 format
let day3 = dayjs("2024-01-29T03:34:48.000Z");
let day4 = dayjs("2024-01-29T15:00:00.000Z");
// Create a dayjs object from a number in milliseconds
let day5 = dayjs(1709286888000);
let day6 = dayjs(1709332800000);
dayjs
objects with Day.js
. It has awesome methods like isBefore()
, isAfter()
, isSame()
, and more. They give you a true or false answer. For example:consol.log(day1.isBefore(day2)); // Output: true
consol.log(day1.isAfter(day2)); // Output: false
consol.log(day1.isSame(day2)); // Output: false
consol.log(day1.isSameOrBefore(day2)); // Output: true
consol.log(day1.isSameOrAfter(day2)); // Output: false
Dayjs
objects and date objects have the same UTC time. That’s how we compare them. But sometimes you need a different time zone. No problem! Just use local()
or utcOffset()
to change the dayjs
objects to the time zone you want.date-fns compare dates
Date-fns is a modern and modular library that lets you do amazing things with dates and times. You can parse, sort dates, and format them in any way you want. You can also use plugins to add more features, like natural language comparisons, relative time, or custom formats.
Using date-fns
is easy. Just install it and import it in your project. Here’s how
// Install date-fns using npm
npm install date-fns
// Import date-fns in your JavaScript file
const { compareAsc, compareDesc, format,
formatDistance, formatRelative, isAfter,
isBefore, isEqual, isSameDay,
isSameMonth, isSameYear } = require("date-fns");
date-fns
in different ways. You can use date objects, strings, or numbers. To make date objects from strings or numbers, use the Date object or parseISO()
. For example:let date1 = new Date("2024-01-29T03:34:48.000Z");
// Tue Jan 29 2024 03:34:48 GMT+0000
let date2 = new Date("2024-01-29T15:00:00.000Z");
// Tue Jan 29 2024 15:00:00 GMT+0000
let date3 = parseISO("2024-01-29T03:34:48.000Z");
// Tue Jan 29 2024 03:34:48 GMT+0000
let date4 = parseISO("2024-01-29T15:00:00.000Z");
// Tue Jan 29 2024 15:00:00 GMT+0000
let date5 = parseISO(1709286888000);
// Tue Jan 29 2024 03:34:48 GMT+0000
let date6 = parseISO(1709332800000);
// Tue Jan 29 2024 15:00:00 GMT+0000
It has many handy functions like compareAsc()
, compareDesc()
, isAfter()
, isBefore()
, isEqual()
, and more. They give you a number or a true/false value to show the result. For example:
console.log(compareAsc(date1, date2)); // Output: -1
console.log(compareDesc(date1, date2)); // Output: 1
console.log(isAfter(date1, date2)); // Output: false
console.log(isBefore(date1, date2)); // Output: true
console.log(isEqual(date1, date2)); // Output: false
console.log(isSameDay(date1, date2)); // Output: true
console.log(isSameMonth(date1, date2)); // Output: true
console.log(isSameYear(date1, date2)); // Output: true
utcToZonedTime()
or zonedTimeToUtc()
to change the date objects to the time zone you want.Conclusion
In this blog post, you have learned how to compare dates in JavaScript using various methods and libraries. You have seen that comparing dates in JavaScript can be tricky, but also fun and expressive. You have learned how to compare dates without time, with time, by day, in different time zones, using natural language, relative time, or custom formats.
You have also learned how to use some of the most popular and useful libraries for working with dates in JavaScript, such as Moment.js, Day.js, and date-fns.
I hope you had fun reading this blog post and that you feel more confident and excited about comparing dates in JavaScript.