Have you ever wondered how to capitalize the first letter of a string in JavaScript? Maybe you want to make your text look more professional, or you need to follow some naming conventions. Whatever the reason, you can easily achieve this task with a few lines of code.
In this blog, I will show you:
- Make the first letter of each word uppercase in a string
- Convert the first letter of each word to uppercase in a string
- Use a template literal to make the first letter of each word uppercase in a string
- Transform the first letter of each word to uppercase in an array
Let’s look in detail.
Capitalize first letter in JavaScript
There is no built-in JavaScript function to capitalize the first letter of a JavaScript string. However, you can write custom functions to capitalize the first character.
In this post, let’s see how to capitalize the first letter of each word in a string with three different approaches.
Method 1: Capitalize first letter using charAt(), slice(), and toUpperCase() methods
This method involves using three built-in string methods. The charAt()
, slice()
, and JavaScript toUpperCase(). The charAt()
method returns the character at a specified index in a string. The JavaScript slice() method returns a substring of a string, starting from a given index and ending at another index (or the end of the string). The toUpperCase()
method returns a string with all lowercase letters converted to uppercase.
To capitalize the first letter of a string, you can use these methods as follows:
// Declare a messageing variable
let message = "javascript";
// Concatenate the first character and the rest of the messageing
let capitalizedmessage = message.charAt(0).toUpperCase() + message.slice(1);
// Log the result to the console
console.log(capitalizedmessage);
// Output: "Javascript"
Pros and Cons
This method is simple and easy to understand. It works for any string, regardless of its length or content. However, it has some drawbacks.
- First, it only capitalizes the first letter of the entire string, not each word in the string.
- If you want to capitalize the first letter of every word, you will need to use a loop or a different method.
Method 2: Capitalize first letter of each word using split() and join() methods
You can use the split()
and join()
methods to capitalize the initial letter of each word in a string. The split()
method separates a string into an array of substrings. Whereas, the join()
method combines an array of strings back together.
Here’s how to utilize these two methods to uppercase the first letter of each word in a string:
let textToCapitalize = "javascript runs anywhere, everywhere!";
let words = textToCapitalize.split(" ");
let capitalizedWords = words.map(word => word
.charAt(0)
.toUpperCase() + word.slice(1));
let capitalizedString = capitalizedWords.join(" ");
console.log(capitalizedString);
// "Javascript Runs Anywhere, Everywhere!"
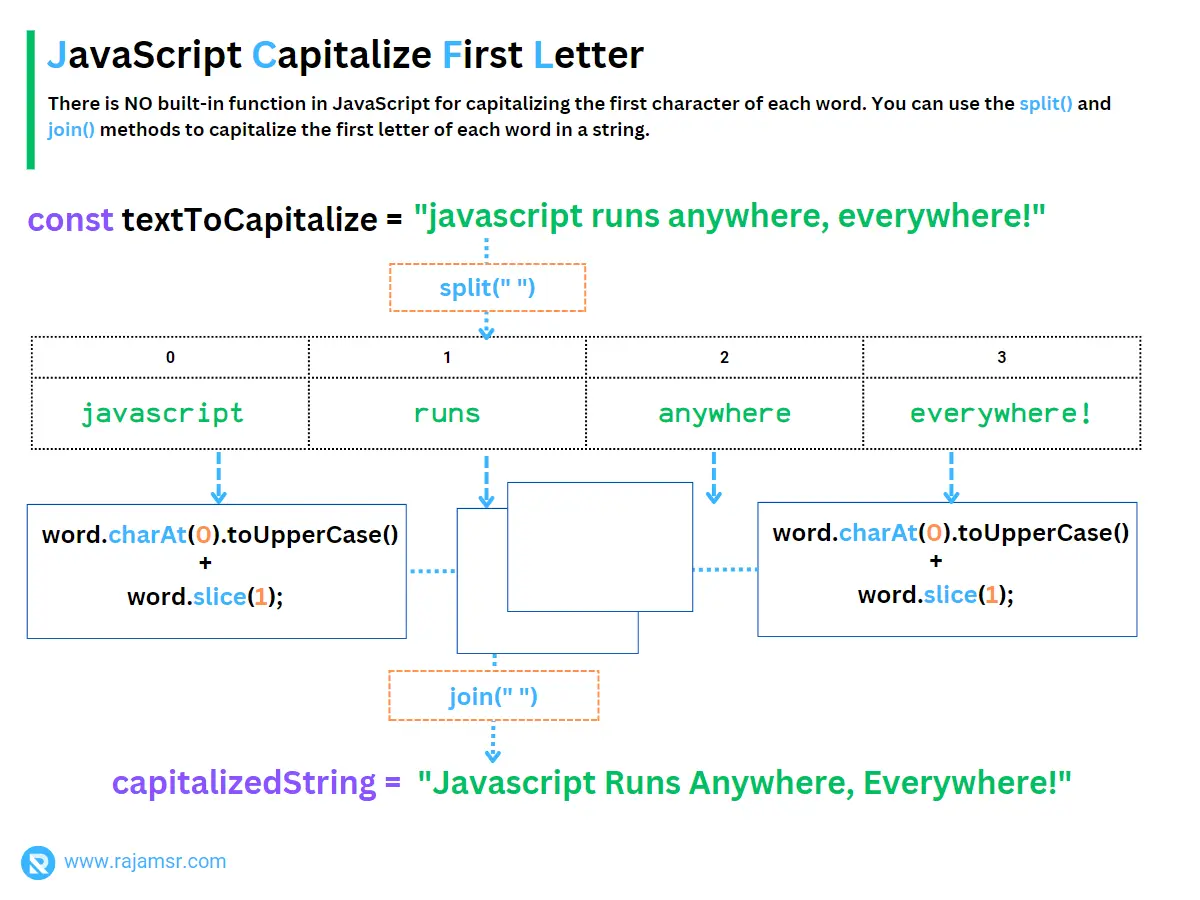
In this example, we first use the split()
method to split the original string into an array of words. The map()
JavaScript array methods loop through each word and convert it to the uppercase first letter.
Finally, we use the join()
method to reassemble the array of first-letter capitalized words into a single string.
Pros and Cons
This method is the most customizable and reusable one. You can write your own logic and rules to capitalize the first letter of a string. It is possible to modify the function to accept other parameters, such as a separator or a case option.
However, it has some drawbacks.
- It may be overkill for a simple task like capitalizing the first letter of a string.
- This approach may introduce errors if you do not write the function correctly or test it thoroughly.
Method 3: JavaScript first letter uppercase using regular expression
Alternatively, you can use a JavaScript regular expression and the replace()
method to capitalize the first of a string.
function capitalizeFirstLetter(inputString) {
return inputString.replace(/\b[a-z]/g, (letter) => { return letter.toUpperCase() });
}
let msg = "javascript runs anywhere, everywhere!";
let capitalizedWords = capitalizeFirstLetter(msg);
console.log(capitalizedWords);
// Output: "Javascript Runs Anywhere, Everywhere!"
Pros and Cons
This method is more flexible and powerful than the previous one. It can capitalize the first letter of every word in the string. Not just the first word. It also does not create any new variables, which can improve the performance and readability of your code.
However, it has some drawbacks.
- It requires some knowledge of regex syntax, which can be complex and confusing.
- This approach may not work for some special characters or languages that do not use spaces to separate words. You may need to adjust the regex pattern to suit your needs.
JavaScript capitalize words with different use cases
What if you simply want to capitalize the first letter of a word in a string rather than all words? You can use the following methods:.
1. Capitalize first letter of a string
The simplest way to capitalize the first letter of a string in JavaScript is to use the built-in toUpperCase()
combined with the JavaScript slice() method used to extract a portion of the string. This method can be used on a string variable or a string literal.
Here is an example of how to use this method:
let textToCapitalize = "hello world";
let capitalizedString = textToCapitalize
.charAt(0)
.toUpperCase() + myString.slice(1);
console.log(capitalizedString);
// Output: "Hello world"
In this case, we used the charAt()
method to retrieve the first character in a string. Then we used the toUpperCase()
method to capitalize just that character. The slice()
method is used to extract the remaining characters from the input string. The resulting text is saved in the JavaScript Variable capitalizedString
.
This approach, however, has a drawback in that it only works for single-letter strings; if you have a string of more than one letter, it will not function.
2. Capitalize the first letter of a string using template literal
In JavaScript, you may also capitalize the initial letter of a string by utilizing template literals and the slice()
method. This approach is a little shorter and easier to read than the previous one.
Here’s an example of how to use this method:
let textToCapitalize = "hello world";
let capitalizedString = `${textToCapitalize.slice(0,1)
.toUpperCase()}${textToCapitalize.slice(1)}`;
console.log(capitalizedString);
// "Hello world"
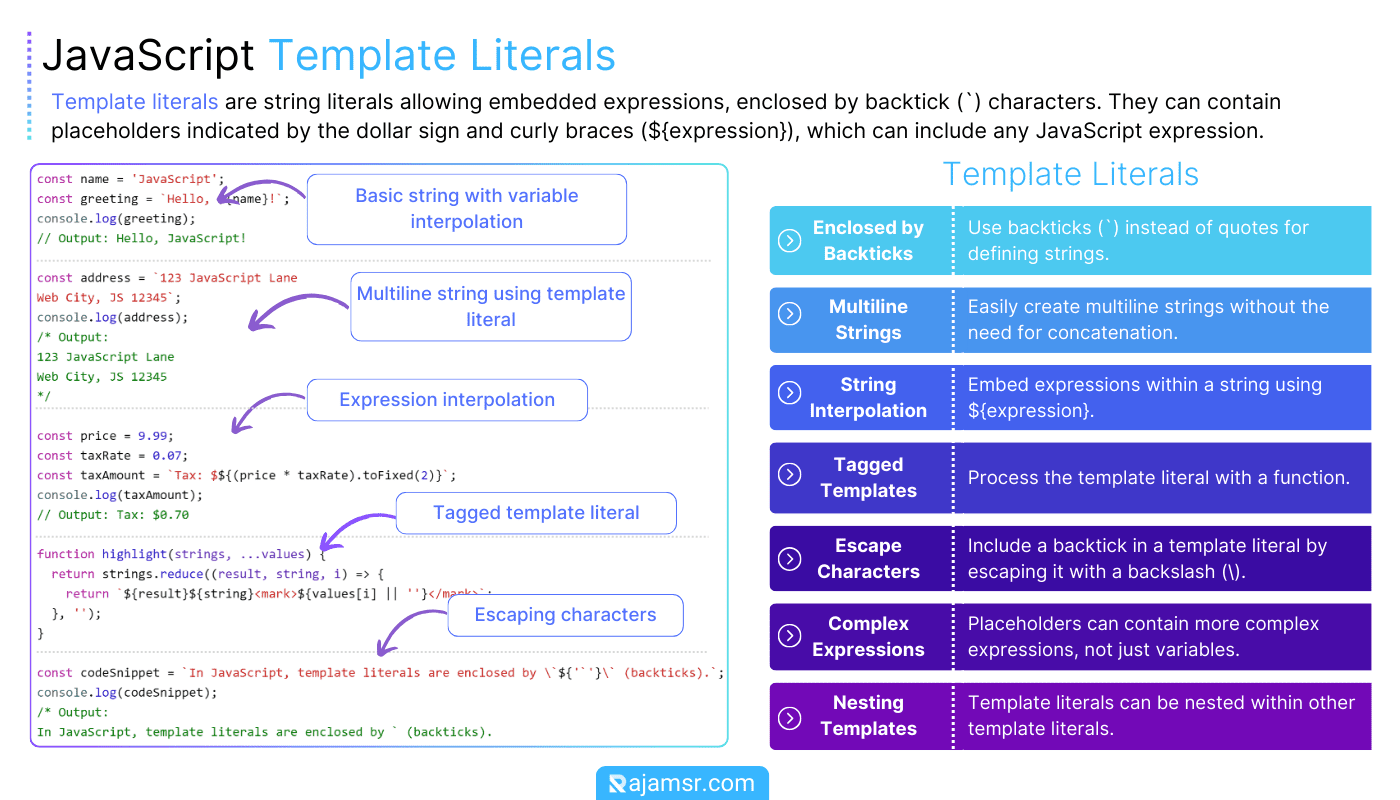
In this example, we use template literals to construct a new string. The string’s capitalized first letter is combined with the rest of the characters using JavaScript string concatenation.
This method is easier to read and less prone to errors than the prior one.
3. Capitalize first letter of each word in array
Let’s assume you have an array of strings and you want to capitalize the first letter of each string. Here is an example of how to do that:
const bookTitles = [
'eloquent javascript',
'javascript: the good parts',
'javascript for kids'];
let firstLetterCapitalizedTitles = bookTitles
.map(b => b.charAt(0).toUpperCase() + b.slice(1))
console.log(firstLetterCapitalizedTitles)
// Output: ["Eloquent javascript",
// "Javascript: the good parts", "Javascript for kids"]
In the above code, the map()
method will iterate through each string book title
, capitalize the first letter of each string to uppercase, and leave the rest of the string. The first-letter capitalized string returns as a new string.
However, if you want to capitalize each word in a book title, you can change line #6
in the above code. This list will split the book title into an array of words and convert the first letter of each word to a capitalized string.
let firstLetterCapitalizedTitles = bookTitles
.map(b => b.split(' ')
.map(w => w.charAt(0).toUpperCase() + w.slice(1)).join(' '))
/* Output:
[""Eloquent Javascript"", "Javascript: The Good Parts",
"Javascript For Kids"]
*/
JavaScript string capitalize all letters
What if you want to capitalize all words in a string using JavaScript? There is no capitalize()
method in JavaScript. However, you can use the built-in string function toUpperCase()
to convert a JavaScript uppercase string, as shown in the example below:
// Capitalize all letters
let textToCapitalize = "hello world";
console.log(textToCapitalize.toUpperCase());
// Output: "HELLO WORLD"
You can use toLowerCase()
method to convert all characters in a string to JavaScript lowercase.
// javascript lowercase string
let textToLowerCase = "HELLO WORLD"
console.log(textToCapitalize.toLowerCase());
// Output: "hello world"
Conclusion
In this post, you learned three different methods to capitalize the first letter of a string in JavaScript, and how to choose the best one for your situation. You also learned how to use charAt()
, slice()
, toUpperCase()
, regex, and custom functions to manipulate strings in JavaScript. Now you can make your text look more professional and follow the naming conventions with ease.
As described in this post, you must create a custom method to capitalize the text’s word or the first letter in each word.
The simple answer for JavaScript capitalizing the first letter of each word in a string is to use split()
and join()
.
Over to you, which approach will you try to capitalize the first letter?