Do you want to learn what is string append in JavaScript? If you are new to JavaScript, you may find it confusing or challenging to join two or more strings together. You may wonder what is the best way to append strings, what are the different methods and operators available, and what are the common use cases and examples of appending strings.
In this blog post, I will show you:
- A brief introduction to strings in JavaScript
- The benefits of appending strings
- Different methods to appending strings
- How to add a comma between strings
- Append two or more strings in JavaScript
- How to insert strings as HTML elements
- How to create a new line after a string
- Repeat a string multiple times
By the end of this post, you will be able to append strings in JavaScript like a pro. Let’s get started!
What is a string in JavaScript?
A string is a sequence of characters enclosed by single or double quotes. For example, ‘Hello’ and “World” are both strings. JavaScript strings can store text, numbers, symbols, or any other data that can be represented as text.
Why append strings in JavaScript?
URLs
, HTML
elements, or anything else that uses text. You can also use it to change and shape data, like adding commas, new lines, or spaces. How cool is that?How to append strings in JavaScript?
There are several ways to append strings in JavaScript, depending on the situation and preference. Here are some of the most common methods and operators:
1. Append string using concatenation operator (+)
The string concatenation operator (+) is used to join two or more strings together. For example
let firstName = 'John';
let lastName = 'Doe';
let fullName = firstName + ' ' + lastName;
// Output: 'John Doe'
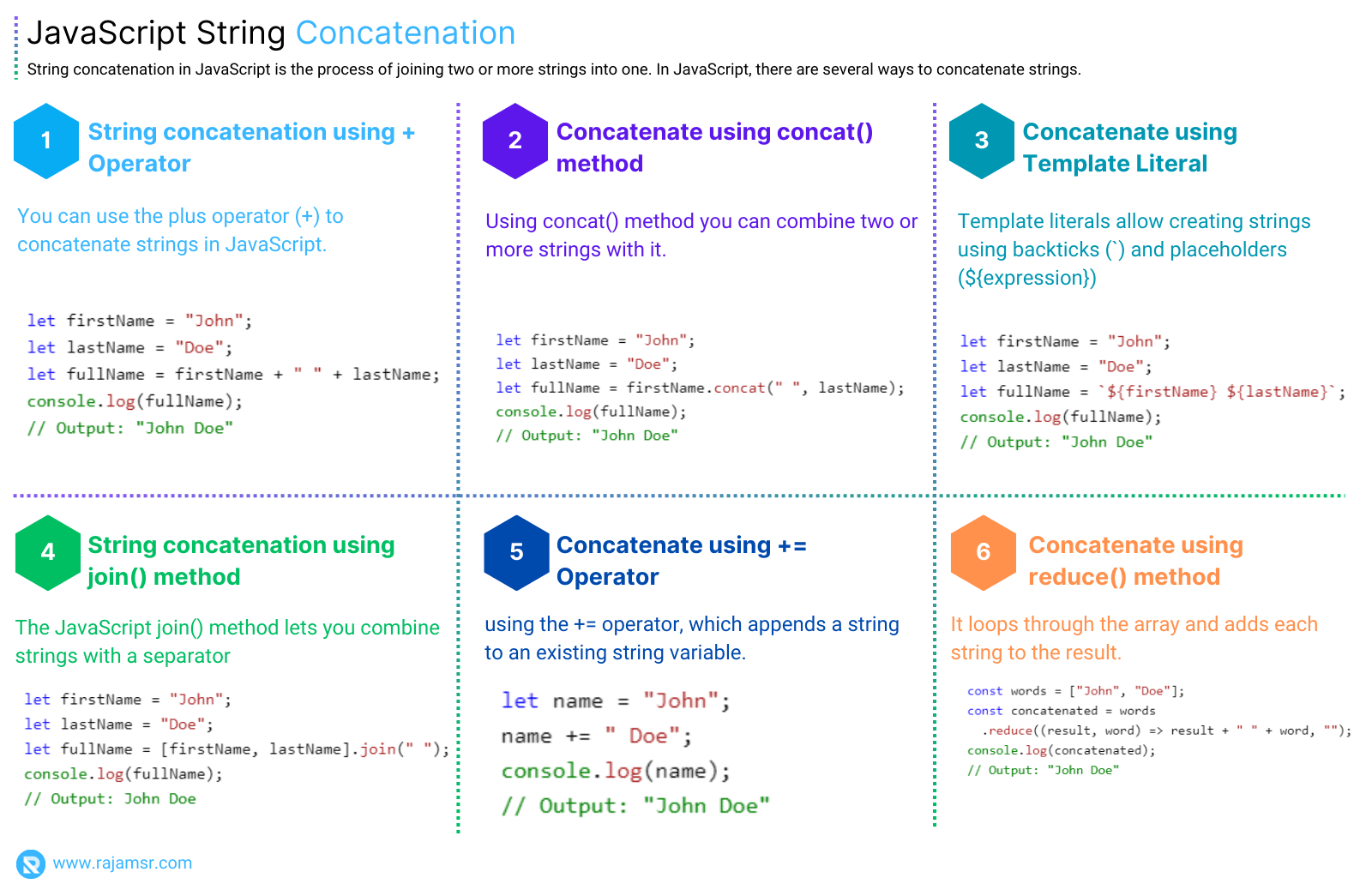
Did you know that you can use the concatenation operator to join different types of data in JavaScript? It’s like a magic glue that sticks things together! But be careful, sometimes the glue can get messy and change the shape of the things you are joining.
For example, if you try to concatenate numbers, booleans, or objects with strings, JavaScript will first turn them into strings and then add them. This might not be what you want. Look at this example:
let age = 30;
let message = 'I am ' + String(age) + ' years old'; // 'I am 30 years old'
let number = String(10) + '5'; // '105'
let boolean = String(true) + 'false'; // 'truefalse'
let object = String({ name: 'JavaScript' }) + ' is awesome!'; // '[object Object] is awesome!'
2. Append to string using template literals (``)
template literals
, and they are awesome! Template literals let you use backticks (`)
instead of quotes ("
) or apostrophes ('
) to make strings. But that’s not all. You can also put expressions inside the backticks, using the syntax ${expression}
. This way, you can insert JavaScript variables, calculations, functions, or anything else into our strings. For example:let firstName = 'John';
let lastName = 'Doe';
let fullName = `${firstName} ${lastName}`;
// Output: 'John Doe'
Template literals let you write JavaScript multiline strings, without having to use annoying escape characters. You can also use them to insert any JavaScript expression you want, like JavaScript math functions, or even conditionals. Here’s an example:
let value1 = 10;
let value2 = 5;
let result = `${value1} + ${value2} = ${value1 + value2}`;
// Output: '10 + 5 = 15'
let name = 'John';
let message = `Hello, ${name ? name : 'stranger'}`;
// Output: 'Hello, John'
let sayHello = () => 'Hello';
let greeting = `${sayHello()} JavaScript!`;
// Output: 'Hello JavaScript!'
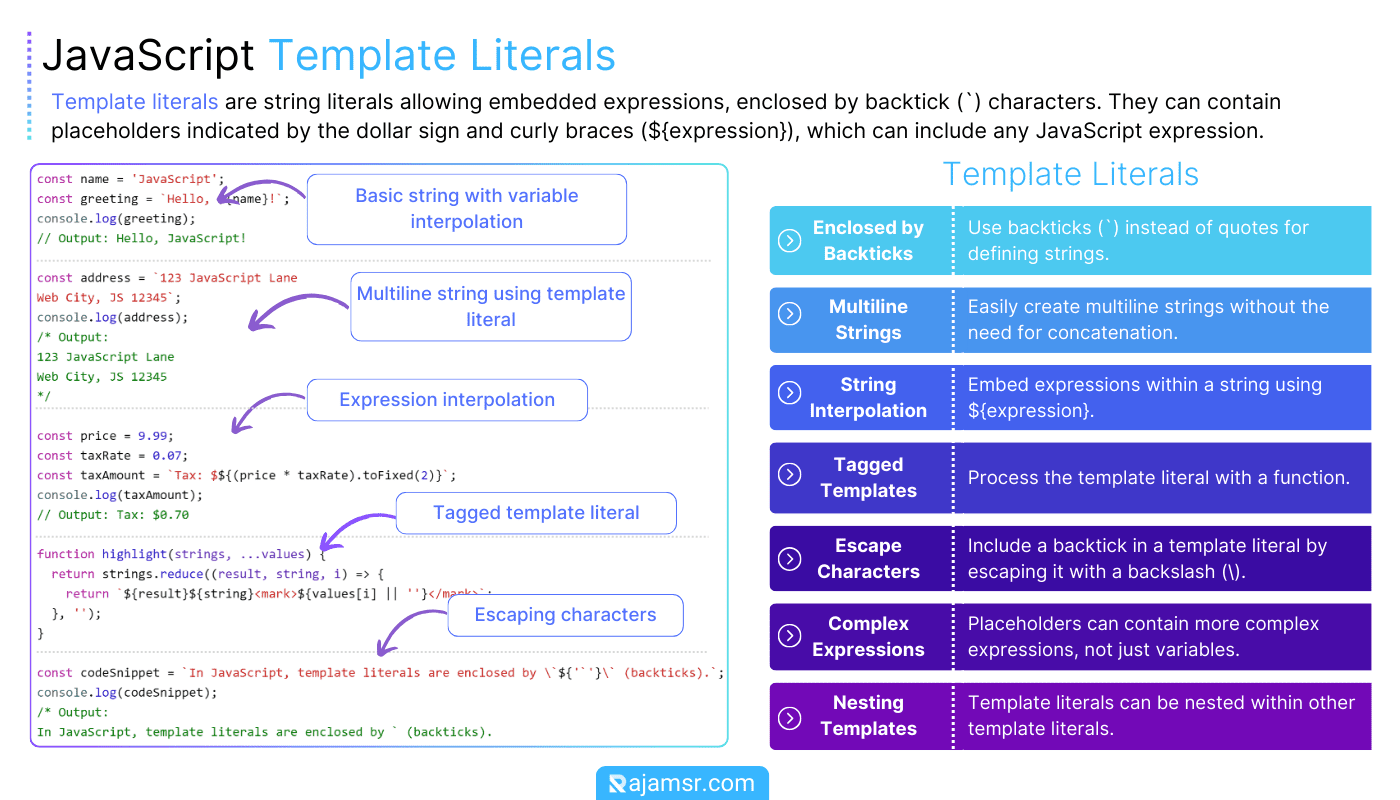
3. String append using the concat() method
concat()
method! This method is part of the String
object, and it creates a new string with the strings you give it. For example:let firstName = 'John';
let lastName = 'Doe';
console.log(firstName.concat(' ', lastName));
// Output: 'John Doe'
concat()
method works. It’s a very useful way to join strings together. You can give it as many arguments as you want, and they don’t have to be strings. You can also use numbers
, booleans
, or objects
. But be careful, because JavaScript will change them to strings before adding them to the end. Sometimes this might not be what you want, so watch out for surprises!4. Append using the join() method
Arrays are so versatile and powerful. JavaScript array methods make our lives easier. One of my favorites is the JavaScript join() method. It lets you turn an array into a string, and you can choose how to separate the elements. For example:
let tags = ['head', 'title', 'body'];
console.log(tags.join(', '));
// Output: 'head, title, body'
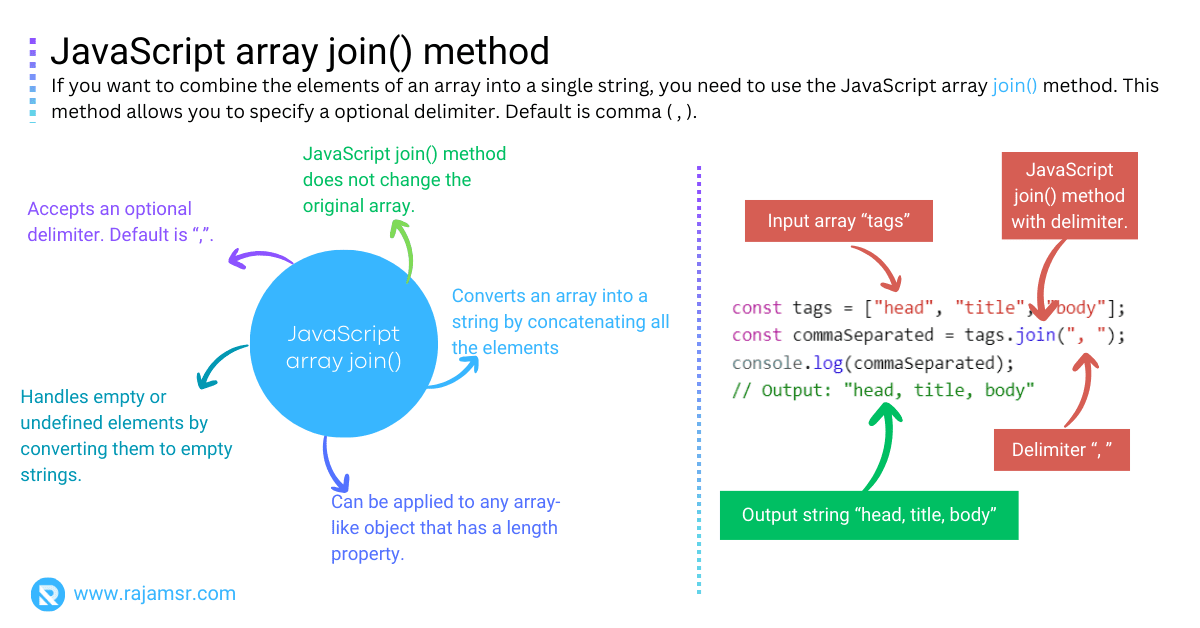
The join()
method lets you combine different pieces of text with a separator of your choice. You can use any string as the separator, even an empty one. You can also use join()
with values that are not strings, like numbers
, booleans
, or non-primitive data type objects. But be careful, because JavaScript will try to change these values into strings before adding them to the text. This might not work as you expect. Here is an example:
let numbers = [1, 2, 3];
let numberString = numbers.join('+'); // Output: '1+2+3'
let booleans = [true, false, true];
let booleanString = booleans.join(' and '); // Output: 'true and false and true'
let objects = [{name: 'Alice'}, {name: 'Bob'}];
let objectString = objects.join(' and '); // Output: '[object Object] and [object Object]'
Append string with comma
+
) to join the strings together. Another way is to use backticks (`
) and put the strings inside them. A third way is to use the join()
method on an array of strings.Here are some examples of how to do this:let firstName = 'John';
let lastName = 'Doe';
// 1. Append string with comma using the concatenation operator (+)
console.log(firstName + ', ' + lastName) // Output: 'John, Doe'
// 2. Append string with comma using template literals (``)
console.log(`${firstName}, ${lastName}`); // Output: 'John, Doe'
// 3. Append string with comma using the join() method
console.log([firstName, lastName].join(', ')); // Output: 'John, Doe'
Append string to string in JavaScript
If you want to append two strings together, you have some options. You can use different methods and operators that work for this purpose. Let’s see an example of how to do it:
let firstName = 'John';
let lastName = 'Doe';
// 1. Using the concatenation operator (+)
console.log(firstName + ' ' + lastName); // Output: 'John Doe'
// 2. Using the template literals (``)
console.log(`${firstName} ${lastName}`); // Output: 'John Doe'
// 3. Using the concat() method
console.log(firstName.concat(' ', lastName)); // Output: 'John Doe'
// 4. Using the join() method
console.log([firstName, lastName].join(' ')); // Output: 'John Doe'
John
and the last name Doe
are concatenated and printed with the full name John Doe
. You can use four different methods: the plus sign (+
), the backticks (`
), the concat()
method, and the join()
method.Append JavaScript string as HTML
HTML
element. Here are some ways to create a string with HTML
:let language = 'JavaScript';
let runsEverywhere = 'runs everywhere!';
let element = document.getElementById('header');
// 1. Using the concatenation operator (+)
let html = '<strong>' + language + '</strong>' + '<span> ' + runsEverywhere + '</span>';
element.innerHTML = html;
// Output: '<strong>JavaScript</strong><p>runs everywhere!</p>'
// 2. Using the template literal
html = `<strong> ${language} </strong> <span> ${runsEverywhere} </span>`;
element.innerHTML = html;
// Output: '<strong>JavaScript</strong><p>runs everywhere!</p>'
// 3. Using the template literal
html = '<strong>'.concat(language)
.concat('</strong> <span>')
.concat(runsEverywhere)
.concat('</span>');
element.innerHTML = html;
// Output: '<strong>JavaScript</strong><p>runs everywhere!</p>'
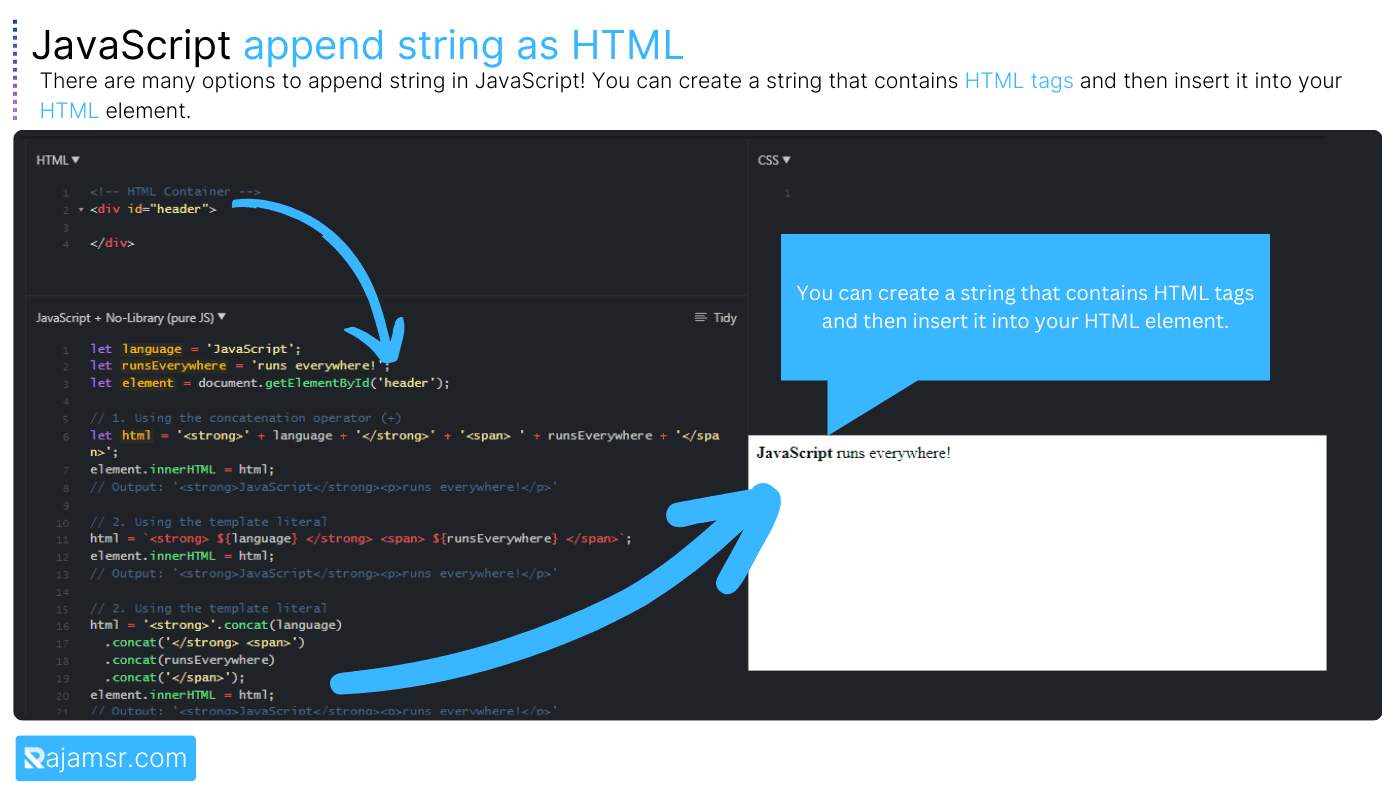
JavaScript append string with new line
new line
, we can use the concatenation operator (+
), the template literals (), or the join()
method, and then add the escape character \n
to indicate a new line. For example:let firstName = 'John';
let lastName = 'Doe';
// 1. Using the concatenation operator (+)
console.log(firstName + '\n' + lastName); // Output: 'John\nDoe'
// 2. Using the template literals (``)
console.log(`${firstName}\n${lastName}`); // Output: 'John\nDoe'
// 3. Using the join() method
console.log([firstName, lastName].join('\n')); // Output: 'John\nDoe'
Append JavaScript string N times
N
times, you can use string repeat()
method, or a loop to repeat the string N
times and then use any of the methods and operators mentioned above to append them. For example:let word = '-';
let n = 10;
// 1. Using the string repeat() method
console.log(word.repeat(n)); // Output: ----------
// 2. Using the concatenation operator (+)
let result = '';
for (let i = 0; i < n; i++) {
result = result + word;
}
console.log(result); // Output: ----------
// 3. Using the template literals (``)
result = '';
for (let i = 0; i < n; i++) {
result = `${result}${word}`; // Output: ----------
}
console.log(result);
// 4. Using the concat() method
result='';
for (let i = 0; i < n; i++) {
result = result.concat(word);
}
console.log(result); // Output: ----------
// 5. Using the join() method
result = '';
result = Array(n + 1).join(word);
console.log(result); // Output: ----------
In the above example, initializes a variable word
with a hyphen -
and another variable n
with the value 10
. It then demonstrates five different methods to create a string of 10
hyphens.
I used a single-character -
to repeat in this example, however, you can also use string.
Frequently asked questions
You can use the += operator to add a character to a string in JavaScript.
let js = "JavaScript"; js +='!'; console.log(js); // Output: "JavaScript!"
You can use the JavaScript push() method to append a string to an array in JavaScript.
let colors = ['Red', 'Green'];
colors.push('Blue');
console.log(colors);
// Output: ["Red", "Green", "Blue"]
You can concatenate a string to a variable using the += operator.
let message = "JavaScript runs";
message += " everywhere on everything";
console.log(message);
// Output: "JavaScript runs everywhere on everything"
Conclusion
In this blog post, you have learned how to append strings in JavaScript, which means adding one string to the end of another string.
I have also covered some common use cases and examples of appending strings with different methods and operators, such as the concatenation operator (+), the template literals (`), the concat() method, and the join() method.
I hope this post has been helpful and informative for you.