Are you tired of inconsistent casing in your JavaScript code? Want to standardize your strings to lowercase? Look no further than our comprehensive guide to JavaScript lowercase! With the toLowerCase()
method, you can easily convert your strings to lowercase and improve the readability and consistency of your code.
In this article, we’ll explore:
- What is a lowercase JavaScript string?
- Why you have to use toLowerCase() method?
- Four string lowercase examples
- Convert string to lowercase except for the first character
- What is the difference between
toLowerCase()
andtoLocaleLowerCase()
? - How to safely handle the lowercase conversion, and more.
Let’s dive deep.
What is the JavaScript toLowerCase() method?
The toLowerCase() method is a built-in function in JavaScript that returns a new string with all the characters converted to lowercase. This method does not change the original string, but creates a new one.
The syntax of the toLowerCase() method is:
string.toLowerCase()
string
is any JavaScript string value. The toLowerCase()
method does not take any parameters, and it returns the lowercase version of the string.Why use the JavaScript lowercase method toLowerCase()?
toLowerCase()
method can be useful for various purposes, such as:Normalizing user input
You can use the toLowerCase()
method to convert user input to lowercase, so that you can compare it with other values or store it in a consistent format.
For example, if you want to check if a user entered a valid email address, you can use the toLowerCase()
method to make sure that the email address is in lowercase before validating it.
Searching and matching strings
You can use the toLowerCase()
method to perform case-insensitive searches and matches on strings.
For example, if you want to find all the occurrences of a word in a text, you can use the toLowerCase()
method to convert both the word and the text to lowercase, and then use the JavaScript indexOf() or JavaScript includes() methods to search for the word.
Formatting and displaying strings
You can use the toLowerCase()
method to format strings in a certain way.
For example, if you want to display a user’s name in lowercase, you can use the toLowerCase()
method to convert the name to lowercase before displaying it.
How to use the toLowerCase() method in JavaScript?
To use the toLowerCase()
method, you need to have a string value that you want to convert to lowercase. You can then call the toLowerCase()
method on the string, and assign the result to a new variable or use it directly.
Here are some examples of how to use the toLowerCase()
method in JavaScript:
// Example 1: Convert a string literal to lowercase
let greeting = "Hello World!";
let lowerGreeting = greeting.toLowerCase();
console.log(lowerGreeting); // Output: hello world!
// Example 2: Convert a string variable to lowercase
let name = "John";
let lowerName = name.toLowerCase();
console.log(lowerName); // // Output: john
// Example 3: Convert a string expression to lowercase
let fullName = "John" + " " + "Doe";
let lowerFullName = fullName.toLowerCase();
console.log(lowerFullName); // Output:john doe
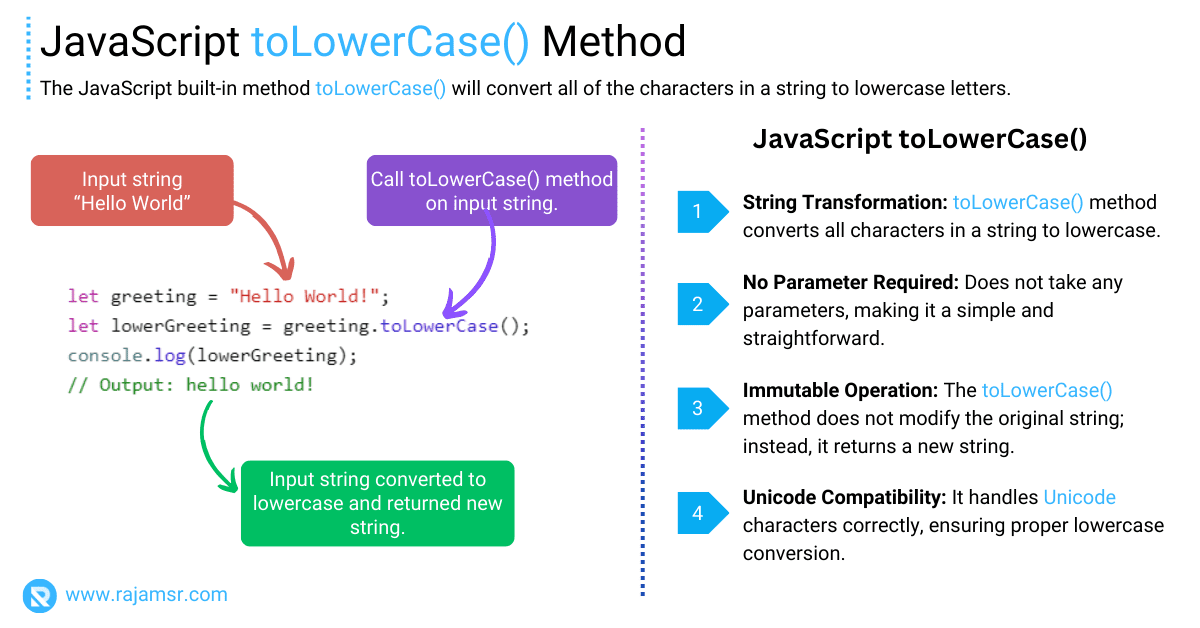
toLowerCase()
method converts all the uppercase letters in the string to lowercase letters, and leaves the rest of the characters unchanged.JavaScript lowercase string different use cases
Let’s see how to make JavaScript string lowercase with different use cases.
1. JavaScript lowercase string using toLowerCase()
toLowerCase()
string method is a built-in method in JavaScript that can convert a string to all lowercase and return a new string. The method can be applied to any string in JavaScript and will return a new string with all the characters in lowercase. The original string remains unchanged.Here is an example of how to use the toLowerCase()
method in JavaScript to lowercase all text:let textToConvert = "JAVASCRIPT IS AWESOME!";
let lowerCaseString = textToConvert.toLowerCase();
console.log(lowerCaseString);
// Output: "javascript is awesome!"
In the above example, the string is converted to lowercase using toLowerCase()
string method.
2. JavaScript Lowercase all but first letter
Assume you have a string with all of the text in capital letters. What if you only want to change the first letter of the string to lowercase?
Here is an example of how to use the toLowerCase()
in JavaScript:
let textToConvert = "JAVASCRIPT IS AWESOME!";
let lowerCaseString = textToConvert
.charAt(0)
.toUpperCase() + textToConvert.slice(1).toLowerCase();
console.log(lowerCaseString);
// Output: "Javascript is awesome!"
The chartAt(0)
method returns the first character from a string. Using the toUpperCase()
JavaScript method, you can convert to upper case. The JavaScript slice() method will return a string, starting from the specified index to the end of the string. Using the toLowerCase()
method, you can convert it into lowercase.
3. JavaScript lowercase all except first letter of each word
You may need to convert a string to lowercase in JavaScript, except for the first character of each word in the string. This is known as Title Case
conversion. Here’s an example of converting a string to a Title Case
:
let textToConvert = "JAVASCRIPT IS AWESOME!"
let words = textToConvert.split(' ');
let titleCase = words.map(w=> w.charAt(0).toUpperCase() + w.slice(1).toLowerCase())
console.log(titleCase.join(' '));
// Output: "Javascript Is Awesome!"
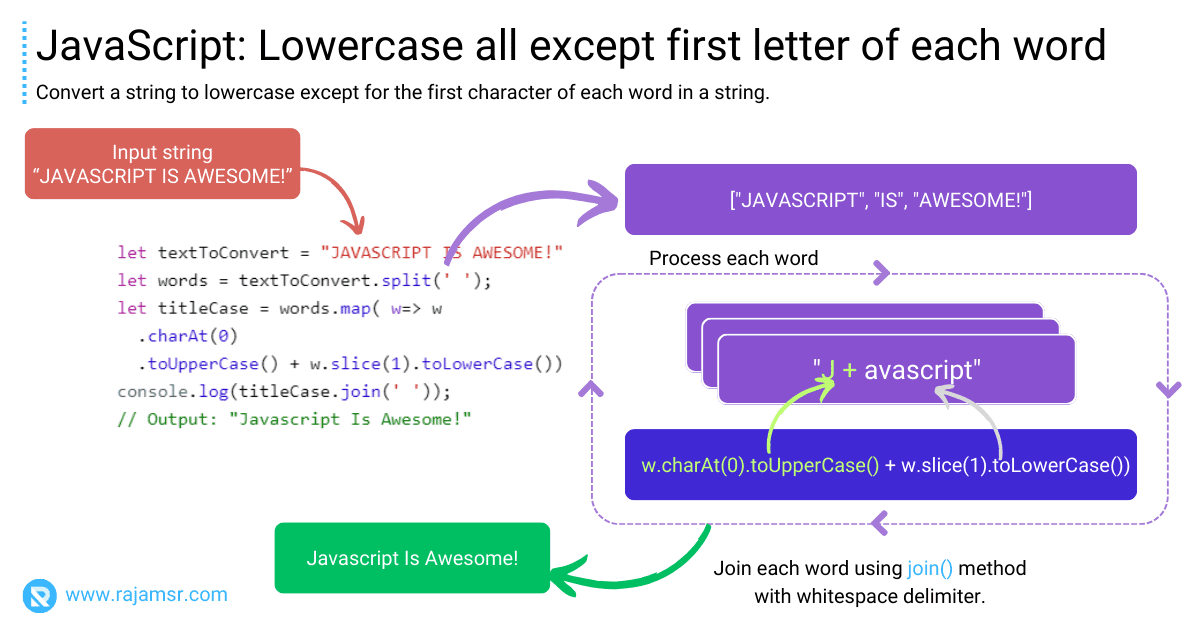
The split()
function divides strings into word arrays. Using the JavaScript array method map(), change the first letter of each word to upper case and the remainder of the characters to lower case. Finally, join all of the phrases using the JavaScript join() method.
4. JavaScript lowercase first letter
You can use the toLowerCase()
and toLocaleLowerCase()
methods to convert a string to lowercase. Here’s an example of a lowercase first letter:
let textToConvert = "JAVASCRIPT IS AWESOME!";
let lowerCaseString = textToConvert
.charAt(0)
.toLowerCase() + textToConvert.slice(1);
console.log(lowerCaseString);
// Output: "jAVASCRIPT IS AWESOME!"
How the JavaScript toLowerCase() works with different types of characters?
The toLowerCase()
method works with any type of character that has a lowercase equivalent, such as letters, numbers, symbols, or accented letters. However, some characters may behave differently than you expect.
1. Letters
toLowerCase()
method converts all the uppercase letters in the English alphabet (A-Z)
to their lowercase counterparts (a-z). For example, "JAVA"
becomes "java"
.let language = "JAVA";
console.log(language.toLowerCase()); // Output: "java"
let greekAlpha = `ΒΙΝΓ`;
console.log(greekAlpha.toLowerCase()); // Output: "βινγ"
2. Numbers
toLowerCase()
method does not change the case of numbers
, since they do not have a lowercase equivalent. For example, "123"
remains "123"
.However, the toLowerCase()
method does affect the case of some symbols that are used with numbers, such as the hexadecimal digits (A-F) or the scientific notation (E). For example, "0xFF"
becomes "0xff"
, and "1.23E4"
becomes "1.23e4"
.let number1 = 123;
console.log(String(number1).toLowerCase()); // Output: "123"
let number2 = '1.23E4';
console.log(number2.toLowerCase()); // Output: "1.23e4"
3. Symbols
toLowerCase()
method does not change the case of most symbols, such as punctuation marks, operators, or brackets. For example, "!@#$%^&*()"
remains "!@#$%^&*()"
.let symbols1 = "!@#$%^&*()";
console.log(symbols1.toLowerCase()); // Output: "!@#$%^&*()"
4. Accented Letters
toLowerCase()
method converts all the uppercase accented letters in the Latin alphabet (À-Ý)
to their lowercase counterparts (à-ý)
. For example, "ÉTÉ"
becomes "été"
.let greek = "ÉTÉ"; // Greek letters
let lowerGreek = greek.toLowerCase(); // Output: "été"
How the toLowerCase() method differs from other similar methods
The toLowerCase()
and toLocaleLowerCase()
methods are used for converting a string to lowercase. However, there is a difference:
JavaScript toUpperCase() method
toLowerCase()
method. For example, "javascript"
becomes "JAVASCRIPT"
const greet = "hello, javascript!";
const upperCaseGreet = greet.toUpperCase();
console.log(upperCaseGreet);
// Output: "HELLO, JAVASCRIPT!"
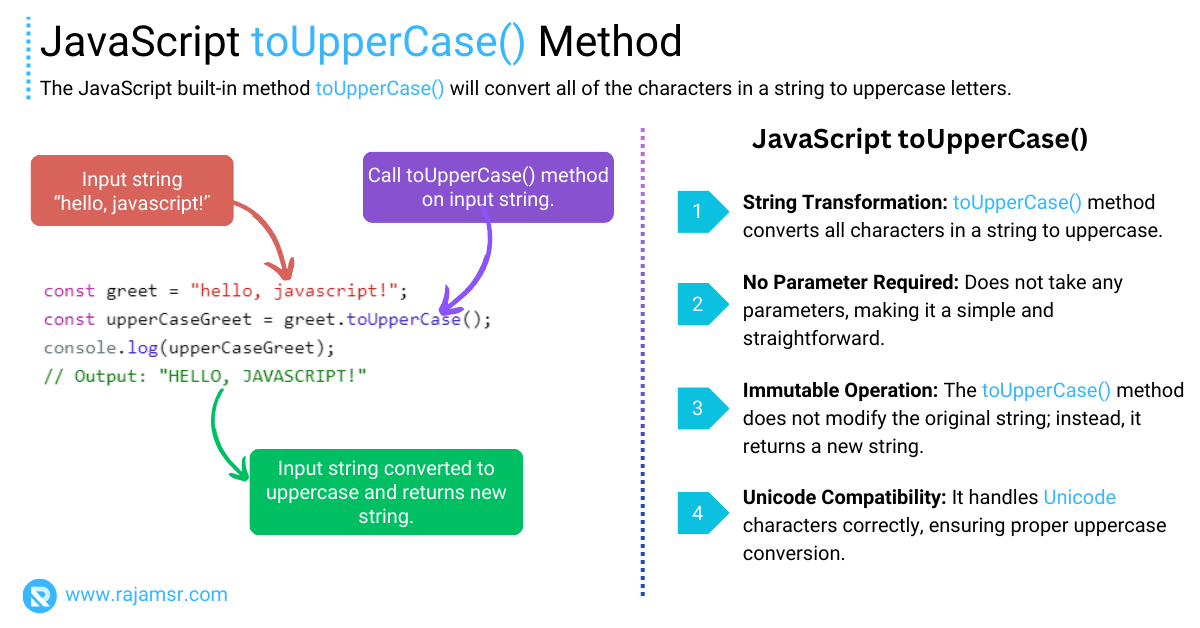
JavaScript toLocaleLowerCase() method
The JavaScript toLocaleLowerCase()
method converts a string to lowercase based on the locale-specific
settings, which might result in different lowercase mappings than the Unicode standard. Let’s take the Turkish word "DINÇ"
and use both methods to convert it to lowercase and see the difference.
let textToConvert = "DINÇ";
console.log(textToConvert.toLowerCase());
// Output: "dinç"
console.log(textToConvert.toLocaleLowerCase('tr'));
// Output: "dınç"
To customize the languages on the browser, go to the settings
page and select the ones you want. The language
you prefer most should be at the top of the list. There is no need to hard-code Turkish (tr)
as your language of choice. You can access it from the languages property of the navigator
object.
let textToConvert = "DINÇ";
console.log(textToConvert.toLocaleLowerCase(navigator.languages[0]));
// Output: "dınç"
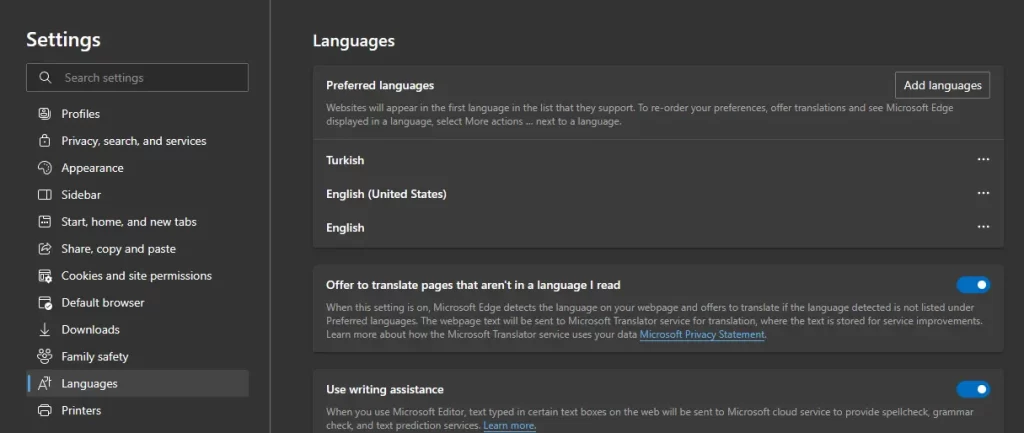
Troubleshoot toLowerCase() is not a function error
"toLowerCase is not a function"
or "toUpperCase is not a function"
when you try to use the toLowerCase()
or toUpperCase()
methods on non-primitive data types. These methods only work on strings
, and use them on other data types like objects
, arrays
, booleans
, or numbers
will result in an error.let textToConvert = [1,2,3]
console.log(textToConvert.toLowerCase());
// Output: TypeError: textToConvert.toLowerCase is not a function
To avoid this issue, use the JavaScript typeof() operator to determine the data type to ensure that the input type is a string before calling the toLowerCase()
or toUpperCase()
methods. The typeof()
operator will return the input type as a string. The following code will just output the message to the console and will not throw any exceptions if the input type is not a string.
let textToConvert = 'JAVASCRIPT'
if(typeof(textToConvert)==='string')
{
console.log(textToConvert.toLowerCase());
}
else{
console.log(`Input is not string type: ${typeof(textToConvert)}`)
}
Tips and best practices for using the toLowerCase() method
There are some tips and best practices that you should follow to use this method effectively and avoid errors or bugs. Here are some of them:
Check the type of the input: The toLowerCase()
method only works with strings. If you pass a non-string value, such as a number
, a boolean
, or an object
, you will get an error. To avoid this, you should check the type of the input before using the toLowerCase()
method, or use the String()
function to convert the input to a string. For example:
let input = 10;
// let lowerInput = input.toLowerCase();
// Error: input.toLowerCase is not a function
let lowerInput = String(input).toLowerCase(); // Output: "10"
Handle errors or exceptions: The toLowerCase()
method may throw an error or an exception if the input is null
or undefined, or if the method is not supported by the browser or the environment. To handle these cases, you should use a try...catch
block or a if...else
statement to catch the error or the exception, and provide an alternative solution or a fallback value.
For example:
try {
let input = null;
// Error: Cannot read property 'toLowerCase' of null
let lowerInput = input.toLowerCase();
} catch (error) {
console.error(error);
// Do something else
}
Use regular expressions: The toLowerCase()
method can only convert the entire string to lowercase. If you want to convert only a part of the string, or perform more complex transformations, such as replacing, removing, or inserting characters, you should use regular expressions. Regular expressions are patterns that can match, search, or manipulate strings in various ways. You can use the replace()
method with a regular expression to convert a string to a lowercase.
Here is an example of conditionally converting string to lowercase using regex:
// Convert only the first letter to lowercase
let lowerInput = "Hello World".replace(/^[A-Z]/, (match) => match.toLowerCase());
console.log(lowerInput);
// Output: "hello World"
// Convert only the vowels to lowercase
let lowerInput2 = "JAVASCRIPT".replace(/[AEIOU]/g, (match) => match.toLowerCase());
console.log(lowerInput2);
// Output: "JaVaSCRiPT"
JavaScript lowercase - frequently asked questions
toLowerCase()
method on a string to convert to lowercase.You can convert a lowercase string to uppercase using the toUpperCase()
method, which is a built-in method of the String object.
var lowercaseString = "hello world"; var uppercaseString = lowercaseString.toUpperCase(); console.log(uppercaseString); // Output: "HELLO WORLD"
You can check if a string is entirely in uppercase or lowercase in JavaScript by comparing it with its lowercase and uppercase versions.
Here’s how you can do it:
function isUppercase(str) { return str === str.toUpperCase(); } function isLowercase(str) { return str === str.toLowerCase(); } var uppercaseString = "HELLO WORLD"; var lowercaseString = "hello world"; console.log(isUppercase(uppercaseString)); // Output: true console.log(isUppercase(lowercaseString)); // Output: false console.log(isLowercase(uppercaseString)); // Output: false console.log(isLowercase(lowercaseString)); // Output: true
You can use the map()
function along with the toLowerCase()
method for strings. Here’s how you can do it:
var originalArray = ["Hello", "World", "JavaScript"]; var lowercaseArray = originalArray.map(function(str) { return str.toLowerCase(); }); console.log(lowercaseArray); // Output: ["hello", "world", "javascript"]
Conclusion
In conclusion, using JavaScript lowercase is a powerful method that can greatly improve the readability and consistency of your code. By converting your strings to lowercase, you can avoid confusion and easily identify matching values.
With the help of this comprehensive guide and real-world examples, you should now have a solid understanding of how to implement lowercase in your own code.
In addition to converting a JavaScript string to lowercase, you learned about how to use toLowerCase() in various scenarios and the distinction between the toLowerCase() and toLocaleLowerCase() methods. How to fix a case conversion problem and the difference between lowercase and uppercase JavaScript strings!